计算机视觉是人工智能领域最具影响力的分支之一,而TensorFlow作为当前最强大的深度学习框架,为计算机视觉任务提供了完整的解决方案。本文将深入探讨TensorFlow在计算机视觉中的应用,从基础概念到最新技术,配合大量代码示例和详细解释,最后提供一个完整的、可立即运行的图像分类系统。
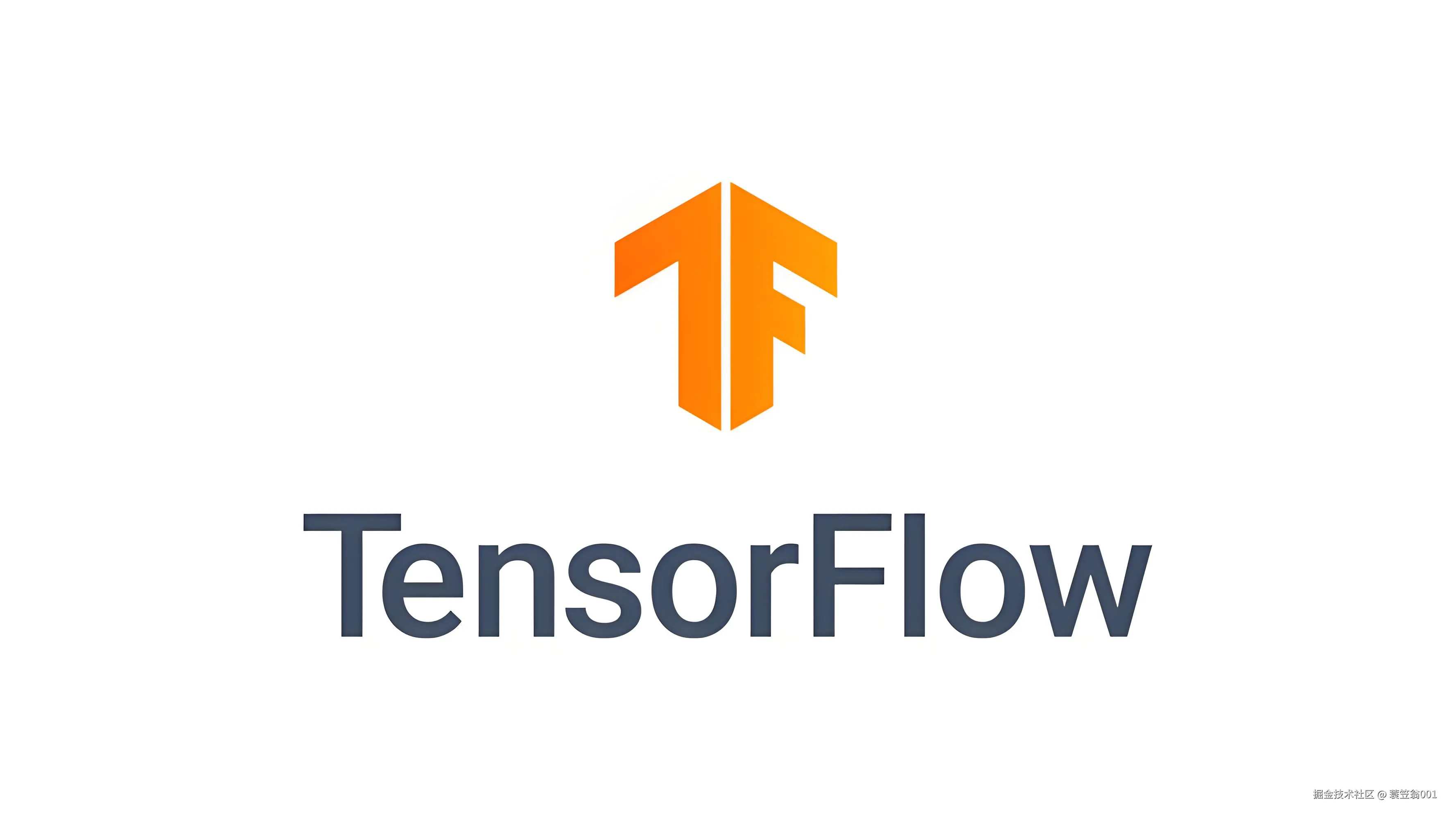
1. 计算机视觉基础与TensorFlow架构
1.1 计算机视觉的核心任务
计算机视觉主要解决以下几类问题:
- 图像分类:识别图像中的主要对象(如猫、狗分类)
- 目标检测:定位并识别图像中的多个对象
- 语义分割:对图像中的每个像素进行分类
- 实例分割:区分同类对象的不同实例
- 图像生成:使用GAN等模型生成新图像
1.2 TensorFlow的计算机视觉栈
TensorFlow为计算机视觉提供了多层次的API支持:
python
import tensorflow as tf
from tensorflow.keras import layers, models, applications
import tensorflow_datasets as tfds
import matplotlib.pyplot as plt
import numpy as np
print("TensorFlow版本:", tf.__version__)
print("GPU可用:", tf.config.list_physical_devices('GPU'))
架构层次说明:
- 底层API:提供张量操作、自动微分等基础功能
- 中层API:Keras层接口,构建模型组件
- 高层API:预建模型和完整工作流
- 工具链:数据管道、可视化、部署工具
2. 数据管道构建与优化
2.1 高效数据加载
python
def load_data(batch_size=64):
# 加载CIFAR-10数据集
(train_ds, test_ds), ds_info = tfds.load(
'cifar10',
split=['train', 'test'],
shuffle_files=True,
as_supervised=True,
with_info=True
)
# 数据预处理函数
def preprocess(image, label):
# 归一化像素值
image = tf.cast(image, tf.float32) / 255.0
# 简单标准化
mean = tf.constant([0.4914, 0.4822, 0.4465])
std = tf.constant([0.2023, 0.1994, 0.2010])
image = (image - mean) / std
return image, label
# 数据增强函数
def augment(image, label):
# 随机水平翻转
image = tf.image.random_flip_left_right(image)
# 随机亮度调整
image = tf.image.random_brightness(image, max_delta=0.2)
# 随机对比度调整
image = tf.image.random_contrast(image, lower=0.8, upper=1.2)
# 随机旋转(-15°到+15°)
image = tf.keras.preprocessing.image.random_rotation(image, 15, row_axis=0, col_axis=1, channel_axis=2)
return image, label
# 构建训练数据管道
train_ds = train_ds.map(preprocess, num_parallel_calls=tf.data.AUTOTUNE)
train_ds = train_ds.map(augment, num_parallel_calls=tf.data.AUTOTUNE)
train_ds = train_ds.shuffle(buffer_size=1000).batch(batch_size).prefetch(tf.data.AUTOTUNE)
# 构建测试数据管道
test_ds = test_ds.map(preprocess, num_parallel_calls=tf.data.AUTOTUNE)
test_ds = test_ds.batch(batch_size).prefetch(tf.data.AUTOTUNE)
return train_ds, test_ds, ds_info
关键技术解析:
-
数据归一化与标准化:
- 归一化将像素值缩放到[0,1]范围
- 标准化进一步调整数据分布(均值0,方差1)
-
数据增强技术:
- 空间变换:翻转、旋转
- 颜色变换:亮度、对比度调整
- 正则化效果:防止过拟合
-
高效数据管道:
map
:并行数据预处理shuffle
:打乱数据顺序prefetch
:重叠数据预处理和模型执行
2.2 数据可视化
python
def visualize_data(dataset, ds_info, num_samples=9):
class_names = ds_info.features['label'].names
plt.figure(figsize=(10, 10))
for i, (image, label) in enumerate(dataset.take(num_samples)):
image = image.numpy()
# 反标准化显示
mean = np.array([0.4914, 0.4822, 0.4465])
std = np.array([0.2023, 0.1994, 0.2010])
image = std * image + mean
image = np.clip(image, 0, 1)
plt.subplot(3, 3, i+1)
plt.imshow(image)
plt.title(class_names[label.numpy()])
plt.axis('off')
plt.tight_layout()
plt.show()
3. 模型构建与训练
3.1 自定义CNN模型
python
def build_cnn_model(input_shape=(32, 32, 3), num_classes=10):
model = models.Sequential([
# 卷积块1
layers.Conv2D(32, (3, 3), activation='relu', padding='same',
input_shape=input_shape,
kernel_regularizer=tf.keras.regularizers.l2(1e-4)),
layers.BatchNormalization(),
layers.Conv2D(32, (3, 3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.MaxPooling2D((2, 2)),
layers.Dropout(0.2),
# 卷积块2
layers.Conv2D(64, (3, 3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.Conv2D(64, (3, 3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.MaxPooling2D((2, 2)),
layers.Dropout(0.3),
# 卷积块3
layers.Conv2D(128, (3, 3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.Conv2D(128, (3, 3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.MaxPooling2D((2, 2)),
layers.Dropout(0.4),
# 分类头
layers.Flatten(),
layers.Dense(128, activation='relu'),
layers.BatchNormalization(),
layers.Dropout(0.5),
layers.Dense(num_classes)
])
# 自定义学习率调度
lr_schedule = tf.keras.optimizers.schedules.ExponentialDecay(
initial_learning_rate=1e-3,
decay_steps=10000,
decay_rate=0.9)
optimizer = tf.keras.optimizers.Adam(learning_rate=lr_schedule)
model.compile(
optimizer=optimizer,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy']
)
return model
模型设计要点:
-
卷积块结构:
- 每个块包含2个卷积层
- 使用批归一化加速收敛
- 最大池化降低空间维度
-
正则化技术:
- L2权重正则化
- Dropout层防止过拟合
- 数据增强(前文实现)
-
优化策略:
- 指数衰减学习率
- Adam优化器自适应调整
3.2 迁移学习实现
python
def build_transfer_model(input_shape=(32, 32, 3), num_classes=10):
# 加载预训练的EfficientNetB0(不包括顶层)
base_model = applications.EfficientNetB0(
input_shape=input_shape,
include_top=False,
weights='imagenet'
)
# 冻结基础模型
base_model.trainable = False
# 自定义顶层
inputs = tf.keras.Input(shape=input_shape)
x = base_model(inputs, training=False)
x = layers.GlobalAveragePooling2D()(x)
x = layers.Dense(256, activation='relu')(x)
x = layers.Dropout(0.5)(x)
outputs = layers.Dense(num_classes)(x)
model = tf.keras.Model(inputs, outputs)
# 使用较低的学习率
optimizer = tf.keras.optimizers.Adam(learning_rate=1e-4)
model.compile(
optimizer=optimizer,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy']
)
return model
迁移学习关键点:
-
预训练模型选择:
- EfficientNet在精度和效率间取得平衡
- 输入尺寸调整为32x32适应CIFAR-10
-
微调策略:
- 初始阶段冻结特征提取器
- 后期可解冻部分层进行微调
-
学习率调整:
- 使用较小的学习率保护预训练权重
4. 模型训练与评估
4.1 训练过程实现
python
def train_model(model, train_ds, test_ds, epochs=50):
# 回调函数配置
callbacks = [
# 早停策略
tf.keras.callbacks.EarlyStopping(
monitor='val_loss',
patience=10,
restore_best_weights=True
),
# 学习率衰减
tf.keras.callbacks.ReduceLROnPlateau(
monitor='val_loss',
factor=0.5,
patience=3,
min_lr=1e-6
),
# TensorBoard日志
tf.keras.callbacks.TensorBoard(
log_dir='./logs',
histogram_freq=1,
profile_batch='500,520'
),
# 模型检查点
tf.keras.callbacks.ModelCheckpoint(
filepath='best_model.h5',
monitor='val_accuracy',
save_best_only=True,
mode='max'
)
]
# 训练模型
history = model.fit(
train_ds,
validation_data=test_ds,
epochs=epochs,
callbacks=callbacks,
verbose=1
)
return history
训练优化策略:
-
动态学习率调整:
- ReduceLROnPlateau根据验证损失自动调整
- 防止训练后期振荡
-
模型保存与恢复:
- 保存验证集上表现最好的模型
- 早停防止过拟合
-
训练监控:
- TensorBoard提供完整的训练可视化
4.2 评估与可视化
python
def evaluate_model(model, test_ds, ds_info):
# 评估模型
test_loss, test_acc = model.evaluate(test_ds, verbose=0)
print(f"测试准确率: {test_acc:.4f}")
print(f"测试损失: {test_loss:.4f}")
# 可视化预测结果
class_names = ds_info.features['label'].names
plt.figure(figsize=(15, 15))
for images, labels in test_ds.take(1):
predictions = model.predict(images)
pred_labels = tf.argmax(predictions, axis=1)
for i in range(25):
plt.subplot(5, 5, i+1)
image = images[i].numpy()
# 反标准化显示
mean = np.array([0.4914, 0.4822, 0.4465])
std = np.array([0.2023, 0.1994, 0.2010])
image = std * image + mean
image = np.clip(image, 0, 1)
plt.imshow(image)
true_label = class_names[labels[i]]
pred_label = class_names[pred_labels[i]]
color = 'green' if true_label == pred_label else 'red'
plt.title(f"True: {true_label}\nPred: {pred_label}", color=color)
plt.axis('off')
plt.tight_layout()
plt.show()
def plot_training_history(history):
plt.figure(figsize=(12, 5))
# 准确率曲线
plt.subplot(1, 2, 1)
plt.plot(history.history['accuracy'], label='训练准确率')
plt.plot(history.history['val_accuracy'], label='验证准确率')
plt.xlabel('Epoch')
plt.ylabel('Accuracy')
plt.ylim([0, 1])
plt.legend()
# 损失曲线
plt.subplot(1, 2, 2)
plt.plot(history.history['loss'], label='训练损失')
plt.plot(history.history['val_loss'], label='验证损失')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.tight_layout()
plt.show()
5. 完整可执行代码
python
import tensorflow as tf
from tensorflow.keras import layers, models, applications, callbacks
import tensorflow_datasets as tfds
import matplotlib.pyplot as plt
import numpy as np
# 1. 数据准备
def prepare_data(batch_size=64):
(train_ds, test_ds), ds_info = tfds.load(
'cifar10',
split=['train', 'test'],
shuffle_files=True,
as_supervised=True,
with_info=True
)
def preprocess(image, label):
image = tf.cast(image, tf.float32) / 255.0
mean = tf.constant([0.4914, 0.4822, 0.4465])
std = tf.constant([0.2023, 0.1994, 0.2010])
image = (image - mean) / std
return image, label
def augment(image, label):
image = tf.image.random_flip_left_right(image)
image = tf.image.random_brightness(image, max_delta=0.2)
image = tf.image.random_contrast(image, lower=0.8, upper=1.2)
return image, label
train_ds = train_ds.map(preprocess).map(augment)
train_ds = train_ds.shuffle(1000).batch(batch_size).prefetch(tf.data.AUTOTUNE)
test_ds = test_ds.map(preprocess)
test_ds = test_ds.batch(batch_size).prefetch(tf.data.AUTOTUNE)
return train_ds, test_ds, ds_info
# 2. 模型构建
def build_enhanced_cnn(input_shape=(32, 32, 3), num_classes=10):
model = models.Sequential([
layers.Conv2D(32, (3,3), activation='relu', padding='same', input_shape=input_shape),
layers.BatchNormalization(),
layers.Conv2D(32, (3,3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.MaxPooling2D((2,2)),
layers.Dropout(0.2),
layers.Conv2D(64, (3,3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.Conv2D(64, (3,3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.MaxPooling2D((2,2)),
layers.Dropout(0.3),
layers.Conv2D(128, (3,3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.Conv2D(128, (3,3), activation='relu', padding='same'),
layers.BatchNormalization(),
layers.MaxPooling2D((2,2)),
layers.Dropout(0.4),
layers.Flatten(),
layers.Dense(128, activation='relu'),
layers.BatchNormalization(),
layers.Dropout(0.5),
layers.Dense(num_classes)
])
optimizer = tf.keras.optimizers.Adam(learning_rate=1e-3)
model.compile(optimizer=optimizer,
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
return model
# 3. 训练与评估
def main():
# 准备数据
train_ds, test_ds, ds_info = prepare_data()
# 可视化样本数据
print("数据集信息:")
print(f"训练样本数: {ds_info.splits['train'].num_examples}")
print(f"测试样本数: {ds_info.splits['test'].num_examples}")
print("类别:", ds_info.features['label'].names)
# 构建模型
model = build_enhanced_cnn()
model.summary()
# 训练模型
print("\n开始训练...")
history = model.fit(
train_ds,
validation_data=test_ds,
epochs=50,
callbacks=[
callbacks.EarlyStopping(patience=10, restore_best_weights=True),
callbacks.ReduceLROnPlateau(factor=0.5, patience=3, min_lr=1e-6),
callbacks.TensorBoard(log_dir='./logs')
]
)
# 评估模型
print("\n评估模型...")
test_loss, test_acc = model.evaluate(test_ds, verbose=0)
print(f"测试准确率: {test_acc:.4f}")
print(f"测试损失: {test_loss:.4f}")
# 可视化结果
plot_training_history(history)
def plot_training_history(history):
plt.figure(figsize=(12, 5))
plt.subplot(1, 2, 1)
plt.plot(history.history['accuracy'], label='训练准确率')
plt.plot(history.history['val_accuracy'], label='验证准确率')
plt.title('模型准确率')
plt.ylabel('准确率')
plt.xlabel('Epoch')
plt.legend()
plt.subplot(1, 2, 2)
plt.plot(history.history['loss'], label='训练损失')
plt.plot(history.history['val_loss'], label='验证损失')
plt.title('模型损失')
plt.ylabel('损失')
plt.xlabel('Epoch')
plt.legend()
plt.tight_layout()
plt.show()
if __name__ == "__main__":
main()
6. 项目扩展与优化方向
6.1 高级模型架构
python
def build_resnet_style_model(input_shape=(32, 32, 3), num_classes=10):
def residual_block(x, filters, strides=1):
shortcut = x
x = layers.Conv2D(filters, (3,3), strides=strides, padding='same')(x)
x = layers.BatchNormalization()(x)
x = layers.Activation('relu')(x)
x = layers.Conv2D(filters, (3,3), padding='same')(x)
x = layers.BatchNormalization()(x)
if strides > 1:
shortcut = layers.Conv2D(filters, (1,1), strides=strides)(shortcut)
shortcut = layers.BatchNormalization()(shortcut)
x = layers.add([x, shortcut])
x = layers.Activation('relu')(x)
return x
inputs = tf.keras.Input(shape=input_shape)
x = layers.Conv2D(32, (3,3), padding='same')(inputs)
x = layers.BatchNormalization()(x)
x = layers.Activation('relu')(x)
x = residual_block(x, 32)
x = residual_block(x, 32)
x = residual_block(x, 64, strides=2)
x = residual_block(x, 64)
x = residual_block(x, 128, strides=2)
x = residual_block(x, 128)
x = layers.GlobalAveragePooling2D()(x)
outputs = layers.Dense(num_classes)(x)
model = tf.keras.Model(inputs, outputs)
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
return model
6.2 混合精度训练
python
policy = tf.keras.mixed_precision.Policy('mixed_float16')
tf.keras.mixed_precision.set_global_policy(policy)
# 注意:最后一层需要保持float32以保证数值稳定性
model = build_enhanced_cnn()
model.layers[-1].dtype_policy = tf.keras.mixed_precision.Policy('float32')
6.3 模型量化与部署
python
# 转换为TFLite格式
converter = tf.lite.TFLiteConverter.from_keras_model(model)
converter.optimizations = [tf.lite.Optimize.DEFAULT]
tflite_model = converter.convert()
# 保存量化模型
with open('quantized_model.tflite', 'wb') as f:
f.write(tflite_model)
# 在移动端加载模型
interpreter = tf.lite.Interpreter(model_content=tflite_model)
interpreter.allocate_tensors()
7. 总结与展望
本文全面介绍了使用TensorFlow实现计算机视觉任务的完整流程:
- 数据准备:高效数据管道构建与增强技术
- 模型设计:从基础CNN到ResNet风格架构
- 训练优化:学习率调度、早停等高级技巧
- 评估部署:模型量化与移动端部署
进一步学习方向:
- Transformer在CV中的应用(ViT、Swin Transformer)
- 自监督学习(SimCLR、MoCo)
- 实时目标检测(YOLOv5、EfficientDet)
- 模型解释性(Grad-CAM、SHAP)
通过这个完整的项目,您已经掌握了TensorFlow计算机视觉的核心技术栈,可以在此基础上探索更复杂的应用场景。