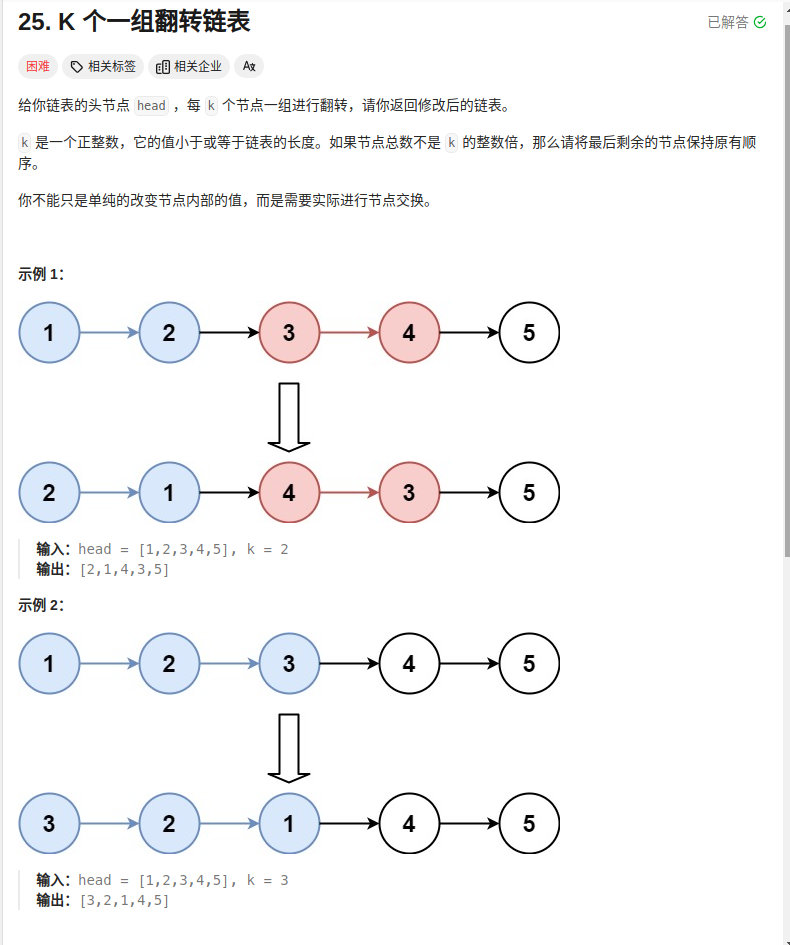
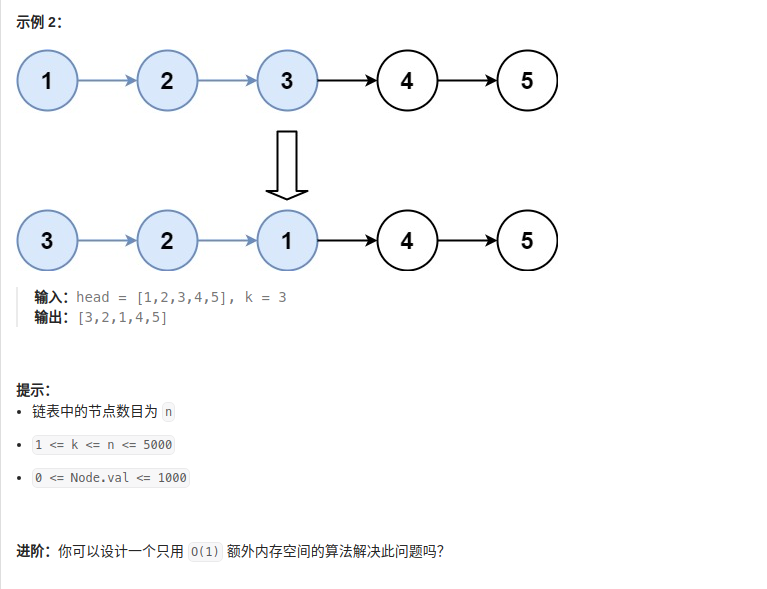
递归法:
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
if(k==1)
return head;
ListNode* newHead = nullptr;
ListNode* cur = head;
int count = 0;
while(cur && count < k){
count++;
newHead = cur;
cur = cur->next;
}
if(count < k)
return head;
//到这里时,newHead是第k个结点,它将成为反转后的新的头结点
ListNode* post = cur;//post是第k+1个结点
ListNode* pre = nullptr;
cur = head;
while(count--){
ListNode* temp = cur->next;
cur->next = pre;
pre = cur;
cur = temp;
}
head->next = reverseKGroup(post,k);//对第k+1个结点及其之后的结点递归处理
return newHead;
}
};