题目
- 原题连接:19. 删除链表的倒数第 N 个结点
1- 思路
模式识别:删除倒数第 n 个结点 ------> 定义 dummyHead 并用双指针实现删除逻辑
2- 实现
⭐19. 删除链表的倒数第 N 个结点------题解思路
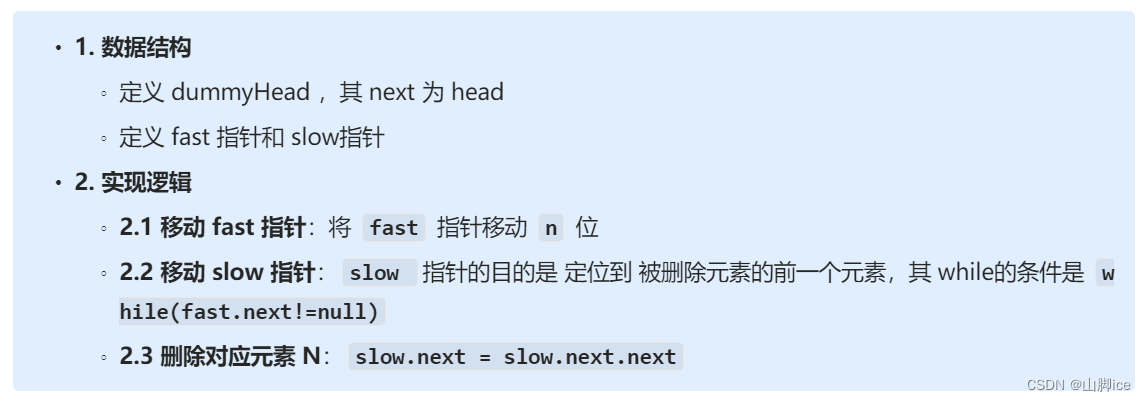
java
class Solution {
public ListNode removeNthFromEnd(ListNode head, int n) {
if(head==null){
return head;
}
ListNode dummyHead = new ListNode(-1);
dummyHead.next = head;
ListNode fast = dummyHead;
ListNode slow = dummyHead;
for(int i = 0 ; i < n ; i++){
fast = fast.next;
}
while(fast.next!=null){
slow = slow.next;
fast = fast.next;
}
slow.next = slow.next.next;
return dummyHead.next;
}
}
3- ACM实现
java
public class removeLastN {
static class ListNode{
int val;
ListNode next;
ListNode(){}
ListNode(int x){
val = x;
}
}
public static ListNode removeLast(ListNode head,int n ){
// 双指针
ListNode dummyHead = new ListNode(-1);
dummyHead.next = head;
ListNode slow = dummyHead;
ListNode fast = dummyHead;
for(int i = 0 ; i < n ;i++){
fast = fast.next;
}
while(fast.next!=null){
slow = slow.next;
fast = fast.next;
}
slow.next = slow.next.next;
return dummyHead.next;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("输入链表长度");
int n = sc.nextInt();
System.out.println("输入链表元素");
ListNode head = null,tail = null;
for(int i = 0 ; i < n;i++){
ListNode newNode = new ListNode(sc.nextInt());
if(head==null){
head = newNode;
tail = newNode;
}else{
tail.next = newNode;
tail = newNode;
}
}
System.out.println("输入删除倒数第几个元素");
int k = sc.nextInt();
ListNode Res = removeLast(head,k);
while(Res!=null){
System.out.print(Res.val+" ");
Res = Res.next;
}
}
}