引言💭
主播在面试中屡次碰壁,感觉自己技术不够,刚好金三银四也快结束了,干脆不面了,不管了,向react进军。🚀🚀🚀
学习react需要具备几个JavaScript的知识点,这篇文章详细介绍this的指向。
一、this
的基本概念
在 JavaScript 中,this
是一个指向当前执行环境的特殊对象。具体来说,this
是一个变量,指向执行当前代码的上下文。它的值不固定,取决于代码的调用方式。
1.1 this
在函数中的默认指向
在传统的 JavaScript 函数中,this
默认指向全局对象(在浏览器中是 window
,在 Node.js 中是 global
)。但是,随着严格模式('use strict'
)的引入,this
在函数内的指向变得更加严格。在严格模式下,this
不再指向全局对象,而是 undefined
。
javascript
// 非严格模式
function foo() {
console.log(this); // 在浏览器中会输出 window 对象
}
foo();
// 严格模式
'use strict';
function bar() {
console.log(this); // 输出 undefined
}
bar();
1.2 this
指向对象的方法
当 this
出现在对象的方法中时,它通常指向该对象。也就是说,this
指向方法所属的对象,这也是 JavaScript 中 this
最常见的指向方式。
javascript
const person = {
name: 'Alice',
greet: function() {
console.log(this.name); // 输出 Alice
}
};
person.greet(); // this 指向 person 对象
1.3 this
指向构造函数的实例
当通过构造函数(new
关键字)创建对象时,this
指向该实例对象。构造函数用于创建一个新的对象实例,并通过 this
绑定到新创建的对象。
ini
function Person(name) {
this.name = name;
}
const alice = new Person('Alice');
console.log(alice.name); // 输出 Alice
1.4 this
指向事件处理函数
在事件处理函数中,this
指向触发事件的 DOM 元素。需要注意的是,如果在事件处理函数内使用箭头函数,this
会继承外部上下文的 this
,而不会指向事件的目标元素。
javascript
const button = document.querySelector('button');
// 普通函数
button.addEventListener('click', function() {
console.log(this); // this 指向 button 元素
});
// 箭头函数
button.addEventListener('click', () => {
console.log(this); // this 指向外部上下文,可能是 window 或其他对象
});
二、判断 this
的指向
2.1 通过 console.log
调试
一种最直接的方式是使用 console.log(this)
来输出 this
的指向。无论在函数内部、对象方法中,还是事件处理器中,this
的指向都可以通过输出进行快速判断。
2.2 instanceof
运算符判断对象类型
可以利用 instanceof
运算符来判断 this
是否为某种类型的实例。例如,我们可以判断 this
是否是一个对象实例,或者是否是某个构造函数创建的对象。
javascript
function Car() {
this.make = 'Toyota';
}
const myCar = new Car();
console.log(myCar instanceof Car); // true,myCar 是 Car 的实例
2.3 typeof
判断
typeof
运算符可以用来判断 this
的基本类型。如果 this
是对象类型,则返回 'object'
。
javascript
function test() {
console.log(typeof this); // 如果在全局作用域中调用,返回 'object'
}
test();
2.4 bind
、call
和 apply
改变 this
的指向
JavaScript 提供了 bind
、call
和 apply
方法来显式地改变 this
的指向。通过这些方法,可以在函数调用时指定 this
的指向,从而方便地判断和控制它的行为。
2.4.1 bind
方法
bind
方法返回一个新函数,该函数的 this
被永久绑定到传入的第一个参数。
javascript
const person = {
name: 'Alice',
greet: function() {
console.log(this.name);
}
};
const greetAlice = person.greet.bind(person); // this 永久绑定为 person
greetAlice(); // 输出 Alice
2.4.2 call
和 apply
方法
call
和 apply
方法都用于指定函数执行时的 this
值。不同的是,call
接受参数列表,而 apply
接受一个数组作为参数。
arduino
function greet() {
console.log(this.name);
}
const person = { name: 'Alice' };
greet.call(person); // 输出 Alice
greet.apply(person); // 输出 Alice
三、this
的高级使用
3.1 箭头函数中的 this
箭头函数的一个特点是,它不会创建自己的 this
,而是从外部上下文中继承 this
的值。因此,箭头函数中的 this
值是静态的,而非动态的。
javascript
const obj = {
name: 'Bob',
greet: function() {
setTimeout(() => {
console.log(this.name); // 输出 Bob,箭头函数继承外部 this
}, 1000);
}
};
obj.greet();
3.2 类中的 this
使用
在 ES6 中,引入了类(class
)的概念。类的方法中的 this
指向类的实例对象。需要注意的是,在类方法中使用箭头函数时,this
依然会继承外部上下文,而不指向类的实例。
javascript
class Person {
constructor(name) {
this.name = name;
}
greet() {
setTimeout(() => {
console.log(this.name); // 箭头函数继承了外部的 this
}, 1000);
}
}
const bob = new Person('Bob');
bob.greet(); // 输出 Bob
总结✒️
在 JavaScript 中,this
的指向取决于函数的调用方式。常见的几种情况包括:
- 在全局作用域中,
this
指向全局对象(浏览器中为window
,Node.js 中为global
)。 - 在对象方法中,
this
指向该对象。 - 在构造函数中,
this
指向新创建的实例对象。 - 在事件处理器中,
this
指向触发事件的 DOM 元素。
通过使用
bind
、call
和apply
方法,可以显式地控制this
的指向。此外,箭头函数会继承外部上下文的this
,这使得箭头函数在回调函数中的使用特别方便。
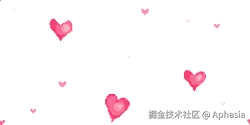