在项目中,我们经常要遇到查询和展示内容,常用的做法是通过文本框,时间控件,按键和datagridview查询和展示内容。下面是一个常见的综合实例,并支持Excel(csv)导入导出,表格列动态调整的功能。
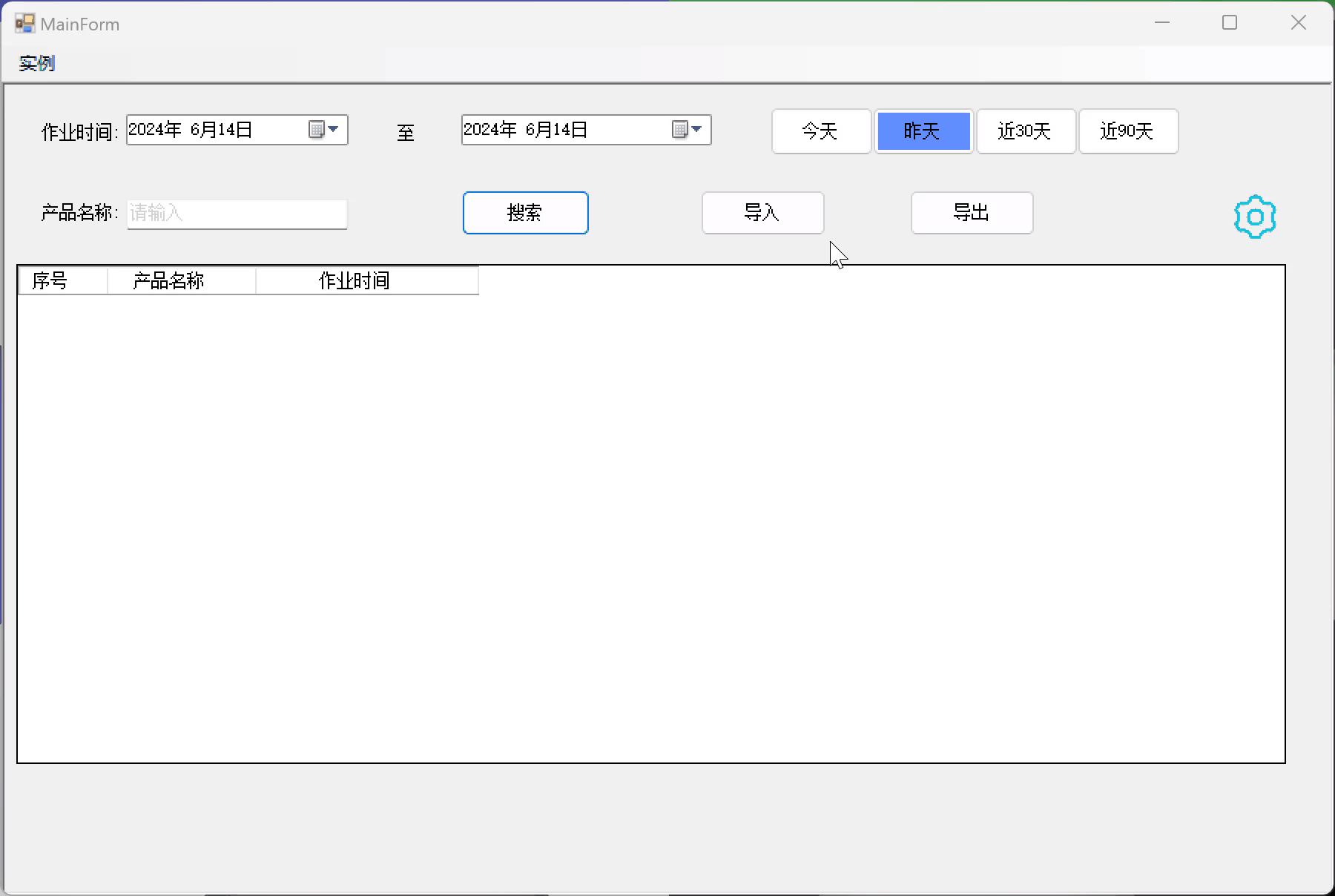
实例代码链接:https://download.csdn.net/download/lvxingzhe3/89436315
Excel(csv)导入到Datagridview:
cs
/// <summary>
/// 将csv文件数据导入datagridview
/// </summary>
/// <param name="csvPath"></param>
/// <returns></returns>
public static void ImportCSV(DataGridView dgv)
{
string filePath;
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "CSV files (*.csv)|*.csv";
openFileDialog.FilterIndex = 0;
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
filePath = openFileDialog.FileName;
}
else
{
return;
}
DataTable dt = new DataTable();
using (StreamReader sr = new StreamReader(filePath))
{
string[] headers = sr.ReadLine().Split(',');
string headerValue = null;
foreach (string header in headers)
{
headerValue = header.Replace("\"", "");
dt.Columns.Add(headerValue);
}
while (!sr.EndOfStream)
{
string[] rows = sr.ReadLine().Split(',');
DataRow dr = dt.NewRow();
for (int i = 0; i < headers.Length; i++)
{
dr[i] = rows[i].Replace("\"", "");
}
dt.Rows.Add(dr);
}
}
dgv.DataSource = dt;
}
Datagridview导出到excel(csv)
cs
/// <summary>
/// DateGridView导出到csv格式的Excel,通用
/// </summary>
/// <param name="dgv"></param>
public static void ExportToCSV(DataGridView dgv)
{
string filePath;
SaveFileDialog saveFileDialog = new SaveFileDialog();
saveFileDialog.Filter = "CSV files (*.csv)|*.csv";
saveFileDialog.FilterIndex = 0;
if (saveFileDialog.ShowDialog() == DialogResult.OK)
{
filePath = saveFileDialog.FileName;
}
else
{
return;
}
using (StreamWriter sw = new StreamWriter(filePath))
{
// 写入列标题
string headers = string.Join(",", dgv.Columns.Cast<DataGridViewColumn>().Select(column => "\"" + column.HeaderText + "\"").ToArray());
sw.WriteLine(headers);
// 写入数据行
foreach (DataGridViewRow row in dgv.Rows)
{
string line = string.Join(",", row.Cells.Cast<DataGridViewCell>().Select(cell => "\"" + cell.Value?.ToString().Replace("\"", "\"\"") + "\"").ToArray());
sw.WriteLine(line);
}
}
}
实例代码链接:https://download.csdn.net/download/lvxingzhe3/89436315