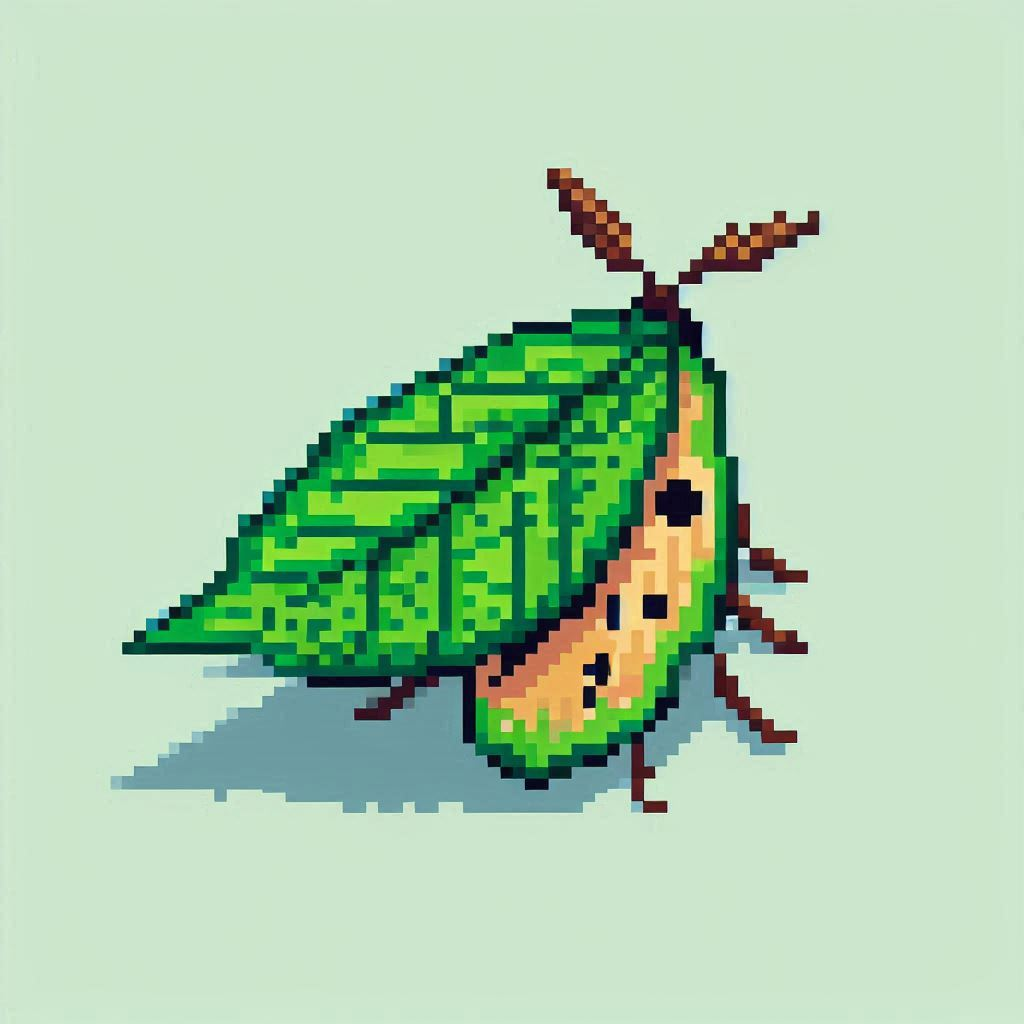
总结
如果我有:
python
# my_life_work.py
def transform(param):
return param * 2
def check(param):
return "bad" not in param
def calculate(param):
return len(param)
def main(param, option):
if option:
param = transform(param)
if not check(param):
raise ValueError("Woops")
return calculate(param)
我可以做一个集成测试,并以这种方式进行测试:
python
from my_life_work import main
def test_main():
assert main("param", False) == 5
assert main("param", True) == 10
with pytest.raises(ValueError):
main("bad_param", False)
或者,我可以使用mocks(专门用于伪造行为的对象)来创建一个独立的单元测试:
python
import pytest
from unittest.mock import patch
from my_life_work import main
@patch("my_life_work.transform")
@patch("my_life_work.check")
@patch("my_life_work.calculate")
def test_main(calculate, check, transform):
check.return_value = True
calculate.return_value = 5
assert main("param", False) == calculate.return_value
transform.assert_not_called()
check.assert_called_with("param")
calculate.assert_called_once_with("param")
transform.return_value = "paramparam"
calculate.return_value = 10
assert main("param", True) == calculate.return_value
transform.assert_called_with("param")
check.assert_called_with("paramparam")
calculate.assert_called_with("paramparam")
with pytest.raises(ValueError):
check.side_effect = ValueError
main("bad_param", False)
check.assert_called_with("param")
transform.assert_not_called()
calculate.assert_not_called()
如果你觉得花哨,我也可以使用 Mockito
来做:
python
import pytest
import my_life_work
from my_life_work import main
def test_main(expect):
expect(my_life_work, times=1).check("param").thenReturn(True)
expect(my_life_work, times=1).calculate("param").thenReturn(5)
expect(my_life_work, times=0).transform(...)
assert main("param", False) == 5
expect(my_life_work, times=1).transform("param").thenReturn("paramparam")
expect(my_life_work, times=1).check("paramparam").thenReturn(True)
expect(my_life_work, times=1).calculate("paramparam").thenReturn(10)
assert main("param", True) == 10
expect(my_life_work, times=1).check("bad_param").thenReturn(False)
expect(my_life_work, times=0).transform(...)
expect(my_life_work, times=0).calculate(...)
with pytest.raises(ValueError):
main("bad_param", False)
不要做你自己
Mock(模拟),也称为测试替身,是专用于伪造行为的对象,因此您可以编写依赖于代码其他部分的单元测试,而无需运行所述代码。
事实上,如果我有一个将行为委托给其他 3 个函数的函数:
python
def transform(param):
return param * 2
def check(param):
return "bad" not in param
def calculate(param):
return len(param)
def main(param, option):
if option:
param = transform(param)
if not check(param):
raise ValueError("Woops")
return calculate(param)
我可以通过两种方式进行测试 main() :
- 创建一个集成测试,该测试将调用函数,执行整个调用链,并获得实际值。
- 创建一个将调用该函数的单元测试,并检查它是否委托了我们期望它委托的内容。
正如我们在之前的文章中所讨论的,这两种方法都有优点和缺点,但每种方法的后果都会通过示例更清楚地显示出来。
我不会再争论如何选择哪个和哪个,但作为一个专业人士,你应该知道如何做到这两点,这样你就可以选择哪一个符合你的目标和限制。
为了实现第二种策略,我们需要Mock来伪造 transform() 和 check() calculate() 。
Mock 基础
mock可以通过两种方式使用:作为对象和作为函数。实际上,Python中的函数也是对象。
首先作为函数,你可以创建一个fake函数,然后使用你想要的参数调用,它将始终有效,并且默认返回一个新的 mock:
python
>>> from unittest.mock import Mock
>>> a_fake_function = Mock()
>>> a_fake_function()
<Mock name='mock()' id='140204477912480'>
>>> a_fake_function(1, "hello", option=True)
<Mock name='mock()' id='140204477912480'>
为了使它更有用,我们可以决定函数应该返回什么,或者它是否应该引发异常。这些虚假结果也称为"存根":
python
>>> another_one = Mock(return_value="tada !")
>>> another_one()
'tada !'
>>> a_broken_one = Mock(side_effect=TypeError('Nope'))
>>> a_broken_one()
Traceback (most recent call last):
...
TypeError: Nope
模拟也可以像对象一样使用。任何不以开头的 _ 属性访问都会返回一个mock。如果该属性是一个方法,您可以调用它,然后,您将返回一个模拟...
python
>>> from unittest.mock import Mock
>>> mocking_bird = Mock()
>>> mocking_bird.chip()
<Mock name='mock.chip()' id='140204462793264'>
>>> mocking_bird.foo(bar=1)
<Mock name='mock.foo(bar=1)' id='140204460043296'>
>>> mocking_bird.color
<Mock name='mock.color' id='140204464845008'>
>>> mocking_bird.name
<Mock name='mock.name' id='140204477913536'>
>>> mocking_bird.child.grand_child.whatever
<Mock name='mock.child.grand_child.whatever' id='140204462902480'>
但是,该 _ 限制意味着如果没有相关 dundder 方法的明确定义,则无法索引或添加模拟。为了避免这个繁琐的过程,请使用 MagicMock,而不是 Mock。大多数时候,你想要MagicMock :
python
>>> Mock()[0]
Traceback (most recent call last):
...
TypeError: 'Mock' object is not subscriptable
>>> MagicMock()[0]
<MagicMock name='mock.__getitem__()' id='140195073495472'>
您可以混合和匹配所有这些行为,因为任何未保留的 MagicMock 参数都可用于设置属性:
python
>>> reasonable_person = MagicMock(eat=Mock(return_value="chocolate"), name="Jack")
>>> reasonable_person.name
'Jack'
>>> reasonable_person.eat(yum=True)
'chocolate'
>>>
如果模拟只是一种玩虚构游戏的方式,那么它们对测试的用处只有一半,但模拟也会记录对它们的调用,因此您可以检查是否发生了某些事情:
python
>>> reasonable_person.eat.call_args_list
[call(yum=True)]
>>> reasonable_person.eat.assert_called_with(yum=True) # passes
>>> reasonable_person.eat.assert_called_with(wait="!") # doesn't pass
Traceback (most recent call last):
...
Actual: eat(yum=True)
>>> reasonable_person.this_method_doesnt_exist.assert_called()
Traceback (most recent call last):
...
AssertionError: Expected 'this_method_doesnt_exist' to have been called.
自动化这些过程
虽然您可以手动创建模拟,但有一些方便的工具可以为您完成一些繁重的工作。
您可以用 create_autospec()
自动创建一个mock,该mock将另一个对象形状匹配
python
>>> class AJollyClass:
... def __init__(self):
... self.gentlemanly_attribute = "Good day"
... self.mustache = True
>>> good_lord = create_autospec(AJollyClass(), spec_set=True)
>>> good_lord.mustache
<NonCallableMagicMock name='mock.mustache' spec_set='bool' id='131030999991728'>
>>> good_lord.other
Traceback (most recent call last):
...
AttributeError: Mock object has no attribute 'other
它也适用于函数:
python
>>> def oh_my(hat="top"):
... pass
>>> by_jove = create_autospec(oh_my, spec_set=True)
>>> by_jove(hat=1)
<MagicMock name='mock()' id='131030900955296'>
>>> by_jove(cat=1)
Traceback (most recent call last):
...
TypeError: got an unexpected keyword argument 'cat'
最后,当你想暂时用模拟交换一个真实对象时,你可以使用 patch()。
python
>>> import requests
>>> from unittest.mock import patch
>>> with patch('__main__.requests'):
... requests.get('http://bitecode.dev')
...
<MagicMock name='requests.get()' id='140195072736224'>
这将在with块中将requests 替换为 mock。
patch()使用装饰器模式@patch('module1.function1')
使用,如果您使用 pytest,这将非常方便,我们将在下面看到。它甚至可以用来替换字典或对象的一部分
patch() 使用起来有点棘手,因为您必须传递一个字符串来表示要替换的内容的虚线路径,但它必须位于使用事物的位置,而不是定义事物的位置。因此,__main__
这里是因为我修补了我在自己的模块中使用的请求。
困惑?
想象一下,在client.py中有一个函数:
python
import requests
def get_data():
return requests.get(...)
如果我在测试中用patch,我不该:
python
with patch('requests'):
get_data()
而应该:
python
with patch('client.requests'):
get_data()
因为我想修补该特定文件中的 requests 引用,而不是一般的。
从集成测试到单元测试
让我们回到我们的 main() 函数:
python
def main(param, option):
if option:
param = transform(param)
if not check(param):
raise ValueError('Woops')
return calculate(param)
如果我必须进行集成测试,我会这样做:
python
from my_life_work import main
def test_main():
assert main("param", False) == 5
assert main("param", True) == 10
with pytest.raises(ValueError):
main("bad_param", False)
如果我想把它变成一个单元测试,那么我会使用mock:
python
import pytest
from unittest.mock import patch
from my_life_work import main
# Careful! The order of patch is the reverse of the order of the params
@patch("my_life_work.transform")
@patch("my_life_work.check")
@patch("my_life_work.calculate")
def test_main(calculate, check, transform):
check.return_value = True
calculate.return_value = 5
# We check that:
# - transform() is not called if option is False
# - check() verifies that the parameter is ok
# - calculate() is called, its return value is the output of main()
assert main("param", False) == calculate.return_value
transform.assert_not_called()
check.assert_called_with("param")
calculate.assert_called_once_with("param")
# Same thing, but transform() should be called, and hence check()
# should receive the transformed result
transform.return_value = "paramparam"
calculate.return_value = 10
assert main("param", True) == calculate.return_value
transform.assert_called_with("param")
check.assert_called_with("paramparam")
calculate.assert_called_with("paramparam")
# We check that if the check fails, that raises the expected error
# an nothing else is called.
with pytest.raises(ValueError):
check.side_effect = ValueError
main("bad_param", False)
check.assert_called_with("param")
transform.assert_not_called()
calculate.assert_not_called()
现在测试是隔离的,快速的,但检查我们的代码是否满足它所依赖的所有函数的约定。
它也很冗长,而且更复杂。
我将写一篇完整的文章来解释为什么你可能想要这样做,何时以及如何这样做。因为我明白,如果你第一次看这种类型的测试,你为什么会对自己而不是以前的版本施加这个并不明显。
模拟的另一个问题是,如果键入错误的属性名称,则不会收到错误。
犯错误很容易,但当你犯错时,很难找到它们。已经设置了一系列故障保护措施,例如在拼写错误 assert_*
时提醒您或提供 create_autospec()
。
尽管如此,我认为由于模拟已经是一个相当复杂的话题,而且很难操纵,因此添加用错别字默默地搞砸一切的可能性对我的口味来说太过分了。
在某些时候,你会深入嵌套模拟中,副作用和返回值来自一些修补对象的方法,不,你不会玩得很开心。
出于这个原因,就像我鼓励你使用 pytest 而不是 unittest 一样,我建议尝试 mockito 而不是用于 unittest.mock 存根。
它有几个优点:
- 一切都是自动规范的,所以你不能错误地创建一个模拟,它接受与你替换的东西具有不同参数/属性的东西。
- 用于检查调用的 API 不在模拟本身上,因此没有拼写错误的风险。
- 提供返回值或引发错误更冗长,但也更明确和具体。
让我们 pip install pytest-mockito
看看在我们的主测试函数中使用它是什么样子的。
python
import pytest
import my_life_work # we need to import this for patching
from my_life_work import main
def test_main(expect):
# We mock my_life_work.check(), expecting it to be called once with
# "param" and we tell it to return True in that case.
# Any other configuration of calls would make the test fail.
expect(my_life_work, times=1).check("param").thenReturn(True)
expect(my_life_work, times=1).calculate("param").thenReturn(5)
# '...' is used to mean "any param". We don't expect transform()
# to be called with anything.
expect(my_life_work, times=0).transform(...)
assert main("param", False) == 5
expect(my_life_work, times=1).transform("param").thenReturn("paramparam")
expect(my_life_work, times=1).check("paramparam").thenReturn(True)
expect(my_life_work, times=1).calculate("paramparam").thenReturn(10)
assert main("param", True) == 10
expect(my_life_work, times=1).check("bad_param").thenReturn(False)
expect(my_life_work, times=0).transform(...)
expect(my_life_work, times=0).calculate(...)
with pytest.raises(ValueError):
main("bad_param", False)
这更清晰、更不容易出错、更不冗长。仍然比以下要复杂得多:
python
def test_main():
assert main("param", False) == 5
assert main("param", True) == 10
with pytest.raises(ValueError):
main("bad_param", False)
这就是为什么我之前说过,集成和 e2e 测试可以让你物有所值,至少在前期是这样。但从长远来看,它们是有代价的,即使乍一看这并不明显。
因此,在以后的文章中,我们将通过分析它将如何影响整个项目测试树,更详细地介绍为什么您可能想要选择其中一个而不是另一个。不过不是下一节,下一j节是关于伪造数据的。