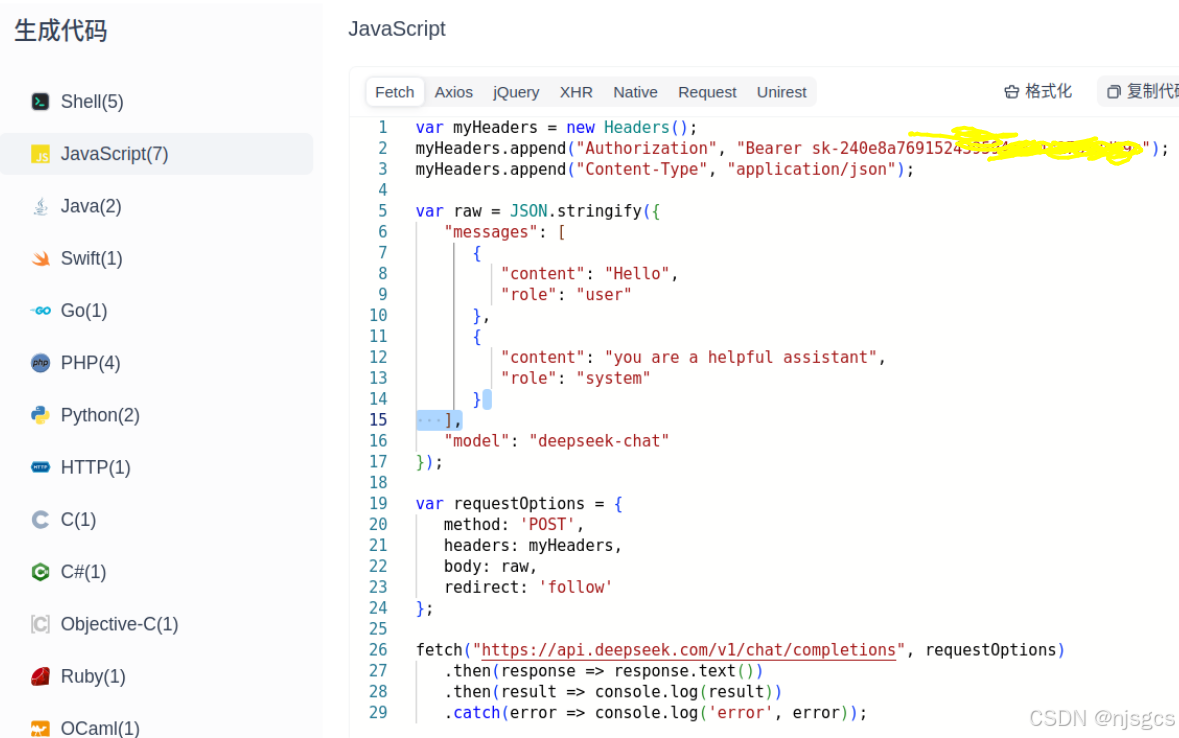
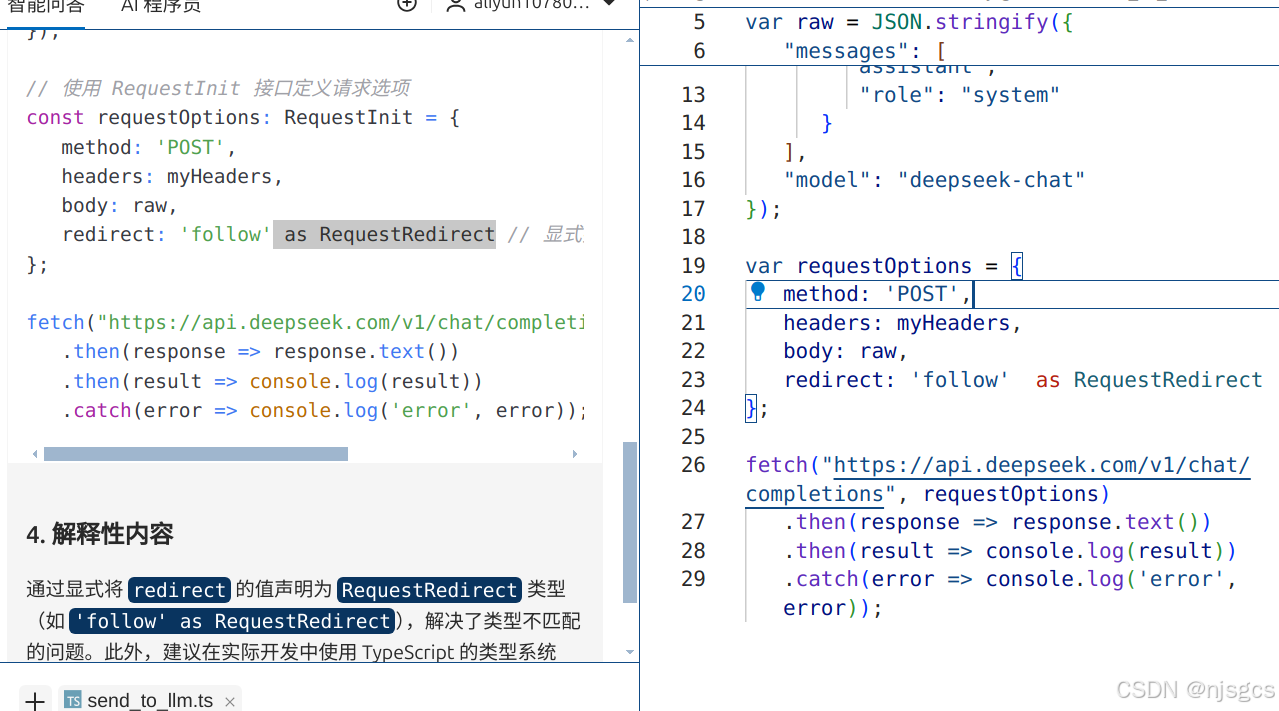
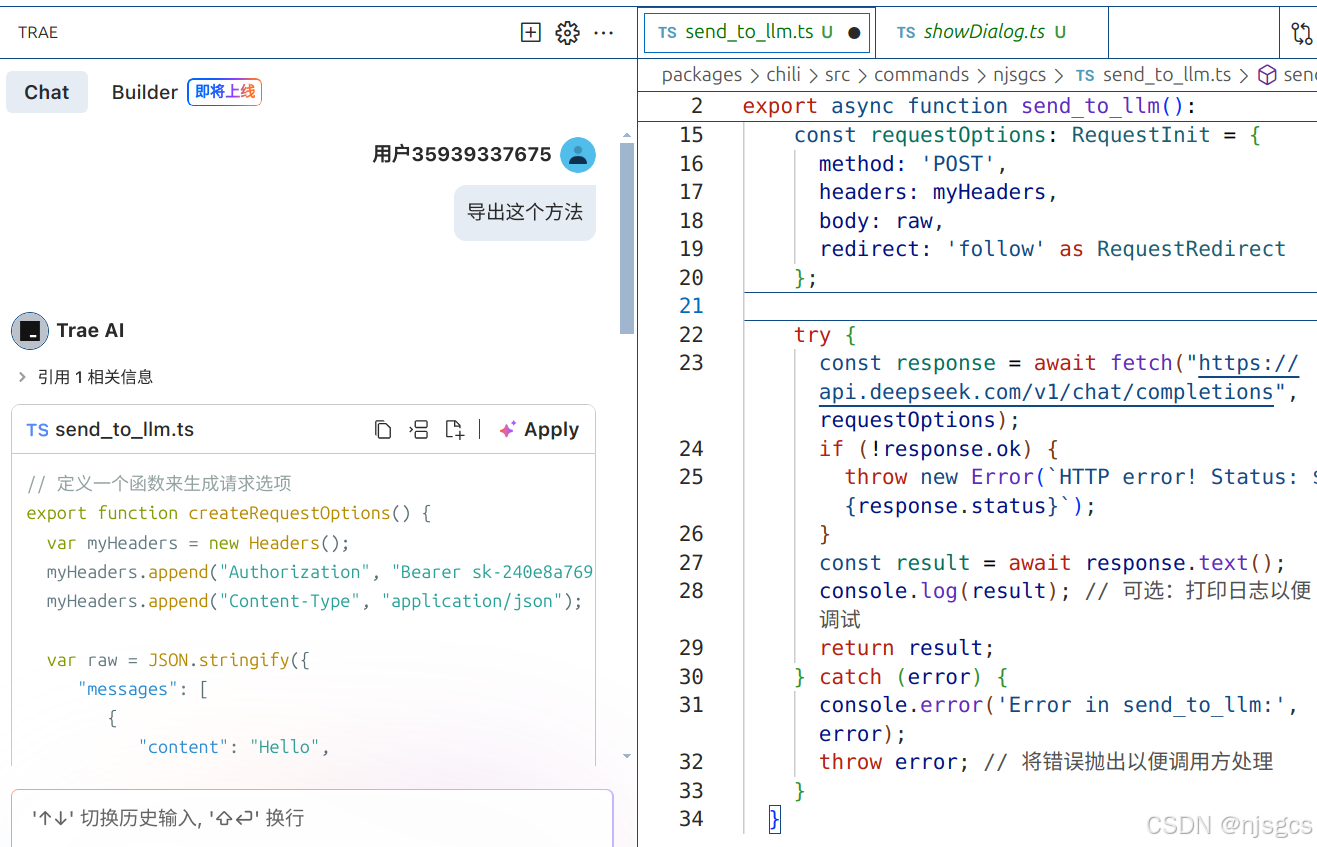
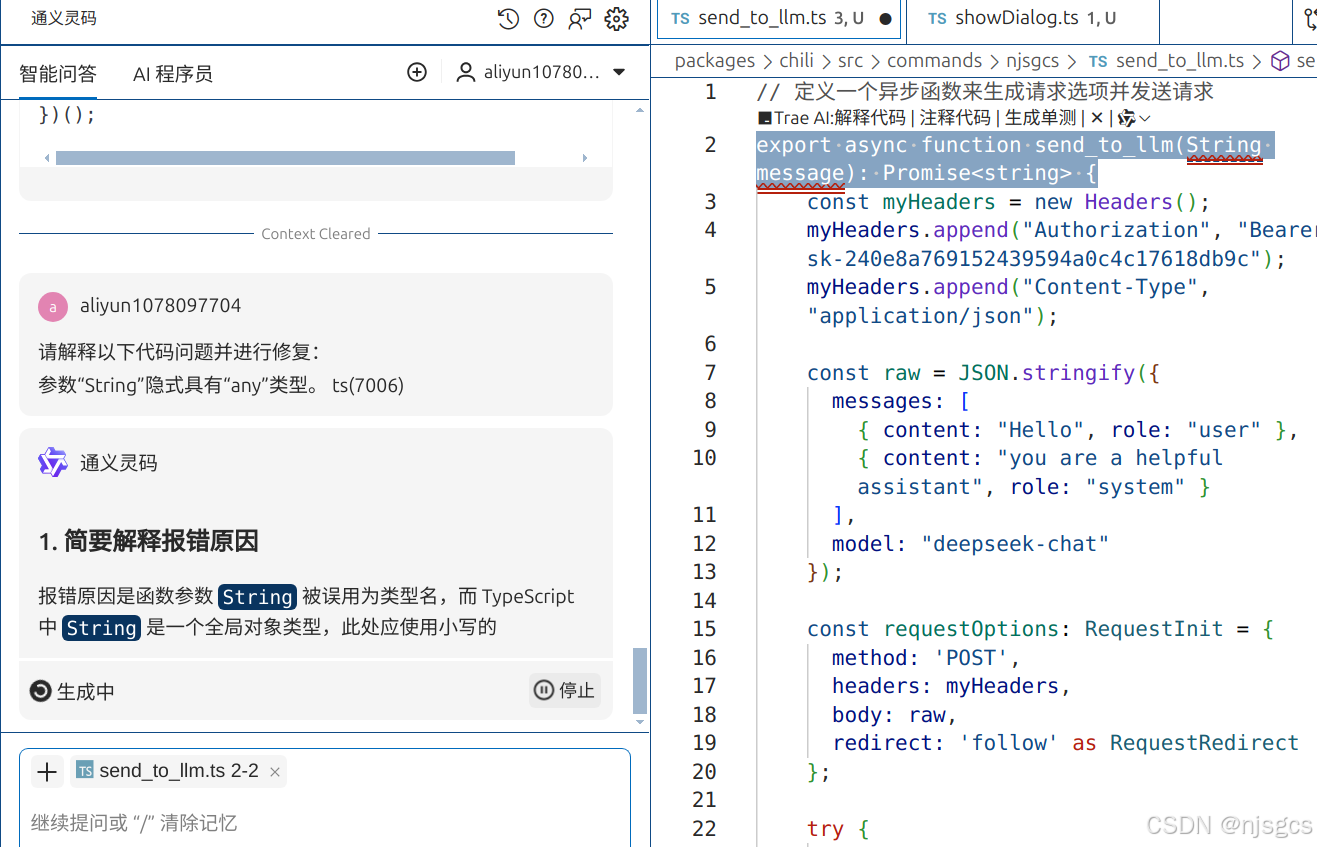
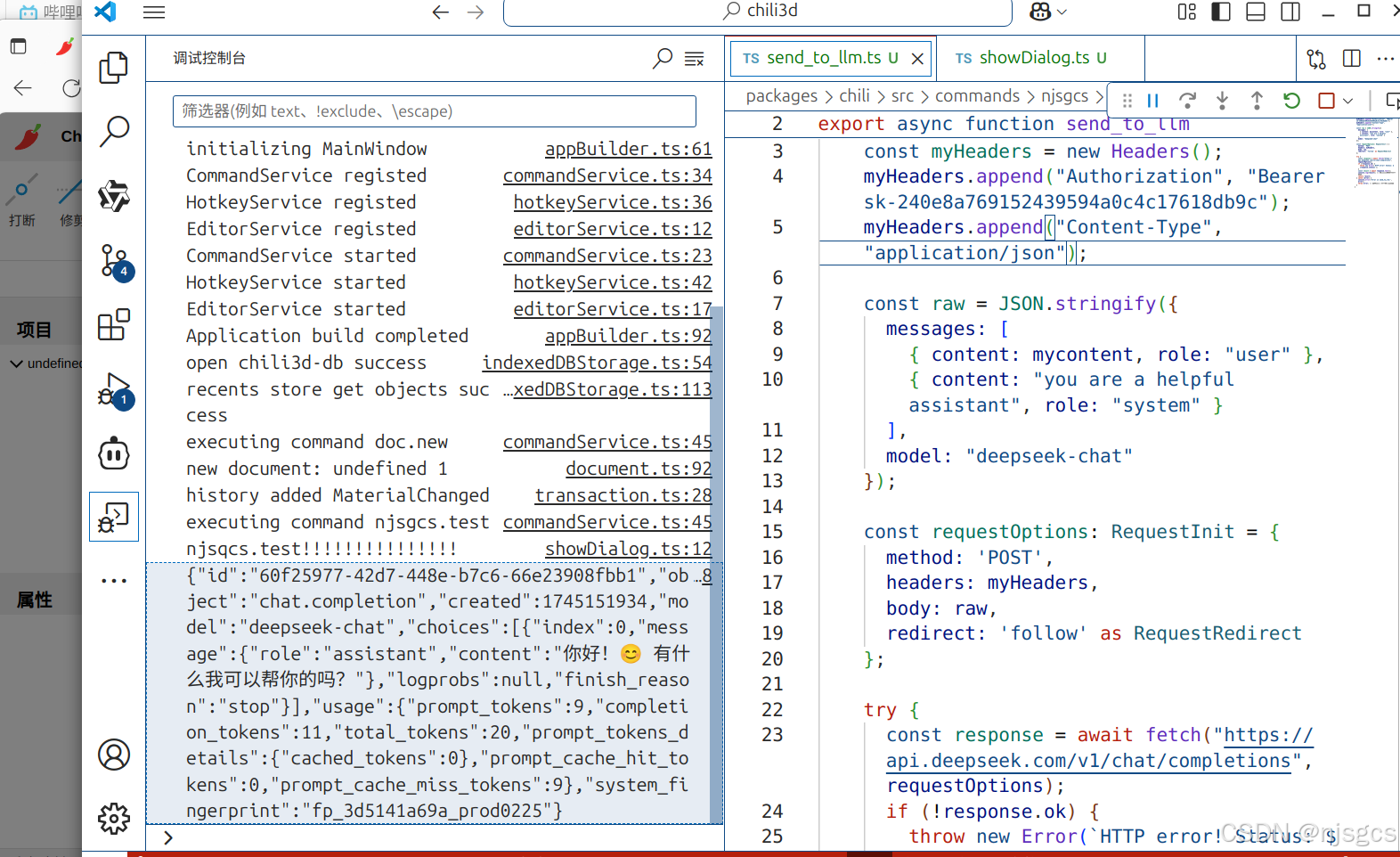
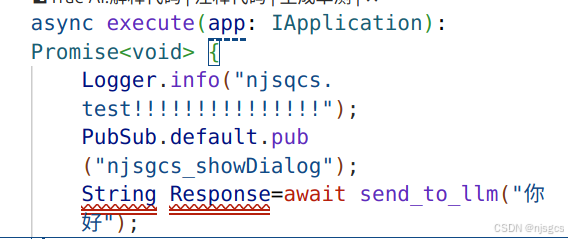
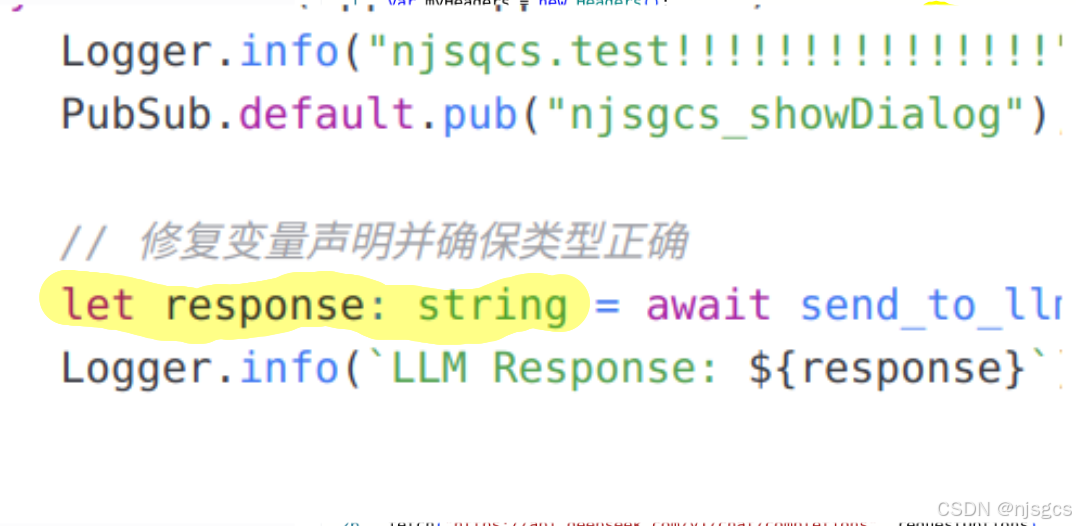
TypeScript
// 定义一个异步函数来生成请求选项并发送请求
export async function send_to_llm(mycontent:String ): Promise<string> {
const myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer sk-240e8a769152439594a0c4c17618db9c");
myHeaders.append("Content-Type", "application/json");
const raw = JSON.stringify({
messages: [
{ content: mycontent, role: "user" },
{ content: "you are a helpful assistant", role: "system" }
],
model: "deepseek-chat"
});
const requestOptions: RequestInit = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow' as RequestRedirect
};
try {
const response = await fetch("https://api.deepseek.com/v1/chat/completions", requestOptions);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const result = await response.text();
console.log(result); // 可选:打印日志以便调试
return result;
} catch (error) {
console.error('Error in send_to_llm:', error);
throw error; // 将错误抛出以便调用方处理
}
}

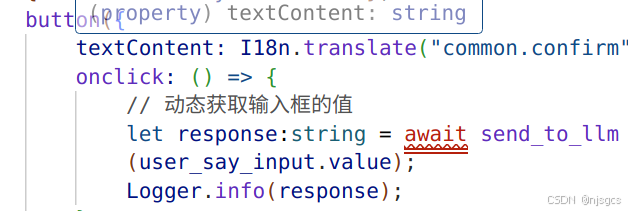

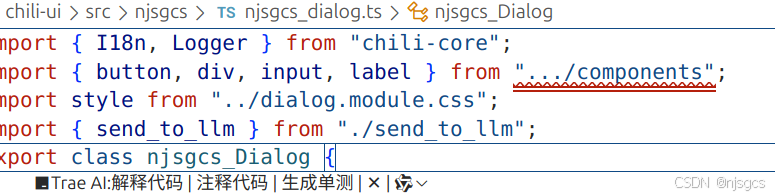

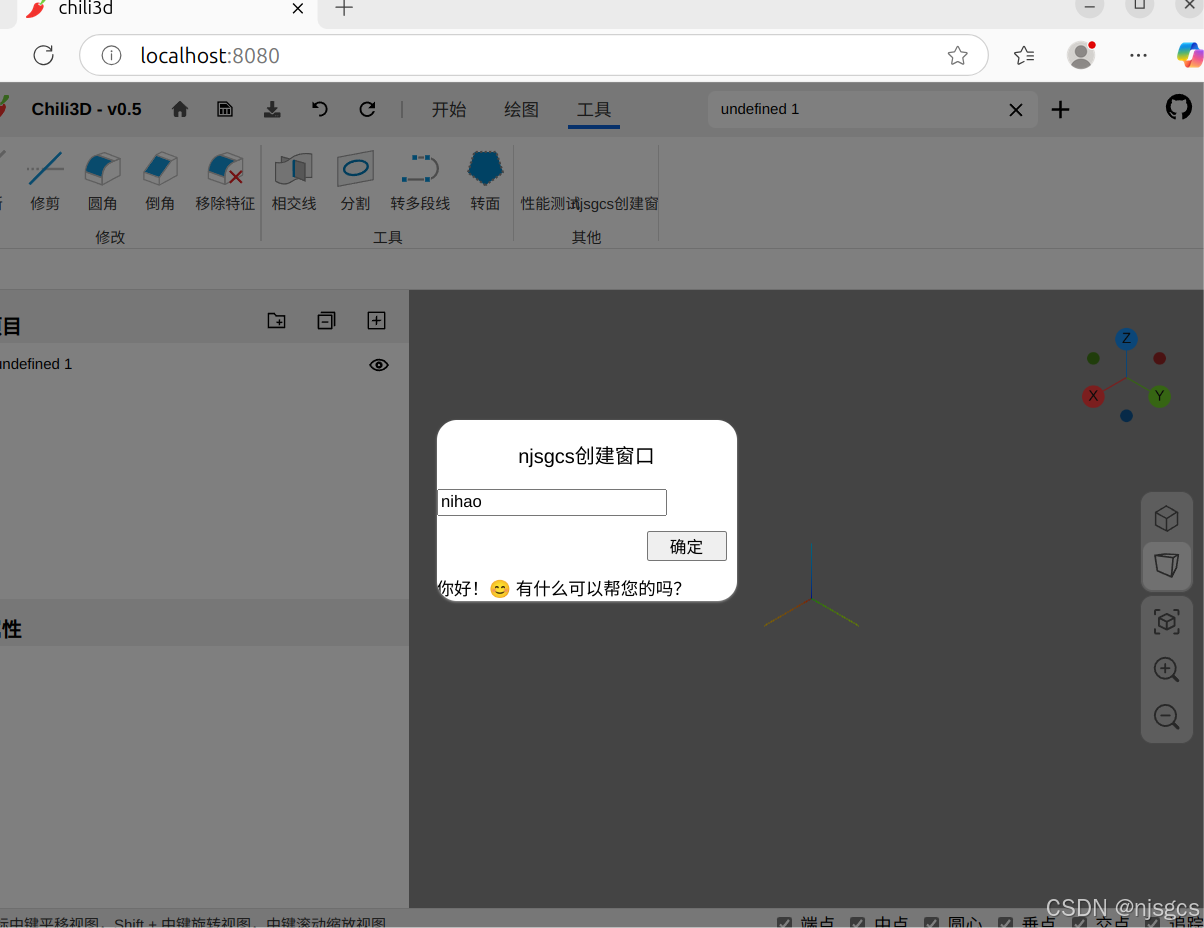
TypeScript
import { I18n, Logger } from "chili-core";
import { button, div, input, label } from "../components";
import style from "../dialog.module.css";
import { send_to_llm } from "./send_to_llm";
export class njsgcs_Dialog {
private constructor() {}
static show() {
const dialog = document.createElement("dialog");
document.body.appendChild(dialog);
// 创建输入框并保存引用
const user_say_input = input({
type: "text",
id: "njsgcs_test_input",
onkeydown: (e: KeyboardEvent) => {
e.stopPropagation();
},
});
// 创建 label 元素并保存引用
const resultLabel = label({ textContent: "" });
dialog.appendChild(
div(
{ className: style.root },
div({ className: style.title }, label({ textContent: I18n.translate("njsgcs_showDialog") })),
div({ className: style.input }, user_say_input),
div(
{ className: style.buttons },
button({
textContent: I18n.translate("common.confirm"),
onclick: async () => {
try {
// 动态获取输入框的值
let response: string = await send_to_llm(user_say_input.value);
// 将 response 解析为 JSON 对象
const jsonResponse = JSON.parse(response);
let content_response: string = jsonResponse.choices[0].message.content;
Logger.info(content_response);
// 将 content_response 赋值给 label
resultLabel.textContent = content_response;
} catch (error) {
Logger.error("Failed to parse response as JSON:", error);
}
},
}),
),
// 添加结果显示区域
div({ className: style.result }, resultLabel)
),
);
dialog.showModal();
}
}