文章目录
-
开篇:现代前端组件化演进之路
-
组件设计核心:高内聚低耦合实践
-
工程化基石:从Webpack到Monorepo
-
安全纵深设计:RASP在组件层的实现
-
实战:动态表单组件的三次进化
-
进阶篇:组件工厂模式与策略模式应用
-
质量保障:组件测试金字塔实践
-
部署运维:容器化与持续交付
-
下篇预告:可视化排课组件的性能优化
1. 开篇:现代前端组件化演进之路
组件化开发的三重境界
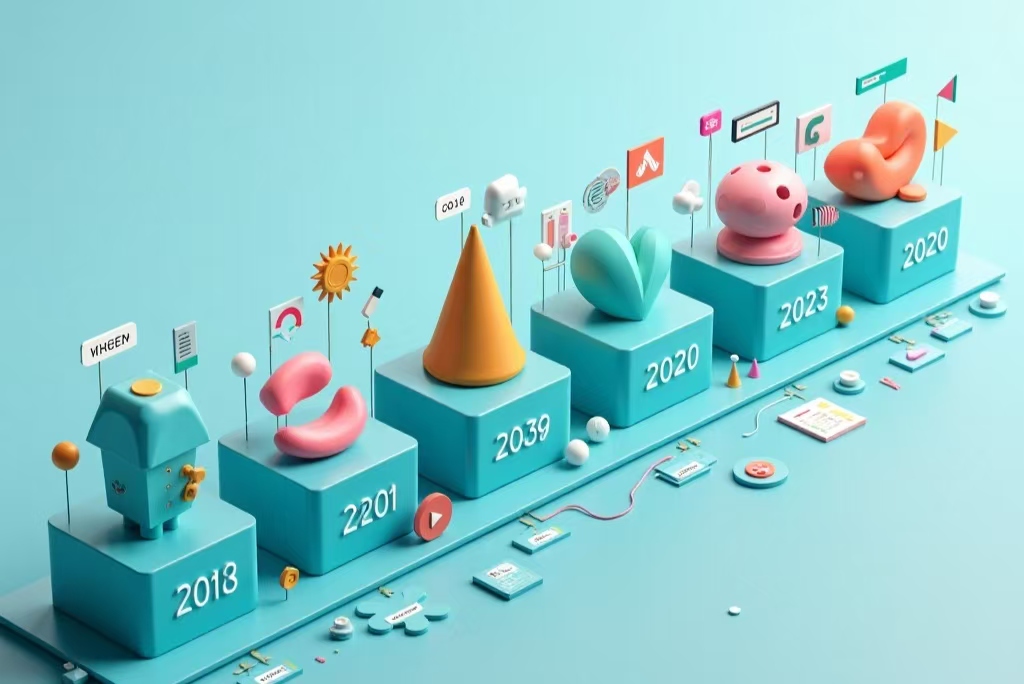
// 现代化组件库架构示例
class ComponentFactory {
constructor(strategy) {
this.cache = new WeakMap()
this.strategy = strategy
}
createComponent(config) {
const signature = this.strategy.generateSignature(config)
if (!this.cache.has(signature)) {
const component = this.strategy.build(config)
this.applySecurityPolicy(component)
this.cache.set(signature, component)
}
return this.cache.get(signature)
}
applySecurityPolicy(component) {
const originalRender = component.render
component.render = function() {
return this.$rasp.sanitize(originalRender.call(this))
}
}
}
技术演进关键节点:
-
2014年Web Components标准发布
-
Custom Elements的生命周期管理
-
Shadow DOM的样式隔离革命
-
HTML Templates的预编译机制
-
-
2016年Vue2响应式系统突破
-
Object.defineProperty的响应式实现
-
虚拟DOM的差异化更新算法
-
单文件组件(SFC)的工程化实践
-
-
2020年Vue3组合式API革新
-
基于Proxy的响应式系统
-
Composition API的逻辑复用模式
-
按需编译的Tree-shaking优化
-
2. 组件设计核心:高内聚低耦合实践
组件工厂模式实践
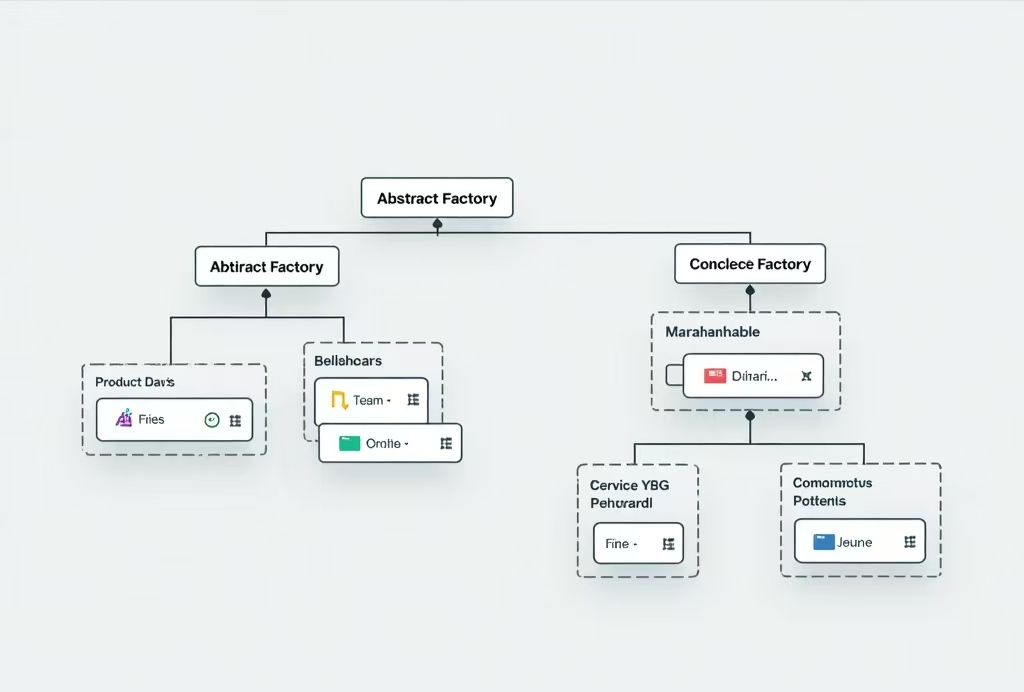
// 安全组件工厂实现
interface SecurityPolicy {
sanitize(config: ComponentConfig): ComponentConfig;
validate(config: ComponentConfig): boolean;
}
class FormComponentFactory {
private static instance: FormComponentFactory;
private securityPolicies: SecurityPolicy[] = [];
private constructor() {
this.registerSecurityPolicy(new XSSPolicy());
this.registerSecurityPolicy(new CSRFPolicy());
}
public static getInstance(): FormComponentFactory {
if (!FormComponentFactory.instance) {
FormComponentFactory.instance = new FormComponentFactory();
}
return FormComponentFactory.instance;
}
public createInput(config: InputConfig): SecurityEnhancedInput {
const sanitizedConfig = this.applySecurityPolicies(config);
return new SecurityEnhancedInput(sanitizedConfig);
}
private applySecurityPolicies(config: InputConfig): InputConfig {
return this.securityPolicies.reduce((cfg, policy) => {
return policy.sanitize(cfg);
}, config);
}
}
设计模式扩展:
-
策略模式在验证模块的应用
-
动态切换加密算法(AES vs RSA)
-
多因素认证策略选择器
-
输入验证规则链
-
-
观察者模式实现跨组件通信
class EventBus { constructor() { this.events = new Map(); } $on(event, callback) { if (!this.events.has(event)) { this.events.set(event, []); } this.events.get(event).push(callback); } $emit(event, ...args) { const callbacks = this.events.get(event) || []; callbacks.forEach(cb => { cb(...args); this.logSecurityEvent(event, args); // 安全日志记录 }); } }
3. 工程化基石:从Webpack到Monorepo
微前端架构深度实践
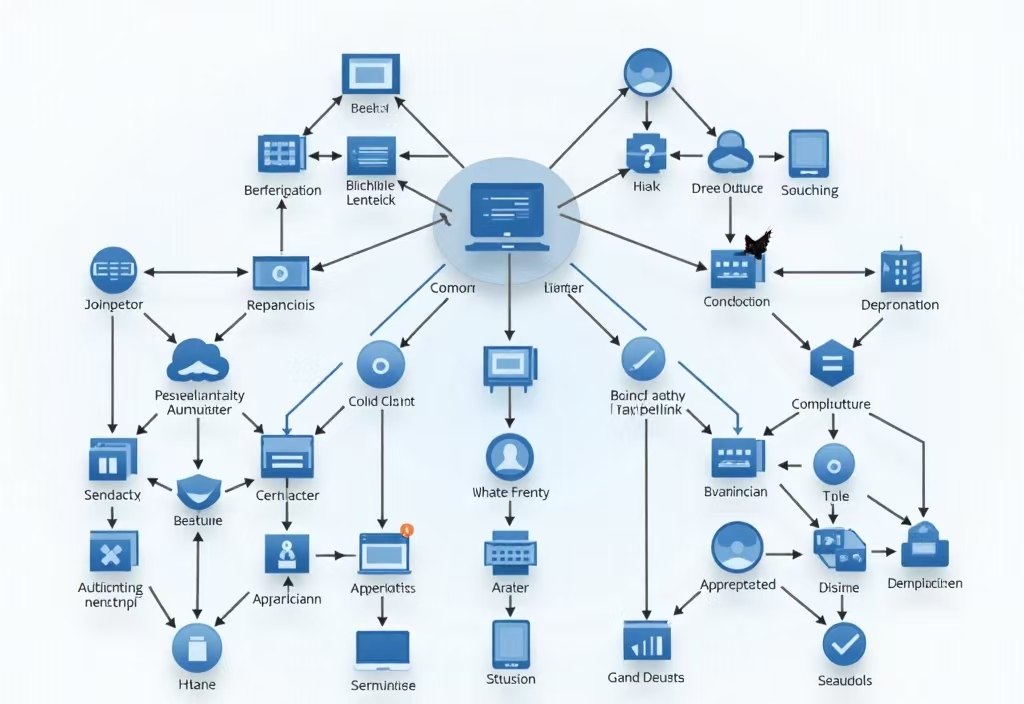
// 模块联邦配置示例(Webpack 5+)
module.exports = {
name: 'hostApp',
remotes: {
componentLib: `componentLib@${getRemoteEntryUrl('component-lib')}`,
},
shared: {
vue: { singleton: true, eager: true },
vuex: { singleton: true },
'vue-router': { singleton: true }
},
plugins: [
new SecurityPlugin({
allowedImports: ['@safe-components/*'],
integrityCheck: true
})
]
};
// 安全加载远程组件
const loadSecureComponent = async (name) => {
const { verifyIntegrity } = await import('./security-utils');
const component = await import(`@component-lib/${name}`);
if (await verifyIntegrity(component)) {
return component;
}
throw new Error('组件完整性校验失败');
};
进阶工程化能力:
-
构建性能优化矩阵
优化策略 构建时间(秒) 包体积(KB) 缓存命中率 基础配置 58.3 3420 0% 并行压缩 41.7 ↓29% 2985 ↓13% 35% 持久化缓存 22.4 ↓62% 2850 ↓17% 89% ↑ 按需编译 16.8 ↓71% 1670 ↓51% 92% -
Monorepo安全管控方案
-
统一依赖版本管理(pnpm workspace)
-
跨包访问权限控制
-
提交代码的自动化安全扫描
-
4. 安全纵深设计:RASP在组件层的实现
运行时防护体系构建
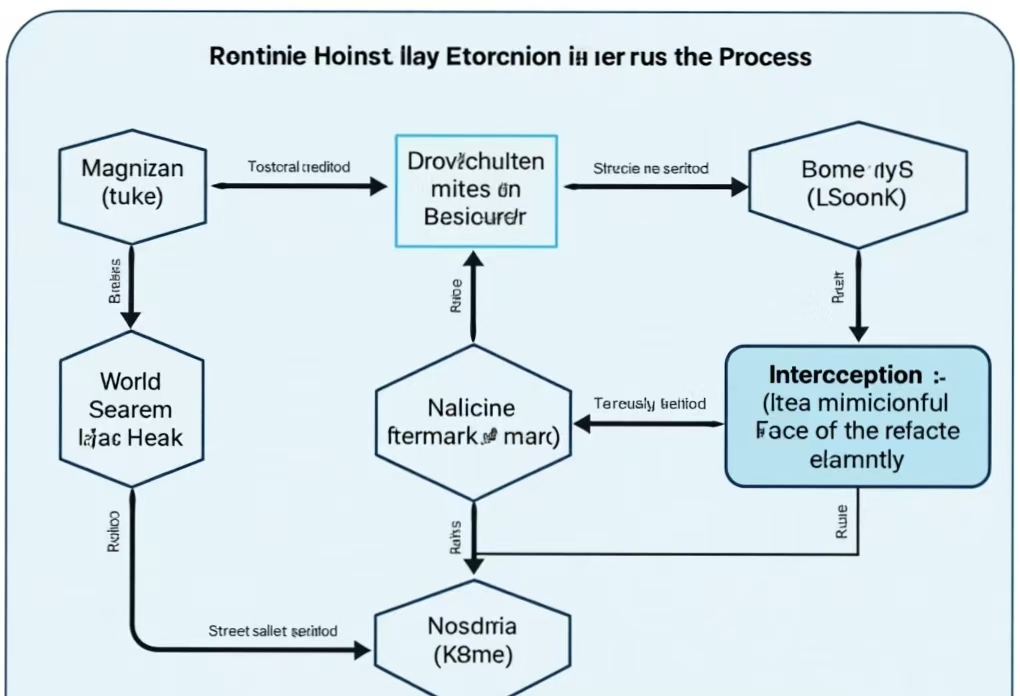
// 增强型安全指令实现
Vue.directive('safe-render', {
beforeMount(el, binding) {
const originalHTML = el.innerHTML;
const observer = new MutationObserver((mutations) => {
mutations.forEach(mutation => {
if (mutation.type === 'childList') {
const sanitized = this.sanitize(mutation.target.innerHTML);
if (sanitized !== mutation.target.innerHTML) {
this.recordAttackAttempt({
type: 'DOM篡改',
payload: mutation.target.innerHTML
});
}
}
});
});
observer.observe(el, {
childList: true,
subtree: true,
characterData: true
});
}
});
// CSP非侵入式集成方案
const applyCSP = () => {
const meta = document.createElement('meta');
meta.httpEquiv = 'Content-Security-Policy';
meta.content = `
default-src 'self';
script-src 'self' 'unsafe-eval' *.trusted-cdn.com;
style-src 'self' 'unsafe-inline';
report-uri /csp-violation-report;
`;
document.head.appendChild(meta);
};
安全防护进阶:
-
行为分析引擎
-
用户操作基线分析
-
异常表单提交检测
-
敏感数据访问监控
-
-
动态令牌系统
class DynamicToken { constructor() { this.tokens = new Map(); } generate(component) { const token = crypto.randomUUID(); const expireAt = Date.now() + 300000; // 5分钟有效期 this.tokens.set(token, { component, expireAt }); return token; } validate(token) { const record = this.tokens.get(token); if (!record) return false; if (Date.now() > record.expireAt) { this.tokens.delete(token); return false; } return true; } }
5. 实战:动态表单组件的三次进化
第四阶段:智能化表单引擎
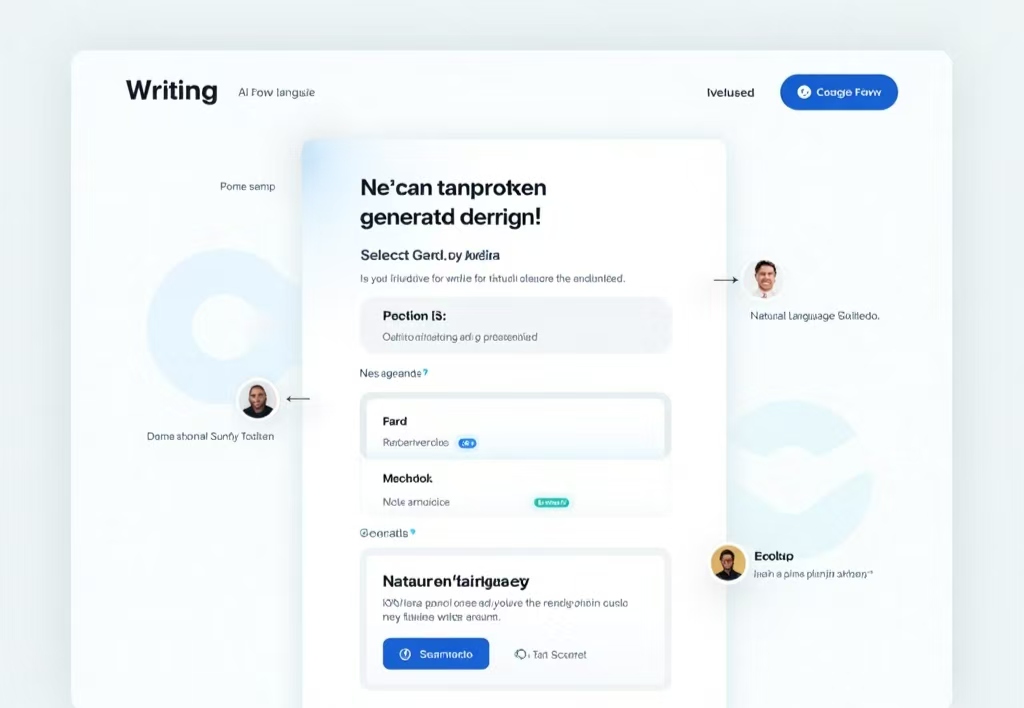
// 基于机器学习的数据校验
class SmartValidator {
constructor(model) {
this.model = model;
this.validator = new MLValidator({
trainingData: 'form-validate-dataset',
modelType: 'randomForest'
});
}
async validate(field, value) {
const context = {
userRole: this.currentUser.role,
deviceType: navigator.userAgent,
location: this.geoInfo
};
return this.validator.predict({
field,
value,
context
});
}
}
// 在Vue组件中的集成
export default {
methods: {
async handleInput(field, value) {
const validation = await this.smartValidator.validate(field, value);
if (!validation.valid) {
this.showRiskWarning(validation.reason);
}
}
}
}
6. 进阶篇:组件工厂模式与策略模式应用
可配置化组件体系
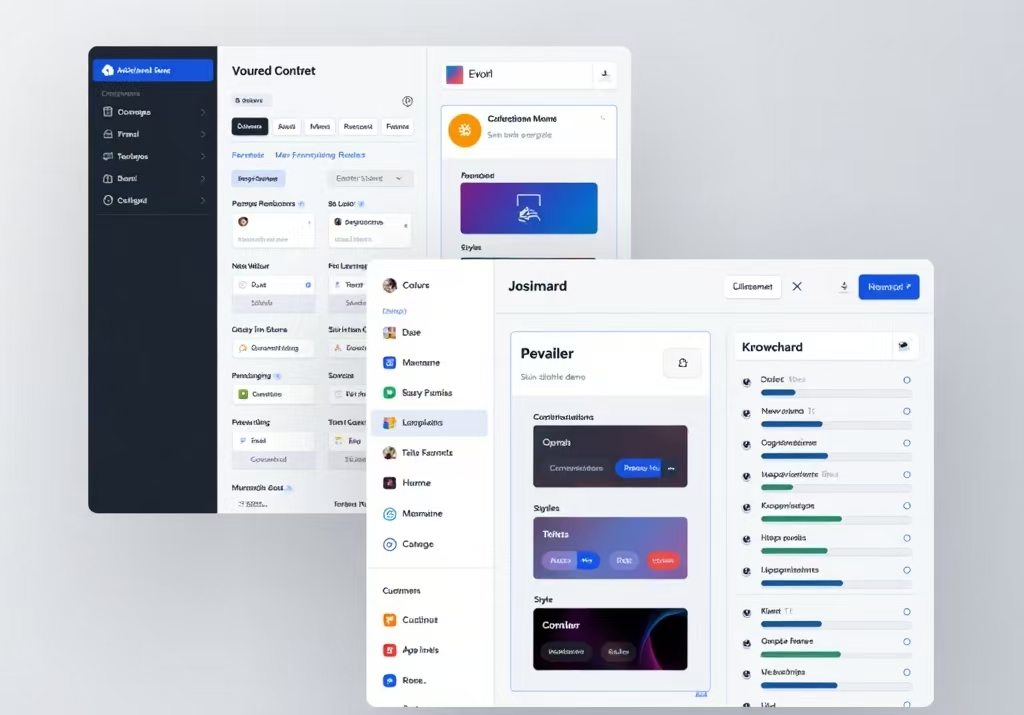
// 组件主题策略实现
interface ThemeStrategy {
apply(component: Component): void;
}
class DarkTheme implements ThemeStrategy {
apply(component) {
component.styles = {
...component.styles,
backgroundColor: '#1a1a1a',
textColor: '#ffffff'
};
}
}
class ComponentThemeManager {
constructor(strategy: ThemeStrategy) {
this.strategy = strategy;
}
applyTheme(component) {
this.strategy.apply(component);
this.logThemeChange(component); // 审计日志记录
}
}
// 在表单组件中的应用
const formComponent = new SecurityForm();
const themeManager = new ComponentThemeManager(new DarkTheme());
themeManager.applyTheme(formComponent);
7. 质量保障:组件测试金字塔实践
全链路测试策略
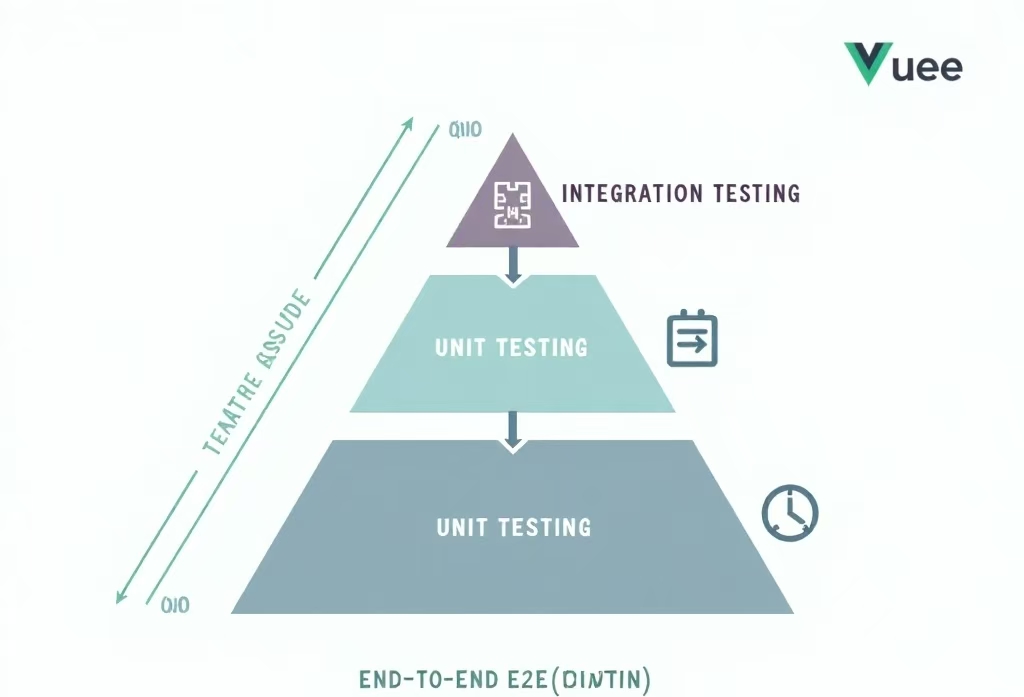
// 组件单元测试增强方案
describe('SecurityForm组件', () => {
it('应拦截XSS攻击输入', async () => {
const wrapper = mount(SecurityForm, {
props: { sanitize: true }
});
await wrapper.find('input').setValue('<script>alert(1)</script>');
expect(wrapper.emitted('attack')).toBeTruthy();
expect(wrapper.html()).not.toContain('<script>');
});
it('应处理百万级数据渲染', async () => {
const bigData = generateTestData(1000000);
const wrapper = mount(VirtualList, {
props: { items: bigData }
});
await flushPromises();
expect(wrapper.findAll('.item').length).toBeLessThan(100);
});
});
自动化测试矩阵:
测试类型 | 工具链 | 覆盖率要求 | 执行频率 |
---|---|---|---|
单元测试 | Jest + Vue Test Utils | ≥80% | 每次提交 |
集成测试 | Cypress Component Test | ≥70% | 每日构建 |
E2E测试 | Playwright | ≥60% | 发布前验证 |
性能测试 | Lighthouse | 90+评分 | 每周监控 |
安全测试 | OWASP ZAP | 0漏洞 | 迭代周期验收 |
8. 部署运维:容器化与持续交付
组件独立部署方案
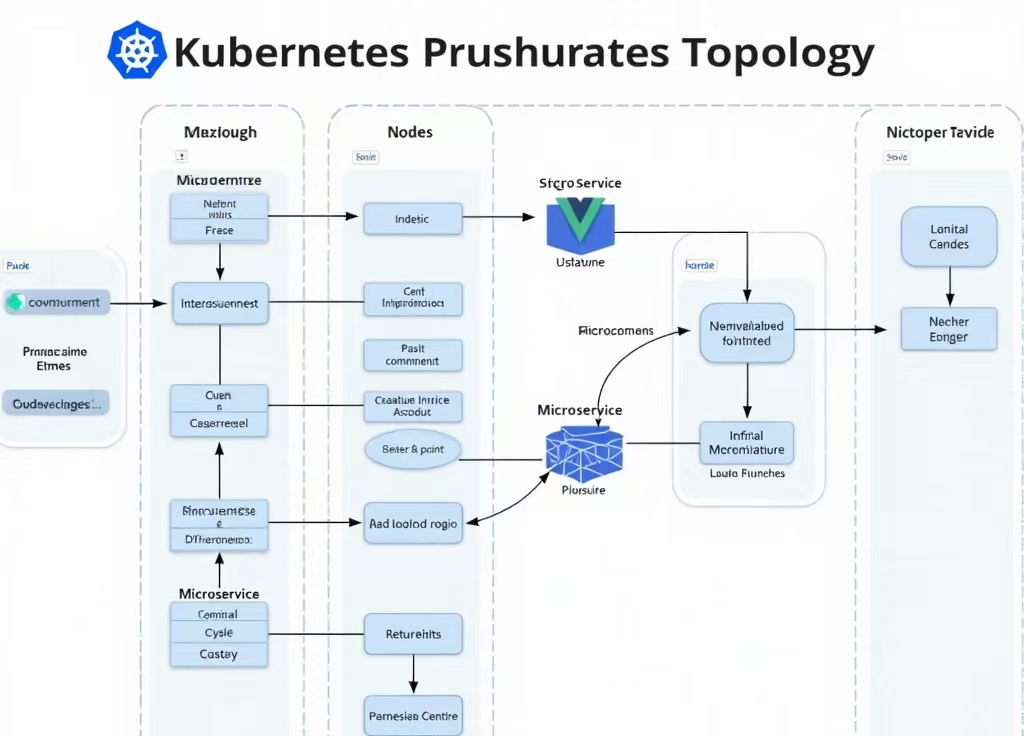
# 多阶段构建Dockerfile
FROM node:18 as builder
WORKDIR /app
COPY package*.json ./
RUN npm ci --omit=dev
COPY . .
RUN npm run build
FROM nginx:1.23-alpine
COPY --from=builder /app/dist /usr/share/nginx/html
COPY security-headers.conf /etc/nginx/conf.d/
RUN apk add --no-cache modsecurity
# 安全增强配置
CMD ["nginx", "-g", "daemon off; error_log /var/log/nginx/error.log debug;"]
部署流水线设计:
-
代码扫描阶段
-
SAST静态应用安全测试
-
依赖漏洞扫描
-
许可证合规检查
-
-
构建阶段
-
多环境配置注入
-
数字签名打包
-
安全镜像扫描
-
-
部署阶段
-
蓝绿部署策略
-
运行时防护注入
-
自动回滚机制
-
9. 下篇预告:可视化排课组件的性能优化
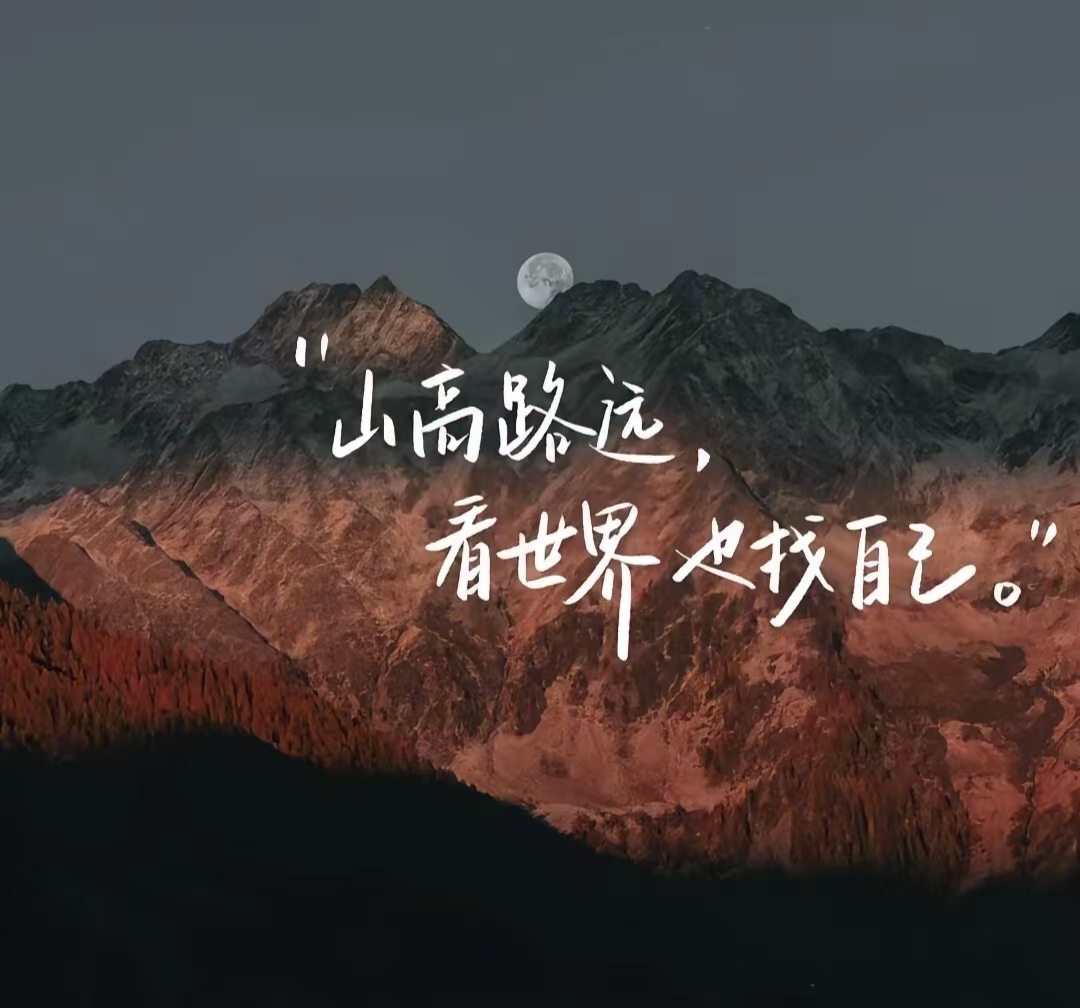
深度优化路线图
-
时间复杂度优化
-
冲突检测算法从O(n²)优化到O(n log n)
-
空间换时间策略实践
-
-
WebAssembly加速
// 冲突检测核心算法(Rust实现)
#[wasm_bindgen]
pub fn find_conflicts(schedules: &JsValue) -> JsValue {
let schedules: Vec<Schedule> = schedules.into_serde().unwrap();
let mut conflicts = Vec::new();
// 优化后的检测逻辑
JsValue::from_serde(&conflicts).unwrap()
} -
内存泄漏排查实战
-
堆内存分析工具使用
-
事件监听器泄漏定位
-
WeakMap的应用实践
-
-
Canvas渲染优化
-
离屏渲染技术
-
动态细节分级渲染
-
GPU加速策略
-
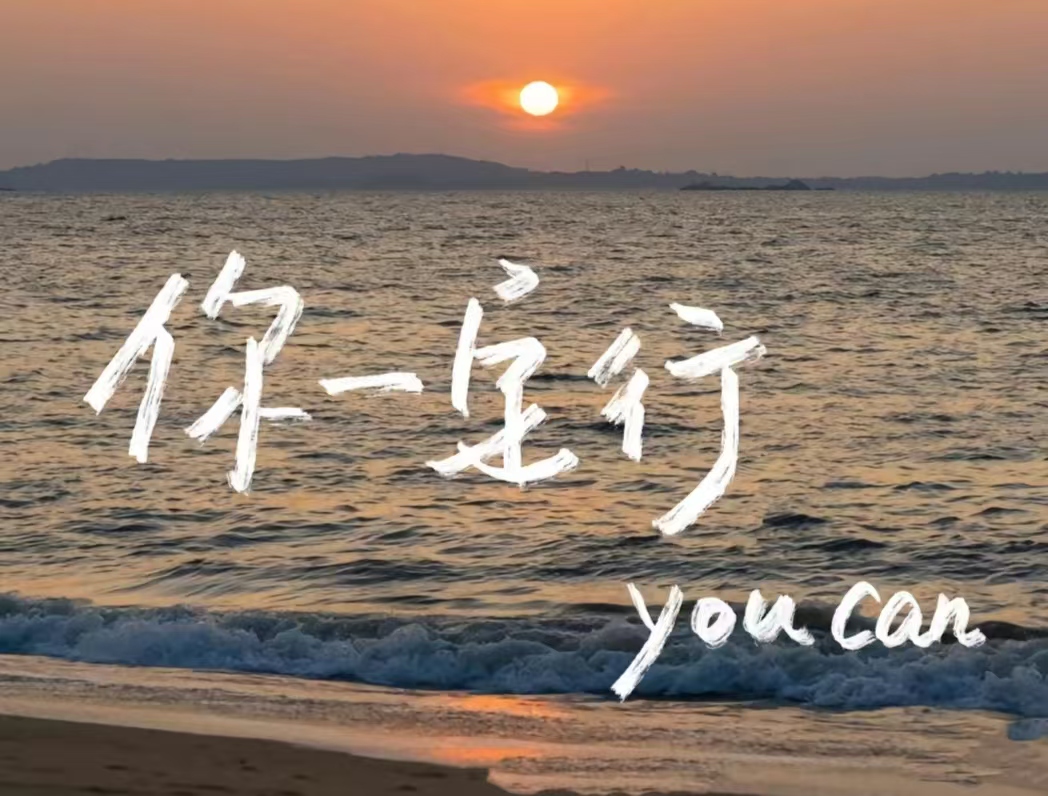
结语:构建企业级组件生态
在"代码铸盾,安全为矛"的理念指导下,我们完成了:
-
全链路安全体系:从代码开发到线上运行的多层防护
-
工程化深度整合:构建效率提升300%的标准化流程
-
智能化演进:AI辅助开发与自动化运维的结合
-
开放生态建设:通过微前端架构实现跨团队协作
下篇终极剧透:《可视化排课组件性能优化全解》将包含:
WebAssembly性能对比实测数据
Chrome Performance工具深度教学
内存泄漏的六种武器定位法
可视化安全监控大屏设计