C# 操作符
一、操作符概览
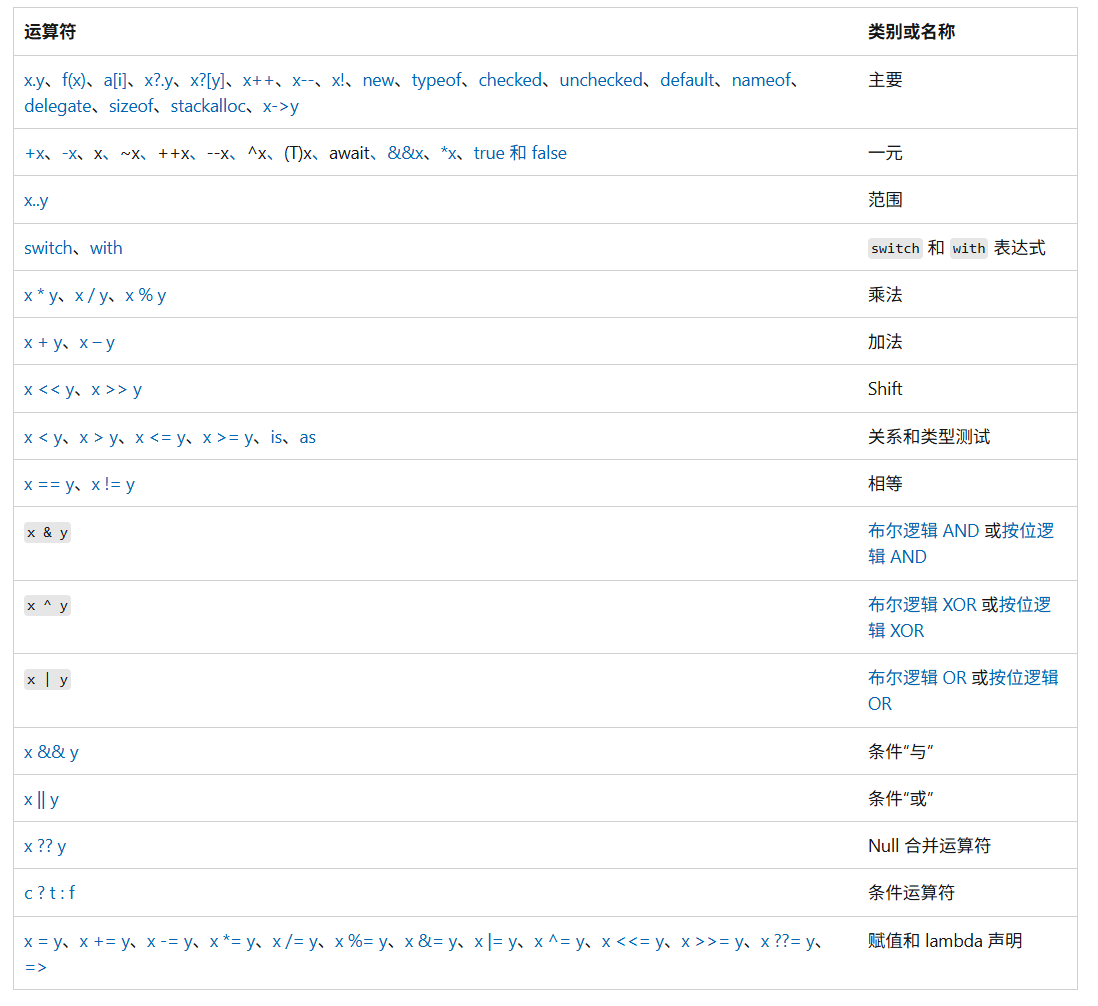
-
操作符(运算符)的本质是函数的简记法
-
操作符不能脱离与它关联的数据类型
csharpint x = 5; int y = 4; int z = x / y; Console.WriteLine(z);//输出1 double a = 5.0; double b = 4.0; double c = a / b; Console.WriteLine(c);//输出1.25
二、优先级与运算顺序
-
按表格顺序从上到下优先级依次降低
-
同优先级操作符从左到右
-
赋值操作符从右到左
csharpint x = 100; int y = 200; int z = 300; x += y += z; Console.WriteLine(x);//600 Console.WriteLine(y);//500 Console.WriteLine(z);//300
三、各类操作符的实例
x.y
成员访问操作符
-
访问外层名称空间的子集名称空间
-
访问名称空间的类型
-
访问类型的静态成员
csharpSystem.IO.File.Create("D:\\HelloWorld");
System.IO
:外层名称空间的子集名称空间IO.File
:名称空间的类型File.Create
:类型的静态成员
-
访问对象的成员
csharpForm myForm = new Form(); myForm.Text = "Hello, World!"; myForm.ShowDialog();
-
f(x)
方法调用操作符 -
a[x]
元素访问操作符csharp//访问数组 int[] myArray1 = new int[10]; int[] myArray2 = new int[] { 1,2,3,4,5}; int[] myArray3 = new int[5] { 1, 2, 3, 4, 5 }; Console.WriteLine(myArray2[0]); //访问字典 class Program { static void Main(string[] args) { Dictionary<string, Student> stuDic = new Dictionary<string, Student>(); for (int i = 0; i < 100; i++) { Student student = new Student(); student.Name = "s_" + i.ToString(); student.Score = i; stuDic.Add(student.Name, student); } Student number6 = stuDic["s_6"]; Console.WriteLine(number6.Score);//6 } } class Student { public string Name; public int Score; }
-
x++
后置自增 ,x--
后置自减 。遇到赋值,先赋值,后自增/自减csharpint x = 100; int y = x++; Console.WriteLine(x);//101 Console.WriteLine(y);//100
-
new
:在内存中创造一个类的实例,(1)立即调用这个实例的构造器,(2)调用实例的初始化器csharpForm myForm1 = new Form(); Form myForm2 = new Form() { Text = "Hello" };
csharp//匿名类型 var person = new { Name = "Mr.Ok", Age = 34 }; Console.WriteLine(person.Name);//Mr.Ok Console.WriteLine(person.Age);//34
-
typeof
操作符:查看一个类型的内部结构csharpType t = typeof(int); Console.WriteLine(t.Namespace);//System Console.WriteLine(t.FullName);//System.Int32 Console.WriteLine(t.Name);//Int32 int c = t.GetMethods().Length; foreach (var mi in t.GetMethods()) { Console.WriteLine(mi.Name);//CompareTo、 Equals、GetHashCode ...... } Console.WriteLine(c);//53
-
default
:获取一个类型的默认值csharp//结构体类型 int x = default(int); Console.WriteLine(x);//0
csharp//引用类型 Form myForm = default(Form); Console.WriteLine(myForm == null);//true
csharpclass Program { static void Main(string[] args) { //枚举类型 Level1 level1 = default(Level1); Console.WriteLine(level1); //Low Level2 level2 = default(Level2); Console.WriteLine(level2); //Medium Level3 level3 = default(Level3); Console.WriteLine(level3); //逻辑出错,输出0 } } enum Level1 { Low, Medium, High } enum Level2 { Low = 1, Medium = 0, High = 2 } enum Level3 { Low = 2, Medium = 1, High = 3 }
-
checked
/unchecked
:检查一个值是否有溢出csharp//第一种用法 uint x = uint.MaxValue; uint y = x+1; Console.WriteLine(x);//4294967295 Console.WriteLine(y);//0 try { uint z = checked(x + 1); Console.WriteLine(z); } catch (OverflowException ex) { Console.WriteLine("There's overflow");//There's overflow }
csharp//第二种用法 uint x = uint.MaxValue; uint y = x + 1; Console.WriteLine(x);//4294967295 Console.WriteLine(y);//0 checked { try { uint z = x + 1; Console.WriteLine(z); } catch (OverflowException ex) { Console.WriteLine("There's overflow");//There's overflow } }
-
sizeof
: 获取基本类型在内存中占的字节数csharpint x = sizeof(int); Console.WriteLine(x);//4
csharpclass Program { static void Main(string[] args) { unsafe { int y = sizeof(Student); Console.WriteLine(y); } } } class Student { int ID; long Score; }
-
->
:箭头操作符。会直接操作内存,因此需要放在不安全的上下文使用。只能操作结构体类型。csharpclass Program { static void Main(string[] args) { unsafe { Student stu; stu.ID = 1; stu.Score = 60; Student* pStu = &stu; pStu->Score = 90; Console.WriteLine(stu.Score);//90 } } } struct Student { public int ID; public long Score; }
-
*x
csharpclass Program { static void Main(string[] args) { unsafe { Student stu; stu.ID = 1; stu.Score = 60; Student* pStu = &stu; pStu->Score = 90; (*pStu).Score = 1000; Console.WriteLine(stu.Score);//100 } } } struct Student { public int ID; public long Score; }
-
-x
:相反数操作符,在二进制的基础上,按位取反,再加1csharpint x = int.MinValue; int y = -x; Console.WriteLine(x)//-2147483648 Console.WriteLine(y);//-2147483648 string xStr = Convert.ToString(x,2).PadLeft(32,'0');//10000000000000000000000000000000 string yStr = Convert.ToString(y, 2).PadLeft(32, '0');//10000000000000000000000000000000 Console.WriteLine(xStr); Console.WriteLine(yStr);
-
(T)x
:强制类型转换操作符-
隐式类型转换
-
不丢失精度的转换
csharp//短整型转换成长整型 int x = int.MaxValue; long y = x; Console.WriteLine(y);
源 目标 sbyte short、int、long、float、double 或 decimal byte short、ushort、int、uint、long、ulong、float、double 或 decimal short int、long、float、double 或 decimal ushort int、uint、long、ulong、float、double 或 decimal int long、float、double 或 decimal uint long、ulong、float、double 或 decimal long float、double 或 decimal char ushort、int、uint、long、ulong、float、double 或 decimal float double ulong float、double 或 decimal -
子类向父类的转换
-
装箱
-
-
显式类型转换
-
有可能丢失精度(甚至发生错误)的转换,即cast
csharpint x = 100; short y = (short)x; Console.WriteLine(y);
源 目标 sbyte byte、ushort、uint、ulong 或 char byte Sbyte 或 char short sbyte、byte、ushort、uint、ulong 或 char ushort sbyte、byte、short 或 char int sbyte、byte、short、ushort、uint、ulong 或 char uint sbyte、byte、short、ushort、int 或 char long sbyte、byte、short、ushort、int、uint、ulong 或 char ulong sbyte、byte、short、ushort、int、uint、long 或 char char sbyte、byte 或 short float sbyte、byte、short、ushort、int、uint、long、ulong、char 或 decimal double sbyte、byte、short、ushort、int、uint、long、ulong、char、float 或 decimal decimal sbyte、byte、short、ushort、int、uint、long、ulong、char、float 或 double -
拆箱
-
使用Convert类
-
ToString方法与各数据类型的Parse/TryParse方法
-
-
自定义类型转换操作符
csharpclass Program { static void Main(string[] args) { Stone stone = new Stone(); stone.Age = 5000; Monkey wukong = (Monkey)stone; Console.WriteLine(wukong.Age);//10 } } class Stone { public int Age; public static explicit operator Monkey(Stone stone) { Monkey m = new Monkey(); m.Age = stone.Age / 500; return m; } } class Monkey { public int Age; }
-
-
/
:除法操作符
csharp
int x1 = 5;
int y1 = 4;
Console.WriteLine(x1 / y1);//1
double x2 = 5.0;
double y2 = 4.0;
Console.WriteLine(x2 / y2);//1.25
csharp
//被除数为0
int x1 = 5;
int y1 = 0;
Console.WriteLine(x1 / y1);//报错
double x2 = 5.0;
double y2 = 0;
Console.WriteLine(x2 / y2);//∞
is
:检验一个对象是不是某个类型的对象
csharp
class Program
{
static void Main(string[] args)
{
Teacher t1 = new Teacher();
Teacher t2 = null;
var result1 = t1 is Teacher;
var result2 = t1 is Hunam;
var result3 = t2 is Teacher;
Console.WriteLine(result1);//True
Console.WriteLine(result2);//True
Console.WriteLine(result3);//False
}
}
class Animal {
public void Eat() {
Console.WriteLine("Eating...");
}
}
class Hunam:Animal
{
public void Think()
{
Console.WriteLine("Thinking...");
}
}
class Teacher : Hunam
{
public void Teach()
{
Console.WriteLine("Teaching...");
}
}
as
csharp
object obj = new Teacher();
Teacher t = obj as Teacher;
if (t != null)
{
t.Teach();//Teaching...
}
&&
和||
,注意短路效应
csharp
int x = 3;
int y = 4;
int a = 3;
if (x > y && a++ > 3)
{
Console.WriteLine("Hello");
}
Console.WriteLine(a);//3
csharp
int x = 5;
int y = 4;
int a = 3;
if (x > y && a++ > 3)
{
Console.WriteLine("Hello");
}
Console.WriteLine(a);//4
csharp
int x = 5;
int y = 4;
int a = 3;
if (x > y || a++ > 3)
{
Console.WriteLine("Hello");//Hello
}
Console.WriteLine(a);//3
csharp
int x = 3;
int y = 4;
int a = 3;
if (x > y || ++a > 3)
{
Console.WriteLine("Hello");//Hello
}
Console.WriteLine(a);//4