- package.json (main,files,version)
- vite.config.ts (entry)
- npm login npm publish
1. Vite配置 (vite.config.js):javascript
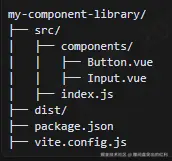
js
import { defineConfig } from 'vite';
import vue from '@vitejs/plugin-vue';
export default defineConfig({
plugins: [vue()],
build: {
lib: {
entry: './src/index.js', // 指定入口文件
name: 'MyComponentLibrary',
fileName: 'index',
formats: ['es', 'umd']
},
rollupOptions: {
external: ['vue'],
output: {
globals: {
vue: 'Vue'
}
}
}
}
});
3. package.json配置:
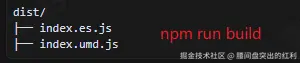
js
{
"name": "my-component-library",
"version": "1.0.0",
"main": "dist/index.umd.js", // UMD格式的入口文件
"module": "dist/index.es.js", // ES模块格式的入口文件
"files": [
"dist"
],
"scripts": {
"build": "vite build",
"publish": "npm publish"
}
}
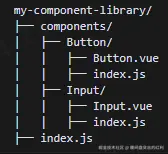
按需导入
js
import { createApp } from 'vue';
import App from './App.vue';
import MyComponentLibrary from 'my-component-library';
const app = createApp(App);
// 按需导入组件
app.use(MyComponentLibrary, {
components: ['Button'] // 只导入Button组件
});
app.mount('#app');
全局导入
js
import { createApp } from 'vue';
import App from './App.vue';
import MyComponentLibrary from 'my-component-library';
const app = createApp(App);
// 全局导入所有组件
app.use(MyComponentLibrary); // 导入并注册所有组件
app.mount('#app')
3. 插件实现
- 为了实现按需导入和全局导入的功能,你需要在你的组件库中提供一个插件,并根据用户的选择来注册组件。
js
import { Button, Input } from './components';
const MyComponentLibrary = {
install(app, options = {}) {
if (options.components) {
// 按需导入
if (options.components.includes('Button')) {
app.component('MyButton', Button);
}
if (options.components.includes('Input')) {
app.component('MyInput', Input);
}
} else {
// 全局导入
app.component('MyButton', Button);
app.component('MyInput', Input);
}
}
};
export default MyComponentLibrary;