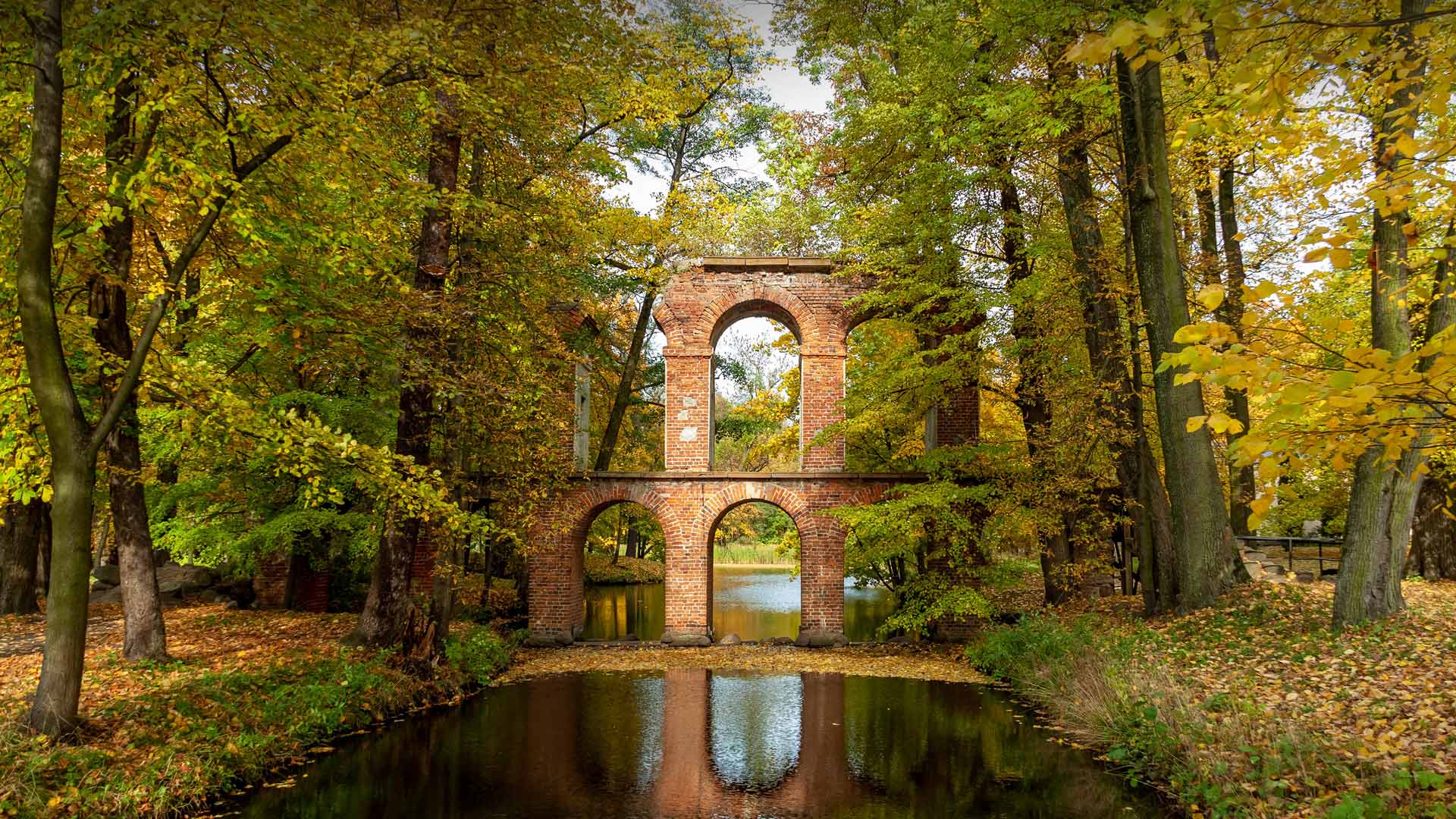
SpringBoot防抖方案(防止表单重复提交)
1.应用场景(什么是防抖)
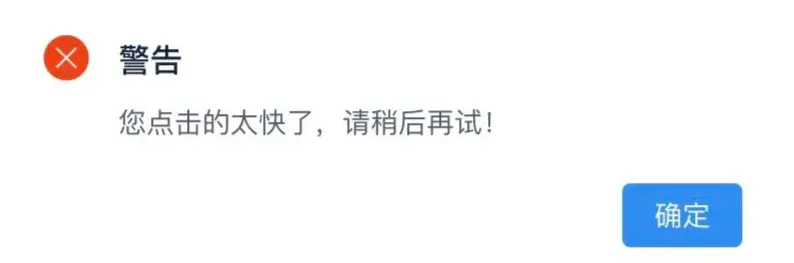
所谓防抖,一是防用户手抖,二是防网络抖动。在Web系统中,表单提交是一个非常常见的功能,如果不加控制,容易因为用户的误操作或网络延迟导致同一请求被发送多次,进而生成重复的数据记录。要针对用户的误操作,前端通常会实现按钮的loading状态,阻止用户进行多次点击。而对于网络波动造成的请求重发问题,仅靠前端是不行的。为此,后端也应实施相应的防抖逻辑,确保在网络波动的情况下不会接收并处理同一请求多次。
2.思路分析
哪一类接口需要防抖?
通常用于表单的提交,相同的参数用户连续的点击,由于网络的波动或者前端没有阻止用户进行多次的提交点击,都有可能会请求重发问题进而产生重复的数据记录。
如何确定接口是重复的?
防抖也即防重复提交,那么如何确定两次接口就是重复的呢?首先,我们需要给这两次接口的调用加一个时间间隔,大于这个时间间隔的一定不是重复提交;其次,两次请求提交的参数比对,不一定要全部参数,选择标识性强的参数即可;最后,如果想做的更好一点,还可以加一个请求地址的对比。
3.分布式部署下如何做接口防抖?
使用共享缓存
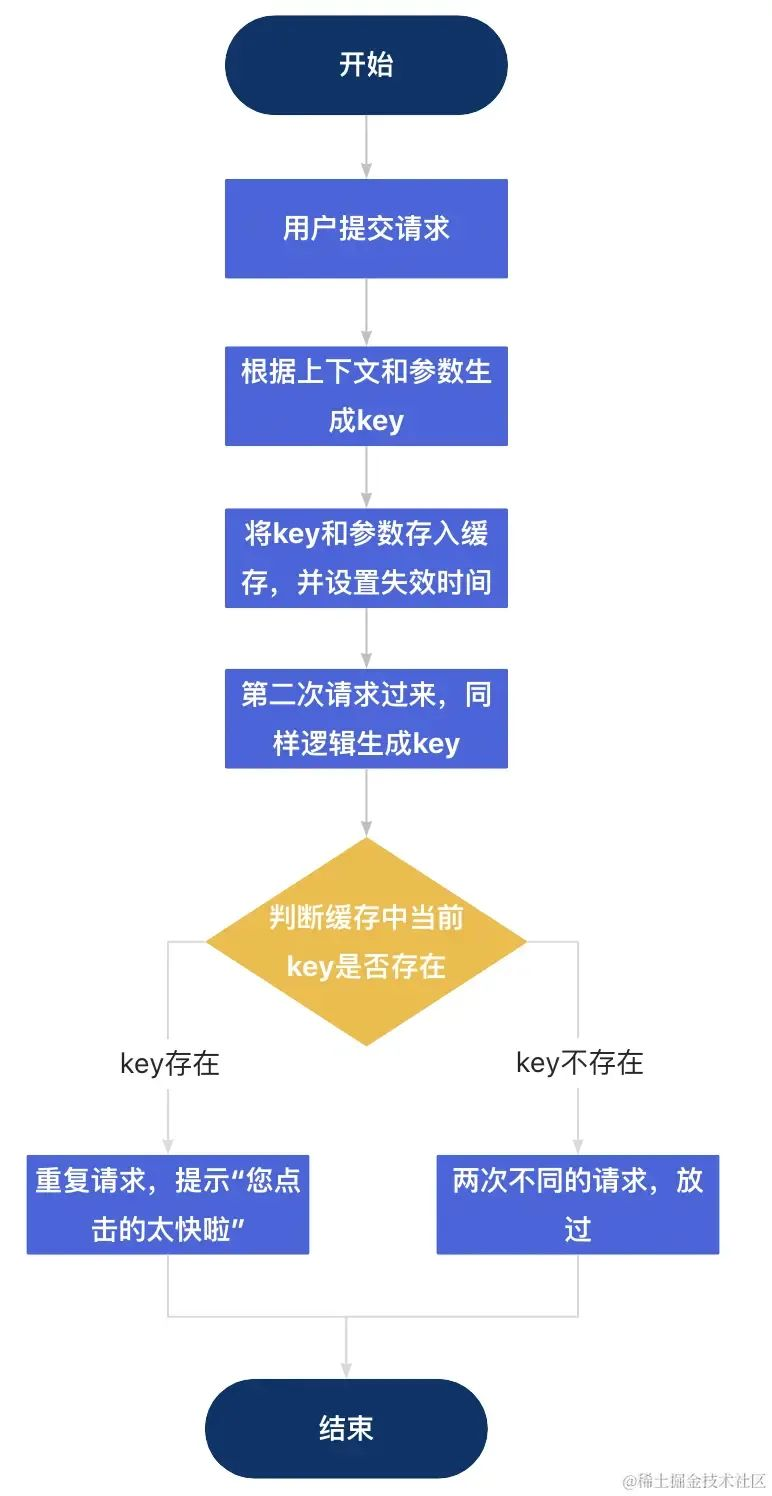
使用分布式锁

4.具体实现
4.0 准备工作
- 引入依赖
xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- spring2.X 集成 redis 所需 common-pool-->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<!--不要带版本号,防止冲突-->
</dependency>
<!--redisson-->
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson-spring-boot-starter</artifactId>
<version>3.10.6</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
</dependencies>
- yaml配置
yaml
spring:
redis:
host: 127.0.0.1
port: 6379
# password: llp123
#Redis 数据库索引(默认为 0)
database: 0
#连接超时时间(毫秒)
timeout: 1800000
lettuce:
pool:
#连接池最大连接数(使用负值表示没有限制)
max-active: 20
#最大阻塞等待时间(负数表示没限制)
max-wait: -1
#连接池中的最大空闲连接
min-idle: 0
- 启动类
java
@SpringBootApplication
public class RepeatSubmitApplication {
public static void main(String[] args) {
SpringApplication.run(RepeatSubmitApplication.class, args);
}
}
4.1定义生成key的注解
首先我们需要定义一个用于生成key的注解,timeUnit是时间的单位,expire用于指定锁过期的时间,prefix用于指定业务前缀可以使用枚举或者常量来定义,delimiter参数之前的分隔符
示例:
repeat-submit&AddRequestVO(userName=zhangsan, userPhone=157, roleIdList=[1, 2, 4])
repeat-submit&zhangsan&157
java
@Target({ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
public @interface RequestLock {
TimeUnit timeUnit() default TimeUnit.SECONDS; // 时间单位,默认为秒
long expire() default 3; // 锁过期时间,默认为3s
String prefix() default "";
String delimiter() default "&";
}
@RequestKeyParam注解用于指定生成key的参数
- 修饰方法的参数则方法参数所有的值会拼接作为key,repeat-submit&AddRequestVO(userName=zhangsan, userPhone=157, roleIdList=[1, 2, 4])
- 修饰字段则字段参数的值作为key, repeat-submit&zhangsan&157
java
/**
* @description 加上这个注解可以将参数设置为key
*/
@Target({ElementType.METHOD, ElementType.PARAMETER, ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
public @interface RequestKeyParam {
}
4.2 核心代码
- 枚举
java
@AllArgsConstructor
@Getter
public enum ResponseCodeEnum {
BIZ_CHECK_FAIL(500),
;
private Integer code;
}
- 异常处理
java
@Data
@NoArgsConstructor
@EqualsAndHashCode(callSuper = true)
public class BizException extends RuntimeException {
/**
* 业务错误码
*
*/
private Integer code;
/**
* 错误提示
*/
private String message;
public BizException(ResponseCodeEnum responseCodeEnum, String message){
this.code = responseCodeEnum.getCode();
this.message = message;
}
}
java
@RestControllerAdvice
@AllArgsConstructor
@Slf4j
public class GlobalExceptionHandler {
/**
* 业务异常
*/
@ExceptionHandler(value = BizException.class)
public Result BizException(BizException ex) {
return Result.error(String.valueOf(BAD_REQUEST.value()), ex.getMessage());
}
}
- 统一返回
java
@Data
public class Result<T> {
private String code;
private String msg;
private T data;
public Result() {
}
public Result(T data) {
this.data = data;
}
/**
* 表示成功的Result,不携带返回数据
*
* @return
*/
public static Result success() {
Result result = new Result();
result.setCode("200");
result.setMsg("success");
return result;
}
/**
* 便是成功的Result,携带返回数据
* 如果需要在static方法使用泛型,需要在static后指定泛型表示 static<T>
*
* @param data
* @return
*/
public static <T> Result<T> success(T data) {
Result<T> result = new Result<>(data);
result.setCode("200");
result.setMsg("success");
return result;
}
/**
* 失败不携带数据
* 将错误的code、msg作为形参,灵活传入
*
* @param code
* @param msg
* @return
*/
public static Result error(String code, String msg) {
Result result = new Result();
result.setCode(code);
result.setMsg(msg);
return result;
}
/**
* 失败携带数据
* 将错误的code、msg、data作为形参,灵活传入
* @param code
* @param msg
* @param data
* @param <T>
* @return
*/
public static <T> Result<T> error(String code, String msg, T data) {
Result<T> result = new Result<>(data);
result.setCode(code);
result.setMsg(msg);
return result;
}
}
- redis配置
java
@EnableCaching
@Configuration
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) {
RedisTemplate<String, Object> template =
new RedisTemplate<>();
System.out.println("template=>" + template);//这里可以验证..
RedisSerializer<String> stringRedisSerializer =
new StringRedisSerializer();
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer =
new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.activateDefaultTyping(
LaissezFaireSubTypeValidator.instance,
ObjectMapper.DefaultTyping.NON_FINAL,
JsonTypeInfo.As.WRAPPER_ARRAY);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setConnectionFactory(factory);
//key序列化方式
template.setKeySerializer(stringRedisSerializer);
//value序列化
template.setValueSerializer(jackson2JsonRedisSerializer);
//value hashmap序列化
template.setHashValueSerializer(jackson2JsonRedisSerializer);
return template;
}
@Bean
public CacheManager cacheManager(RedisConnectionFactory factory) {
RedisSerializer<String> stringRedisSerializer =
new StringRedisSerializer();
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
//解决查询缓存转换异常的问题
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.activateDefaultTyping(
LaissezFaireSubTypeValidator.instance,
ObjectMapper.DefaultTyping.NON_FINAL,
JsonTypeInfo.As.WRAPPER_ARRAY);
jackson2JsonRedisSerializer.setObjectMapper(om);
// 配置序列化(解决乱码的问题),过期时间600秒
RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofSeconds(600))
.serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(stringRedisSerializer))
.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(jackson2JsonRedisSerializer))
.disableCachingNullValues();
RedisCacheManager cacheManager = RedisCacheManager.builder(factory)
.cacheDefaults(config)
.build();
return cacheManager;
}
}
- redisson配置
java
@Configuration
public class RedissonConfig {
@Bean
public RedissonClient redissonClient() {
Config config = new Config();
// 这里假设你使用单节点的Redis服务器
config.useSingleServer()
// 使用与Spring Data Redis相同的地址
.setAddress("redis://127.0.0.1:6379")
// 如果有密码
//.setPassword("xxxx");
// 其他配置参数
.setDatabase(0)
.setConnectionPoolSize(10)
.setConnectionMinimumIdleSize(2);
// 创建RedissonClient实例
return Redisson.create(config);
}
}
- 从缓存中获取key值
java
public class RequestKeyGenerator {
/**
* 获取LockKey
*
* @param joinPoint 切入点
* @return
*/
public static String getLockKey(ProceedingJoinPoint joinPoint) {
// 获取连接点的方法签名对象
MethodSignature methodSignature = (MethodSignature) joinPoint.getSignature();
// Method对象
Method method = methodSignature.getMethod();
// 获取Method对象上的注解对象
RequestLock requestLock = method.getAnnotation(RequestLock.class);
// 获取方法参数
final Object[] args = joinPoint.getArgs();
// 获取Method对象上所有的注解
final Parameter[] parameters = method.getParameters();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < parameters.length; i++) {
final RequestKeyParam keyParam = parameters[i].getAnnotation(RequestKeyParam.class);
// 如果属性不是RequestKeyParam注解,则不处理
if (keyParam == null) {
continue;
}
// 如果属性是RequestKeyParam注解,则拼接 连接符 "& + RequestKeyParam"
sb.append(requestLock.delimiter()).append(args[i]);
}
// 如果方法上没有加RequestKeyParam注解
if (StringUtils.isEmpty(sb.toString())) {
// 获取方法上的多个注解(为什么是两层数组:因为第二层数组是只有一个元素的数组)
final Annotation[][] parameterAnnotations = method.getParameterAnnotations();
// 循环注解
for (int i = 0; i < parameterAnnotations.length; i++) {
final Object object = args[i];
// 获取注解类中所有的属性字段
final Field[] fields = object.getClass().getDeclaredFields();
for (Field field : fields) {
// 判断字段上是否有RequestKeyParam注解
final RequestKeyParam annotation = field.getAnnotation(RequestKeyParam.class);
// 如果没有,跳过
if (annotation == null) {
continue;
}
// 如果有,设置Accessible为true(为true时可以使用反射访问私有变量,否则不能访问私有变量)
field.setAccessible(true);
// 如果属性是RequestKeyParam注解,则拼接 连接符" & + RequestKeyParam"
sb.append(requestLock.delimiter()).append(ReflectionUtils.getField(field, object));
}
}
}
// 返回指定前缀的key
return requestLock.prefix() + sb;
}
}
- redis方式实现
java
import com.llp.repeatsubmit.annotaion.RequestLock;
import com.llp.repeatsubmit.constant.ResponseCodeEnum;
import com.llp.repeatsubmit.exception.BizException;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.annotation.Order;
import org.springframework.data.redis.connection.RedisStringCommands;
import org.springframework.data.redis.core.RedisCallback;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.types.Expiration;
import org.springframework.util.StringUtils;
import java.lang.reflect.Method;
/**
* @description 缓存实现
*/
@Aspect
@Configuration
@Order(2)
public class RedisRequestLockAspect {
private final StringRedisTemplate stringRedisTemplate;
@Autowired
public RedisRequestLockAspect(StringRedisTemplate stringRedisTemplate) {
this.stringRedisTemplate = stringRedisTemplate;
}
@Around("execution(public * * (..)) && @annotation(com.llp.repeatsubmit.annotaion.RequestLock)")
public Object interceptor(ProceedingJoinPoint joinPoint) {
MethodSignature methodSignature = (MethodSignature)joinPoint.getSignature();
Method method = methodSignature.getMethod();
RequestLock requestLock = method.getAnnotation(RequestLock.class);
if (StringUtils.isEmpty(requestLock.prefix())) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "重复提交前缀不能为空");
}
// 获取自定义key
final String lockKey = RequestKeyGenerator.getLockKey(joinPoint);
// 使用RedisCallback接口执行set命令,设置锁键;设置额外选项:过期时间和SET_IF_ABSENT选项
// SET_IF_ABSENT是 RedisStringCommands.SetOption 枚举类中的一个选项,
// 用于在执行 SET 命令时设置键值对的时候,如果键不存在则进行设置,如果键已经存在,则不进行设置。
final Boolean success = stringRedisTemplate.execute(
(RedisCallback<Boolean>) connection -> connection.set(lockKey.getBytes(), new byte[0],
Expiration.from(requestLock.expire(), requestLock.timeUnit()),
RedisStringCommands.SetOption.SET_IF_ABSENT));
if (!success) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "您的操作太快了,请稍后重试");
}
try {
return joinPoint.proceed();
} catch (Throwable throwable) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "系统异常");
}
}
}
-
redisson方式实现
- 校验key值是否存在,如果存在且尚未过期则为重复提交
- 如果key值不存在或者已过期,则放行
java
import com.llp.repeatsubmit.annotaion.RequestLock;
import com.llp.repeatsubmit.constant.ResponseCodeEnum;
import com.llp.repeatsubmit.exception.BizException;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.reflect.MethodSignature;
import org.redisson.api.RLock;
import org.redisson.api.RedissonClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.annotation.Order;
import org.springframework.util.StringUtils;
import java.lang.reflect.Method;
/**
* @description 分布式锁实现
*/
@Aspect
@Configuration
@Order(2)
public class RedissonRequestLockAspect {
private RedissonClient redissonClient;
@Autowired
public RedissonRequestLockAspect(RedissonClient redissonClient) {
this.redissonClient = redissonClient;
}
@Around("execution(public * * (..)) && @annotation(com.llp.repeatsubmit.annotaion.RequestLock)")
public Object interceptor(ProceedingJoinPoint joinPoint) {
MethodSignature methodSignature = (MethodSignature)joinPoint.getSignature();
Method method = methodSignature.getMethod();
RequestLock requestLock = method.getAnnotation(RequestLock.class);
if (StringUtils.isEmpty(requestLock.prefix())) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "重复提交前缀不能为空");
}
// 获取自定义key
final String lockKey = RequestKeyGenerator.getLockKey(joinPoint);
// 使用Redisson分布式锁的方式判断是否重复提交
RLock lock = redissonClient.getLock(lockKey);
boolean isLocked = false;
try {
// 尝试抢占锁
isLocked = lock.tryLock();
// 没有拿到锁说明已经有了请求了
if (!isLocked) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "您的操作太快了,请稍后重试");
}
// 拿到锁后设置过期时间
lock.lock(requestLock.expire(), requestLock.timeUnit());
try {
return joinPoint.proceed();
} catch (Throwable throwable) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "系统异常");
}
} catch (Exception e) {
throw new BizException(ResponseCodeEnum.BIZ_CHECK_FAIL, "您的操作太快了,请稍后重试");
} finally {
// 释放锁
if (isLocked && lock.isHeldByCurrentThread()) {
lock.unlock();
}
}
}
}
4.3测试
java
@RestController
@RequiredArgsConstructor
public class RepeatSubmitController {
private final UserService userService;
@PostMapping("/add")
@RequestLock(prefix = "repeat-submit")
/**
*@RequestKeyParam修饰方法时,表示方法的所有参数拼接作为key repeat-submit&AddRequestVO(userName=zhangsan, userPhone=157, roleIdList=[1, 2, 4])
* @RequestKeyParam修饰字段时,表示该字段的值作为key, repeat-submit&zhangsan&157
* 如果key存在且为超过过期时间则会拒绝重复提交
*/
public Result add(@RequestBody @RequestKeyParam AddRequestVO add) {
userService.add(add);
return Result.success();
}
}
java
public interface UserService {
void add(AddRequestVO add);
}
java
@Service
public class UserServiceImpl implements UserService {
@Override
public void add(AddRequestVO add) {
}
}
- 正常请求
请求参数
json
{
"userName": "zhangsan",
"userPhone": "157",
"roleIdList":[1,2,4]
}
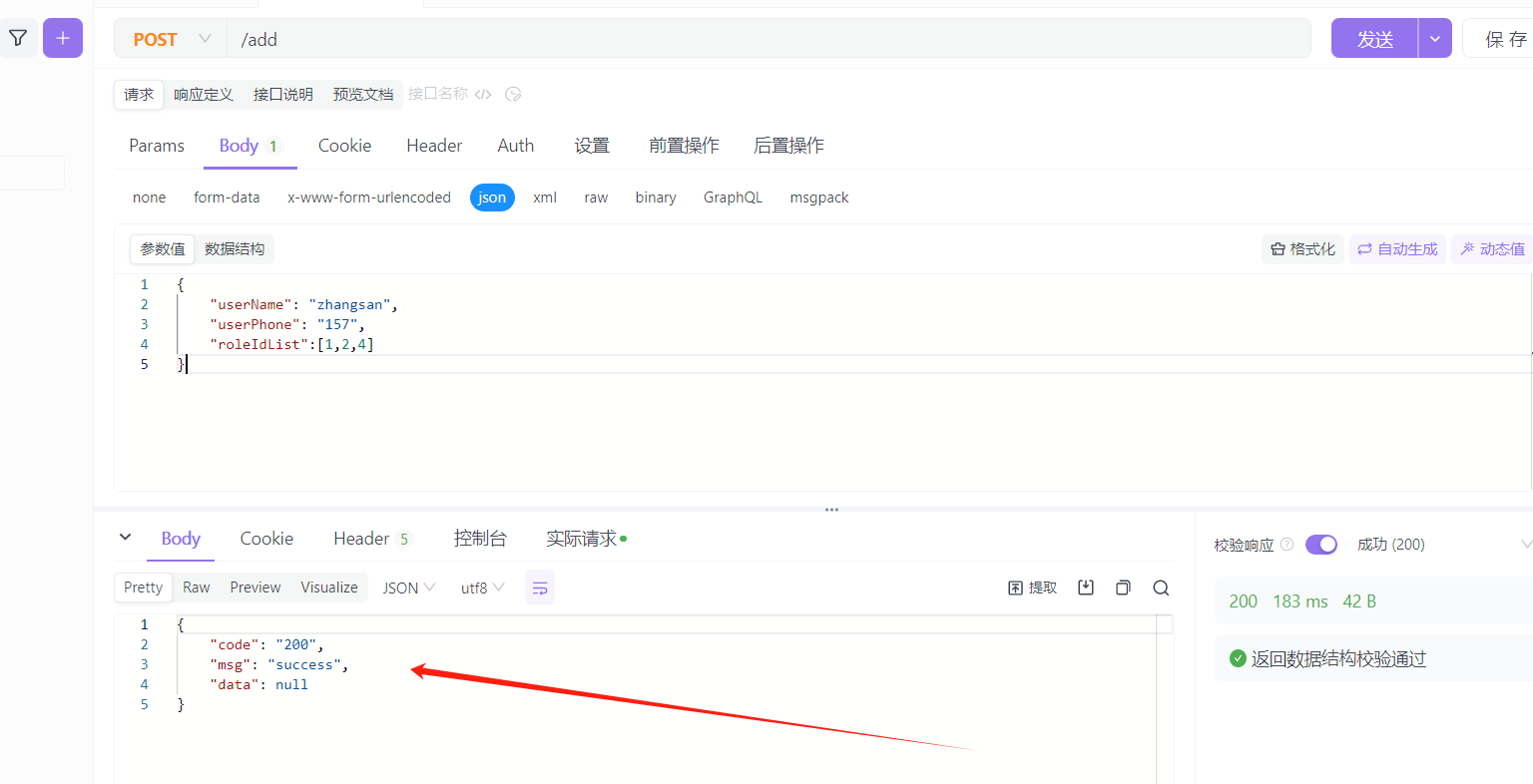
- 触发防抖
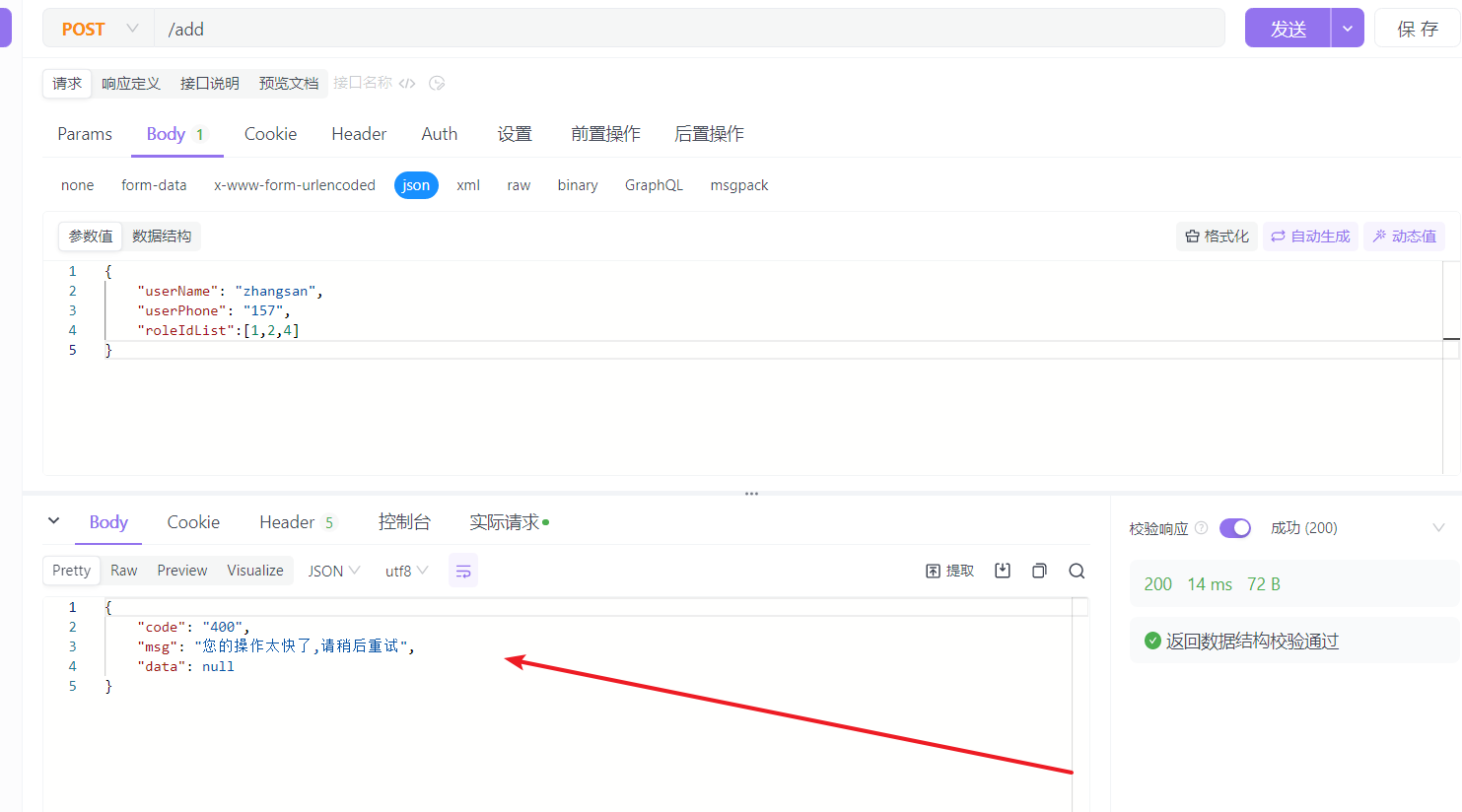