引入thymeleaf
介绍
Thymeleaf 是一款用于 Web 和独立环境 的现代化服务器端 Java 模板引擎,主要用于生成动态 HTML 页面、电子邮件、XML 等。它的核心设计目标是提供优雅且自然的模板语法,同时保持与静态 HTML 的高度兼容性,使前端开发人员可以直接在浏览器中预览模板效果,而无需依赖后端服务。
我们的商城系统本应该也是前后端分离的,就像后台管理系统那样,然而出于教学考虑,前后端分离的话就会屏蔽掉很多细节,所以我们进行服务端的页面渲染式开发(有点儿类似freemarker)
动静分离:nginx在后面部署的时候,我们可以将微服务中的页面的静态资源部署到nginx中。分担微服务的压力。
静指的是:图片、js、css等静态资源(以实际文件存在的方式)
每一个微服务只来管理自己的页面,最终做到每一个微服务都可以独立部署、运行、升级。
每个微服务都是独立自治的,每一个微服务的数据库、技术都是自治的。不一定商品服务用java开发,用php、js都可以,无论是从技术层面、架构层面还是业务都是独立自治的。
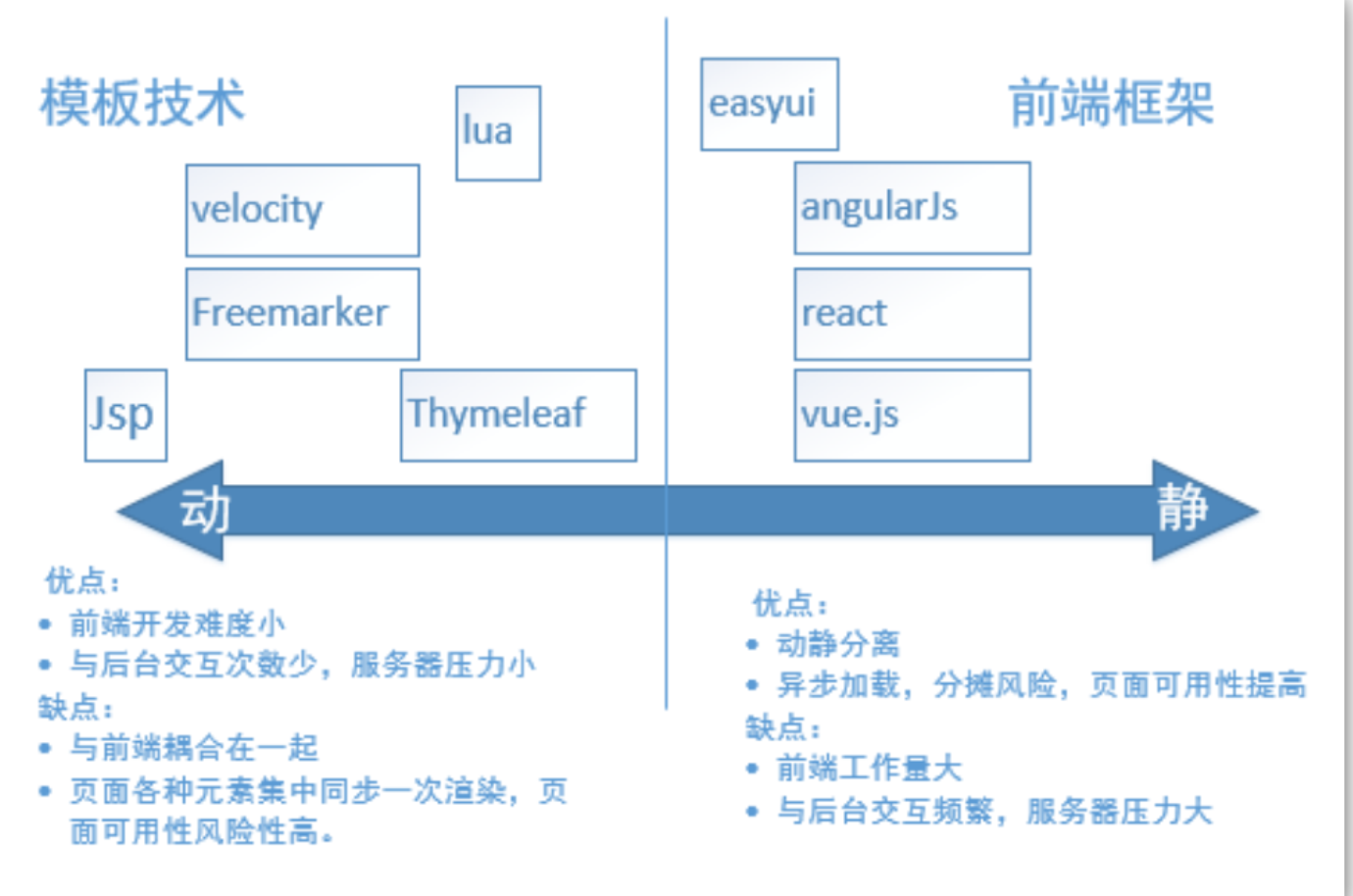
引入
导入依赖:
xml
<!--模板引擎-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
修改application.yml文件
yml
spring:
thymeleaf:
cache: false #关闭缓存,为了在生产环境下可以实时看到数据
mvc:
static-path-pattern: /static/** # 加载不出样式文件时需要加上这个
将静态资源放到static目录下,同时页面html文件放入到templates文件夹下面去,因为spring boot访问项目的时候默认会找index.html
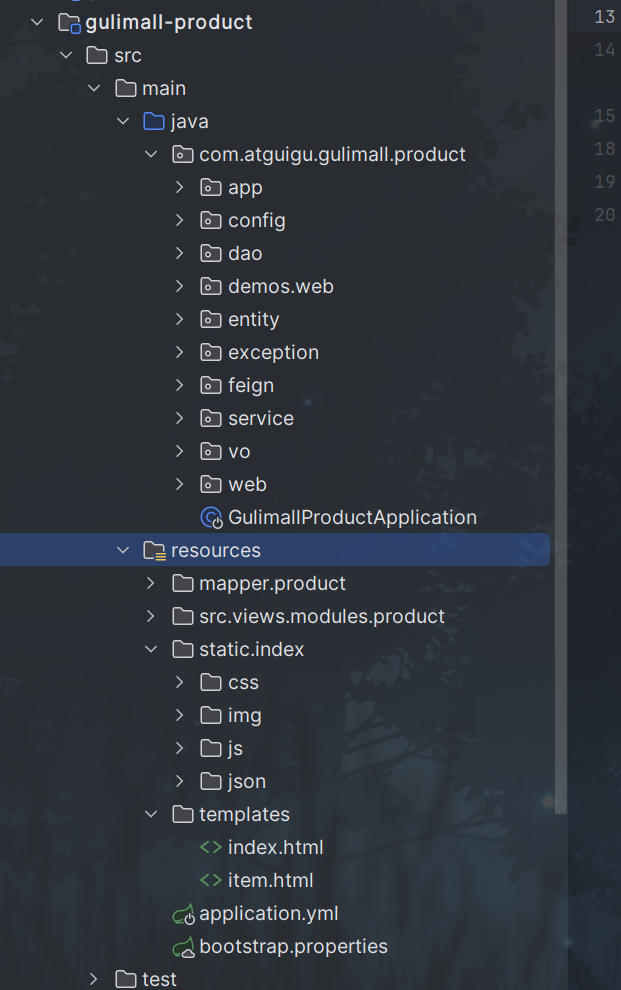
静态资源的访问
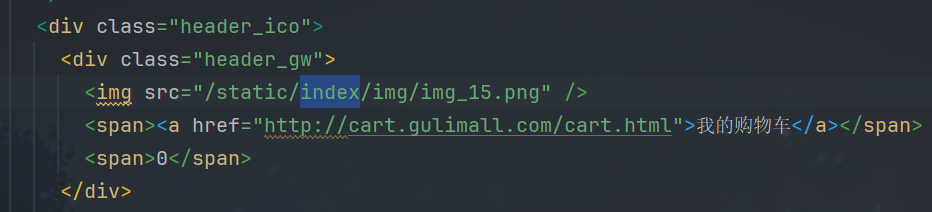
引入dev-tools依赖,使得修改后不重启服务器实时更新
xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
商城一级分类渲染
java
package com.atguigu.gulimall.product.web;
import com.atguigu.gulimall.product.entity.CategoryEntity;
import com.atguigu.gulimall.product.service.CategoryService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import java.util.List;
@Controller
public class IndexController {
@Autowired
CategoryService categoryService;
@GetMapping({"/","/index.html"}) // 配置访问localhost:10000/或者localhost:10000/index.html来到首页的映射
public String indexPage(Model model){ // 查出一级类目,使用Model对象封装
// 1.查出所有1级分类
List<CategoryEntity> entities = categoryService.getLevel1Categorys();
// 返回视图地址
// 使用视图解析器进行拼串
model.addAttribute("categories",entities);
return "index";
}
}
@Override
public List<CategoryEntity> getLevel1Categorys() {
return baseMapper.selectList(new QueryWrapper<CategoryEntity>().eq("parent_cid",0));
}
商城二三级分类渲染
修改js文件,使得页面不在获取静态数据
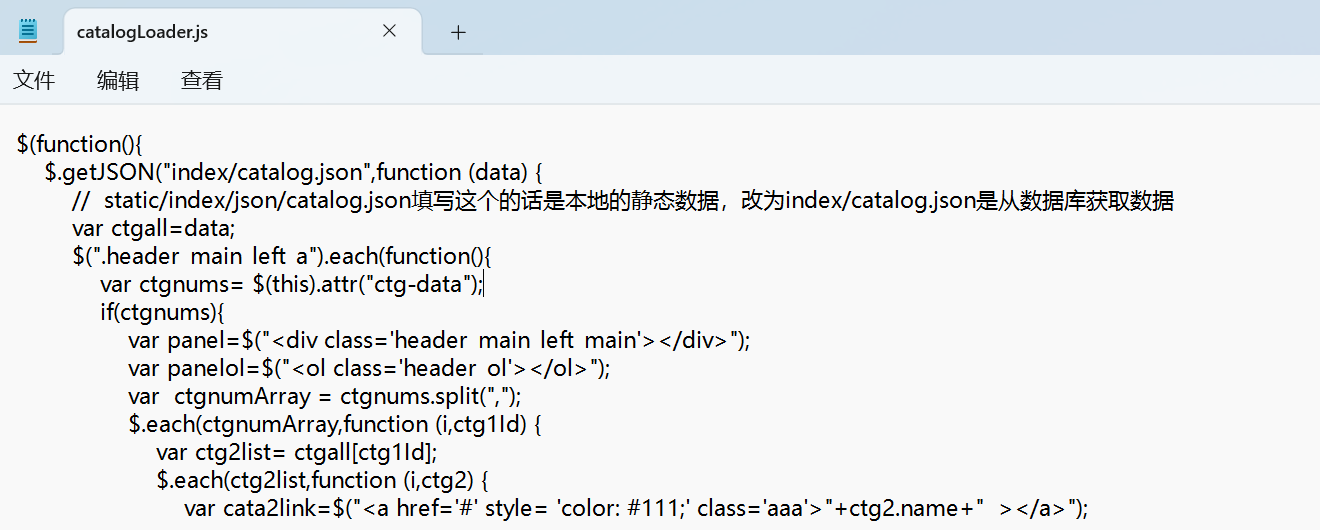
根据提供的静态json数据文件封装对应的接口返回类
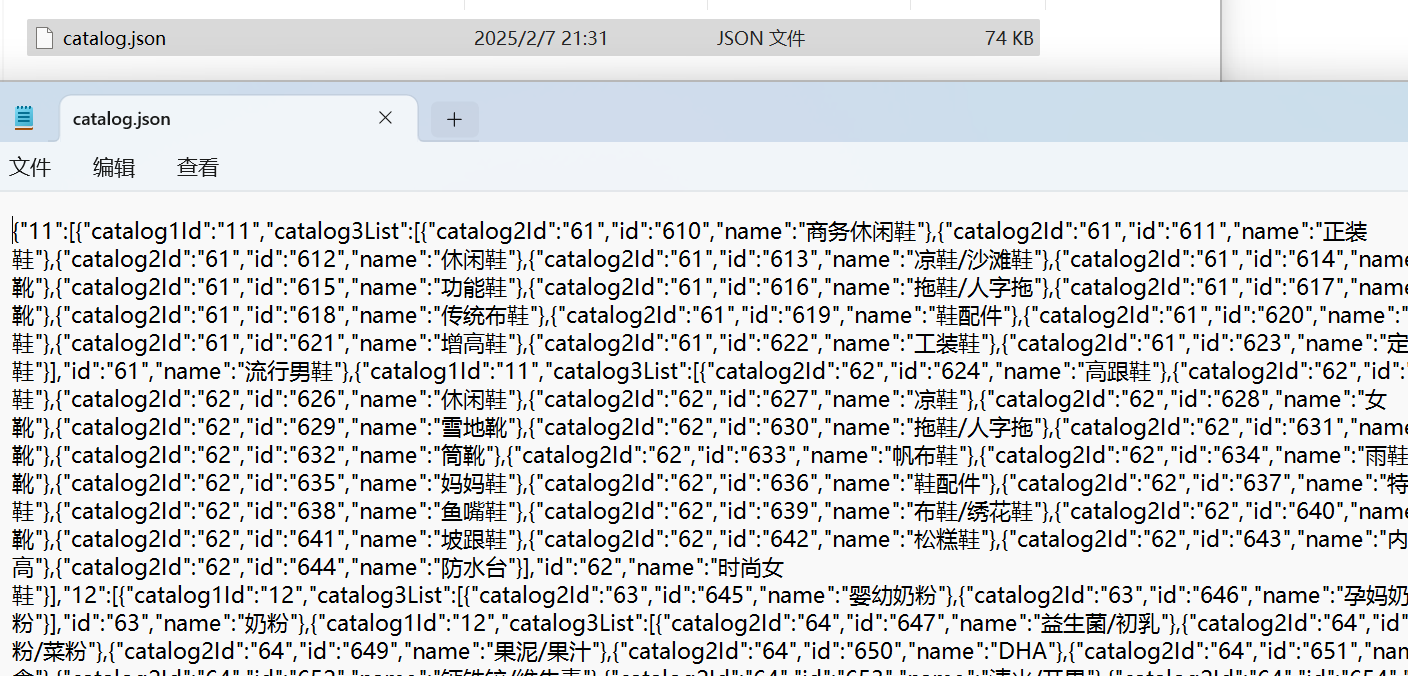
java
import lombok.Data;
import java.util.List;
/**
* 二三级分类id,首页分类数据
**/
@Data
public class Catalog2VO {
/**
* 一级父分类的id
*/
private String catalog1Id;
/**
* 三级子分类
*/
private List<Category3Vo> catalog3List;
private String id;
private String name;
/**
* 三级分类vo
*/
@Data
public static class Category3Vo {
/**
* 父分类、二级分类id
*/
private String catalog2Id;
private String id;
private String name;
}
}
编写分类数据获取接口
java
@Controller
public class IndexController {
@Autowired
CategoryService categoryService;
@GetMapping({"/","/index.html"})
public String indexPage(Model model){
// 1.查出所有1级分类
List<CategoryEntity> entities = categoryService.getLevel1Categorys();
// 返回视图地址
// 使用视图解析器进行拼串
model.addAttribute("categories",entities);
return "index";
}
/**
* 获取分类数据
* @return
*/
@ResponseBody // 返回的是数据而不是页面,要标上注解@ResponseBody
@GetMapping("/index/catalog.json")
public Map<String, List<Catalog2VO>> getCatalogJson(){
Map<String, List<Catalog2VO>> map = categoryService.getCatalogJson();
return map;
}
}
java
@Override
public Map<String, List<Catalog2VO>> getCatalogJson() {
// 1.查出所有1级分类
List<CategoryEntity> leve1Categorys = getLevel1Categorys();
// 2.封装数据
Map<String, List<Catalog2VO>> collect = leve1Categorys.stream().collect(Collectors.toMap(k -> k.getCatId().toString(), v -> {
// 2.1 每一个一级分类,查到这个一级分类的二级分类
List<CategoryEntity> categoryEntities = baseMapper.selectList(new QueryWrapper<CategoryEntity>().eq("parent_cid", v.getCatId()));
// 2.2 封装上面的结果
List<Catalog2VO> catalog2VOS = null;
if (categoryEntities != null) {
catalog2VOS = categoryEntities.stream().map(l2 -> {
Catalog2VO catalog2VO = new Catalog2VO();
catalog2VO.setId(l2.getCatId().toString());
catalog2VO.setName(l2.getName());
catalog2VO.setCatalog1Id(v.getCatId().toString());
// 找当前二级分类的三级分类封装成vo
List<CategoryEntity> level3Category = baseMapper.selectList(new QueryWrapper<CategoryEntity>().eq("parent_cid", l2.getCatId()));
if (level3Category != null) {
List<Catalog2VO.Category3Vo> collect3 = level3Category.stream().map(l3 -> {
return new Catalog2VO.Category3Vo(catalog2VO.getId(), l3.getCatId().toString(),l3.getName());
}).collect(Collectors.toList());
catalog2VO.setCatalog3List(collect3);
}
return catalog2VO;
}).collect(Collectors.toList());
}
return catalog2VOS;
}));
return collect;
}