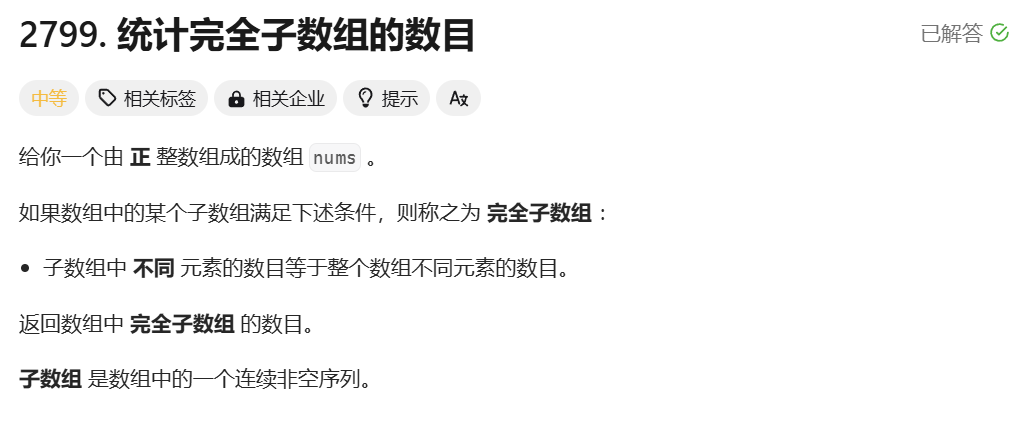
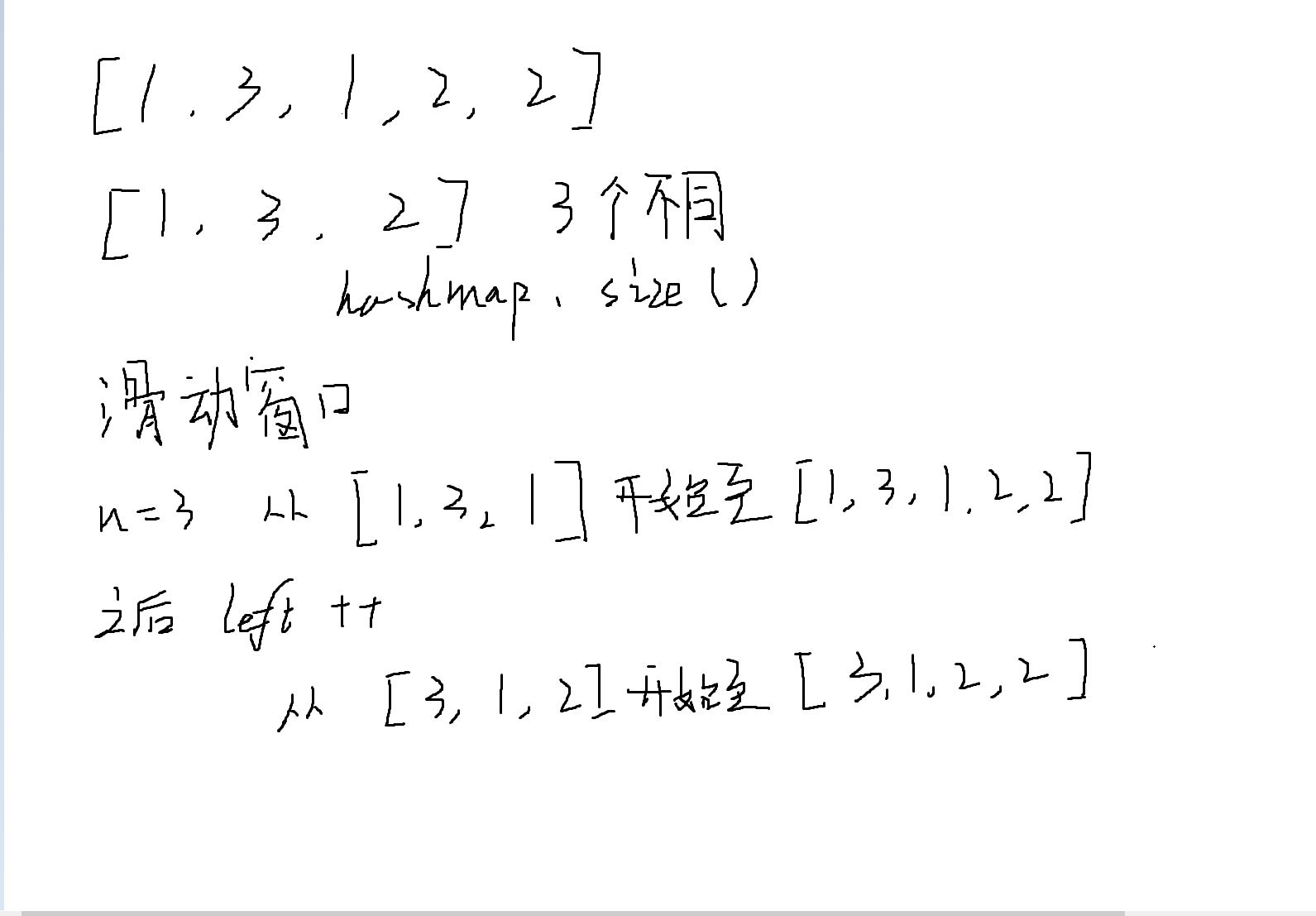
使用hashmap加滑动窗口解决问题
例如List[1,3,1,2,2]
我们通过hashmap.put(nums[i],hashmap.getOrDefault(nums[i],0)+1)
后再通过hashmap.size()
获取键值对的数量,即不同值的数量。
滑动窗口思想如上所示:
class Solution {
public int countCompleteSubarrays(int[] nums) {
int n = 0;
HashMap<Integer,Integer> map = new HashMap<>();
for(int i=0;i<nums.length;i++){
map.put(nums[i],map.getOrDefault(nums[i],0)+1);
}
n = map.size();
System.out.println("n:"+n);
int left = 0;
int right = n;
int total = 0;
while(right<=nums.length){
HashMap<Integer,Integer> hashmap = new HashMap<>();
for(int i=left;i<nums.length;i++){
hashmap.put(nums[i],hashmap.getOrDefault(nums[i],0)+1);
if(hashmap.size()==n) total++;
}
left++;
right = n+left;
}
return total;
}
}
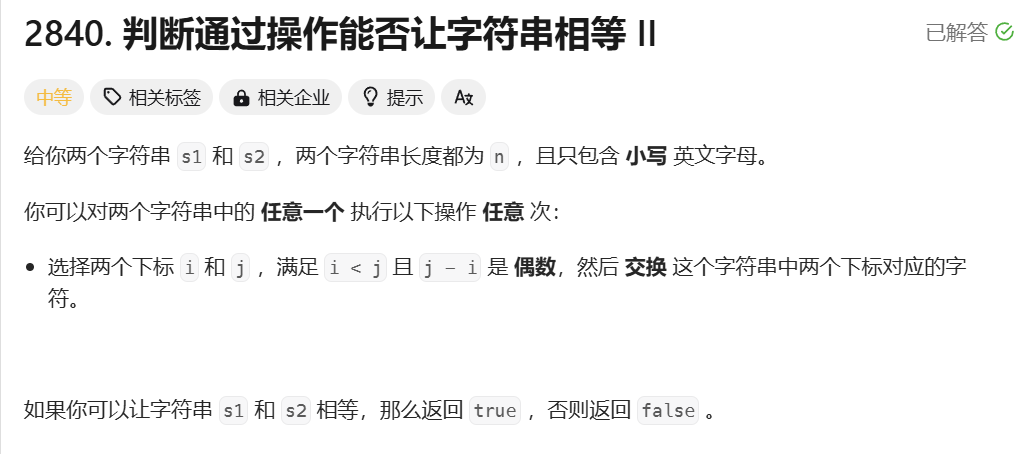
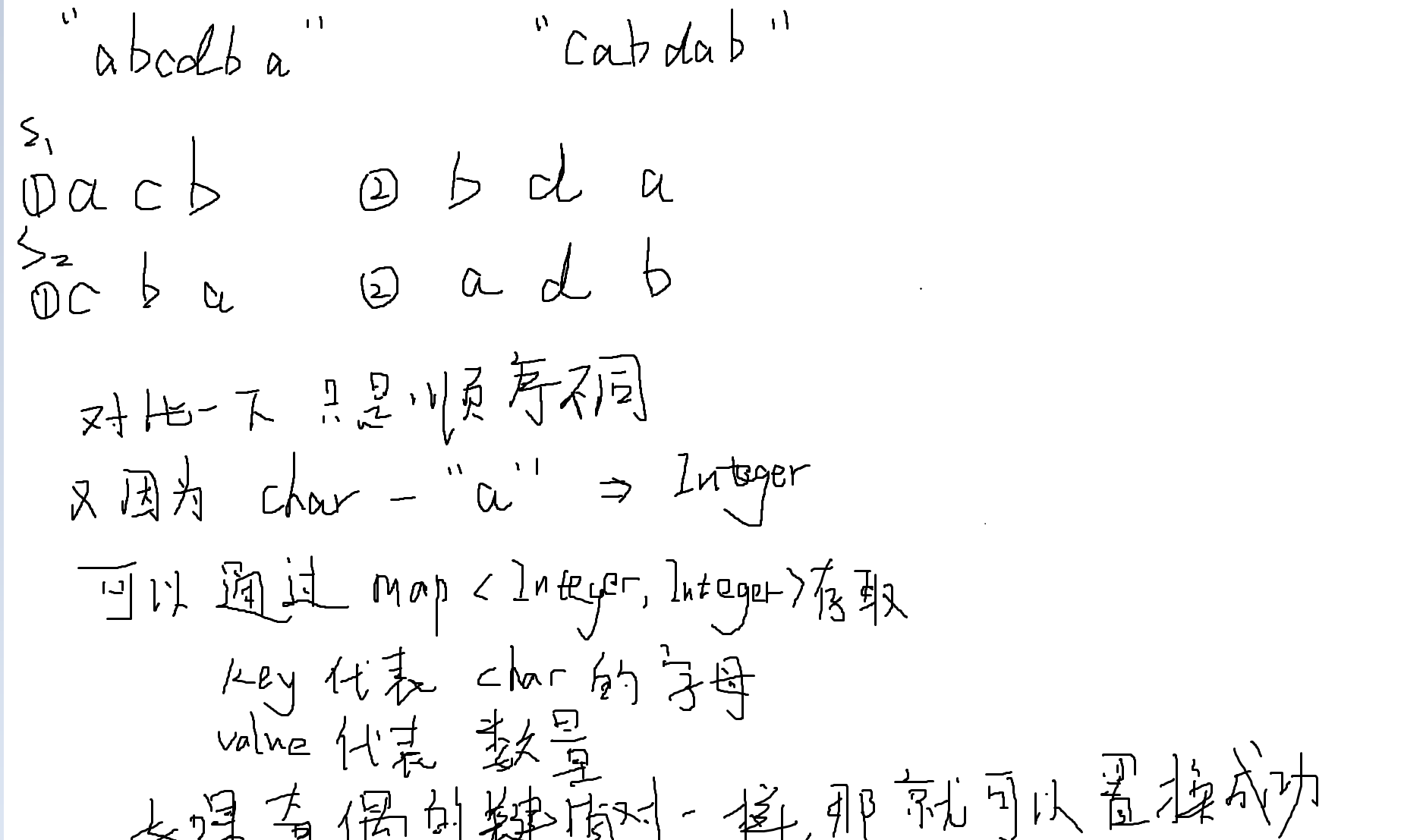
如图所示为一种解决方法,也可以使用二维数组解决
class Solution {
public boolean checkStrings(String s1, String s2) {
int[][] cnt1 = new int[2][26];
int[][] cnt2 = new int[2][26];
for (int i = 0; i < s1.length(); i++) {
cnt1[i % 2][s1.charAt(i) - 'a']++;
cnt2[i % 2][s2.charAt(i) - 'a']++;
}
return Arrays.deepEquals(cnt1, cnt2);
}
}