在 C++ 中,可以将复杂的结构体保存到二进制文件中,并从二进制文件中读取它。为了实现这一点,你可以使用文件流库 <fstream>
。以下是一个示例,展示如何将一个复杂的结构体保存到二进制文件中,并从二进制文件中读取它。
1. 示例结构体
假设我们有一个复杂的结构体 Person
,其中包含一些基本数据类型和字符串。
cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cstring>
struct Person {
char name[50];
int age;
double height;
std::vector<int> scores;
// Method to serialize the structure to a binary stream
void serialize(std::ofstream& ofs) const {
ofs.write(name, sizeof(name));
ofs.write(reinterpret_cast<const char*>(&age), sizeof(age));
ofs.write(reinterpret_cast<const char*>(&height), sizeof(height));
size_t scores_size = scores.size();
ofs.write(reinterpret_cast<const char*>(&scores_size), sizeof(scores_size));
ofs.write(reinterpret_cast<const char*>(scores.data()), scores.size() * sizeof(int));
}
// Method to deserialize the structure from a binary stream
void deserialize(std::ifstream& ifs) {
ifs.read(name, sizeof(name));
ifs.read(reinterpret_cast<char*>(&age), sizeof(age));
ifs.read(reinterpret_cast<char*>(&height), sizeof(height));
size_t scores_size;
ifs.read(reinterpret_cast<char*>(&scores_size), sizeof(scores_size));
scores.resize(scores_size);
ifs.read(reinterpret_cast<char*>(scores.data()), scores_size * sizeof(int));
}
};
保存结构体到二进制文件
cpp
void savePersonToFile(const Person& person, const std::string& filename) {
std::ofstream ofs(filename, std::ios::binary);
if (!ofs) {
std::cerr << "Error opening file for writing: " << filename << std::endl;
return;
}
person.serialize(ofs);
ofs.close();
}
从二进制文件读取结构体
cpp
Person loadPersonFromFile(const std::string& filename) {
Person person;
std::ifstream ifs(filename, std::ios::binary);
if (!ifs) {
std::cerr << "Error opening file for reading: " << filename << std::endl;
return person;
}
person.deserialize(ifs);
ifs.close();
return person;
}
示例用法
cpp
int main() {
Person person;
std::strcpy(person.name, "John Doe");
person.age = 30;
person.height = 5.9;
person.scores = {85, 90, 78};
std::string filename = "person.dat";
// Save to binary file
savePersonToFile(person, filename);
// Load from binary file
Person loaded_person = loadPersonFromFile(filename);
// Display loaded person information
std::cout << "Name: " << loaded_person.name << std::endl;
std::cout << "Age: " << loaded_person.age << std::endl;
std::cout << "Height: " << loaded_person.height << std::endl;
std::cout << "Scores: ";
for (int score : loaded_person.scores) {
std::cout << score << " ";
}
std::cout << std::endl;
return 0;
}
解释
-
序列化和反序列化方法:
serialize
方法将结构体的各个成员写入到二进制文件中。deserialize
方法从二进制文件中读取结构体的各个成员。
-
文件流:
- 使用
std::ofstream
打开文件进行写操作,并设置为二进制模式(std::ios::binary
)。 - 使用
std::ifstream
打开文件进行读操作,并设置为二进制模式(std::ios::binary
)。
- 使用
-
字符串和动态数组的处理:
std::vector
的大小和数据都需要序列化和反序列化,因为它是一个动态数组。char[]
字符数组可以直接写入和读取。
通过上述方法,你可以将复杂的结构体保存到二进制文件中,并从二进制文件中读取它。这种方法可以确保结构体的数据被正确保存和恢复。
2. 运行结果
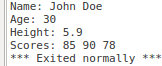