什么是juc
在java的java.util.concurrent包下的工具。
锁
传统的synchronize
java
public class SealTicket {
int total = 50;
public synchronized void seal() {
if (total > 0) {
System.out.println(Thread.currentThread().getName()
+ "卖出第"
+ (total--)
+ "张,剩余:" + total
);
}
}
}
JUC 的锁
java
public class SealTicket {
int total = 50;
Lock lock = new ReentrantLock();
public void seal() {
lock.lock();
if (total > 0) {
System.out.println(Thread.currentThread().getName()
+ "卖出第"
+ (total--)
+ "张,剩余:" + total
);
}
lock.unlock();
}
}
Synchronize 和 Lock的区别
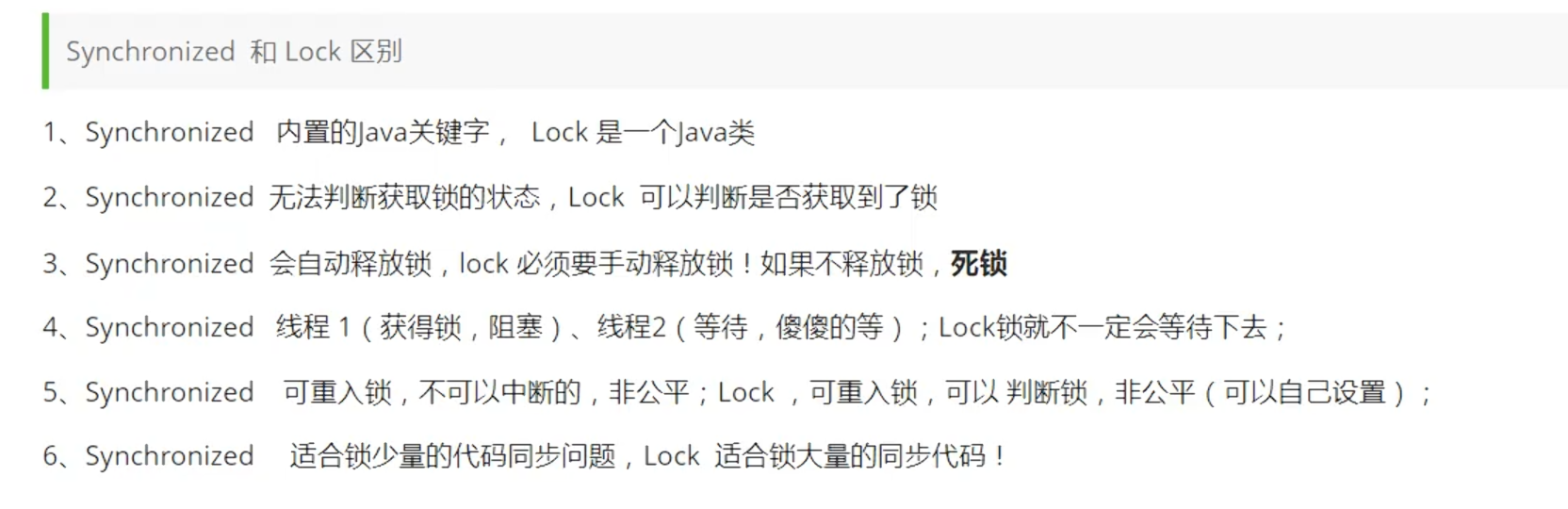
生产者和消费者问题
版本 synchronize
java
public class Data {
int i = 0;
public synchronized void add() throws InterruptedException {
if (i != 0) {
this.wait();
}
i++;
System.out.println(Thread.currentThread().getName() + "=="+ i);
this.notifyAll();
}
public synchronized void remove() throws InterruptedException {
if (i == 0) {
this.wait();
}
i--;
System.out.println(Thread.currentThread().getName() + "=="+ i);
this.notifyAll();
}
}
public class Producer {
public static void main(String[] args) {
Data data = new Data();
new Thread(() -> {
for (int i = 0; i < 60; i++) {
try {
data.add();
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}, "a").start();
new Thread(() -> {
for (int i = 0; i < 60; i++) {
try {
data.remove();
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}, "b").start();
// new Thread(() -> {
// for (int i = 0; i < 60; i++) {
// sealTicket.seal();
// }
// }, "c").start();
}
}
版本 Lock
8锁问题
锁是什么,锁锁的是谁?