文章目录
- 一、项目概述
- 二、项目准备
-
- [2.1 安装依赖](#2.1 安装依赖)
- [2.2 获取 Google Maps API Key](#2.2 获取 Google Maps API Key)
- 三、项目结构
- 四、实现功能
-
- [4.1 配置 Google Maps API](#4.1 配置 Google Maps API)
- [4.2 创建 MapComponent 组件](#4.2 创建 MapComponent 组件)
- [4.3 路线规划功能](#4.3 路线规划功能)
- [4.4 配置 Vue Router 路由](#4.4 配置 Vue Router 路由)
- 五、总结
一、项目概述
在这个教程中,我们将使用 Vue 3 和 Google Maps API 创建一个具有地图展示、用户定位和路线规划功能的应用。用户可以查看地图、获取当前位置,并输入起点与终点,查看最佳行车路线。
二、项目准备
2.1 安装依赖
首先,我们需要安装 Vue 3 项目,并且集成 Google Maps API。可以通过 Vue CLI 创建一个新项目:
bash
vue create vue-google-maps
cd vue-google-maps
npm install vue-router
接着,我们安装 Google Maps JavaScript API 的包(如 @react-google-maps/api
):
bash
npm install @react-google-maps/api
2.2 获取 Google Maps API Key
到 Google Cloud Console 获取 API Key。创建一个新的项目,并启用 Maps JavaScript API 和 Directions API。记得将生成的 API Key 替换为你自己的。
三、项目结构
项目结构如下:
css
src/
├── assets/
├── components/
│ └── MapComponent.vue
├── views/
│ └── Home.vue
├── App.vue
└── main.js
四、实现功能
4.1 配置 Google Maps API
在 src/main.js
中,配置 Google Maps API:
javascript
import { createApp } from 'vue'
import App from './App.vue'
import { defineCustomElements } from '@ionic/pwa-elements/dist/loader';
createApp(App).mount('#app')
defineCustomElements(window);
4.2 创建 MapComponent 组件
在 src/components/MapComponent.vue
中,创建一个地图组件:
javascript
<template>
<div id="map" style="width: 100%; height: 500px;"></div>
</template>
<script>
import { onMounted } from 'vue';
export default {
name: 'MapComponent',
props: {
apiKey: {
type: String,
required: true,
}
},
methods: {
initMap() {
const map = new google.maps.Map(document.getElementById("map"), {
center: { lat: 40.7128, lng: -74.0060 },
zoom: 12,
});
const geocoder = new google.maps.Geocoder();
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(position => {
const userPos = {
lat: position.coords.latitude,
lng: position.coords.longitude
};
map.setCenter(userPos);
});
}
}
},
mounted() {
const script = document.createElement("script");
script.src = `https://maps.googleapis.com/maps/api/js?key=${this.apiKey}&callback=initMap`;
script.async = true;
document.head.appendChild(script);
window.initMap = this.initMap;
}
}
</script>
4.3 路线规划功能
在地图中加入路线规划功能,用户输入起点与终点,点击按钮后展示路线。代码如下:
javascript
<template>
<div>
<div id="map" style="width: 100%; height: 500px;"></div>
<div>
<input v-model="origin" placeholder="起点地址" />
<input v-model="destination" placeholder="终点地址" />
<button @click="getRoute">获取路线</button>
</div>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
name: 'RoutePlanner',
props: {
apiKey: {
type: String,
required: true,
}
},
setup() {
const origin = ref('');
const destination = ref('');
const getRoute = () => {
const directionsService = new google.maps.DirectionsService();
const directionsRenderer = new google.maps.DirectionsRenderer();
directionsRenderer.setMap(map);
const request = {
origin: origin.value,
destination: destination.value,
travelMode: google.maps.TravelMode.DRIVING,
};
directionsService.route(request, (result, status) => {
if (status === google.maps.DirectionsStatus.OK) {
directionsRenderer.setDirections(result);
} else {
alert("无法获取路线: " + status);
}
});
};
return { origin, destination, getRoute };
},
mounted() {
const script = document.createElement("script");
script.src = `https://maps.googleapis.com/maps/api/js?key=${this.apiKey}&callback=initMap&libraries=drawing,places`;
script.async = true;
document.head.appendChild(script);
window.initMap = () => {
map = new google.maps.Map(document.getElementById("map"), {
center: { lat: 40.7128, lng: -74.0060 },
zoom: 12,
});
};
}
}
</script>
4.4 配置 Vue Router 路由
在 src/views/Home.vue
中,使用 MapComponent
组件,并传入 API Key:
javascript
<template>
<div>
<h1>Vue 3 + Google Maps API 示例</h1>
<MapComponent :apiKey="apiKey" />
<RoutePlanner :apiKey="apiKey" />
</div>
</template>
<script>
import MapComponent from '@/components/MapComponent.vue';
import RoutePlanner from '@/components/RoutePlanner.vue';
export default {
name: 'Home',
components: {
MapComponent,
RoutePlanner,
},
data() {
return {
apiKey: 'YOUR_GOOGLE_MAPS_API_KEY',
};
},
};
</script>
五、总结
本教程通过 Vue 3 和 Google Maps API 实现了地图展示、实时定位和路线规划功能。用户可以查看当前位置,输入起点和终点后,查看驾车路线。你可以根据需要进行更多自定义,比如添加地图标记、根据用户选择规划不同的交通方式等功能。
到这里,这篇文章就和大家说再见啦!我的主页里还藏着很多 篇 前端 实战干货,感兴趣的话可以点击头像看看,说不定能找到你需要的解决方案~
创作这篇内容花了很多的功夫。如果它帮你解决了问题,或者带来了启发,欢迎:
点个赞❤️ 让更多人看到优质内容
关注「前端极客探险家」🚀 每周解锁新技巧
收藏文章⭐️ 方便随时查阅
📢 特别提醒:
转载请注明原文链接,商业合作请私信联系
感谢你的阅读!我们下篇文章再见~ 💕
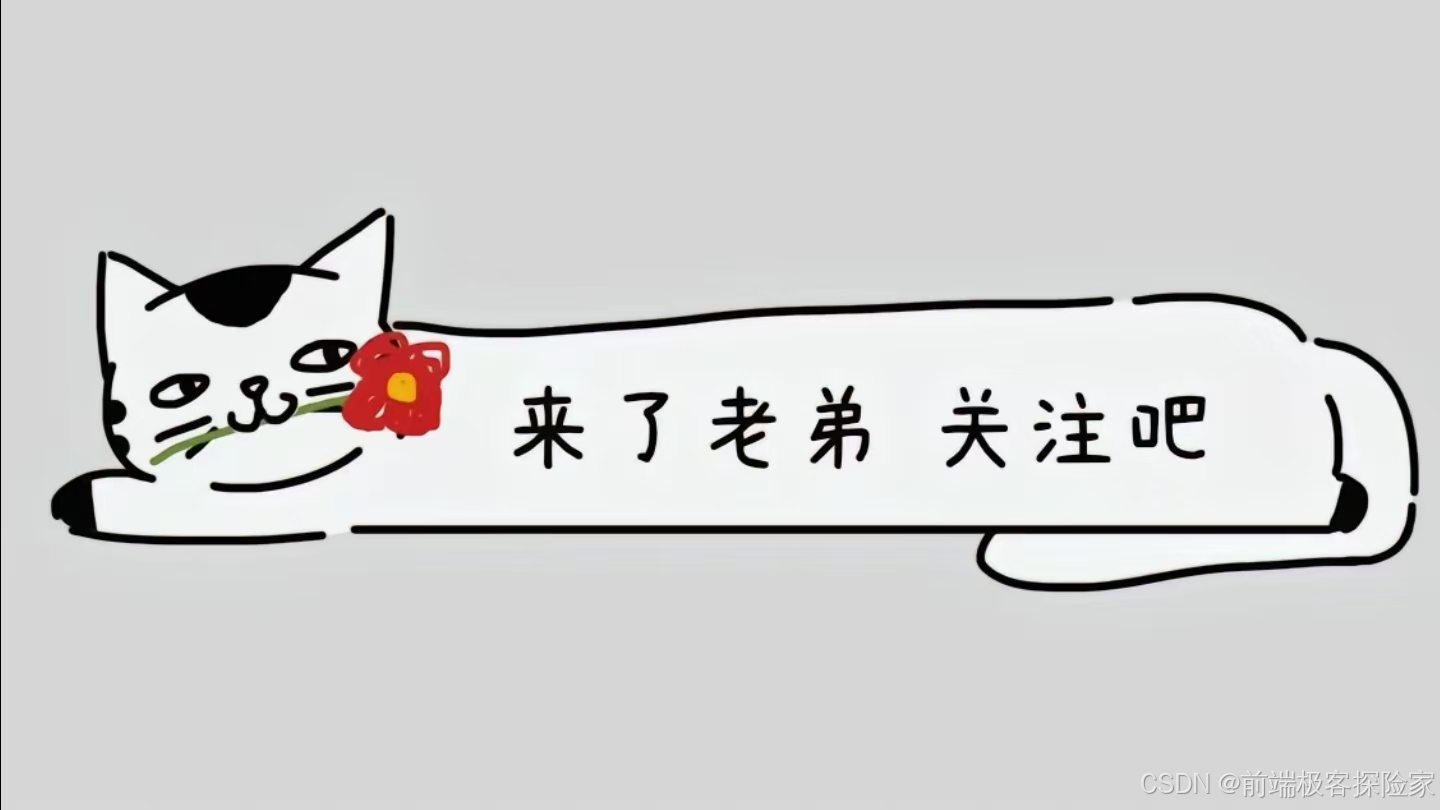