文章目录
-
- [深入分析 Android Service (四)](#深入分析 Android Service (四))
- [1. 使用 Messenger 进行通信](#1. 使用 Messenger 进行通信)
- [2. 详细示例:使用 Messenger 进行通信](#2. 详细示例:使用 Messenger 进行通信)
-
- [2.1 创建 MessengerService](#2.1 创建 MessengerService)
- [2.2 在 Activity 中绑定服务并发送消息](#2.2 在 Activity 中绑定服务并发送消息)
- [3. 使用 AIDL 进行进程间通信](#3. 使用 AIDL 进行进程间通信)
-
- [3.1 定义 AIDL 接口](#3.1 定义 AIDL 接口)
- [3.2 实现 AIDL 接口](#3.2 实现 AIDL 接口)
- [3.3 在客户端绑定 AIDL 服务](#3.3 在客户端绑定 AIDL 服务)
- [4. 优缺点分析](#4. 优缺点分析)
-
- [4.1 Messenger](#4.1 Messenger)
- [4.2 AIDL](#4.2 AIDL)
- [5. 总结](#5. 总结)
深入分析 Android Service (四)
1. 使用 Messenger 进行通信
Messenger
提供了一种轻量级的 IPC(进程间通信)机制,适合在进程间或组件间发送简单消息。Messenger
基于 Handler
实现,便于处理异步消息。
2. 详细示例:使用 Messenger 进行通信
以下是一个使用 Messenger
在 Activity
和 Service
之间进行通信的示例。
2.1 创建 MessengerService
首先,创建一个 MessengerService
类,继承自 Service
。
java
public class MessengerService extends Service {
static final int MSG_SAY_HELLO = 1;
class IncomingHandler extends Handler {
@Override
public void handleMessage(Message msg) {
switch (msg.what) {
case MSG_SAY_HELLO:
Toast.makeText(getApplicationContext(), "Hello!", Toast.LENGTH_SHORT).show();
break;
default:
super.handleMessage(msg);
}
}
}
final Messenger messenger = new Messenger(new IncomingHandler());
@Override
public IBinder onBind(Intent intent) {
return messenger.getBinder();
}
}
在这个示例中,MessengerService
接收并处理从客户端发送的消息。
2.2 在 Activity 中绑定服务并发送消息
在 Activity
中绑定 MessengerService
并发送消息:
java
public class MainActivity extends AppCompatActivity {
Messenger messenger = null;
boolean isBound = false;
private ServiceConnection connection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
messenger = new Messenger(service);
isBound = true;
}
@Override
public void onServiceDisconnected(ComponentName name) {
messenger = null;
isBound = false;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Intent intent = new Intent(this, MessengerService.class);
bindService(intent, connection, Context.BIND_AUTO_CREATE);
Button sendButton = findViewById(R.id.sendButton);
sendButton.setOnClickListener(v -> {
if (isBound) {
Message msg = Message.obtain(null, MessengerService.MSG_SAY_HELLO, 0, 0);
try {
messenger.send(msg);
} catch (RemoteException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
if (isBound) {
unbindService(connection);
isBound = false;
}
}
}
3. 使用 AIDL 进行进程间通信
AIDL(Android Interface Definition Language)是一种用于定义接口的语言,使得在不同的进程之间进行复杂的数据通信成为可能。适合需要在不同进程间传递复杂数据结构的场景。
3.1 定义 AIDL 接口
创建一个 AIDL 文件 IMyAidlInterface.aidl
:
aidl
package com.example;
interface IMyAidlInterface {
int add(int a, int b);
}
3.2 实现 AIDL 接口
在 Service
中实现 AIDL 接口:
java
public class MyAidlService extends Service {
private final IMyAidlInterface.Stub binder = new IMyAidlInterface.Stub() {
@Override
public int add(int a, int b) {
return a + b;
}
};
@Override
public IBinder onBind(Intent intent) {
return binder;
}
}
3.3 在客户端绑定 AIDL 服务
在 Activity
中绑定 MyAidlService
并调用方法:
java
public class MainActivity extends AppCompatActivity {
IMyAidlInterface myAidlService = null;
boolean isBound = false;
private ServiceConnection connection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
myAidlService = IMyAidlInterface.Stub.asInterface(service);
isBound = true;
}
@Override
public void onServiceDisconnected(ComponentName name) {
myAidlService = null;
isBound = false;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Intent intent = new Intent(this, MyAidlService.class);
bindService(intent, connection, Context.BIND_AUTO_CREATE);
Button addButton = findViewById(R.id.addButton);
addButton.setOnClickListener(v -> {
if (isBound) {
try {
int result = myAidlService.add(5, 3);
Toast.makeText(MainActivity.this, "Result: " + result, Toast.LENGTH_SHORT).show();
} catch (RemoteException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
if (isBound) {
unbindService(connection);
isBound = false;
}
}
}
4. 优缺点分析
4.1 Messenger
优点:
- 实现简单,适合轻量级 IPC。
- 基于
Handler
,容易与 Android 的消息机制集成。
缺点:
- 只适合传递简单数据,不适用于复杂数据结构或大数据传输。
4.2 AIDL
优点:
- 强大的跨进程通信能力,支持复杂数据结构。
- 自动生成接口和 Stub 代码,减少编码错误。
缺点:
- 实现复杂,增加开发和维护难度。
- 性能开销较高,不适合频繁调用。
5. 总结
通过上述示例和分析,深入理解了 Android 中 Service
的设计和实现,以及如何在 Activity
与 Service
之间进行高效的通信。选择合适的通信方式(如 Messenger
或 AIDL
)取决于具体需求和应用场景。合理使用 Service
和通信机制,可以有效提升 Android 应用的性能和用户体验。
理解 Service
的工作机制、生命周期管理和通信方法,是构建高效、稳定的 Android 应用的重要一环。希望这些示例和详细说明能够帮助开发者更好地理解和使用 Service
,实现更强大和高效的应用功能。
|----------------------------------|
| 欢迎点赞|关注|收藏|评论,您的肯定是我创作的动力 |
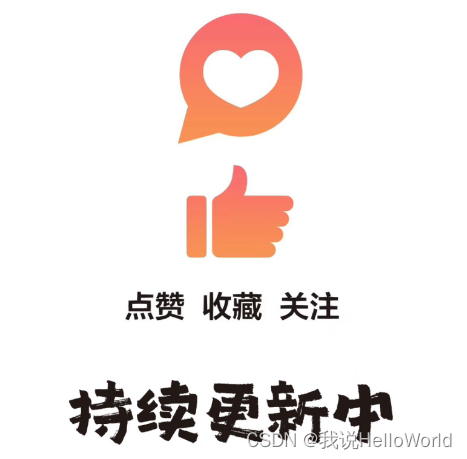