文章目录
- 平方数(数学)
- DNA序列(固定长度的滑动窗口)
- [压缩字符串 (双指针 + 模拟)](#压缩字符串 (双指针 + 模拟))
- [chika和蜜柑 (top k问题 + 排序 + pair)](#chika和蜜柑 (top k问题 + 排序 + pair))
平方数(数学)
题解
- 先将x开根号,开完根号会去掉小数点后面的数,开完根号后的数乘开完根号后的数,和x相减,开完根号后的数加1翻倍,和x相减,比较相减后的数哪个数小,就选那个最近的数
代码
cpp
#include<iostream>
#include<math.h>
using namespace std;
int main()
{
long long x;
cin >> x;
long long y = ((long long)sqrt(x)) * ((long long)sqrt(x));
long long p = (long long)sqrt(x) + 1;
long long k = p * p;
if(abs(y - x) < abs(k - x))
{
cout << y << '\n';
}
else cout << k << '\n';
return 0;
}
DNA序列(固定长度的滑动窗口)
题解
- 固定长度的滑动窗口
- 进窗口,判断,出窗口,更新结果
- 进窗口:统计统计C和G的个数,right++,判断和出窗口:如果left下标的数等于C或者是G,count--,left++,更新结果:长度等于x,更新结果
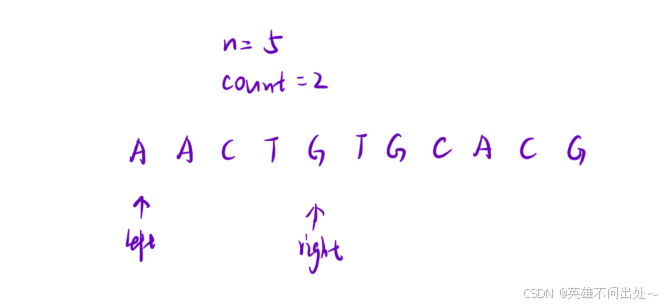
代码
cpp
// 因为忘了substr没写出来,想出来用滑动窗口
// 把题目还理解为必须要至少一个C和一个G
#include <iostream>
#include<string>
using namespace std;
int main()
{
string s;
int x;
cin >> s >> x;
int n = s.size();
int left = 0,right = 0;
int count = 0;
string t;
int ans = 0;
while(right < n)
{
if(s[right] == 'C' || s[right] == 'G')
{
count++;
}
if(right - left + 1 == x)
{
if(count > ans)
{
t = s.substr(left,x);
ans = count;
}
if(s[left] == 'C' || s[left] == 'G')
{
count--;
}
left++;
}
if(right - left + 1 < x)
right++;
}
cout << t << '\n';
return 0;
}
压缩字符串 (双指针 + 模拟)
题解
- 双指针 + 模拟
- 先让left和right都指向起点,如果right+1位置的数不等于right位置的数,或者是right的下一个位置的数越界了,更新字符串,再让right += 1,left = right,继续上面的操作
代码
cpp
class Solution
{
public:
string compressString(string param)
{
// 写错了,写成了处理边界情况的那种了
// left下标和right下标不相等才更新字符串
int left = 0,right = 0,n = param.size();
string t;
while(right < n)
{
while(right + 1 < n && param[right] == param[right+1]) right++;
int len = right - left + 1;
t += param[left];
if(len > 1) t += to_string(len);
right = right + 1;
left = right;
}
return t;
}
};
chika和蜜柑 (top k问题 + 排序 + pair)
题解
- topk问题 + 排序
- 首先是让甜度尽可能大,酸度尽可能小
- 甜度不同时,比较甜度,甜度大的在前
甜度相同时,比较酸度,酸度小的在前 - 这样有两个数据,需要使用pair键值对
代码
cpp
#include<iostream>
#include<algorithm>
using namespace std;
int n,k;
typedef pair<int,int> PII; // <酸度,甜度>
const int N = 2e5 + 10;
PII arr[N];
int main()
{
cin >> n >> k;
for(int i = 0;i < n;i++) cin >> arr[i].first;
for(int i = 0;i < n;i++) cin >> arr[i].second;
sort(arr,arr+n,[&](const PII& a,const PII& b)
{
if(a.second != b.second) return a.second > b.second;
else return a.first < b.first;
});
long long t = 0;
long long s = 0;
for(int i = 0;i < k;i++)
{
s += arr[i].first;
t += arr[i].second;
}
cout << s << " " << t << '\n';
return 0;
}