Collections
1、Counter 计数器
counter:计数器 类似字典 统计可迭代对象中元素的出现次数,
Counter({'b': 3, 'c': 2, 'a': 1, 'd': 1}) 相当于字典{'b': 3, 'c': 2, 'a': 1, 'd': 1}
a.items() 取键值对 对应为dict_items([('a', 1), ('b', 3), ('c', 2), ('d', 1)])
也可以是 list(a.items()) 输出对应为 [('a', 1), ('b', 3), ('c', 2), ('d', 1)]
for k,v in a.items():
print(k,v)
##5 统计最长出现的m个元素
two=a.most_common(2)
代码
python
##counter:计数器 类似字典 统计可迭代对象中元素的出现次数
from collections import Counter
a=Counter()
a=Counter('abccdbb')
print(a)
##1键值对
print(a.items())
##2遍历输出键值 例如
##a 1
##b 3
##c 2
##d 1
for k,v in a.items():
print(k,v)
##3 索引取值
print(a['a'])
##4 利用字典初始化每个元素和出现次数
a=Counter({'a':1,'b':2,'c':3})
print(a)
a=Counter(a=1,b=2,c=3)
print(a)
##5 统计最长出现的m个元素
two=a.most_common(2)
print(two)
[('b', 3), ('c', 2)]
##6 使用新的可迭代对象更新计数
new=['b','m']
a.update(new)
print(a)
Counter({'b': 3, 'c': 2, 'a': 1, 'd': 1})
Counter({'b': 4, 'c': 2, 'a': 1, 'd': 1, 'm': 1})
##7 数学运算
counter1=Counter(a=1,b=1)
counter2=Counter(a=3,b=2)
##加法
add_r=counter1+counter2
print(add_r)
##减法
sub_r=counter1-counter2
print(sub_r)
##交集
iter_r=counter1&counter2
print(iter_r)
##并集
union_r=counter1 | counter2
print(union_r)Counter({'b': 3, 'c': 2, 'a': 1, 'd': 1})
Counter({'a': 4, 'b': 3})
Counter()
Counter({'a': 1, 'b': 1})
Counter({'a': 3, 'b': 2})
##8 获取所有元素
all_num=list(a.elements())
##首字母出现次序
print(all_num)
Counter({'b': 3, 'c': 2, 'a': 1, 'd': 1})
['a', 'b', 'b', 'b', 'c', 'c', 'd']
##每个元素重复的次数等于其计数。
##9 计算总和
##pyhon 3.10以上
print(a.total())
##10 清空
a.clear()
print(a)
2、deque双端队列
重要知识点:
初始化
appendleft(x) popleft extendleft(可迭代对象)
rotate 循环 正数向右移动n步 负数向左移动n步
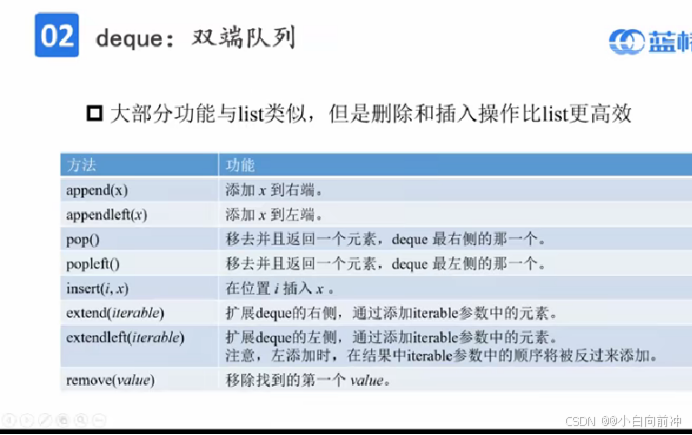
代码
python
##双端列表
from collections import deque
##使用可迭代对象列表,元组等创建双端列表
lst1=[1,2,3,4,5]
a=deque(lst1)
print(a)
##双端列表特殊用法
##1 左侧添加元素
a.appendleft(0)
print(a)
##2 左侧弹出元素
first_value=a.popleft()
print(first_value)
print(list(a))
print(' '.join(map(str,a)))
print(','.join(map(str,a)))
##deque([0, 1, 2, 3, 4, 5])
##0
##[1, 2, 3, 4, 5]
##1 2 3 4 5
##1,2,3,4,5
##3 扩展
left=['a','b','c']
right=['e','f','g']
##扩展左侧
a.extendleft(left)
print(a)
##deque(['c', 'b', 'a', 1, 2, 3, 4, 5])
##扩展左侧 倒过来了
##扩展右侧
a.extend(right)
print(a)
##4 一般用法 移除找到的第一个value
a.remove(1)
lst1.remove(1)
print(lst1)
##5 循环 正数向右移动n步 负数向左移动n步
##向右
a.rotate(2)
print(a)
##deque([1, 2, 3, 4, 5])
##deque([4, 5, 1, 2, 3])
##向左
a.rotate(-3)
print(a)
##deque([1, 2, 3, 4, 5])
##deque([4, 5, 1, 2, 3])
##6 限制 deque 的最大长度 添加一个元素会移走一个元素
d=deque(maxlen=5)
d.extend(lst1)
print(d)
##添加元素'a'
d.append('a')
print(d)
3、defaultdict有默认值的字典
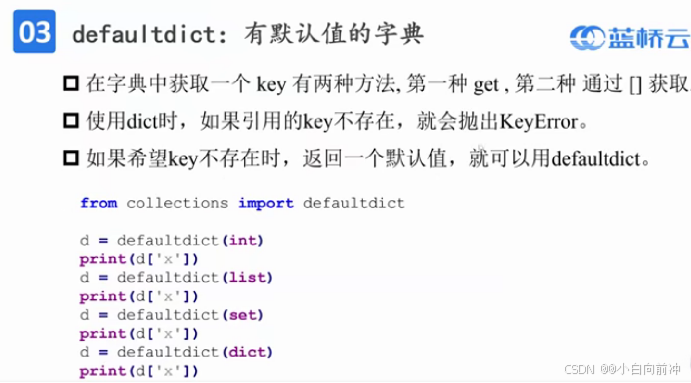
重要知识点
from collections import defaultdict
from collections import Counter
s=[('yellow',1),('blue',2),('yellow',3),('blue',4),('red',1)]
d=defaultdict(list)
for k,v in s:
d[k].append(v)
print(dict(d))
4、OrderedDict有序字典
重要知识点:
popitem last默认为True 删除最后一个 否则删除第一个
代码
python
##有序字典
####popitem last默认为True 删除最后一个 否则删除第一个
from collections import OrderedDict
data=[('a',1),('b',2),('c',3)]
d=OrderedDict(data)
print(d)
while len(d)!=0:
print('删除元素为',d.popitem())
print(d)
##OrderedDict([('a', 1), ('b', 2), ('c', 3)])
##删除元素为 ('c', 3)
##OrderedDict([('a', 1), ('b', 2)])
##删除元素为 ('b', 2)
##OrderedDict([('a', 1)])
##删除元素为 ('a', 1)
##OrderedDict()
data=[('a',1),('b',2),('c',3)]
d=OrderedDict(data)
print(d)
while len(d)!=0:
print('删除元素为',d.popitem(False))
print(d)
##OrderedDict([('a', 1), ('b', 2), ('c', 3)])
##删除元素为 ('a', 1)
##OrderedDict([('b', 2), ('c', 3)])
##删除元素为 ('b', 2)
##OrderedDict([('c', 3)])
##删除元素为 ('c', 3)
##OrderedDict()
5、heapq堆
重要知识点
1、连续输出最小元素:
a=[6,3,2,4,7,1,5]
##1 无序列表转换为最小堆
heapq.heapify(a)
while len(a):
print(heapq.heappop(a),end=' ')
2、连续输出最大元素
将列表取反 构造最大堆 然后弹出的时候加上负号
import heapq
a=[1,2,3,4,5]
b=[-x for x in a]
heapq.heapify(b)
while len(b):
print(-heapq.heappop(b),end=' ')
代码
python
import heapq
####使用list表示一个堆
a=[6,3,2,4,7,1,5]
##1 无序列表转换为最小堆
heapq.heapify(a)
print(a)
##2 最小堆中添加元素x
heapq.heappush(a,8)
print(a)
##3 弹出并返回最小元素
heapq.heappop(a)
print(a)
##4 弹出并返回最小元素 同时添加元素x
m=heapq.heapreplace(a,0)
print(m)
print(a)
##一直弹出最小的元素 排序从小到大
while len(a):
print(heapq.heappop(a),end=' ')
itertools
1、accumulate 有限迭代器
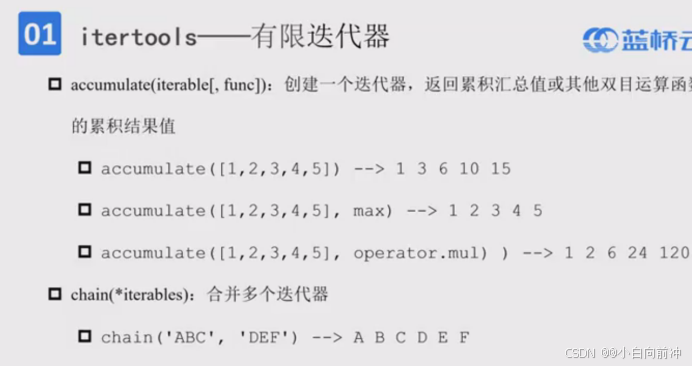
python
from itertools import accumulate,chain,product,permutations,combinations
import operator
a=[1,4,2,3,5]
##前缀和
b=list(accumulate(a))
print(b)
##累计输出最大
c=list(accumulate(a,max))
print(c)
##[1, 5, 7, 10, 15]
##[1, 4, 4, 4, 5]
##累计乘积operator.mul
d=list(accumulate(a,operator.mul))
print(d)
##[1, 4, 8, 24, 120]
##
print(list(chain([4,6,5],[1,2,3])))
2、product combinations permutations
重要知识点:
1、##product product(多个可迭代对象,repeat=)可迭代对象的笛卡尔积,穷举所有组合
2、##permutations(可迭代对象,r=None)
##由可迭代对象生成的长度为r的排列,r未指定或为None或为可迭代对象长度
3、##combination(可迭代对象,r)返回输入可迭代对象中元素组成长度为r的序列
代码
python
##排列组合迭代器
##product product(多个可迭代对象,repeat=)可迭代对象的笛卡尔积,穷举所有组合
a=[1,2,3,4]
b=[5,6,7,8]
print(list(product(a,b)))
##[(1, 5), (1, 6), (1, 7), (1, 8), (2, 5), (2, 6), (2, 7), (2, 8), (3, 5), (3, 6), (3, 7), (3, 8), (4, 5), (4, 6), (4, 7), (4, 8)]
##repeat 表示这些可迭代序列重复的次数
d=list(product('ab',repeat=2))
##[('a', 'a'), ('a', 'b'), ('b', 'a'), ('b', 'b')])
##类似于 product('ab','ab')
print(d)
##permutations(可迭代对象,r=None)
##由可迭代对象生成的长度为r的排列,r未指定或为None或为可迭代对象长度
a=list(permutations('ABCD',2))
##排列有重复的
print(a)
##[('A', 'B'), ('A', 'C'), ('A', 'D'), ('B', 'A'), ('B', 'C'), ('B', 'D'), ('C', 'A'), ('C', 'B'), ('C', 'D'), ('D', 'A'), ('D', 'B'), ('D', 'C')]
b=list(permutations([1,2,3,4],3))
print(b)
print(len(b))
##[(1, 2, 3), (1, 2, 4), (1, 3, 2), (1, 3, 4), (1, 4, 2), (1, 4, 3), (2, 1, 3), (2, 1, 4), (2, 3, 1), (2, 3, 4), (2, 4, 1), (2, 4, 3), (3, 1, 2), (3, 1, 4), (3, 2, 1), (3, 2, 4), (3, 4, 1), (3, 4, 2), (4, 1, 2), (4, 1, 3), (4, 2, 1), (4, 2, 3), (4, 3, 1), (4, 3, 2)]
24
b=list(permutations([1,2,3,4]))
print(b)
print(len(b))
##[(1, 2, 3, 4), (1, 2, 4, 3), (1, 3, 2, 4), (1, 3, 4, 2), (1, 4, 2, 3), (1, 4, 3, 2), (2, 1, 3, 4), (2, 1, 4, 3), (2, 3, 1, 4), (2, 3, 4, 1), (2, 4, 1, 3), (2, 4, 3, 1), (3, 1, 2, 4), (3, 1, 4, 2), (3, 2, 1, 4), (3, 2, 4, 1), (3, 4, 1, 2), (3, 4, 2, 1), (4, 1, 2, 3), (4, 1, 3, 2), (4, 2, 1, 3), (4, 2, 3, 1), (4, 3, 1, 2), (4, 3, 2, 1)]
24
##combination(可迭代对象,r)返回输入可迭代对象中元素组成长度为r的序列
m=list(combinations('ABCD',2))
##4*3*2*1/2*1*2*1
print(m)
print(len(m))
print(math.comb(4,2))
n=list(permutations('ABCD',2))
##4*3*2*1/2*1
print(n)
print(len(n))
any all 用法
代码
python
##pyhon 内置函数all any用法
nums=[4,6,8]
result=all(num%2==0 for num in nums)
print(result)
##any用于检查 可迭代对象是否至少一个元素为真
str1=('1','hello','')
result2=any(str1)
print(result2)
time 模块
重要知识点
不支持减法
1.时间戳 time.time()
2.格式化时间
##本地时间转换成字符串
str1=time.strftime('%Y-%m-%d %H:%M:%S',t )
print(str1)
str2=time.strftime('%Y-%m-%d',t)
print(str2)
str3=time.strftime('%H:%M:%S',t)
print(str3)
##将字符串解析为时间
time_str='2025-04-05 14:33:57'
format_str='%Y-%m-%d %H:%M:%S'
str2=time.strptime(time_str,format_str )
print(str2)
time.struct_time(tm_year=2025, tm_mon=4, tm_mday=5, tm_hour=14, tm_min=33, tm_sec=57, tm_wday=5, tm_yday=95, tm_isdst=-1)
##2
time_str1='2025/04/05 14:33:57'
format_str1='%Y/%m/%d %H:%M:%S'
str2=time.strptime(time_str1,format_str1)
print(str2)
time.struct_time(tm_year=2025, tm_mon=4, tm_mday=5, tm_hour=14, tm_min=33, tm_sec=57, tm_wday=5, tm_yday=95, tm_isdst=-1)
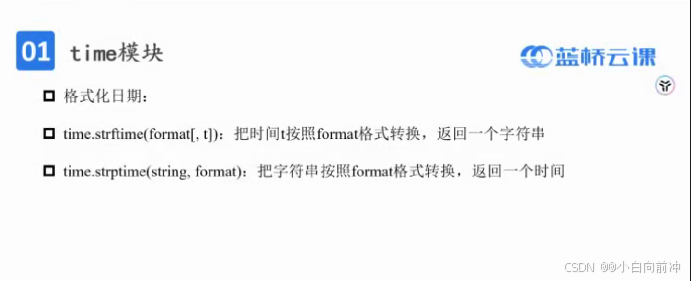
代码
python
##时间日期
import time
##时间戳
start_time=time.time()
print(start_time)
time.sleep(3)
end_time=time.time()
print(end_time)
print('运行时间为:{:.0f}秒'.format(end_time-start_time))
##本地时间
t=time.localtime()
print(t)
##time.struct_time(tm_year=2025, tm_mon=4, tm_mday=5, tm_hour=14, tm_min=22, tm_sec=50, tm_wday=5, tm_yday=95, tm_isdst=0)
print(t.tm_year)
print(t.tm_mon)
print(t.tm_mday)
##......
##tm_wday 一周第几天
##tm_yday 一年第几天
##tm_isdst 夏令标识
##格式化日期
##本地时间转换成字符串
str1=time.strftime('%Y-%m-%d %H:%M:%S',t)
print(str1)
str2=time.strftime('%Y-%m-%d',t)
print(str2)
str3=time.strftime('%H:%M:%S',t)
print(str3)
##2025-04-05 14:31:25
##2025-04-05
##14:31:25
##将字符串解析为时间
time_str='2025-04-05 14:33:57'
format_str='%Y-%m-%d %H:%M:%S'
str2=time.strptime(time_str,format_str)
print(str2)
time.struct_time(tm_year=2025, tm_mon=4, tm_mday=5, tm_hour=14, tm_min=33, tm_sec=57, tm_wday=5, tm_yday=95, tm_isdst=-1)
##2
time_str1='2025/04/05 14:33:57'
format_str1='%Y/%m/%d %H:%M:%S'
str2=time.strptime(time_str1,format_str1)
print(str2)
time.struct_time(tm_year=2025, tm_mon=4, tm_mday=5, tm_hour=14, tm_min=33, tm_sec=57, tm_wday=5, tm_yday=95, tm_isdst=-1)
datetime 模块
重要知识点:
date支持减法 支持比较大小
time不支持减法需转换为datetime进行 支持比较大小
datetime 支持减法支持比较大小 获取日期 a.date() 获取时间 a.time()
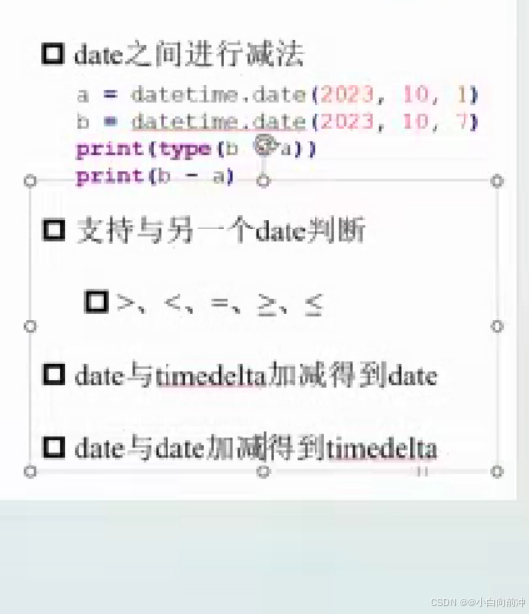
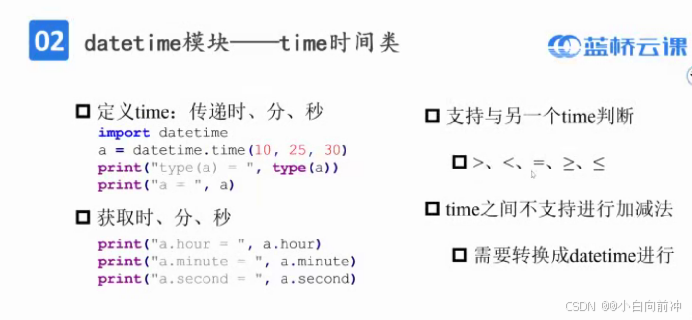
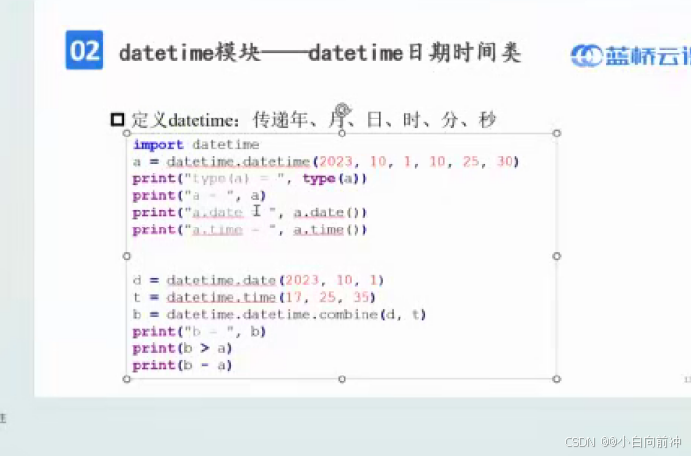
- datetime 模块里面有五个
##datetime模块
##date 日期
##time 时间
##datetime 日期时间
##timedelta 时间间隔
2.格式化
##datetime模块 日期时间和字符串的转换
datetime_str=a.strftime('%Y-%m-%d %H:%M:%S') a是一个datetime对象
print(datetime_str)
a=datetime.datetime.strptime (datetime_str,'%Y-%m-%d %H:%M:%S')
代码
python
import datetime
##datetime模块
##date 日期
##time 时间
##datetime 日期时间
##timedelta 时间间隔
##date 日期类 支持比较 支持加减法
a=datetime.date(2025,4,5)
b=datetime.date(2023,6,7)
delta=b-a
print(delta)
print(b>a)
##时间类 支持比较 不支持加减法
c=datetime.time(14,48,30)
d=datetime.time(14,49,30)
print(c.hour)
print(c.minute)
print(c.second)
print(d>c)
##日期时间模块 支持比较 支持加减法
a=datetime.datetime(2025,4,5,14,51,57)
b=datetime.datetime(2024,4,6,14,33,7)
d=datetime.date(2025,4,7)
e=datetime.time(15,30,33)
f=datetime.datetime.combine(d,e)
print(f)
print(a-b)
print(b==a)
print(b>a)
##星期几
print(a.weekday())
##datetime模块 timedelta类
delta=datetime.timedelta(days=100)
delta1=a-b
print(delta1.days)
print(delta1.seconds)
##print(delta1.total_seconds())
print(delta1.microseconds)
##datetime模块 日期时间和字符串的转换
datetime_str=a.strftime('%Y-%m-%d %H:%M:%S')
print(datetime_str)
a=datetime.datetime.strptime(datetime_str,'%Y-%m-%d %H:%M:%S')
print(a)
print(type(a))