string
- [1. 前言](#1. 前言)
- [2. Member functions](#2. Member functions)
-
- [2.1 constructor](#2.1 constructor)
- [2.2 operator=](#2.2 operator=)
- [3. Iterators](#3. Iterators)
-
- [3.1 begin end cbegin cend](#3.1 begin end cbegin cend)
- [3.2 rbegin rend](#3.2 rbegin rend)
- [4. Capacity](#4. Capacity)
-
- [4.1 size length](#4.1 size length)
- [4.2 capacity](#4.2 capacity)
- [4.3 reserve](#4.3 reserve)
- [4.4 resize](#4.4 resize)
- [5. Modifiers](#5. Modifiers)
-
- [5.1 insert](#5.1 insert)
- [5.2 replace](#5.2 replace)
- [5.3 append](#5.3 append)
- [5.4 assign](#5.4 assign)
1. 前言
常见的string用法。
2. Member functions
constructor/operator=
cpp
void test1()
{
string str1;
string str2 = "hello ATS";
string str3 = str2;
//string str4(str2, 6, 6);
string str4(str2, 6);
cout << str1 << endl;
cout << str2 << endl;
cout << str3 << endl;
cout << str4 << endl;
}
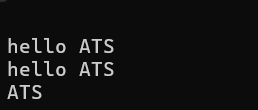
2.1 constructor

常用的构造包含这三种
2.2 operator=

可以用string也可以用字符
3. Iterators
begin/end/rbegin/rend/cbegin/cend
迭代器的类型是string::iterator ,也可以用auto简化。
cpp
void test2()
{
string str1 = "hello ATS";
/*string::iterator it1 = str1.begin();
string::iterator it2 = str1.end();
for (it1 = str1.begin(); it1 < it2; it1++)
{
cout << *it1 << " ";
}
cout << endl;*/
for (auto i = str1.begin(); i < str1.end(); i++)
{
cout << *i << " ";
}
cout << endl;
string str2 = "STA olleh";
for (auto i = str2.rbegin(); i < str2.rend(); i++)
{
cout << *i << " ";
}
cout << endl;
const string str3 = "hello ATS"; // 如果是const修饰的内容
string::const_iterator it1 = str3.cbegin();
string::const_iterator it2 = str3.cend();
for (it1 = str3.begin(); it1 < str3.end(); it1++)
{
cout << *it1 << " ";
//*it1 = 'x'; // 不可修改
}
cout << endl;
string str4 = "hello ATS";
for (auto i = str4.begin(); i < str4.end(); i++)
{
// auto i = str4.cbegin(); i < str4.cend(); i++ // 报错
*i = 'x';
}
for (auto i = str4.rbegin(); i < str4.rend(); i++)
{
cout << *i << " ";
}
cout << endl;
}

3.1 begin end cbegin cend
begin指向第一个元素,endl指向最后一个元素的下一个元素。
对于begin和end,可以对迭代器指向的内容进行修改。对于cbegin和cend,无论对象是否有const修饰,都不能修改。
3.2 rbegin rend
rbegin指向最后一个元素的,rendl指向首个元素的前一个元素。和begin/end相反。
4. Capacity
size/length/resize/reserve/empty
cpp
void test3()
{
// size可以拿到字符串的长度 length也可以 但推荐用size 可以和其他容器保持一致
// 不会计算\0
string str1 = "hello ATS";
cout << "str1:" << str1.size() << endl;
cout << "str1:" << str1.length() << endl;
cout << endl;
string str2 = "hello ATS";
cout << "str2:" << str2.capacity() << endl;
cout << endl;
string str3 = "hello ATSxxxx";
cout << "str3:" << str3.capacity() << endl;
cout << endl;
string str4 = "hello ATSxxxxxxxxxxxxxxxxxxxxxxx";
cout << "str4:" << str4.capacity() << endl;
cout << endl;
// 实际上的capacity比显示的多一个 因为没有计算\0
// vs下扩容capacity 第一次不能严格认为是扩容,是编译器把数组里的内容扩容到堆上
// 之后的扩容每次接近扩容1.5倍
// reserve的作用是改变string的capacity 不会改变其size大小
string str5 = "hello ATSxxxxxxxxxxxxxxxxxxxxxxx";
str5.reserve(200);
cout << "str5:" << str5.capacity() << endl;
// 在vs里,如果缩容后的capacity小于现有capacity,编译器不会进行缩容,这点上不同编译器有不同处理结果
str5.reserve(20);
cout << "str5:" << str5.capacity() << endl;
cout << endl;
// reserve的常见用法是 已知需要的空间大小 提前开辟出来
// resize
string str6 = "hello ATS";
cout << "str6:" << str6 << endl;
cout << "str6.size:" << str6.size() << endl;
cout << "str6.capacity:" << str6.capacity() << endl;
str6.resize(5);
cout << "str6:" << str6 << endl;
cout << "str6.size:" << str6.size() << endl;
cout << "str6.capacity:" << str6.capacity() << endl;
str6.resize(20);
cout << "str6:" << str6 << endl;
cout << "str6.size:" << str6.size() << endl;
cout << "str6.capacity:" << str6.capacity() << endl;
cout << endl;
// resize的改变时,capacity也可能改变
string str7 = "hello ATS";
cout << "str7:" << str7 << endl;
cout << "str7.size:" << str7.size() << endl;
cout << "str7.capacity:" << str7.capacity() << endl;
str7.resize(20, 'x');
cout << "str7:" << str7 << endl;
cout << "str7.size:" << str7.size() << endl;
cout << "str7.capacity:" << str7.capacity() << endl;
cout << endl;
// empty返回bool值
string str8 = "hello ATS";
if (str8.empty())
cout << "str8 is empty" << endl;
else
cout << "str8 is not empty" << endl;
}
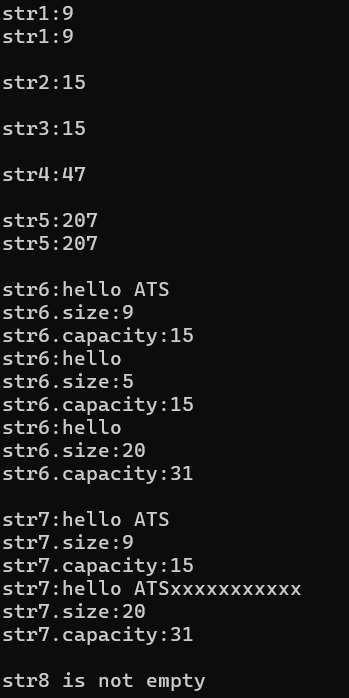
4.1 size length
作用类似,计算字符串的长度,结果不包括终止符。
推荐用size,方便和其他容器保持一致。
4.2 capacity
capacity的计算结果比实际少一个,忽略了终止符。
vs下扩容capacity 第一次不能严格认为是扩容,是编译器把数组里的内容扩容到堆上,之后的扩容每次接近扩容1.5倍。
4.3 reserve

- reserve的作用是改变string的capacity 不会改变其size大小
- 在vs里,如果缩容后的capacity小于现有capacity,编译器不会进行缩容,这点上不同编译器有不同处理结果
- reserve的常见用法是 已知需要的空间大小 提前开辟出来
4.4 resize

- 改变size大小
- resize的改变时,capacity也可能改变
5. Modifiers
operator+=/push_back/insert/erase/replace/swap/append/assign/pop_back
cpp
void test4()
{
// += 没什么好说的,就是重载操作符使得可以直接在string上添加内容
string str1 = "hello ATS";
str1 += " hello world";
cout << "str1:" << str1 << endl;
cout << endl;
// push_back 向字符串后面插入
string str2 = "hello ATS";
str2.push_back('x');
str2.push_back('x');
str2.push_back('x');
cout << "str2:" << str2 << endl;
cout << endl;
// insert
string str3 = "hello ATS";
str3.insert(0, str2);
cout << "str3:" << str3 << endl;
str3.insert(0, str2, 6);
cout << "str3:" << str3 << endl;
cout << endl;
string str4 = "hello ATS";
str4.insert(str4.size(), 'x', 5);
cout << "str4:" << str4 << endl;
cout << endl;
// 效率很低 时间复杂度是O(N) 不建议用
// erase // 删除
string str5 = "hello ATS";
str5.erase(5);
cout << "str5:" << str5 << endl;
cout << endl;
// 缺点和insert一样
// replace 替换掉string中的某部分
string str6 = "hello ATS";
str6.replace(5, 1, "xxx"); // 把空格改为xxx
cout << "str6:" << str6 << endl;
cout << endl;
// 缺点和insert一样
// swap 交换
string str7 = "hello ATS";
string str8 = "xxx";
str7.swap(str8);
cout << "str7:" << str7 << endl;
cout << "str8:" << str8 << endl;
cout << endl;
// append 在末尾扩容
string str9 = "hello ATS";
str9.append(str7);
cout << "str9:" << str9 << endl;
cout << endl;
// assign 替换
//string& assign(const string & str);
string str10 = "hello ATS";
string str11 = "xxx";
str10.assign(str11);
cout << "str10:" << str10 << endl;
cout << endl;
//pop_back 删除末尾
string str12 = "hello ATS";
str12.pop_back();
str12.pop_back();
str12.pop_back();
cout << "str12:" << str12 << endl;
cout << endl;
}
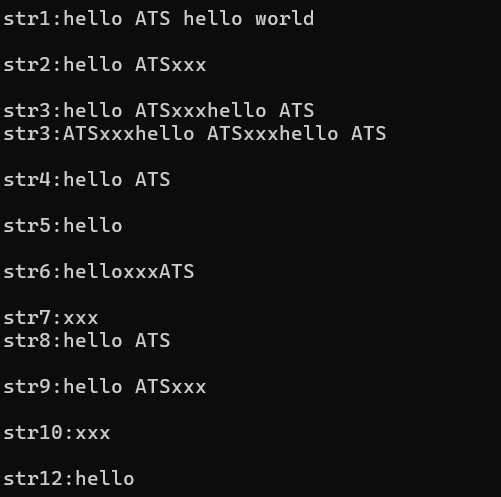
5.1 insert
cpp
string& insert(size_t pos, const string & str); // 在pos位置插入string
string& insert(size_t pos, const string & str, size_t subpos, size_t sublen); // 在pos位置插入string的subpos位置后sublen长度
string & insert(size_t pos, const char* s); // 插入字符
string& insert(size_t pos, const char* s, size_t n); // 插入n个字符
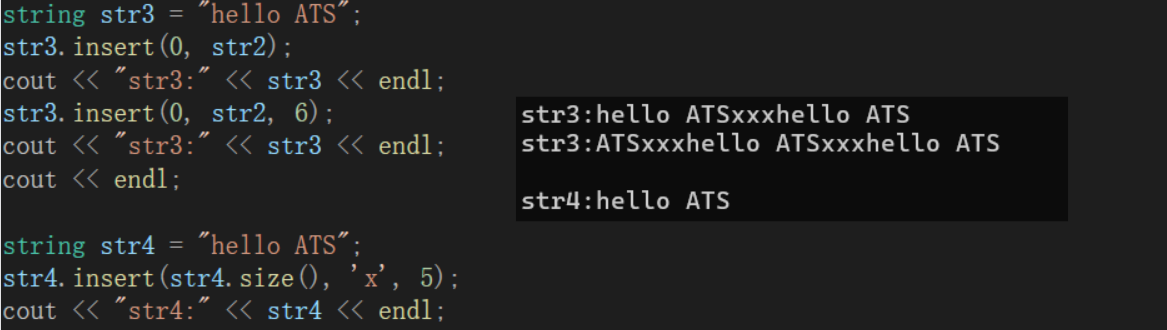
效率很低 时间复杂度是O(N) 不建议用
5.2 replace
替换掉string中的某部分
cpp
string& replace(size_t pos, size_t len, const string & str); // 可以用下标
string& replace(iterator i1, iterator i2, const string & str); // 也可以用迭代器
string& replace (size_t pos, size_t len, const string& str,size_t subpos, size_t sublen);
//也可以传字符
replace和erase的缺点与insert类似。
5.3 append
在末尾扩容
cpp
string& append(const string & str);
string& append (const string& str, size_t subpos, size_t sublen);
5.4 assign
替换
cpp
string& assign(const string & str);