- 操作系统:ubuntu22.04
- OpenCV版本:OpenCV4.9
- IDE:Visual Studio Code
- 编程语言:C++11
算法描述
将图像从 RGB 色彩空间转换为灰度。
R、G 和 B 通道值的常规范围是 0 到 255。生成的灰度值计算方式如下:
dst ( I ) = 0.299 ∗ src ( I ) . R + 0.587 ∗ src ( I ) . G + 0.114 ∗ src ( I ) . B \texttt{dst} (I)= \texttt{0.299} * \texttt{src}(I).R + \texttt{0.587} * \texttt{src}(I).G + \texttt{0.114} * \texttt{src}(I).B dst(I)=0.299∗src(I).R+0.587∗src(I).G+0.114∗src(I).B
注意:
函数的文字 ID 是 "org.opencv.imgproc.colorconvert.rgb2gray"
函数原型
cpp
GMat cv::gapi::RGB2Gray
(
const GMat & src
)
参数
- 参数 src: 输入图像,8 位无符号三通道图像 CV_8UC3。
代码示例
cpp
#include <opencv2/opencv.hpp>
#include <opencv2/gapi.hpp>
#include <opencv2/gapi/core.hpp> // 包含核心功能
#include <opencv2/gapi/imgproc.hpp> // 包含图像处理功能
int main() {
// 读取一个RGB图像
cv::Mat rgb_img = cv::imread("/media/dingxin/data/study/OpenCV/sources/images/Lenna.png");
if (rgb_img.empty()) {
std::cerr << "Error: Image not found!" << std::endl;
return -1;
}
// 定义G-API图
cv::GMat src;
auto gray = cv::gapi::RGB2Gray(src);
cv::GComputation comp(cv::GIn(src), cv::GOut(gray));
// 创建输出矩阵
cv::Mat out_gray;
// 应用计算图并执行转换,指定使用默认的CPU后端
comp.apply(cv::gin(rgb_img), cv::gout(out_gray),
cv::compile_args(cv::gapi::kernels()));
// 显示结果
cv::imshow("Original RGB Image", rgb_img);
cv::imshow("Converted Gray Image", out_gray);
cv::waitKey(0);
return 0;
}
运行结果
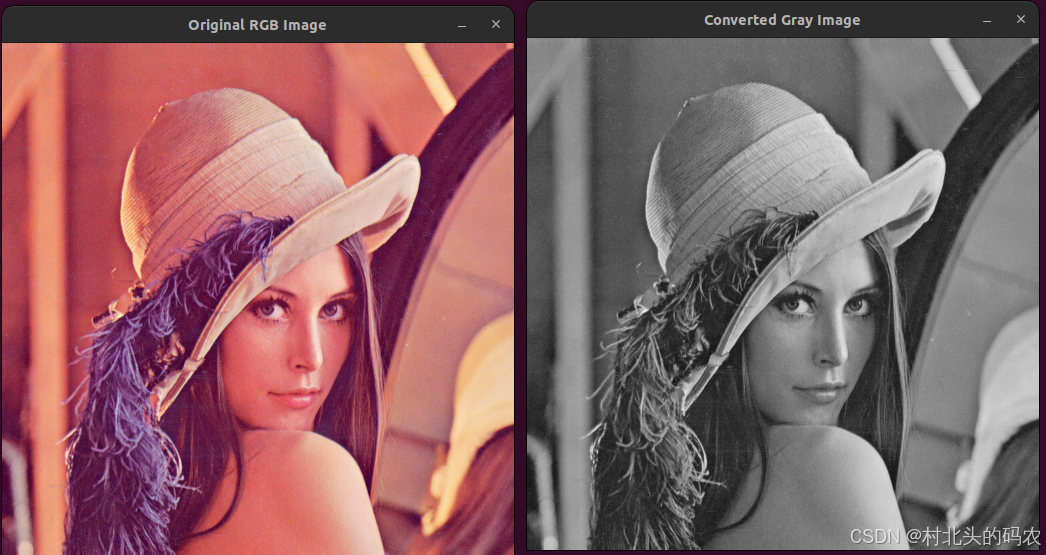