目录
[2.1 python环境](#2.1 python环境)
[2.2 Visual Studio Code编译](#2.2 Visual Studio Code编译)
[3.1 代码构思](#3.1 代码构思)
[3.2 代码实例](#3.2 代码实例)
[3.3 运行结果](#3.3 运行结果)
1.认识Python
Python 是一个高层次的结合了解释性、编译性、互动性和面向对象的脚本语言。
Python 的设计具有很强的可读性,相比其他语言经常使用英文关键字或标点符号,它具有比其他语言更有特色的语法结构。
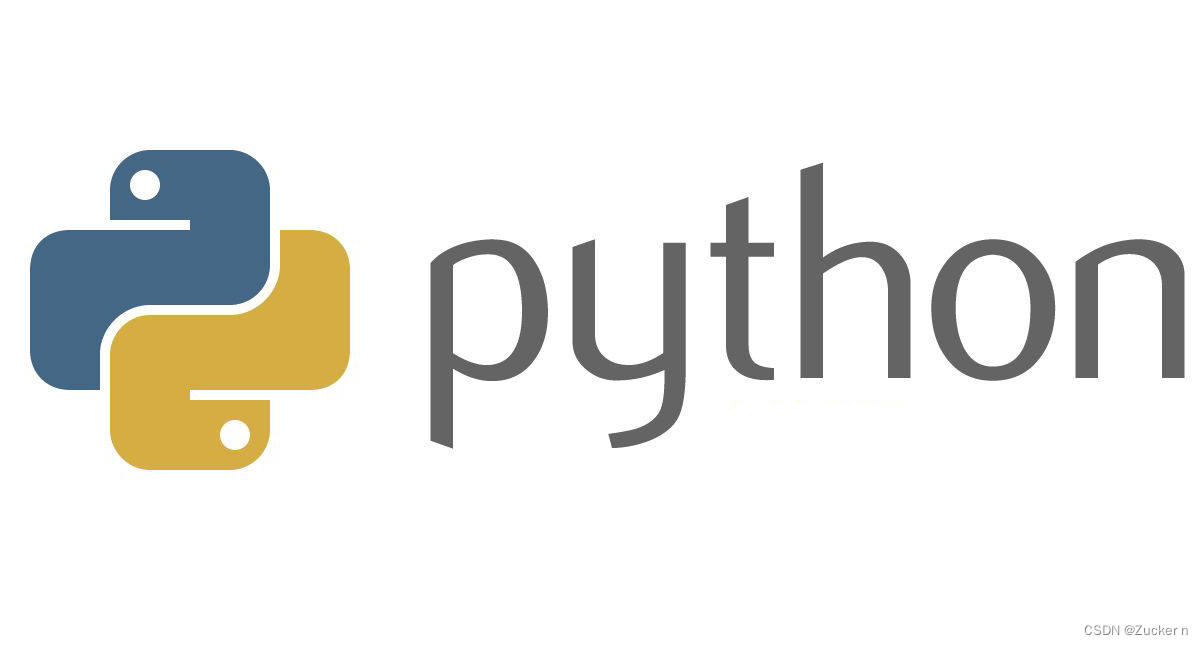
2.环境与工具
2.1 python环境
在Windows上使用命令行窗口查看所安装的python版本
python --version

2.2 Visual Studio Code编译
Visual Studio Code是一款由微软开发且跨平台的免费源代码编辑器。该软件以扩展的方式支持语法高亮、代码自动补全、代码重构功能,并且内置了命令行工具和Git 版本控制系统。

3.登录和注册窗口
3.1 代码构思
通过定义五个函数,实现对磁盘文件的读和写、用户登录判断、注册信息判断、保存以及注册窗口界面的生成。
3.2 代码实例
import tkinter as tk
from tkinter import messagebox
import pickle
import os
# 用pickle模块将字典变量进行序列转换并写入文件
def write_file(path, dic):
with open(path, 'wb') as f:
pickle.dump(dic, f)
def read_file(path):
with open(path, 'rb') as f:
dic = pickle.load(f)
return dic
# 判断用户登录时录入的信息是否正确
def login():
if os.path.exists('name.pickle'):
userinfo = read_file('name.pickle')
else:
userinfo = {}
name = txt_name.get()
passwd = txt_passwd.get()
if name in userinfo.keys():
if userinfo[name] == passwd:
messagebox.showinfo('登录成功', '欢迎您,' + name)
else:
messagebox.showerror('登录失败', '密码错误')
txt_name.set('')
txt_passwd.set('')
e_name.focus()
else:
messagebox.showerror('登录失败', '用户名不存在')
# 实现用户注册功能
def reg(regwin, path, name, passwd, passwd2):
if passwd != passwd2:
messagebox.showerror('注册失败', '两次输入的密码不一致')
return
if os.path.exists(path):
userinfo = read_file(path)
else:
userinfo = {}
if name in userinfo.keys():
messagebox.showerror('注册失败', '用户名已存在')
return
else:
userinfo.update({name: passwd})
write_file(path, userinfo)
messagebox.showinfo('注册成功', '欢迎您,' + name)
regwin.destroy()
# 生成一个注册窗口界面
def create_regwindow():
regwin = tk.Toplevel(win)
regwin.title('注册')
regwin.geometry('300x250')
lb_name = tk.Label(regwin, text='用户名', bg='gainsboro', font=('arial', 12), height=1, width=10)
lb_name.place(x=50, y=50)
lb_passwd = tk.Label(regwin, text='密码', bg='gainsboro', font=('arial', 12), height=1, width=10)
lb_passwd.place(x=50, y=100)
lb_passwd2 = tk.Label(regwin, text='确认密码', bg='gainsboro', font=('arial', 12), height=1, width=10)
lb_passwd2.place(x=50, y=150)
txt_name = tk.StringVar()
e_name = tk.Entry(regwin, textvariable=txt_name, font=('arial', 12), width=15)
e_name.place(x=150, y=50, anchor='nw')
txt_passwd = tk.StringVar()
e_passwd = tk.Entry(regwin, textvariable=txt_passwd, font=('arial', 12), show='*')
e_passwd.place(x=150, y=100, anchor='nw')
txt_passwd2 = tk.StringVar()
e_passwd2 = tk.Entry(regwin, textvariable=txt_passwd2, font=('arial', 12), show='*')
e_passwd2.place(x=150, y=150, anchor='nw')
# 生成一个注册按钮
btn_reg = tk.Button(regwin, text='注册', bg='gainsboro', font=('arial', 12), height=1, width=10,
command=lambda: reg(regwin, './part4/name.pickle', txt_name.get(), txt_passwd.get(), txt_passwd2.get()))
btn_reg.place(x=100, y=200, anchor='nw')
if __name__ == '__main__':
win = tk.Tk()
win.title('登录')
win.geometry('300x200')
win['background'] = 'gainsboro'
lb_name = tk.Label(win, text='用户名', bg='gainsboro', font=('arial', 12), height=1, width=10)
lb_name.place(x=50, y=50, anchor='nw')
lb_passwd = tk.Label(win, text='密码', bg='gainsboro', font=('arial', 12), height=1, width=10)
lb_passwd.place(x=50, y=100, anchor='nw')
txt_name = tk.StringVar()
e_name = tk.Entry(win, textvariable=txt_name, font=('arial', 12), width=15)
e_name.place(x=150, y=50, anchor='nw')
txt_passwd = tk.StringVar()
e_passwd = tk.Entry(win, textvariable=txt_passwd, font=('arial', 12), show='*')
e_passwd.place(x=150, y=100, anchor='nw')
btn_log = tk.Button(win, text='登录', bg='gainsboro', font=('arial', 12), height=1, width=10, command=login)
btn_log.place(x=50, y=150, anchor='nw')
btn_reg = tk.Button(win, text='注册', bg='gainsboro', font=('arial', 12), height=1, width=10, command=create_regwindow)
btn_reg.place(x=150, y=150, anchor='nw')
win.mainloop()
3.3 运行结果
登录
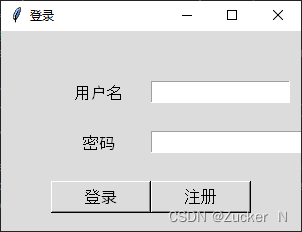
注册(以用户名111,密码111为例)
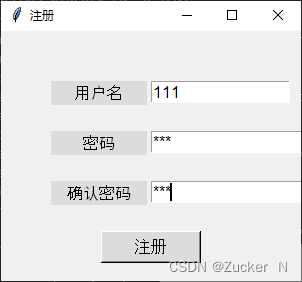
登录成功
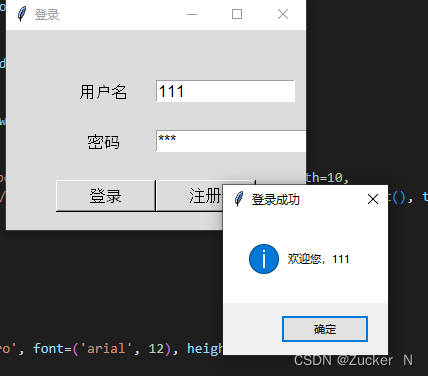
4.总结
通过定义函数实现可视化界面,通过利用python库实现信息的交互。