应用场景与需求分析
在移动端应用开发中,倒计时功能是常见的交互需求,例如限时促销活动、订单超时提醒、考试倒计时等场景。本文将基于 Uniapp 框架,实现一个从当前日期到给定日期的倒计时组件,支持显示 HH:mm:ss 格式的剩余时间,并具备自动更新和资源释放机制。
实现思路
- 通过 props 接收目标时间字符串(如 '2024-12-31 23:59:59')
- 在组件挂载时启动定时器,每秒计算当前时间与目标时间的差值
- 使用 dayjs 的 duration 方法将时间差格式化为 HH:mm:ss
- 当时间差小于等于 0 时,清除定时器并显示 00:00:00
- 组件销毁前清除定时器,避免内存泄漏
完整代码
javascript
<template>
<view class="count-down">
<text>剩余时间:{{ timeData }}</text>
</view>
</template>
<script>
import dayjs from 'dayjs'
import duration from 'dayjs/plugin/duration'
dayjs.extend(duration)
export default {
props: {
time: {
type: String,
default: ''
}
},
data() {
return {
timeData: '00:00:00',
timer: null
}
},
mounted() {
if (this.time) {
this.updateTime()
this.timer = setInterval(this.updateTime, 1000)
}
},
beforeDestroy() {
if (this.timer) {
clearInterval(this.timer)
}
},
methods: {
updateTime() {
const diff = dayjs(this.time).diff(dayjs())
this.timeData =
diff > 0 ? dayjs.duration(diff).format('HH:mm:ss') : '00:00:00'
if (diff <= 0) {
clearInterval(this.timer)
}
}
}
}
</script>
<style scoped lang="scss">
.count-down {
color: gold;
}
</style>
使用
javascript
<c-count-down :time="time"></c-count-down>
效果展示
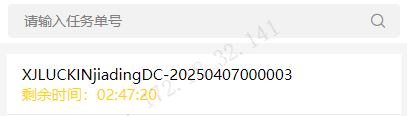