Django 的 DeleteView
是一个基于类的视图,用于处理对象的删除操作。
1,添加视图函数
Test/app3/views.py
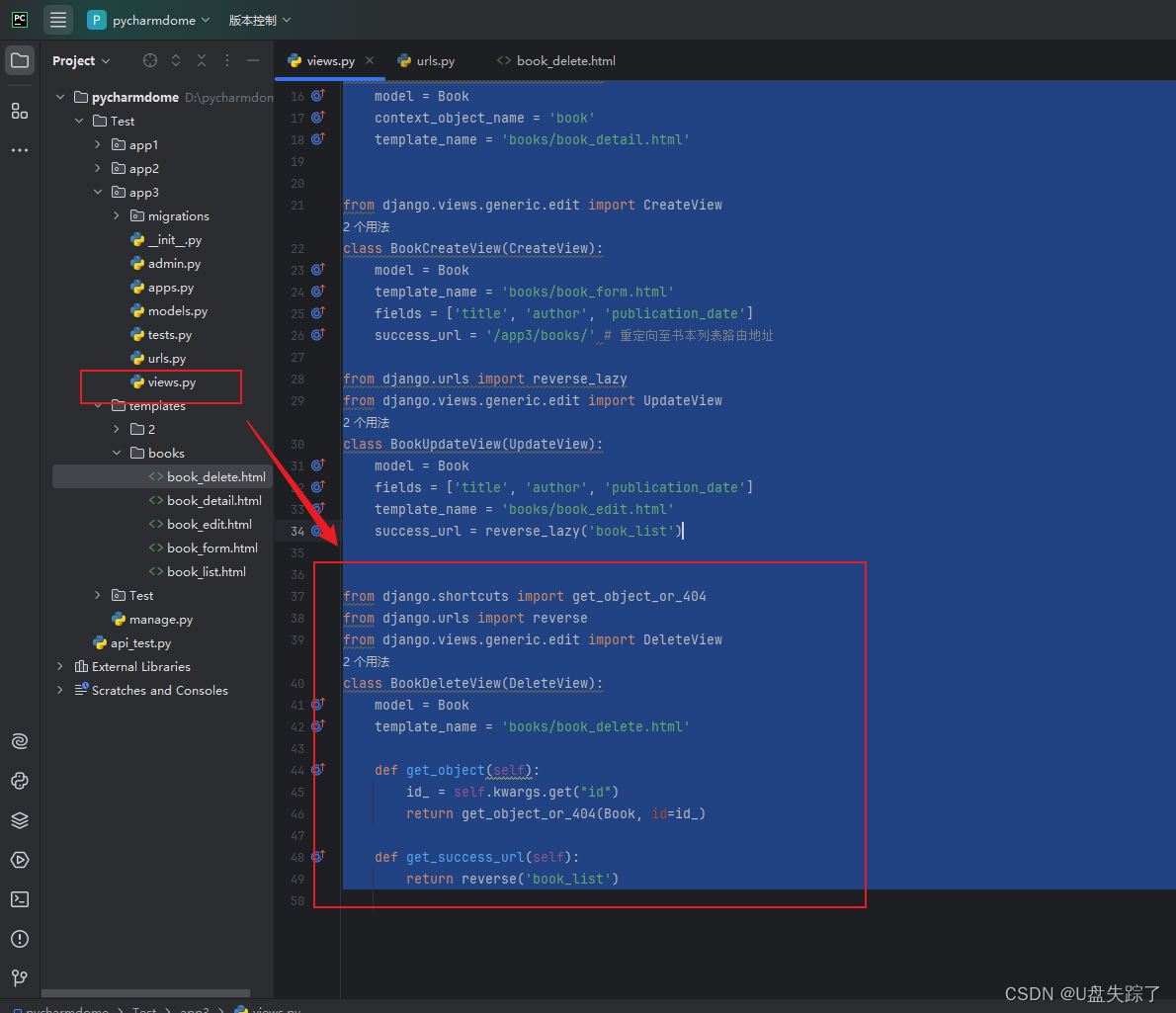
from django.shortcuts import render
# Create your views here.
from .models import Book
from django.views.generic import ListView
class BookListView(ListView):
model = Book
context_object_name = 'books'
template_name = 'books/book_list.html'
paginate_by = 10 # 设置展示页数数据
from django.views.generic import DetailView
class BookDetailView(DetailView):
model = Book
context_object_name = 'book'
template_name = 'books/book_detail.html'
from django.views.generic.edit import CreateView
class BookCreateView(CreateView):
model = Book
template_name = 'books/book_form.html'
fields = ['title', 'author', 'publication_date']
success_url = '/app3/books/' # 重定向至书本列表路由地址
from django.urls import reverse_lazy
from django.views.generic.edit import UpdateView
class BookUpdateView(UpdateView):
model = Book
fields = ['title', 'author', 'publication_date']
template_name = 'books/book_edit.html'
success_url = reverse_lazy('book_list')
from django.shortcuts import get_object_or_404
from django.urls import reverse
from django.views.generic.edit import DeleteView
class BookDeleteView(DeleteView):
model = Book
template_name = 'books/book_delete.html'
def get_object(self):
id_ = self.kwargs.get("id")
return get_object_or_404(Book, id=id_)
def get_success_url(self):
return reverse('book_list')
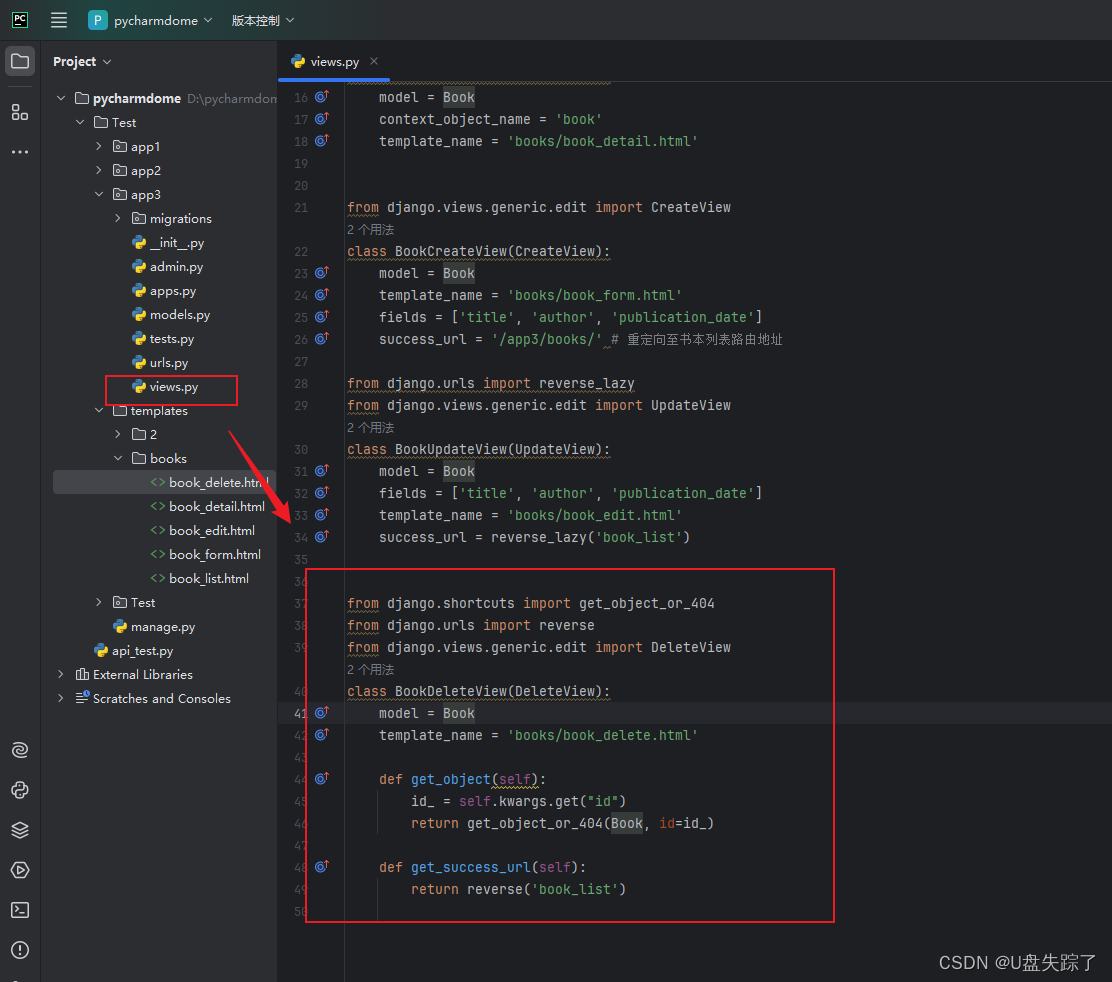
2,添加路由地址
Test/app3/urls.py
from django.urls import path
from . import views
from .views import BookListView
from .views import BookDetailView
from .views import BookCreateView
from .views import BookUpdateView
from .views import BookDeleteView
urlpatterns = [
path('books/', BookListView.as_view(), name='book_list'),
path('books/<int:pk>/', BookDetailView.as_view(), name='book_detail'),
path('books/new/', BookCreateView.as_view(), name='book_new'),
path('books/<int:pk>/edit/', BookUpdateView.as_view(), name='BookUpdateView'),
path('books/delete/<int:id>/', BookDeleteView.as_view(), name='book_delete'),
]
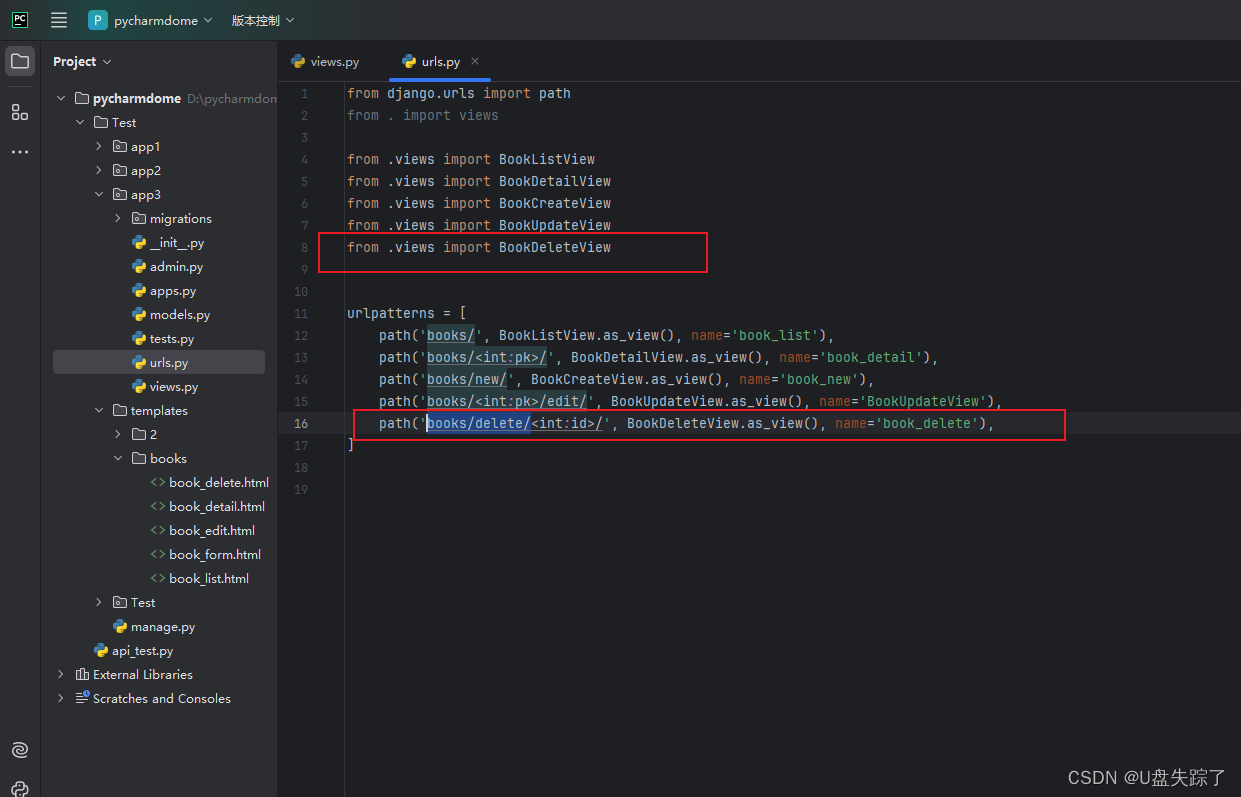
3,添加html代码
Test/templates/books/book_delete.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form method="POST">
{% csrf_token %}
<p>你确定要删除这本书吗?</p>
<button type="submit">确认删除</button>
</form>
</body>
</html>
4,访问页面
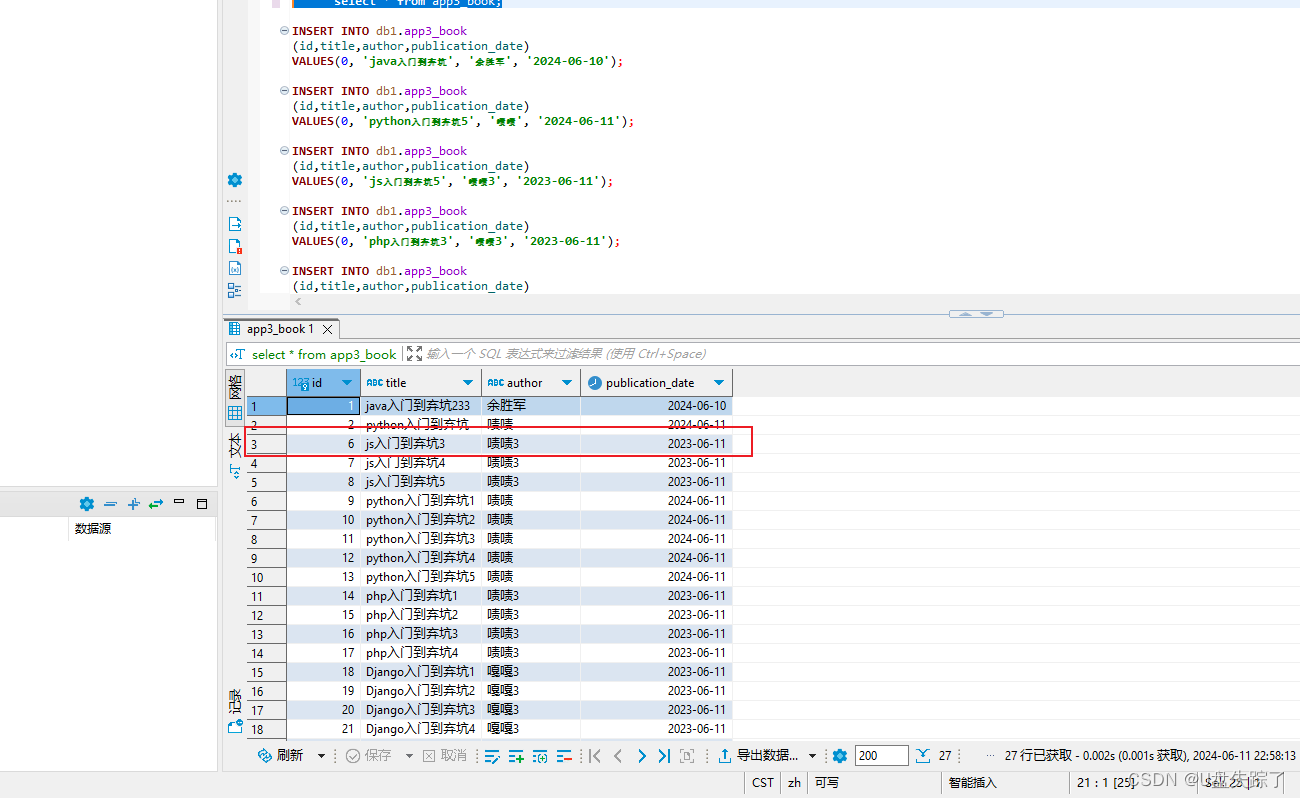
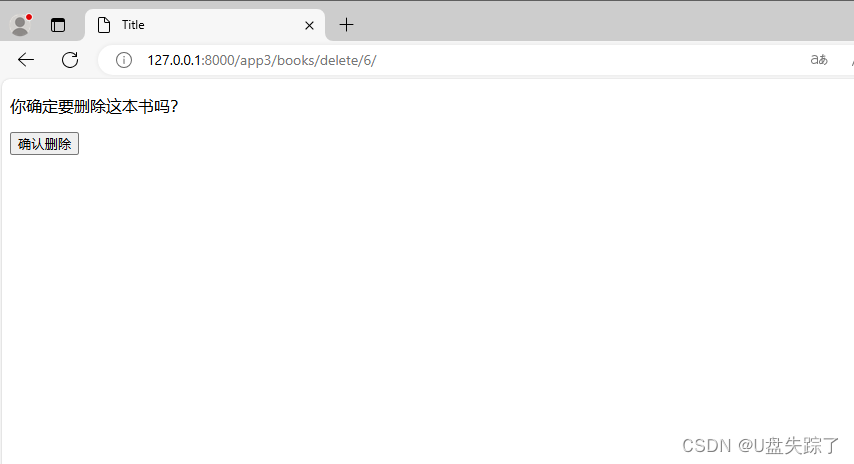
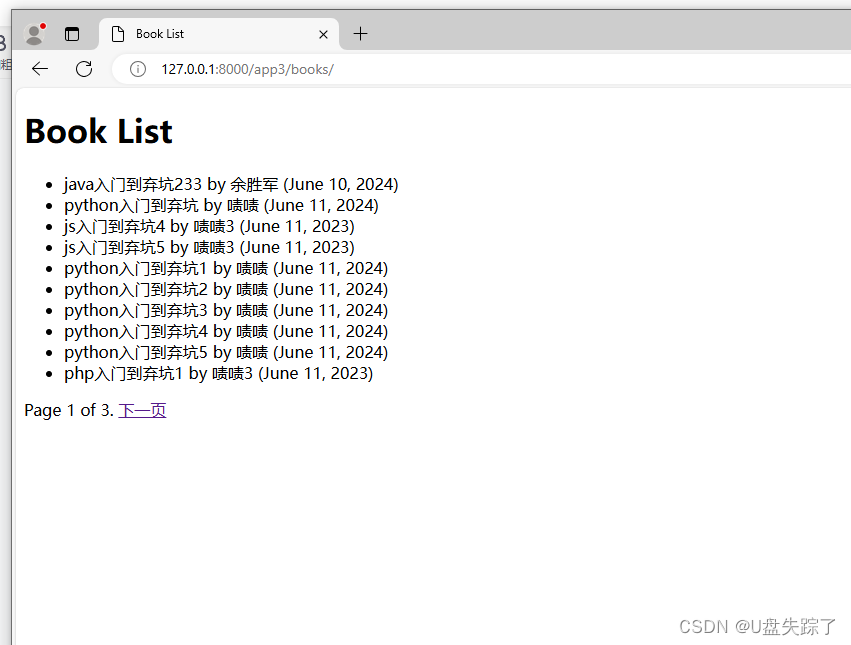
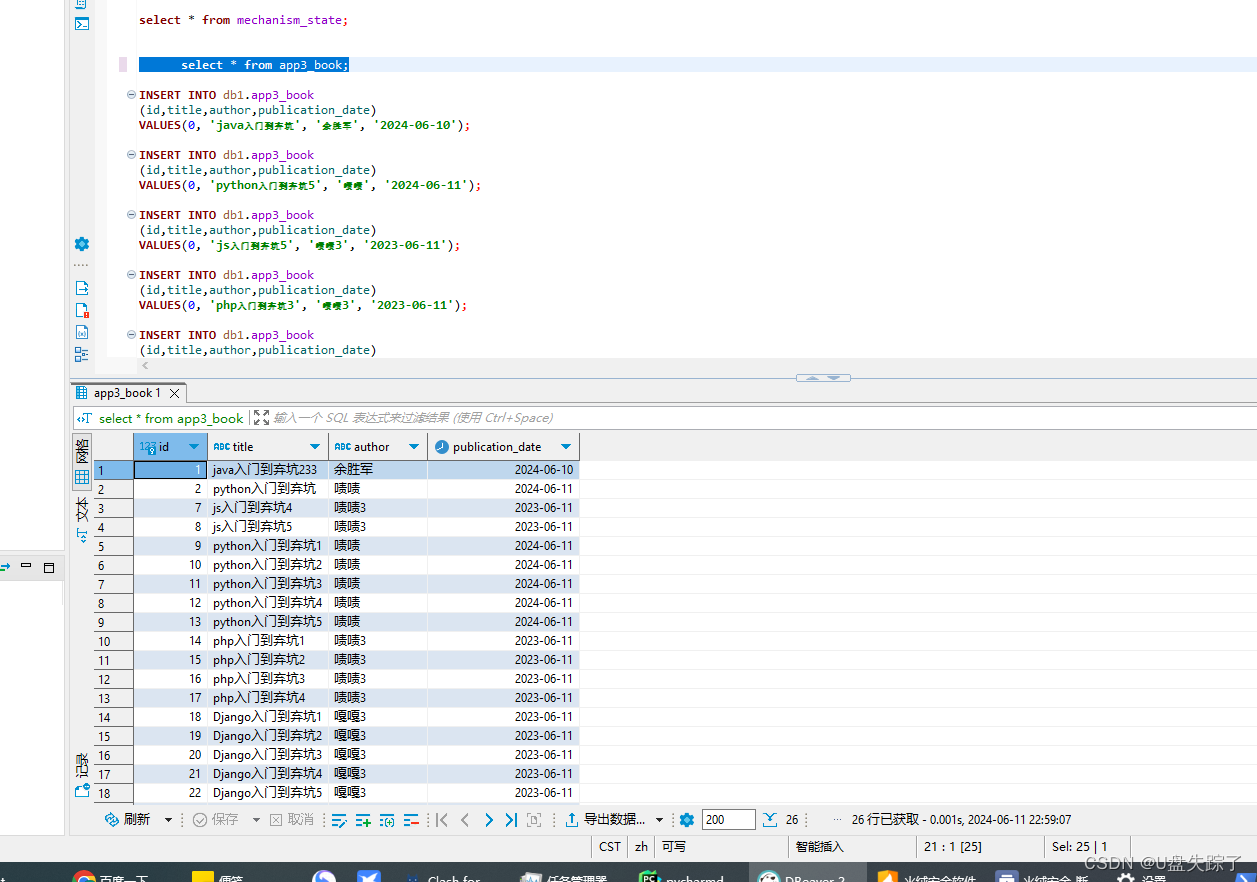