1.概述:
BOM(Browser Object Model,浏览器对象模型)是 JavaScript 中的一个重要概念,它提供了一系列对象来访问和操作浏览器的功能和信息。
与 DOM(Document Object Model)主要关注文档结构不同,BOM 主要处理浏览器窗口以及相关的功能,如导航栏、地址栏、浏览器历史记录等。
可以把 BOM 看作是连接 JavaScript 和浏览器的桥梁,通过它可以控制浏览器的行为和获取浏览器相关的各种数据。
2.BOM的内置对象:
* Window 表示整个浏览器的窗口
* Navigator 表示浏览器的信息
* Location 浏览器的地址信息
* History 浏览器历史记录
* Screen 表示用户的屏幕信息
3.Window对象:获取窗口信息
1.浏览器添加弹窗:
plain
window.alert("警告弹窗");
//确认弹窗
let bool = window.confirm("确定吗?")
console.log(bool)
//提示弹窗
let str = window.prompt("系统提示","我是默认值")
console.log(str)
2.延时器:setTimeout
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
window.setTimeout(function () {
console.log("执行了延时器")
},1000) //1 秒后执行*/
</script>
</body>
</html>
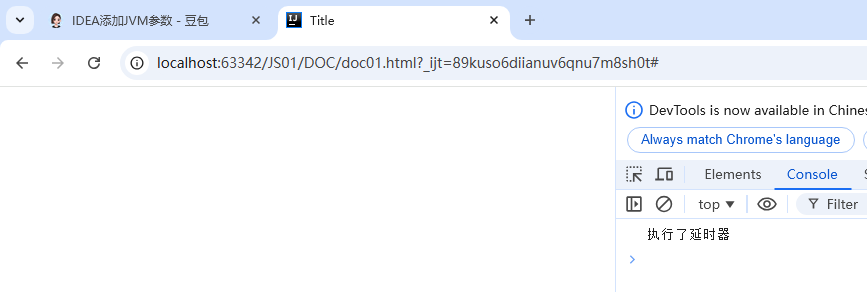
当页面加载完成后,延时1秒执行该函数;
3.定时器:setInterval
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
let timer = window.setInterval(function () {
console.log("执行了定时器")
},1000) // 间隔一秒后执行
</script>
</body>
</html>
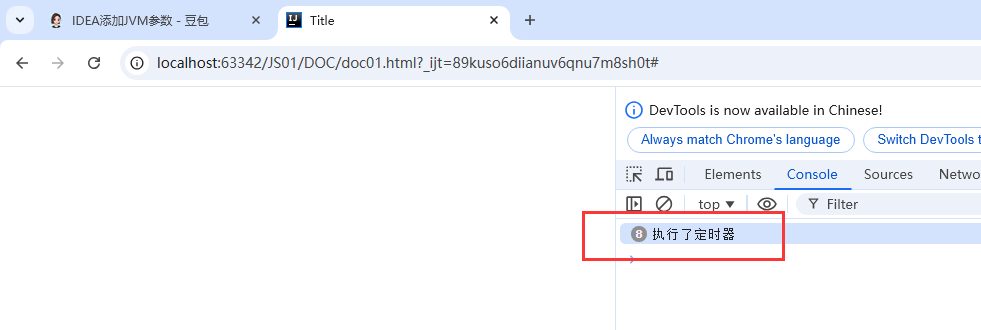
页面加载完成后,每隔一秒执行一次
4.取消延时器或定时器:
plain
//清除延时器
window.clearTimeout()
//清除定时器
window.clearInterval(timer);
4. Navigator对象:获取浏览器信息
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//获取浏览器信息
let ua = navigator.userAgent;
console.log(ua)
</script>
</body>
</html>
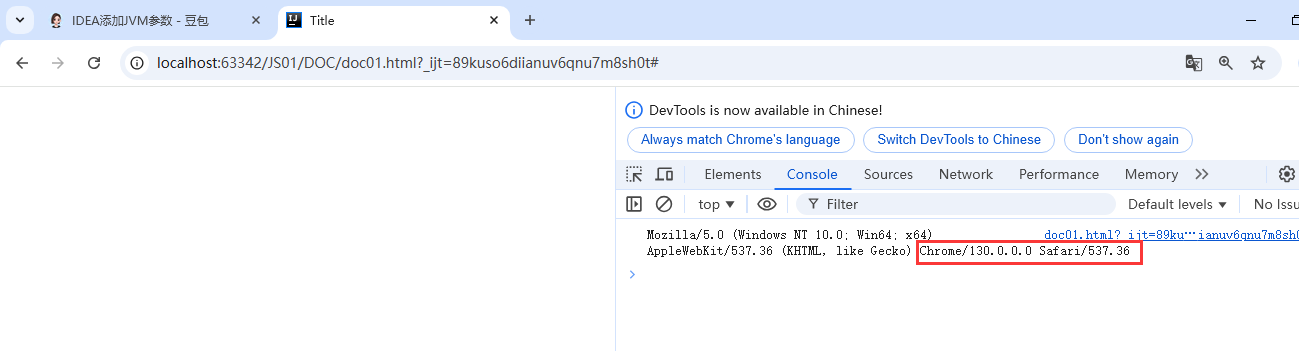
5. Location:获取地址栏信息:
1.获取地址栏信息:
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//获取地址栏信息
// console.log(location)
// console.log(location.href)//获取地址的全路径
// console.log(location.origin)//获取地址的来源
// console.log(location.hostname) //获取地址中的主机名
// console.log(location.host)//获取主机
// console.log(location.port)//获取端口部分
// console.log(location.pathname)//获取当前的地址部分
// console.log(location.search)// 获取地址的参数部分
</script>
</body>
</html>
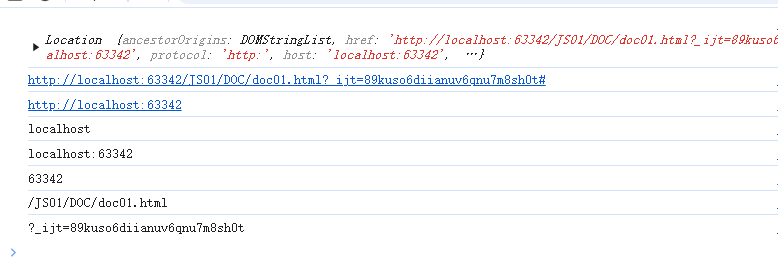
2.跳转到其他路径:
方式1:用'='直接赋值新路径
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//跳转 //修改的路径
location = "https://www.baidu.com"
</script>
</body>
</html>

方式2:href超链接:
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//跳转 //修改的路径
location.href = "https://www.baidu.com"
</script>
</body>
</html>

方式3:assign
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//跳转 //修改的路径
location.assign("https://www.baidu.com")
</script>
</body>
</html>

3.刷新页面:reload
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
//跳转 //修改的路径
location.reload(true)
</script>
</body>
</html>
4.替换页面:replace
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<script>
// 替换页面 不产生历史记录
location.replace("https://www.baidu.com")
</script>
</body>
</html>
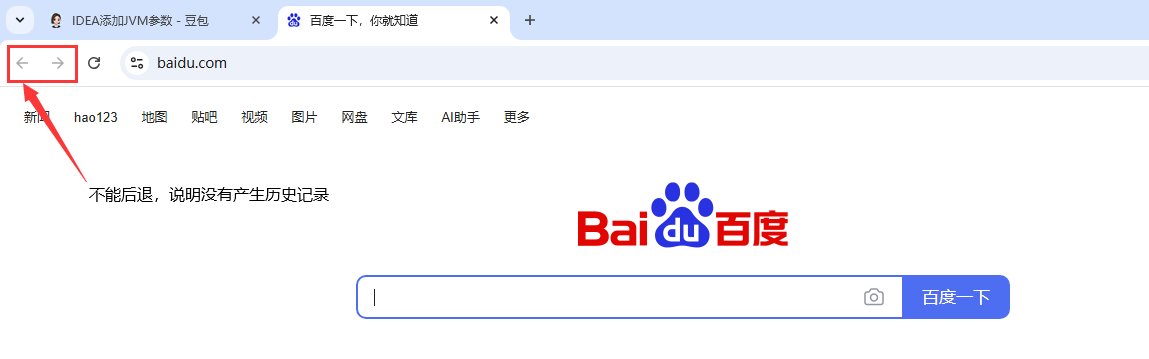
6.History对象:获取浏览器历史记录
案例:通过历史记录对象的前进或后退实现页面跳转
创建3个页面:
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>我是A页面</h1>
<a href="B.html">跳转到B页面</a>
</body>
</html>
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>我是B页面</h1>
<a href="C.html">跳转到C页面</a>
<button onclick="forward()">点我前进</button>
<script>
function forward() {
//历史记录前进1步
history.forward();
//前进多步
// history.go(3)
}
</script>
</body>
</html>
plain
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>我是C页面</h1>
<button onclick="back()">点我后退</button>
<script>
//获取历史记录长度
console.log(history.length)
function back() {
//后退1步
history.back();
//后退多步
// history.go(-3)
}
</script>
</body>
</html>
点击A页面超链接时,跳转到B页面
点击B页面超链接时,跳转到C页面
点击C页面的后退按钮,历史记录后退一步到B页面(可后退多步)
当点击B页面前进按钮时,历史记录前进一步到C页面(可前进多步);