运行在VS2022,x86,Debug下。
29. 桥接模式
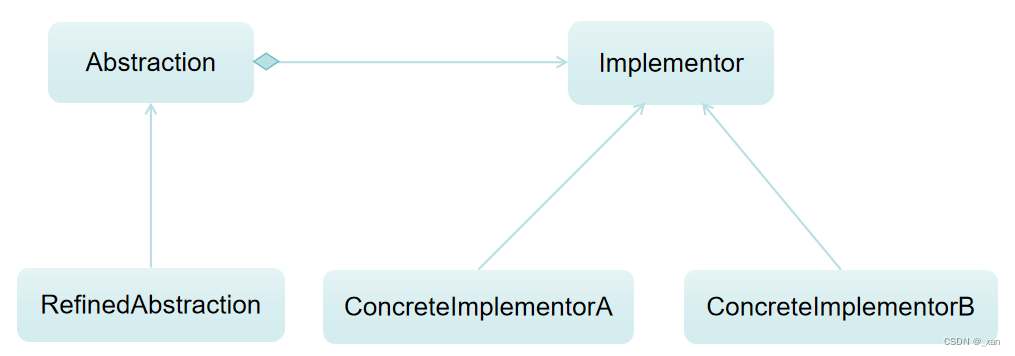
-
桥接模式将抽象与实现分离,使二者可以独立地变化。
-
应用:如在游戏开发中,多个角色和多个武器交叉组合时。可以使用桥接模式,定义角色抽象类,武器抽象类,两者通过桥接建立关联,使角色和武器之间的关系是松耦合的,可以独立变化,方便游戏后期修改或新填内容。
-
实现
- 实现体接口。
- 具体实现体。
- 抽象体接口,使用实现体对象。
- 具体抽象体。
-
代码如下。
cpp
//实现体接口:武器接口
class WeaponImplementor {
public:
virtual void attack() = 0;
};
//具体实现体:剑
class SwordImplementor : public WeaponImplementor
{
public:
void attack() { cout << "Slash with Sword" << endl; }
};
//具体实现体:弓箭
class BowImplementor : public WeaponImplementor
{
public:
void attack() { cout << "Shoot with Bow" << endl; }
};
//具体实现体:枪
class GunImplementor : public WeaponImplementor
{
public:
void attack() { cout << "Shoot with Gun" << endl; }
};
//抽象体接口:角色接口
class CharacterAbstraction
{
protected:
WeaponImplementor* usedWeapon; //桥接关系,使用武器对象
public:
CharacterAbstraction(WeaponImplementor* weapon): usedWeapon(weapon){}
void setWeapon(WeaponImplementor* weapon) { usedWeapon = weapon; }
virtual void operation() = 0;
};
//具体抽象体:战士
class WarriorAbstraction : public CharacterAbstraction
{
public:
WarriorAbstraction(WeaponImplementor* weapon) : CharacterAbstraction(weapon) {}
void operation()
{
cout << "Warrior ";
usedWeapon->attack();
}
};
//具体抽象体:弓手
class ArcherAbstraction : public CharacterAbstraction
{
public:
ArcherAbstraction(WeaponImplementor* weapon) : CharacterAbstraction(weapon) {}
void operation()
{
cout << "Archer ";
usedWeapon->attack();
}
};
int main()
{
//创建武器
SwordImplementor sword;
BowImplementor bow;
GunImplementor gun;
//创建角色,并分配武器
WarriorAbstraction warrior(&gun);
warrior.operation();
warrior.setWeapon(&sword);
warrior.operation();
ArcherAbstraction archer(&bow);
archer.operation();
return 0;
}