一、Qt跨平台开发核心优势
1.统一代码基
通过Qt的抽象层(Qt Platform Abstraction, QPA),同一套代码可编译部署到Windows、macOS、Linux、嵌入式系统(如ARM设备)甚至移动端(通过Qt for Android/iOS)。
2.模块化架构
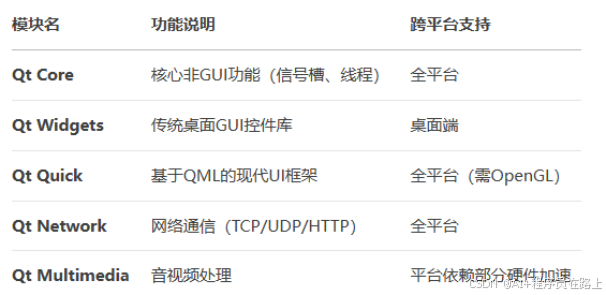
二、跨平台开发关键技术点
1. 平台差异处理
- 文件系统路径
使用Qt API代替原生路径操作:
cpp
// 获取文档目录
QString docPath = QStandardPaths::writableLocation(QStandardPaths::DocumentsLocation);
// 跨平台路径拼接
QString filePath = QDir(docPath).filePath("data.txt");
- 条件编译
处理平台特有代码:
cpp
#ifdef Q_OS_WINDOWS
// Windows专用代码(如注册表操作)
QSettings reg("HKEY_CURRENT_USER\\Software", QSettings::NativeFormat);
#elif defined(Q_OS_MACOS)
// macOS专用代码(如调用Objective-C)
macosShowNotification();
#endif
2.多分辨率适配
- DPI缩放
在`main.cpp`中启用高DPI支持:
cpp
QApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QApplication::setAttribute(Qt::AA_UseHighDpiPixmaps);
- 响应式布局
使用`QGridLayout` + 尺寸策略:
cpp
QWidget *widget = new QWidget;
QHBoxLayout *layout = new QHBoxLayout(widget);
layout->addWidget(button1);
layout->addWidget(button2);
layout->setContentsMargins(10, 5, 10, 5); // 统一边距
3. 多语言支持
- TS文件翻译
步骤:
- 在`.pro`中添加:
qmake
TRANSLATIONS = app_zh_CN.ts app_en_US.ts
- 使用`lupdate`提取字符串:
bash
lupdate project.pro
-
用Qt Linguist编辑翻译文件
-
发布时加载QM文件:
cpp
QTranslator translator;
translator.load(":/i18n/app_zh_CN.qm");
app.installTranslator(&translator);
三、跨平台构建与部署
1. 编译配置
- Windows
使用MSVC或MinGW编译器,`.pro`配置示例:
qmake
win32 {
LIBS += -ladvapi32 # Windows API库
RC_FILE = app.rc # 版本信息文件
}
- macOS
生成App Bundle:
bash
qmake -spec macx-clang
make
macdeployqt MyApp.app -dmg
- Linux
生成AppImage:
bash
linuxdeployqt MyApp -appimage
2. 依赖管理
- 动态链接库
使用`windeployqt`(Windows)或`macdeployqt`(macOS)自动复制依赖:
bash
windeployqt --compiler-runtime MyApp.exe
- 静态编译
在`.pro`中配置静态构建:
qmake
CONFIG += static
四、平台适配最佳实践
1. UI风格统一化
- 强制使用Fusion风格
cpp
QApplication::setStyle(QStyleFactory::create("Fusion"));
- 自定义平台样式**
css
/* stylesheet.qss */
QPushButton {
background-color: #2C3E50;
color: white;
border-radius: 4px;
padding: 5px;
}
/* macOS特定调整 */
#ifdef Q_OS_MACOS
QMenuBar {
background-color: #ECF0F1;
}
#endif
2. 系统功能调用
- 使用Qt封装接口*
cpp
// 打开URL
QDesktopServices::openUrl(QUrl("https://qt.io"));
// 系统通知(Qt 5.14+)
QSystemTrayIcon::showMessage("提示", "任务已完成");
- 平台原生API集成
cpp
// 调用Windows API
#ifdef Q_OS_WIN
#include <Windows.h>
void setWallpaper(const QString &path) {
SystemParametersInfo(SPI_SETDESKWALLPAPER, 0, (PVOID)path.utf16(), SPIF_UPDATEINIFILE);
}
#endif
五、调试与优化
1.多平台调试
- 远程调试Linux
配置Qt Creator的远程设备:
<img src="https://example.com/qt-remote-debug.png" width="500">
- Android真机调试
启用USB调试模式,使用`adb logcat`查看日志
2. 性能分析工具
- QML Profiler
分析QML界面渲染性能
- **Heob**(Windows内存检测)
检测内存泄漏
六、常见问题解决方案
1. 中文乱码
-
源码文件保存为UTF-8 BOM格式
-
在`main.cpp`中设置编码:
cpp
QTextCodec::setCodecForLocale(QTextCodec::codecForName("UTF-8"));
2. 跨平台字体差异
- 打包时嵌入字体:
cpp
QFontDatabase::addApplicationFont(":/fonts/SourceHanSansCN-Regular.otf");
3.安装包签名
-
Windows:使用`signtool`进行代码签名
-
macOS:申请开发者证书并签名:
bash
codesign --deep --force --verify --verbose --sign "Developer ID" MyApp.app
通过遵循上述实践,Qt跨平台应用可达到接近原生体验。建议结合持续集成(如GitHub Actions)实现自动化多平台构建,并通过Qt Virtual Keyboard等模块增强移动端适配能力。