下面演示在GraphQL clients访问GraphQL 接口
1 修改baseType.js
添加可供用户访问的静态资源路径

代码如下:
const express = require('express');
const {buildSchema} = require('graphql');
const grapqlHTTP = require('express-graphql').graphqlHTTP;
// 定义schema,查询和类型
const schema = buildSchema(`
type Account {
name: String
age: Int
sex: String
department: String
salary(city: String): Int
}
type Query {
getClassMates(classNo: Int!): [String]
account(username: String): Account
}
`)
// 定义查询对应的处理器
const root = {
getClassMates({ classNo}) {
const obj = {
31: ['张三', '李四', '王五'],
61: ['张大三', '李大四', '王大五']
}
return obj[classNo];
},
account({ username}) {
const name = username;
const sex = 'man';
const age = 18;
const department = '开发部';
const salary = ({city}) => {
if(city === "北京" || city == "上海" || city == "广州" || city == "深圳") {
return 10000;
}
return 3000;
}
return {
name,
sex,
age,
department,
salary
}
}
}
const app = express();
app.use('/graphql', grapqlHTTP({
schema: schema,
rootValue: root,
graphiql: true
}))
// 公开文件夹,供用户访问静态资源
app.use(express.static('public'))
app.listen(3000);
2 编写index.html页面
设置参数
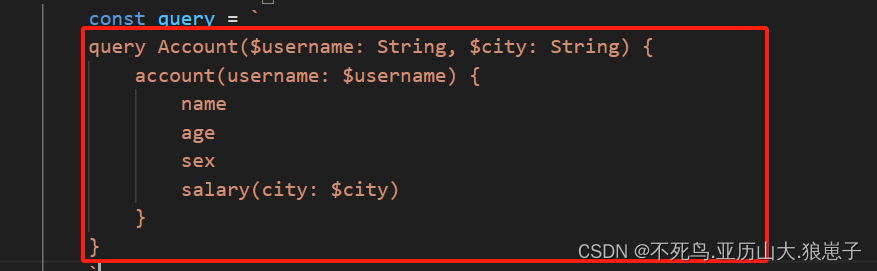
设置传入的数值
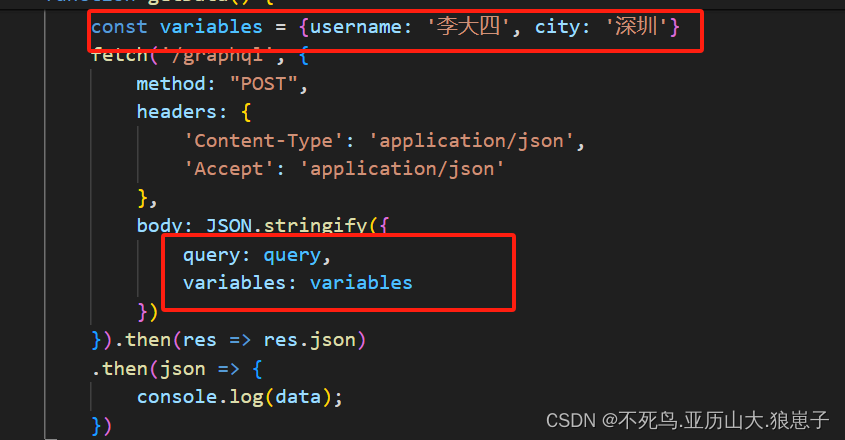
完整代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<button onclick="getData()">获取数据</button>
</body>
<script>
function getData() {
const query = `
query Account($username: String, $city: String) {
account(username: $username) {
name
age
sex
salary(city: $city)
}
}
`
const variables = {username: '李大四', city: '深圳'}
fetch('/graphql', {
method: "POST",
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: JSON.stringify({
query: query,
variables: variables
})
}).then(res => res.json)
.then(json => {
console.log(data);
})
}
</script>
</html>
启动服务:
node baseType.js
访问地址后,点击获取数据按钮,效果如下:
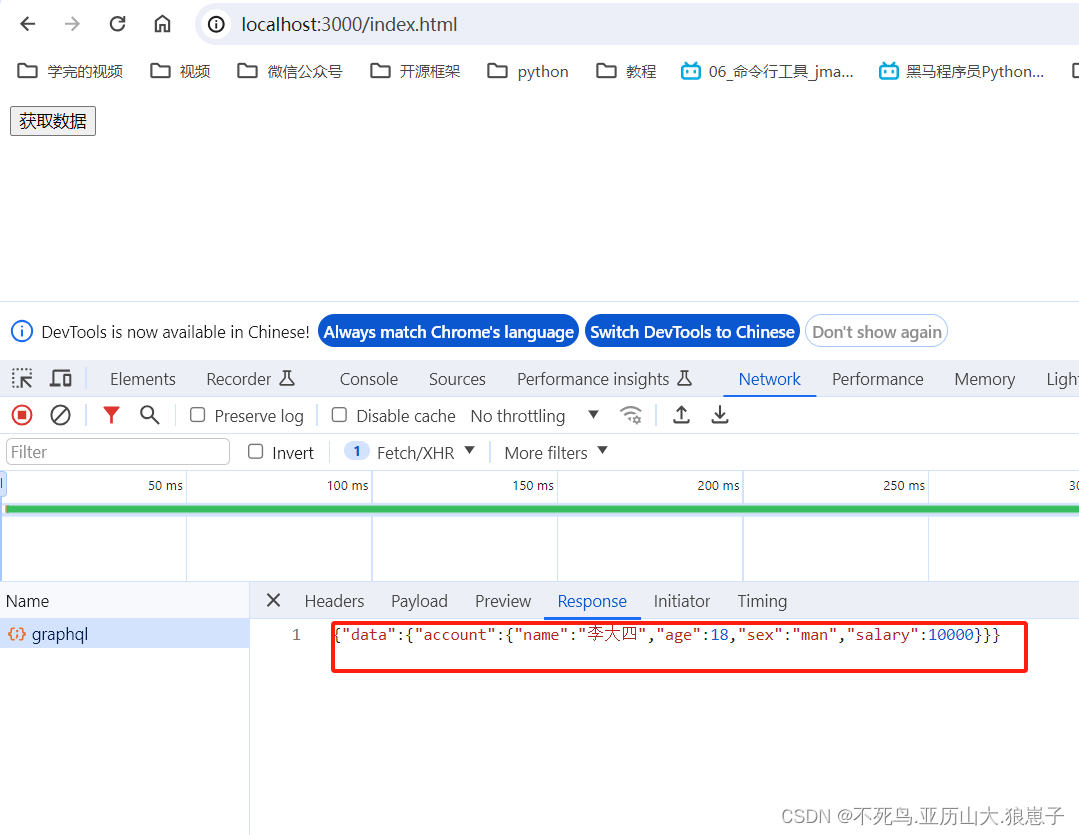