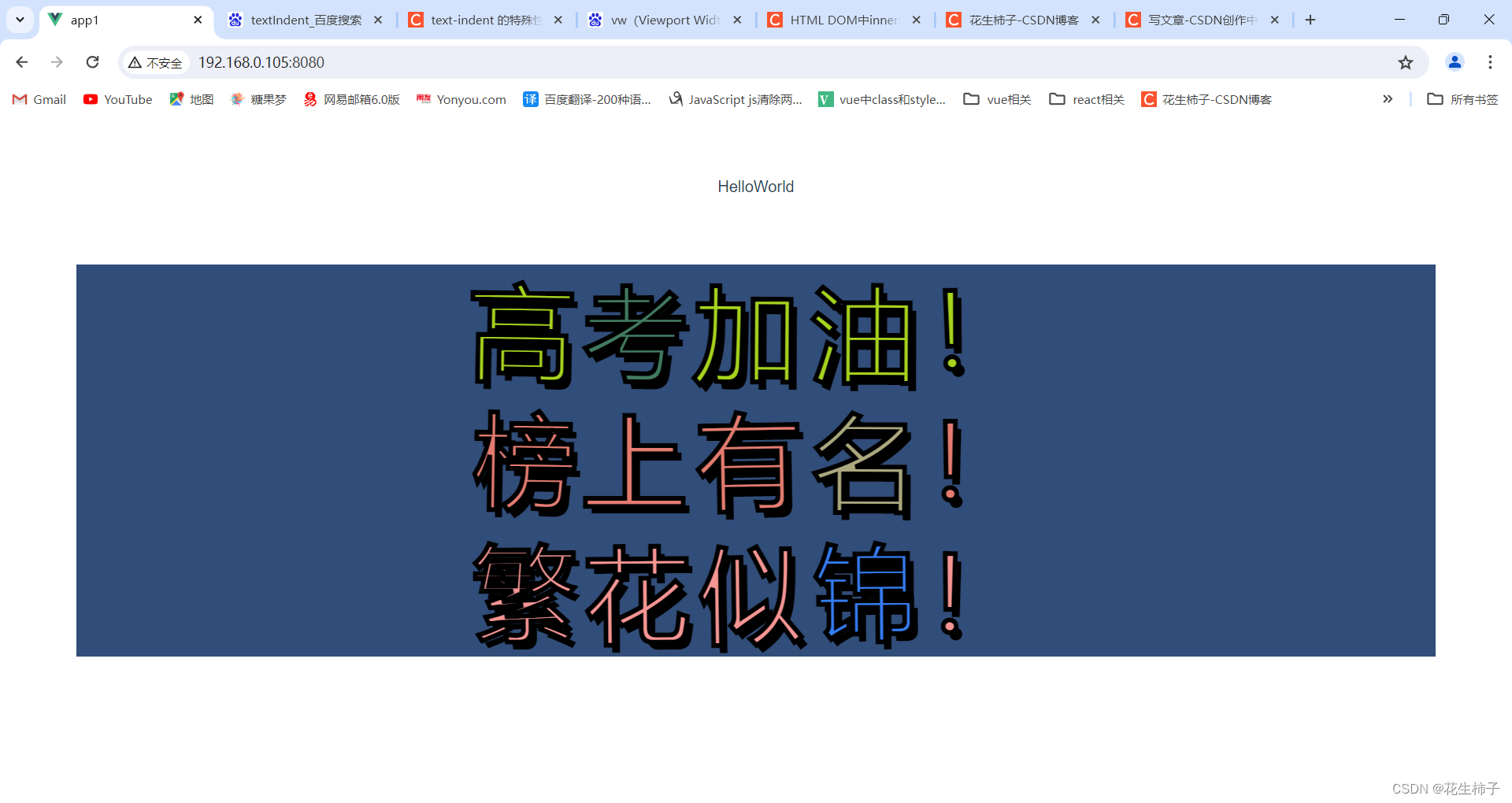
1、获取文本内容
首先,获取每个<div>
元素的文本内容,并清空其内部HTML(innerHTML = "")。
2、创建<span>
元素
然后,它遍历文本的每个字符,为每个字符创建一个新的<span>
元素,并将该字符设置为<span>
元素的文本内容。
3、设置样式
为每个<span>
元素设置外边距(marginRight),这样字符之间会有一定的间隔。
4、打乱顺序
使用shuffle函数随机打乱<span>
元素的顺序。这个函数通过Fisher-Yates算法实现随机置换。
5、应用随机变换
applyRandomTransform函数为每个<span>
元素随机生成位移(xOffset和yOffset)和旋转角度(rotation),并应用CSS的transform属性来改变每个字符的位置和方向。
6、随机颜色变化
changeColorSequentially函数逐个改变<span>
元素的颜色,每次只改变一个字符的颜色,并在颜色变化时保持顺序感。它使用getRandomColor函数生成随机颜色代码。
7、定时器
通过setInterval函数,changeColorSequentially和applyRandomTransform函数被定期调用,使得颜色和变换效果持续发生,创建动态变化的效果。
8、文本描边和阴影
在CSS中,使用-webkit-text-stroke-width和-webkit-text-stroke-color为文本添加描边效果,使用text-shadow添加阴影效果,增强文本的视觉效果。
<template>
<div>
<!-- <img alt="Vue logo" src="./assets/logo.png"> -->
<HelloWorld msg="Welcome to Your Vue.js App" />
<!-- <router-view /> -->
<div class="bodys">
<div class="rugrats">高考加油!</div>
<div class="rugrats">榜上有名!</div>
<div class="rugrats">繁花似锦!</div>
</div>
</div>
</template>
<script>
import HelloWorld from "./components/HelloWorld.vue";
export default {
name: "App",
components: {
HelloWorld,
},
mounted() {
this.init();
},
methods: {
init() {
const divs = document.querySelectorAll(".rugrats");
divs.forEach(function (div) {
const text = div.textContent;
div.innerHTML = "";
for (let i = 0; i < text.length; i++) {
const span = document.createElement("span");
span.textContent = text[i];
span.style.marginRight = "1vw";
div.appendChild(span);
}
let spans = div.querySelectorAll("span");
function shuffle(array) {
for (let i = array.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
const temp = array[i];
array[i] = array[j];
array[j] = temp;
}
return array;
}
spans = shuffle(Array.from(spans));
function getRandomValue() {
return Math.random() * 0.4 - 0.24;
}
function applyRandomTransform() {
spans.forEach(function (span) {
const xOffset = getRandomValue() * 10;
const yOffset = getRandomValue() * 15;
const rotation = getRandomValue() * 6;
span.style.transform =
"translate(" +
xOffset +
"px, " +
yOffset +
"px) rotate(" +
rotation +
"deg)";
span.style.textIndent = xOffset + "px";
});
}
function getRandomColor() {
const letters = "0123456789ABCDEF";
let color = "#";
for (var i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
let currentIndex = 0;
function changeColorSequentially() {
spans.forEach(function (span, index) {
let colorIndex = (index + currentIndex) % spans.length;
span.style.color =
colorIndex === 0
? getRandomColor()
: spans[colorIndex - 1].style.color;
});
currentIndex = (currentIndex + 1) % spans.length;
}
setInterval(changeColorSequentially, 250);
setInterval(applyRandomTransform, 100);
});
},
},
};
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
.bodys {
margin: 10vh;
text-align: center;
font-size: calc(5vw + 4vh);
background: #314d79;
color: #fff;
font-family: sans-serif;
letter-spacing: -0.3vw;
overflow: hidden;
font-family: "Lacquer", system-ui;
}
.rugrats {
margin: 0 auto;
text-align: center;
}
.rugrats span {
display: inline-block;
transition: color linear 0.5s forwards;
-webkit-text-stroke-width: 0.32vw;
-webkit-text-stroke-color: black;
text-shadow: 0.4vw 0.5vw #000;
}
.rugrats span {
text-transform: capitalize;
text-transform: lowercase;
}
</style>