目录
题目:
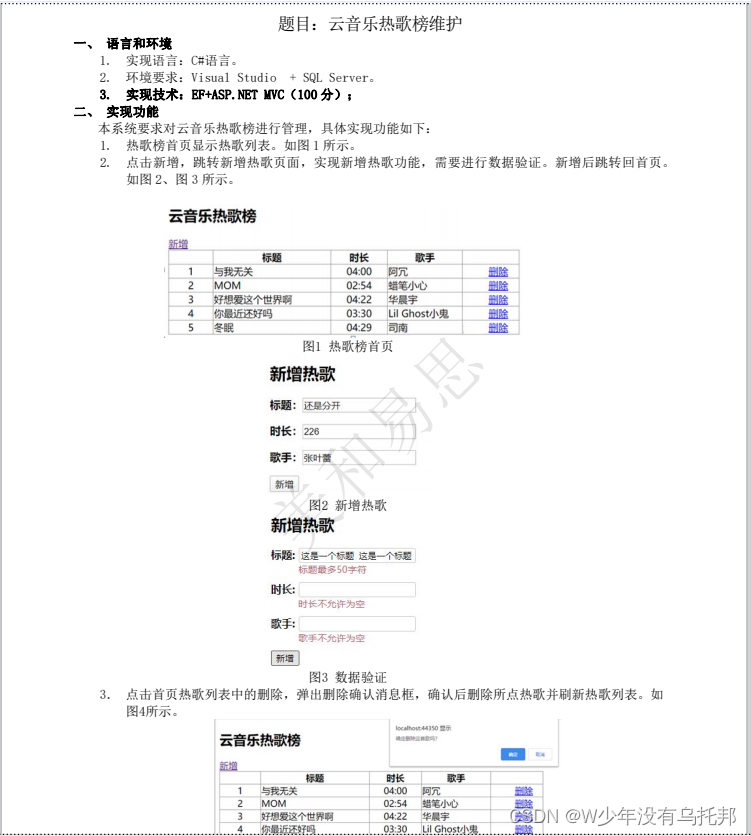
实现过程
控制器代码
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using MvcApplication1.Models;
namespace MvcApplication1.Controllers
{
public class HomeController : Controller
{
//
// GET: /Home/
public ActionResult Index()
{
ViewBag.Show = BLL.PropertyInfoManager.Show();
return View();
}
public ActionResult Jia()
{
return View();
}
[HttpPost]
public ActionResult Jia(string Title, string Duration, string Singer)
{
HotSong model = new HotSong();
model.Title = Title;
model.Duration =int.Parse(Duration);
model.Singer = Singer;
BLL.PropertyInfoManager.Add(model);
return RedirectToAction("Index");
}
public ActionResult Delect(int id)
{
BLL.PropertyInfoManager.Delect(id);
return RedirectToAction("Index");
}
}
}
DAL
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using MvcApplication1.Models;
namespace MvcApplication1.DAL
{
public class PropertyInfoServices
{
public static List<HotSong> Show() {
CloudMusicDBEntities db = new CloudMusicDBEntities();
return db.HotSongs.ToList();
}
public static HotSong FindModel(int id)
{
CloudMusicDBEntities db = new CloudMusicDBEntities();
return db.HotSongs.SingleOrDefault(x => x.Id == id);
}
public static bool Delect(int id)
{
HotSong model = FindModel(id);
CloudMusicDBEntities db = new CloudMusicDBEntities();
db.Entry(model).State = System.Data.EntityState.Deleted;
return db.SaveChanges() > 0;
}
public static bool Add(HotSong model)
{
CloudMusicDBEntities db = new CloudMusicDBEntities();
db.Entry(model).State = System.Data.EntityState.Added;
return db.SaveChanges() > 0;
}
}
}
BLL
csusing System; using System.Collections.Generic; using System.Linq; using System.Web; using MvcApplication1.Models; namespace MvcApplication1.BLL { public class PropertyInfoManager { public static List<HotSong> Show() { return DAL.PropertyInfoServices.Show(); } public static bool Delect(int id) { return DAL.PropertyInfoServices.Delect(id); } public static bool Add(HotSong model) { return DAL.PropertyInfoServices.Add(model); } } }
Index
html
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div>
<a href="/Home/Jia">添加</a>
<table border="1" style=" position:absolute;top:5%;right:70%">
<tr>
<th></th>
<th>标题</th>
<th>时长</th>
<th>歌手</th>
<th></th>
</tr>
@foreach (var item in @ViewBag.Show as List<MvcApplication1.Models.HotSong>)
{
<tr>
<td>@item.Id</td>
<td>@item.Title</td>
@{
var a = item.Duration % 60;
var b = item.Duration / 60;
}
<td>@b:@a </td>
<td>@item.Singer</td>
<td>@Html.ActionLink("删除", "Delect", new { id = @item.Id }, new {onclick="return confirm('确定删除吗?')" })</td>
</tr>
}
</table>
</div>
</body>
</html>
Jia
html
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Index</title>
</head>
<body>
<div>
<a href="/Home/Jia">添加</a>
<table border="1" style=" position:absolute;top:5%;right:70%">
<tr>
<th></th>
<th>标题</th>
<th>时长</th>
<th>歌手</th>
<th></th>
</tr>
@foreach (var item in @ViewBag.Show as List<MvcApplication1.Models.HotSong>)
{
<tr>
<td>@item.Id</td>
<td>@item.Title</td>
@{
var a = item.Duration % 60;
var b = item.Duration / 60;
}
<td>@b:@a </td>
<td>@item.Singer</td>
<td>@Html.ActionLink("删除", "Delect", new { id = @item.Id }, new { onclick = "return confirm('确定删除吗?')" })</td>
</tr>
}
</table>
</div>
</body>
</html>