CMSIS 2.0接口中的Mutex(互斥锁)是用于在多线程环境中保护共享资源的访问机制。Mutex(互斥锁)是一种特殊的信号量,用于确保同一时间只有一个线程可以访问特定的共享资源。 在嵌入式系统或多线程应用中,当多个线程需要访问同一资源时,如果没有适当的同步机制,可能会导致数据不一致或其他问题。Mutex就是用来解决这类问题的。这些函数通常不能在中断服务程序(ISR)中调用,因为中断的上下文可能与这些函数的设计不符。 在使用Mutex时,必须确保正确地获取和释放锁,以避免死锁或其他同步问题。
Mutex API
|-----------------|------------------------------|
| API名称 | 说明 |
| osMutexNew | 创建并初始化一个互斥锁 |
| osMutexGetName | 获得指定互斥锁的名字 |
| osMutexAcquire | 获得指定的互斥锁的访问权限,若互斥锁已经被锁,则返回超时 |
| osMutexRelease | 释放指定的互斥锁 |
| osMutexGetOwner | 获得指定互斥锁的所有者线程 |
| osMutexDelete | 删除指定的互斥锁 |
代码编写
修改D:\DevEcoProjects\test\src\vendor\rtplay\rt_hi3861\demo\BUILD.gn文件
# Copyright (c) 2023 Beijing HuaQing YuanJian Education Technology Co., Ltd
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import("//build/lite/config/component/lite_component.gni")
lite_component("demo") {
features = [
#"base_00_helloworld:base_helloworld_example",
#"base_01_led:base_led_example",
#"base_02_loopkey:base_loopkey_example",
#"base_03_irqkey:base_irqkey_example",
#"base_04_adc:base_adc_example",
#"base_05_pwm:base_pwm_example",
#"base_06_ssd1306:base_ssd1306_example",
#"kernel_01_task:kernel_task_example",
#"kernel_02_timer:kernel_timer_example",
#"kernel_03_event:kernel_event_example",
"kernel_04_mutex:kernel_mutex_example",
]
}
创建D:\DevEcoProjects\test\src\vendor\rtplay\rt_hi3861\demo\kernel_04_mutex文件夹
文件夹中创建D:\DevEcoProjects\test\src\vendor\rtplay\rt_hi3861\demo\kernel_04_mutex\kernel_mutex_example.c文件D:\DevEcoProjects\test\src\vendor\rtplay\rt_hi3861\demo\kernel_04_mutex\BUILD.gn文件
# Copyright (c) 2023 Beijing HuaQing YuanJian Education Technology Co., Ltd
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
static_library("kernel_mutex_example") {
sources = [
"kernel_mutex_example.c"
]
include_dirs = [
"//utils/native/lite/include",
"//kernel/liteos_m/kal/cmsis",
]
}
/*
* Copyright (c) 2023 Beijing HuaQing YuanJian Education Technology Co., Ltd
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
#include <stdio.h>
#include <unistd.h>
#include "ohos_init.h"
#include "cmsis_os2.h"
osThreadId_t Task1_ID; // 任务1 ID
osThreadId_t Task2_ID; // 任务2 ID
osMutexId_t Mutex_ID; // 定义互斥锁 ID
uint8_t buff[20] = {0}; // 定义一个共享资源
#define TASK_STACK_SIZE 1024
#define TASK_DELAY_TIME 1 // s
/**
* @description: 任务1
* @param {*}
* @return {*}
*/
void Task1(void)
{
int i = 0;
printf("enter Task 1.......\n");
while (1) {
osMutexAcquire(Mutex_ID, osWaitForever); // 请求互斥锁
// 操作共享数据 写数据
printf("write Buff Data: \n");
for (i = 0; i < sizeof(buff); i++) {
buff[i] = i;
}
printf("\n");
osMutexRelease(Mutex_ID); // 释放互斥锁
sleep(TASK_DELAY_TIME);
}
}
/**
* @description: 任务2
* @param {*}
* @return {*}
*/
void Task2(void)
{
int i = 0;
printf("enter Task 2.......\n");
while (1) {
osMutexAcquire(Mutex_ID, osWaitForever); // 请求互斥锁
// 操作共享数据 读数据
printf("read Buff Data: \n");
for (i = 0; i < sizeof(buff); i++) {
printf("%d \n", buff[i]);
}
printf("\n");
osMutexRelease(Mutex_ID); // 释放互斥锁
sleep(TASK_DELAY_TIME);
}
}
/**
* @description: 初始化并创建任务
* @param {*}
* @return {*}
*/
static void kernel_mutex_example(void)
{
printf("Enter kernel_mutex_example()!\n");
// 创建互斥锁
Mutex_ID = osMutexNew(NULL);
if (Mutex_ID != NULL) {
printf("ID = %d, Create Mutex_ID is OK!\n", Mutex_ID);
}
osThreadAttr_t taskOptions;
taskOptions.name = "Task1"; // 任务的名字
taskOptions.attr_bits = 0; // 属性位
taskOptions.cb_mem = NULL; // 堆空间地址
taskOptions.cb_size = 0; // 堆空间大小
taskOptions.stack_mem = NULL; // 栈空间地址
taskOptions.stack_size = TASK_STACK_SIZE; // 栈空间大小 单位:字节
taskOptions.priority = osPriorityNormal; // 任务的优先级
Task1_ID = osThreadNew((osThreadFunc_t)Task1, NULL, &taskOptions); // 创建任务1
if (Task1_ID != NULL) {
printf("ID = %d, Create Task1_ID is OK!\n", Task1_ID);
}
taskOptions.name = "Task2"; // 任务的名字
taskOptions.priority = osPriorityNormal; // 任务的优先级
Task2_ID = osThreadNew((osThreadFunc_t)Task2, NULL, &taskOptions); // 创建任务2
if (Task2_ID != NULL) {
printf("ID = %d, Create Task2_ID is OK!\n", Task2_ID);
}
}
SYS_RUN(kernel_mutex_example);
使用build,编译成功后,使用upload进行烧录。
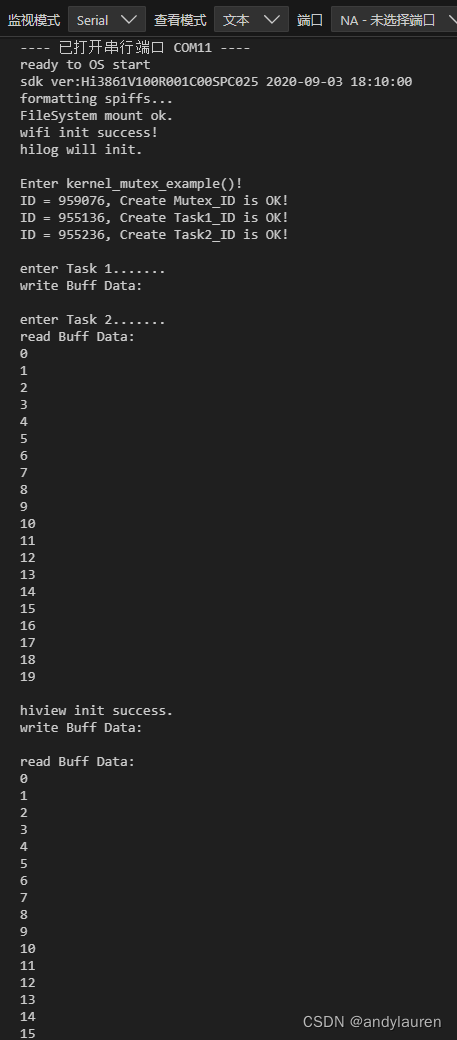