因为这个项目license问题无法开源,更多技术支持与服务请加入我的知识星球。
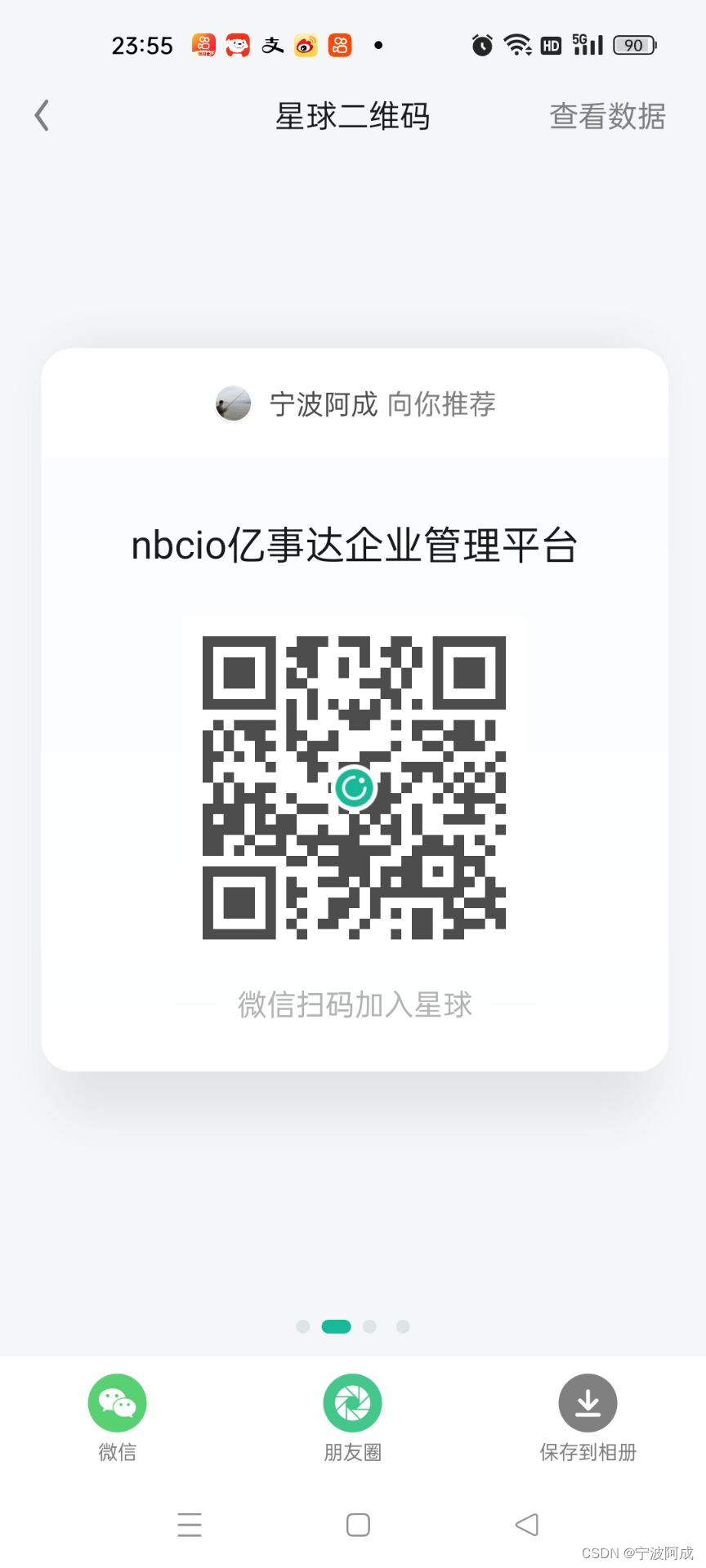
1、api接口部分
javascript
import { defHttp } from '/@/utils/http/axios';
enum Api {
flowRecord = '/flowable/task/flowRecord',
finishedListNew = '/flowable/task/finishedListNew',
revokeProcess = '/flowable/task/revokeProcess',
recallProcess = '/flowable/task/recallProcess',
}
// 任务流转记录
export const flowRecord = (params: any) => defHttp.get({ url: Api.flowRecord, params },{ isTransformResponse: false });
// 查询已办任务列表
export const finishedListNew = (params: any) => defHttp.get({ url: Api.finishedListNew, params });
// 撤回任务
export const revokeProcess = (data: any) => defHttp.post({ url: Api.revokeProcess, data },{ isTransformResponse: false });
// 收回任务
export const recallProcess = (data: any) => defHttp.post({ url: Api.recallProcess, data },{ isTransformResponse: false });
2、列表数据显示部分
javascript
import {BasicColumn} from '/@/components/Table';
import {FormSchema} from '/@/components/Table';
import { rules} from '/@/utils/helper/validator';
import { render } from '/@/utils/common/renderUtils';
//列表数据
export const columns: BasicColumn[] = [
{
title: '#',
dataIndex: '',
key:'rowIndex',
width:60,
align:"center"
},
{
title:'任务编号',
align:"center",
dataIndex: 'procInsId'
},
{
title:'流程名称',
align:"center",
dataIndex: 'procDefName'
},
{
title:'任务节点',
align:"center",
dataIndex: 'taskName'
},
{
title:'流程类别',
align:"center",
dataIndex: 'category'
},
{
title:'流程版本',
align:"center",
dataIndex: 'procDefVersion'
},
{
title:'业务主键',
align:"center",
dataIndex: 'businessKey'
},
{
title:'流程发起人',
align:"center",
dataIndex: 'startUserName'
},
{
title:'接收时间',
align:"center",
dataIndex: 'createTime'
},
{
title:'审批时间',
align:"center",
dataIndex: 'finishTime'
},
{
title:'耗时',
align:"center",
dataIndex: 'duration'
},
];
//查询数据
export const searchFormSchema: FormSchema[] = [
{
label: "流程名称",
field: 'procDefName',
component: 'Input',
//colProps: {span: 6},
},
{
label: "接收日期",
field: 'createTime',
component: 'DatePicker',
//colProps: {span: 6},
},
{
label: "创建人员",
field: 'createBy',
component: 'Input',
//colProps: {span: 6},
},
];
//表单数据
export const formSchema: FormSchema[] = [
{
title:'任务编号',
align:"center",
dataIndex: 'procInsId'
},
{
title:'流程名称',
align:"center",
dataIndex: 'procDefName'
},
{
title:'任务节点',
align:"center",
dataIndex: 'taskName'
},
{
title:'流程类别',
align:"center",
dataIndex: 'category'
},
{
title:'流程版本',
align:"center",
dataIndex: 'procDefVersion'
},
{
title:'业务主键',
align:"center",
dataIndex: 'businessKey'
},
{
title:'流程发起人',
align:"center",
dataIndex: 'startUserName'
},
{
title:'接收时间',
align:"center",
dataIndex: 'createTime'
},
{
title:'审批时间',
align:"center",
dataIndex: 'finishTime'
},
{
title:'耗时',
align:"center",
dataIndex: 'duration'
},
// TODO 主键隐藏字段,目前写死为ID
{
label: '',
field: 'id',
component: 'Input',
show: false,
},
];
// 高级查询数据
export const superQuerySchema = {
procInsId: {title: '任务编号',order: 0,view: 'text', type: 'string',},
procDefName: {title: '流程名称',order: 1,view: 'text', type: 'string',},
category: {title: '流程类别',order: 2,view: 'text', type: 'string',},
procDefVersion: {title: '流程版本',order: 3,view: 'text', type: 'string',},
businessKey: {title: '业务主键',order: 4,view: 'text', type: 'string',},
startUserName: {title: '流程发起人',order: 5,view: 'text', type: 'string',},
createTime: {title: '接收时间',order: 6,view: 'date', type: 'string',},
finishTime: {title: '审批时间',order: 7,view: 'date', type: 'string',},
duration: {title: '耗时',order: 8,view: 'text', type: 'string',},
taskName: {title: '当前节点',order: 9,view: 'text', type: 'string',},
};
3、效果图如下
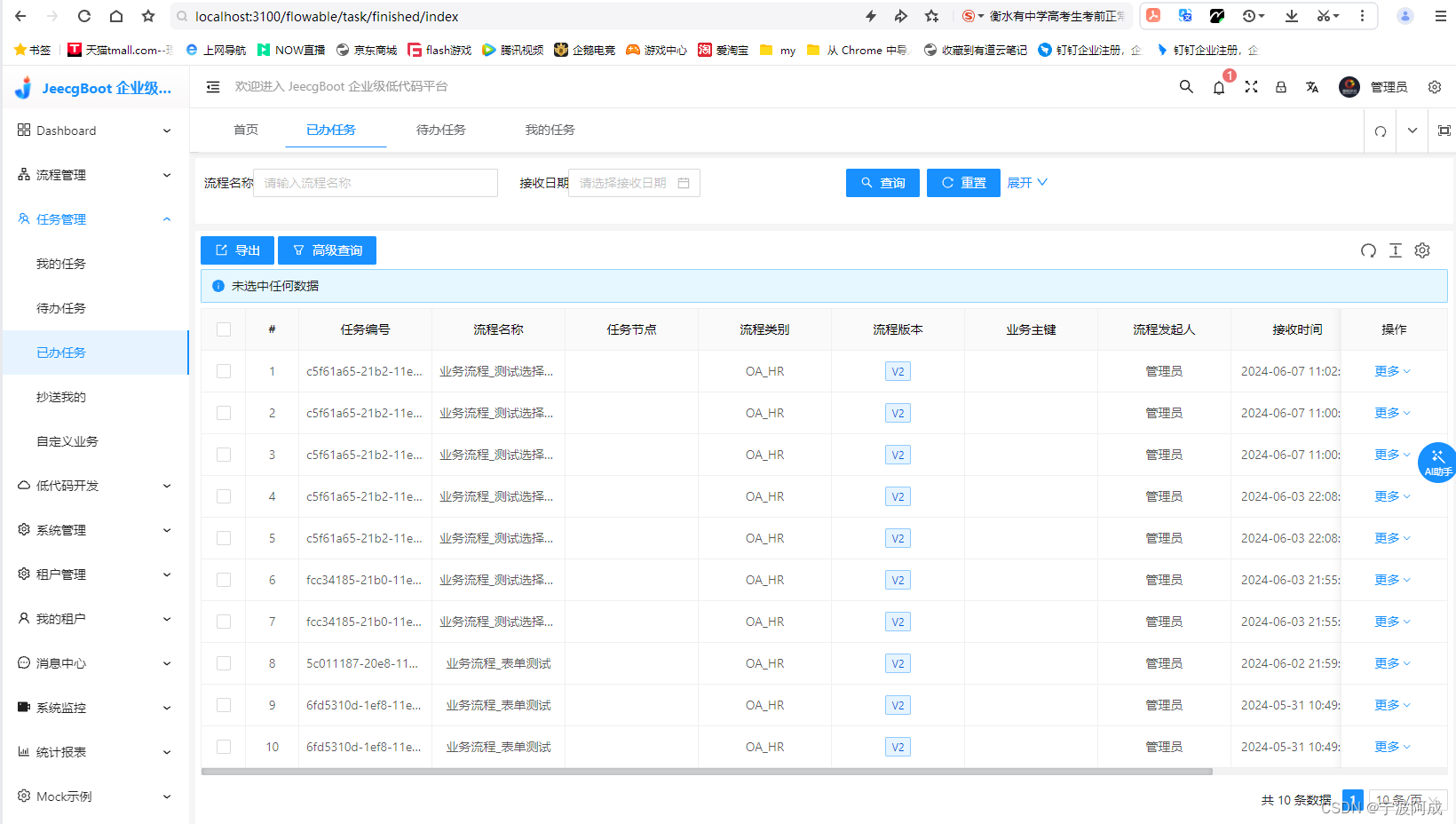