文章目录
一、环境准备
首先,确保你的Qt安装包含了QtWebEngine模块。我的Qt是5.12.9并且使用MSVC来编译项目。在项目文件中需要添加以下配置,其中在Qt中配置MSVC,建议去看看这位大佬的博客:Qt 添加MSVC2017编译器(2022年保姆级教程,不安装完整VS)_qt msvc2017-CSDN博客
确保:
QT += core gui webenginewidgets
二、代码实现
mainwindow.cpp,主要实现的简单网页浏览器,其通过QWebEngineView组件实现了网页内嵌功能。如下为测试demo文件代码:
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QWebEngineView>
#include <QWebEnginePage>
#include <QWebEngineProfile>
#include <QStyle>
#include <QApplication>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 设置窗口标题和大小
setWindowTitle("Web Browser");
resize(1024, 768);
// 创建工具栏
toolBar = new QToolBar(this);
addToolBar(toolBar);
// 创建地址栏
urlLineEdit = new QLineEdit(this);
urlLineEdit->setPlaceholderText("Enter URL (e.g., https://www.google.com)");
urlLineEdit->setStyleSheet("QLineEdit { padding: 5px; border-radius: 3px; }");
toolBar->addWidget(urlLineEdit);
// 创建前进按钮
goButton = new QPushButton("Go", this);
goButton->setStyleSheet("QPushButton { padding: 5px 15px; background-color: #4CAF50; color: white; border: none; border-radius: 3px; }"
"QPushButton:hover { background-color: #45a049; }");
toolBar->addWidget(goButton);
// 创建网页视图
webView = new QWebEngineView(this);
setCentralWidget(webView);
// 创建进度条
progressBar = new QProgressBar(this);
progressBar->setMaximumHeight(2);
progressBar->setTextVisible(false);
progressBar->setStyleSheet("QProgressBar { border: none; background-color: #f0f0f0; }"
"QProgressBar::chunk { background-color: #4CAF50; }");
statusBar()->addPermanentWidget(progressBar);
// 连接信号和槽
connect(goButton, &QPushButton::clicked, this, &MainWindow::loadPage);
connect(urlLineEdit, &QLineEdit::returnPressed, this, &MainWindow::loadPage);
connect(webView, &QWebEngineView::urlChanged, this, &MainWindow::updateUrl);
connect(webView, &QWebEngineView::loadProgress, this, &MainWindow::updateProgress);
connect(webView, &QWebEngineView::titleChanged, this, &MainWindow::updateTitle);
// 设置初始页面
webView->setUrl(QUrl("https://www.google.com"));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::loadPage()
{
QString url = urlLineEdit->text();
if (!url.startsWith("http://") && !url.startsWith("https://")) {
url = "https://" + url;
}
webView->setUrl(QUrl(url));
}
void MainWindow::updateUrl(const QUrl &url)
{
urlLineEdit->setText(url.toString());
}
void MainWindow::updateProgress(int progress)
{
progressBar->setValue(progress);
if (progress == 100) {
progressBar->hide();
} else {
progressBar->show();
}
}
void MainWindow::updateTitle(const QString &title)
{
setWindowTitle(title + " - Web Browser");
}
三、测试
1.打开博客网页:
2.打开B站网页:
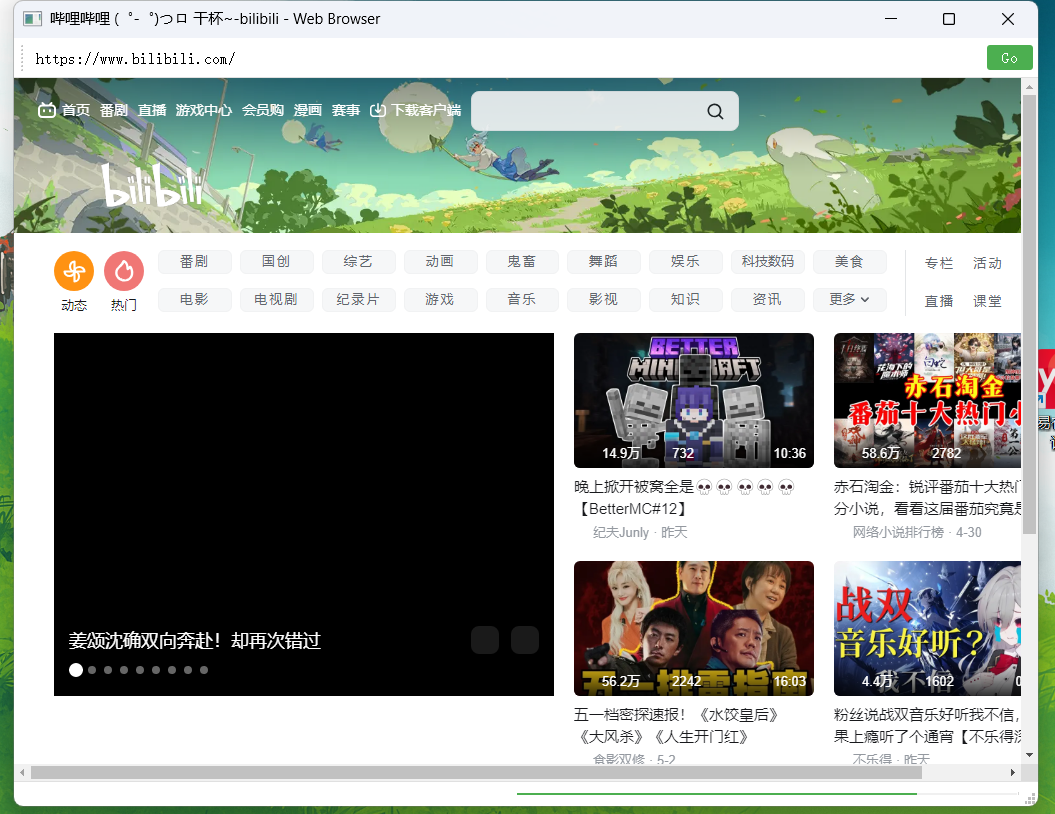
合理!!!!!