main.qml
cpp
import QtQuick
import QtQuick3D
import QtQuick3D.AssetUtils
import QtQuick3D.Helpers
import QtQuick3D.Particles3D
import QtQuick3D.Xr
import QtQuick.Controls
// qt 6.8
Window {
visible: true
width: 640
height: 480
title: qsTr("3D scene")
// predefine camera
Node {
id: standAloneScene
Node {
PerspectiveCamera {
id: cameraPerspectiveOne
z: 600
}
PropertyAnimation on eulerRotation.x {
loops: Animation.Infinite
duration: 5000
to: -360
from: 0
}
}
PerspectiveCamera {
id: cameraPerspectiveTwo
position: Qt.vector3d(0, 200, 300)
eulerRotation.x: -30
z: 600
}
OrthographicCamera {
id: cameraOrthographicLeft
x: -600
eulerRotation.y: -90
}
}
Rectangle {
id: topLeft
anchors.top: parent.top
anchors.left: parent.left
width: parent.width * 0.5
height: parent.height * 0.5
color: "#848895"
border.color: "black"
View3D {
anchors.fill: parent
camera: activeCamera // 使用相机
PerspectiveCamera { // 定义透视投影3D->2D相机
id: activeCamera
z: 400
}
DirectionalLight { // 光源
color: "white"
eulerRotation.x: -30 // 欧拉角度
eulerRotation.y: -70
}
Model {
x: -100
source: "#Cube" // 内置Mesh
materials: PrincipledMaterial { // 材质着色
baseColor: "red"
}
}
Model {
x: 100
source: "#Sphere" // 内置Mesh
materials: PrincipledMaterial { // 材质着色
baseColor: "green"
}
}
environment: SceneEnvironment { // 背景色
clearColor: "skyblue"
backgroundMode: SceneEnvironment.Color
}
WasdController { // wasd键控制相机
controlledObject: activeCamera
}
}
Label {
text: "Front"
anchors.top: parent.top
anchors.left: parent.left
anchors.margins: 10
color: "#222840"
font.pointSize: 14
}
}
Rectangle {
id: topRight
anchors.top: parent.top
anchors.right: parent.right
width: parent.width * 0.5
height: parent.height * 0.5
color: "#848895"
border.color: "black"
View3D {
id: topRightView
anchors.fill: parent
importScene: standAloneScene
camera: cameraOrthographicLeft
DirectionalLight {
color: "white"
}
PointLight {
y: 200
color: "#d9c62b"
brightness: 5
castsShadow: true // 阴影投射
shadowFactor: 75
Model { // 寻找光源位置,白色小立方体
source: "#Cube"
scale: Qt.vector3d(0.01, 0.01, 0.01)
materials: PrincipledMaterial {
lighting: PrincipledMaterial.NoLighting
}
}
}
Model {
position: Qt.vector3d(0, -200, 0)
source: "#Cylinder"
scale: Qt.vector3d(2, 0.2, 1)
materials: [ PrincipledMaterial { // 多材质着色
baseColor: "red"
}
]
}
Model {
position: Qt.vector3d(0, 150, 0)
source: "#Sphere"
materials: [ PrincipledMaterial {
baseColor: "blue"
}
]
SequentialAnimation on y {
loops: Animation.Infinite
NumberAnimation {
duration: 3000
to: -150
from: 150
easing.type: Easing.InQuad
}
NumberAnimation {
duration: 3000
to: 150
from: -150
easing.type: Easing.OutQuad
}
}
}
WaterBottle {
id: modelInstance
scale: Qt.vector3d(10, 10, 10)
NumberAnimation on eulerRotation.y {
from: 0
to: 360
duration: 3000
loops: Animation.Infinite
}
}
environment: SceneEnvironment { // 天空盒背景
backgroundMode: SceneEnvironment.SkyBox
lightProbe: Texture {
textureData: ProceduralSkyTextureData {
}
}
}
}
Label {
text: "Front"
anchors.top: parent.top
anchors.right: parent.right
anchors.margins: 10
color: "#222840"
font.pointSize: 14
}
RoundButton {
text: "Camera 1"
anchors.centerIn: parent
anchors.bottom: parent.top
anchors.margins: 10
highlighted: topRightView.camera == cameraPerspectiveOne
implicitWidth: 100
onClicked: {
topRightView.camera = cameraPerspectiveOne
}
}
}
Button {
anchors.right: parent.right
text: "debug"
onClicked: debugView.visible = !debugView.visible
DebugView { // render statics data
id: debugView
source: topRightView
anchors.top: parent.bottom
anchors.right: parent.right
}
}
}
效果展示
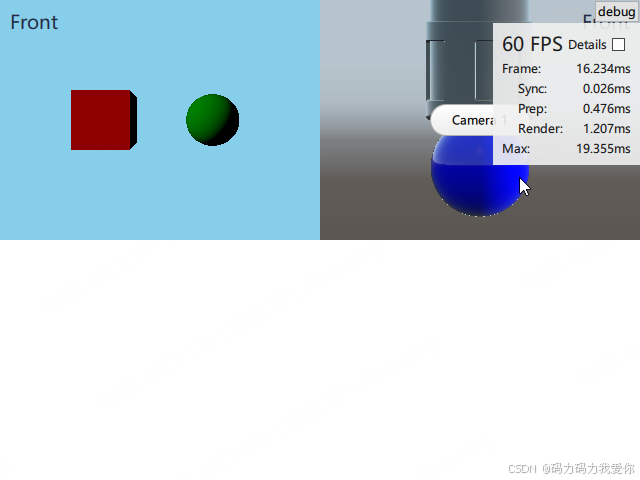