目录
一、分隔链表
用两个list存一下小于和大于等于 x的节点
最后串起来就行
java
public ListNode partition(ListNode head, int x) {
ListNode ret = new ListNode(1);
ListNode cur = ret;
List<ListNode> small = new ArrayList<>();
List<ListNode> big = new ArrayList<>();
List<ListNode> equal = new ArrayList<>();
while(head != null){
if(head.val < x){
small.add(head);
} else if(head.val >= x){
big.add(head);
}
head = head.next;
}
for(int i = 0;i < small.size();i++){
cur.next = small.get(i);
cur = cur.next;
}
for(int i = 0;i < big.size();i++){
cur.next = big.get(i);
cur = cur.next;
}
if(big.size() > 0){
big.get(big.size() - 1).next = null;
} else if(small.size() > 0){
small.get(small.size() - 1).next = null;
}
return ret.next;
}
二、旋转链表
题目意思就是每次把末尾最后一个放到最左边
可以用list集合存一下 每次去掉最后一个,并把对其头插
需要考虑一下k特别大的情况 取余一下
java
public ListNode rotateRight(ListNode head, int k) {
List<ListNode> list = new LinkedList<>();
ListNode ret = new ListNode(1);
ListNode cur = ret;
while(head != null){
list.add(head);
head = head.next;
}
if(list.size() > 0){
k = k % list.size();
}
while(k-- != 0){
if(list.size() > 0){
ListNode first = list.get(list.size() - 1);
list.remove(list.size() - 1);
list.addFirst(first);
}
}
for(int i = 0;i < list.size();i++){
cur.next = list.get(i);
cur = cur.next;
}
cur.next = null;
return ret.next;
}
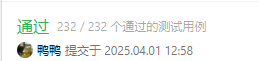
三、删除链表中重复的数字
用map记录一下,哪些是重复数字,哪些不是重复数字
java
public ListNode deleteDuplicates(ListNode head) {
Map<Integer,Boolean> map = new HashMap<>();
ListNode cur = head;
while(cur != null){
if(map.containsKey(cur.val)){
map.put(cur.val,false);
}else{
map.put(cur.val,true);
}
cur = cur.next;
}
cur = head;
ListNode ret = new ListNode(1);
ListNode curRet = ret;
while(cur != null){
if(map.get(cur.val)){
curRet.next = cur;
curRet = curRet.next;
}
cur = cur.next;
}
curRet.next = null;
return ret.next;
}
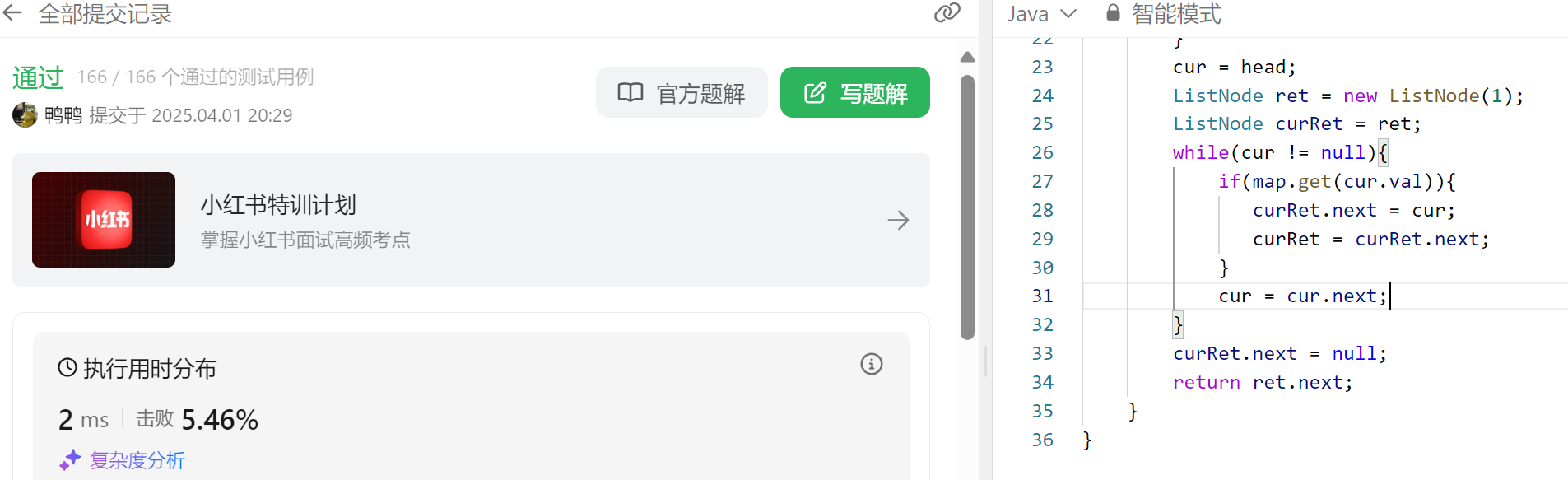