图形化用户界面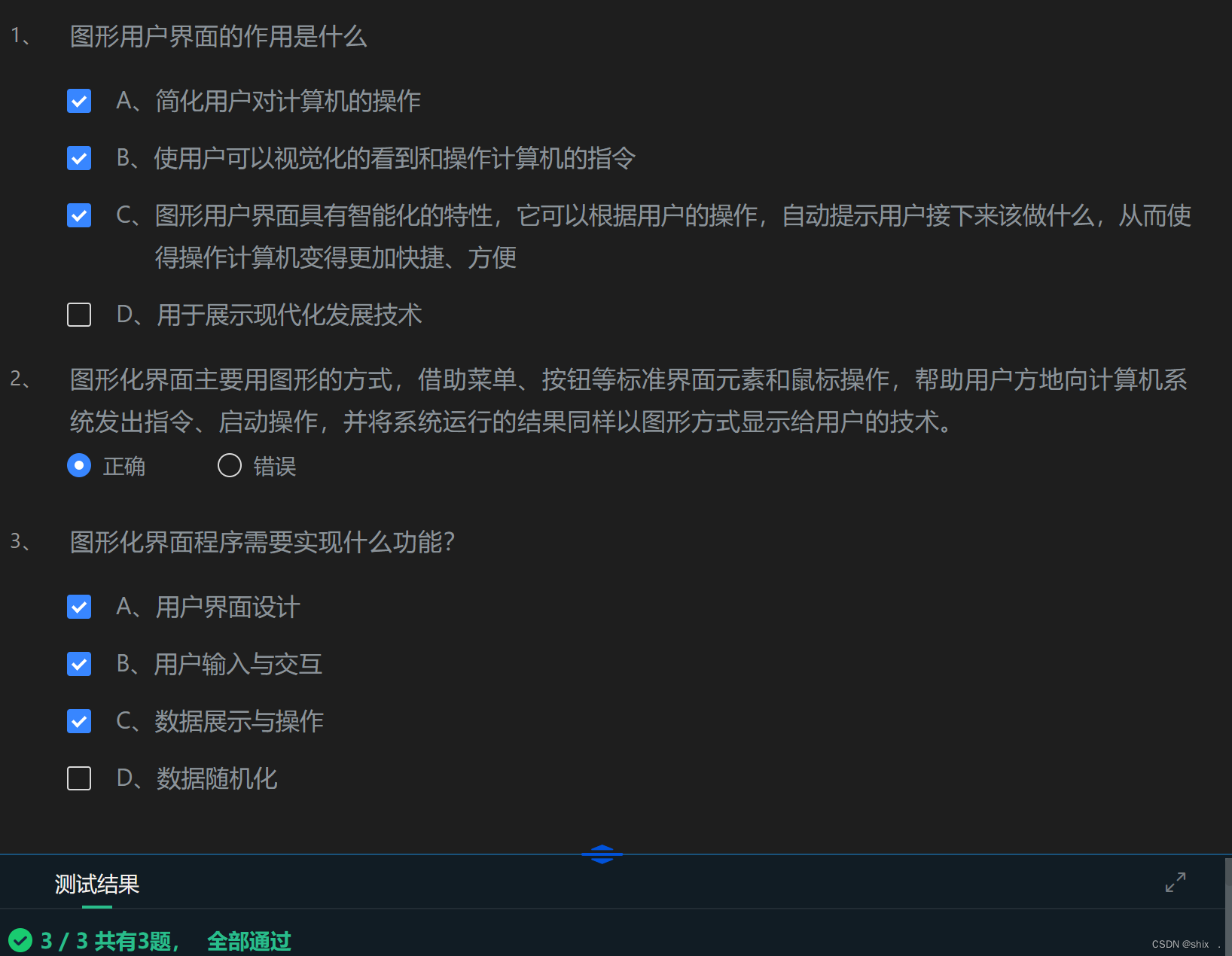
java
复制代码
import java.awt.*;
import javax.swing.*;
public class GraphicsTester extends JFrame {
public GraphicsTester() {
super("Graphics Demo");
setSize(480, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void paint(Graphics g) {
super.paint(g);
g.setFont(new Font("Serif", Font.BOLD, 12)); // 字体Serif,粗体,12号
g.setColor(Color.BLUE);
g.drawString("Hello, World!", 20, 50); // 在(20, 50)位置绘制字符串
g.setFont(new Font("Serif", Font.ITALIC, 14)); // 字体Serif,斜体,14号
g.setColor(Color.RED);
g.drawString("Graphics Demo", 250, 50); // 在(250, 50)位置绘制字符串
// 绘制直线
g.setColor(Color.GREEN);
g.drawLine(50, 100, 150, 100); // 从(50, 100)到(150, 100)绘制直线
// 绘制空心矩形
g.setColor(Color.BLACK);
g.drawRect(170, 50, 100, 50);
// 绘制实心矩形
g.setColor(Color.YELLOW);
g.fillRect(170, 120, 100, 50);
g.setColor(Color.CYAN);
g.drawRoundRect(200, 120, 100, 50, 20, 20); // 在(200, 120)位置绘制一个带圆角的空心矩形
// 绘制实心圆角矩形
g.setColor(Color.MAGENTA);
g.fillRoundRect(200, 180, 100, 50, 20, 20); // 在(200, 180)位置绘制一个带圆角的实心矩形
// 绘制标准矩形模拟3D效果(没有真正的draw3DRect和fill3DRect方法)
g.setColor(Color.LIGHT_GRAY);
g.fillRect(50, 50, 100, 50); // 底部颜色
g.setColor(Color.GRAY);
g.drawRect(50, 50, 100, 50); // 边缘颜色,模拟阴影效果
// 绘制空心椭圆
g.setColor(Color.BLUE);
g.drawOval(50, 190, 100, 50);
// 绘制实心椭圆
g.setColor(Color.GREEN);
g.fillOval(170, 190, 100, 50);
// 绘制圆弧
g.setColor(Color.RED);
g.drawArc(50, 260, 100, 50, 45, 180); // 从45度开始,画180度的圆弧
// 绘制扇形
g.setColor(Color.PINK);
g.fillArc(170, 260, 100, 50, 0, 90); // 从0度开始,画90度的扇形
// 绘制多边形
int[] xPoints = {50, 100, 100, 50};
int[] yPoints = {20, 20, 70, 70};
int nPoints = 4;
g.setColor(Color.ORANGE);
g.drawPolygon(xPoints, yPoints, nPoints); // 绘制空心多边形
g.setColor(Color.CYAN);
g.fillPolygon(xPoints, yPoints, nPoints); // 绘制实心多边形
}
public static void main(String args[]) {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
JFrame.setDefaultLookAndFeelDecorated(true);
GraphicsTester tester = new GraphicsTester();
tester.setVisible(true);
} catch (ClassNotFoundException e) {
// 如果Look and Feel类找不到,打印异常信息
e.printStackTrace();
} catch (UnsupportedLookAndFeelException e) {
// 如果Look and Feel不受支持,打印异常信息
e.printStackTrace();
} catch (InstantiationException e) {
// 如果Look and Feel实例化失败,打印异常信息
e.printStackTrace();
} catch (IllegalAccessException e) {
// 如果访问Look and Feel类受限,打印异常信息
e.printStackTrace();
}
}
}
java
复制代码
import java.awt.Color;
import java.awt.GradientPaint;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.geom.*;
import javax.swing.*;
public class test3 extends JFrame {
public test3() {
super("word"); //调用基类构造方法
setVisible(true); //显示窗口
setSize(480, 250); //设置窗口大小
System.out.println(getSize());
}
public void paint(Graphics g) {
super.paint(g);
// ########## Start ##########
Graphics2D g2d = (Graphics2D) g;
g2d.setPaint(Color.BLACK);
g2d.drawString("Hello, 3D Rectangle!", 50, 50);
// 模拟 draw3DRect
int x = 100, y = 100, width = 100, height = 50;
g2d.setColor(Color.LIGHT_GRAY);
g2d.drawRect(x, y, width, height);
g2d.setColor(Color.GRAY.darker());
g2d.drawLine(x, y + height - 1, x + width - 1, y + height - 1);
g2d.drawLine(x + width - 1, y, x + width - 1, y + height - 2);
// 模拟 fill3DRect
int fillX = 150, fillY = 150, fillWidth = 100, fillHeight = 50;
GradientPaint gp = new GradientPaint(
fillX, fillY, Color.YELLOW,
fillX, fillY + fillHeight, Color.ORANGE,
true
);
g2d.setPaint(gp);
g2d.fillRect(fillX, fillY, fillWidth, fillHeight);
g2d.setColor(Color.ORANGE.darker());
g2d.drawLine(fillX, fillY + fillHeight, fillX + fillWidth, fillY + fillHeight);
Point2D.Float p1 = new Point2D.Float(200.f, 75.f);
Point2D.Float p2 = new Point2D.Float(250.f, 75.f);
GradientPaint g1 = new GradientPaint(p1, Color.red, p2, Color.yellow, true);
Arc2D.Float arc = new Arc2D.Float(p1.x - (p2.x - p1.x) / 2, 75.f, 130.f, p2.x - p1.x, 0.f, 360.f, 2);
//绘制实心矩形
g2d.setPaint(Color.BLUE); // 设置画笔颜色为蓝色
Rectangle2D rect = new Rectangle2D.Float(100, 100, 100, 100); // 创建一个矩形对象
g2d.fill(rect); // 填充矩形
// ########## End ##########
sout(p1);
sout(p2);
}
public void sout(Point2D.Float p) {
System.out.println(p);
}
public static void main(String args[]) {
test3 application = new test3();
application.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
java
复制代码
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
public class test4 extends javax.swing.JFrame {
int index;
String input;
String choosedStr;
public test4() {
initComponents();
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jButton1 = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
textField1 = new java.awt.TextField();
jScrollPane1 = new javax.swing.JScrollPane();
jList1 = new javax.swing.JList<>();
textArea1 = new java.awt.TextArea();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jButton1.setText("确定");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jLabel1.setText("input");
// ########## Start ##########
// 设置 textField1 监听
textField1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
textField1ActionPerformed(evt);
}
});
// ########## End ##########
jList1.setModel(new javax.swing.AbstractListModel<String>() {
String[] strings = {"Item1", "Item2", "Item3", "Item4", "Item5"};
public int getSize() {
return strings.length;
}
public String getElementAt(int i) {
return strings[i];
}
});
// ########## Start ##########
// 设置 jList1 鼠标监听
jList1.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
jList1MouseClicked(evt);
}
});
// ########## End ##########
jScrollPane1.setViewportView(jList1);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(55, 55, 55)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1)
.addGap(46, 46, 46)
.addComponent(textField1, javax.swing.GroupLayout.PREFERRED_SIZE, 79, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 147, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(94, 94, 94)
.addComponent(textArea1, javax.swing.GroupLayout.PREFERRED_SIZE, 232, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addGap(85, 85, 85)
.addComponent(jButton1)))
.addContainerGap(63, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(55, 55, 55)
.addComponent(jLabel1))
.addGroup(layout.createSequentialGroup()
.addGap(45, 45, 45)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(textArea1, javax.swing.GroupLayout.PREFERRED_SIZE, 228, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createSequentialGroup()
.addComponent(textField1, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(32, 32, 32)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))))
.addGap(18, 18, 18)
.addComponent(jButton1)
.addContainerGap(146, Short.MAX_VALUE))
);
pack();
}// </editor-fold>//GEN-END:initComponents
private void textField1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_textField1ActionPerformed
input = textField1.getText();
}//GEN-LAST:event_textField1ActionPerformed
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed
String []shix={"","touge","is","nice"};
textField1.setText(shix[index]);
input = textField1.getText();//第一行输出input
textField1.setText("");
textArea1.setText(input + "\n" + "Selection is Item" + index);
System.out.println(input + "\n" + "Selection is Item" + index);
}//GEN-LAST:event_jButton1ActionPerformed
private void jList1MouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_jList1MouseClicked
index = jList1.getSelectedIndex() + 1;
}//GEN-LAST:event_jList1MouseClicked
public static void main(String args[]) {
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new test4().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton jButton1;
private javax.swing.JLabel jLabel1;
private javax.swing.JList<String> jList1;
private javax.swing.JScrollPane jScrollPane1;
private java.awt.TextArea textArea1;
private java.awt.TextField textField1;
// End of variables declaration//GEN-END:variables
}