1. ArrayList的缺陷
当在ArrayList任意位置插入或者删除元素时,就需要将后序元素整体往前或者往后搬移,时间复杂度为O(n),效率比较低,因此ArrayList不适合做任意位置插入和删除比较多的场景。因此:java 集合中又引入了LinkedList,即链表结构。
2. 链表
2.1 链表的概念及结构
链表是一种物理存储结构上非连续存储结构,数据元素的逻辑顺序是通过链表中的引用链接次序实现的 。
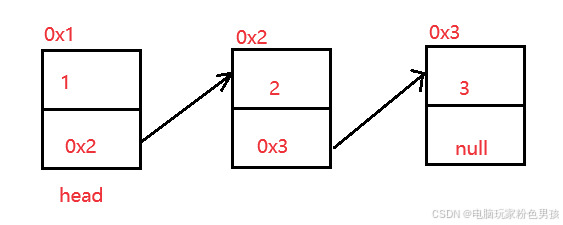
2.2 链表的实现
1.头插法 addFirst()
java
public void addFirst(int data) {
ListNode node = new ListNode(data);
node.next = head;
head = node;
}
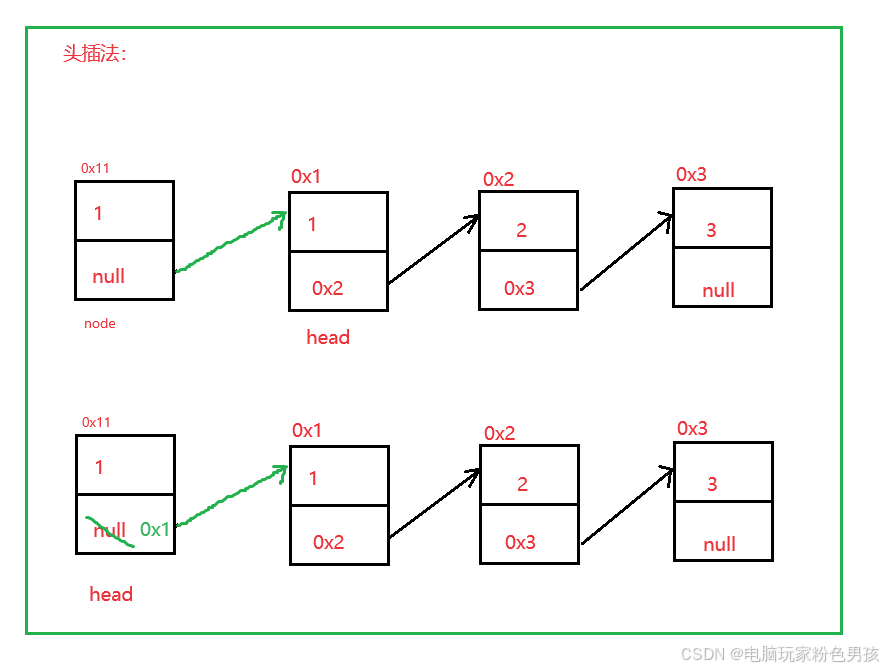
2.尾插法 addLast()
java
public void addList(int data) {
ListNode node = new ListNode(data);
if (head == null) {
head = node;
}
ListNode cur = head;
while (cur.next != null) {
cur = cur.next;
}
cur.next = node;
}
3.addIndex()
java
public void addIndex(int index, int data) {
int len = size();
// 不合法
if (index < 0 || index > len) {
System.out.println("index不合法");
return;
}
// 空链表
if (index == 0) {
addFirst(data);
return;
}
// 尾部插入
if (index == len) {
addLast(data);
return;
}
//中间位置插入
ListNode cur = head;
while (index - 1 != 0) {
cur = cur.next;
index--;
}
ListNode node = new ListNode(data);
node.next = cur.next;
cur.next = node;
}
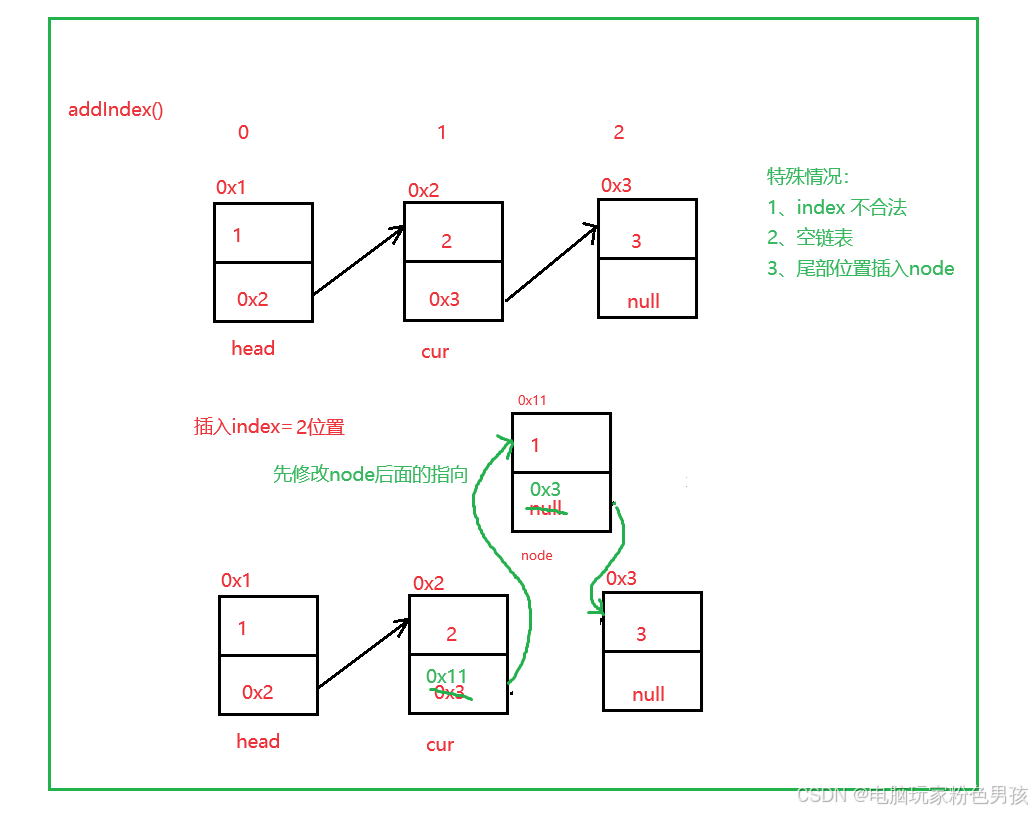
4.contains()
java
public boolean contains(int key) {
ListNode cur = head;
while (cur != null) {
if (cur.val == key) {
return true;
}
cur = cur.next;
}
return false;
}
5.remove()
java
public void remove(int key) {
if (head == null) {
return;
}
if (head.val == key) {
head = head.next;
}
ListNode cur = findNodeOfKey(key);
if (cur == null) {
return;
}
ListNode del = cur.next;
cur.next = del.next;
}
private ListNode findNodeOfKey(int key) {
ListNode cur = head;
while (cur.next != null) {
if (cur.next.val == key) {
return cur;
}
cur = cur.next;
}
return null;
}
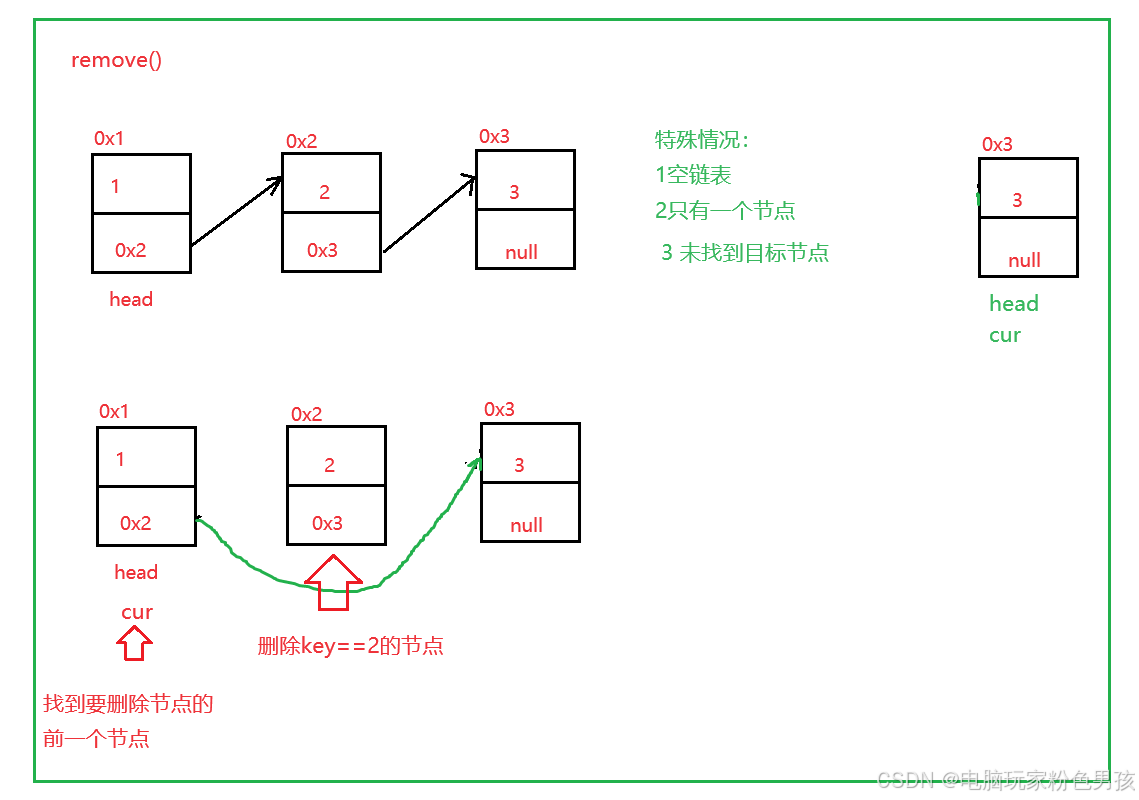
6.removeAllKey()
java
public void removeAllKey(int key) {
ListNode cur = head.next;
ListNode prev = head;
while (cur != null) {
if (cur.val == key) {
prev.next = cur.next;
} else {
prev = cur;
}
cur = cur.next;
}
if (head.val == key) {
head = head.next;
}
}
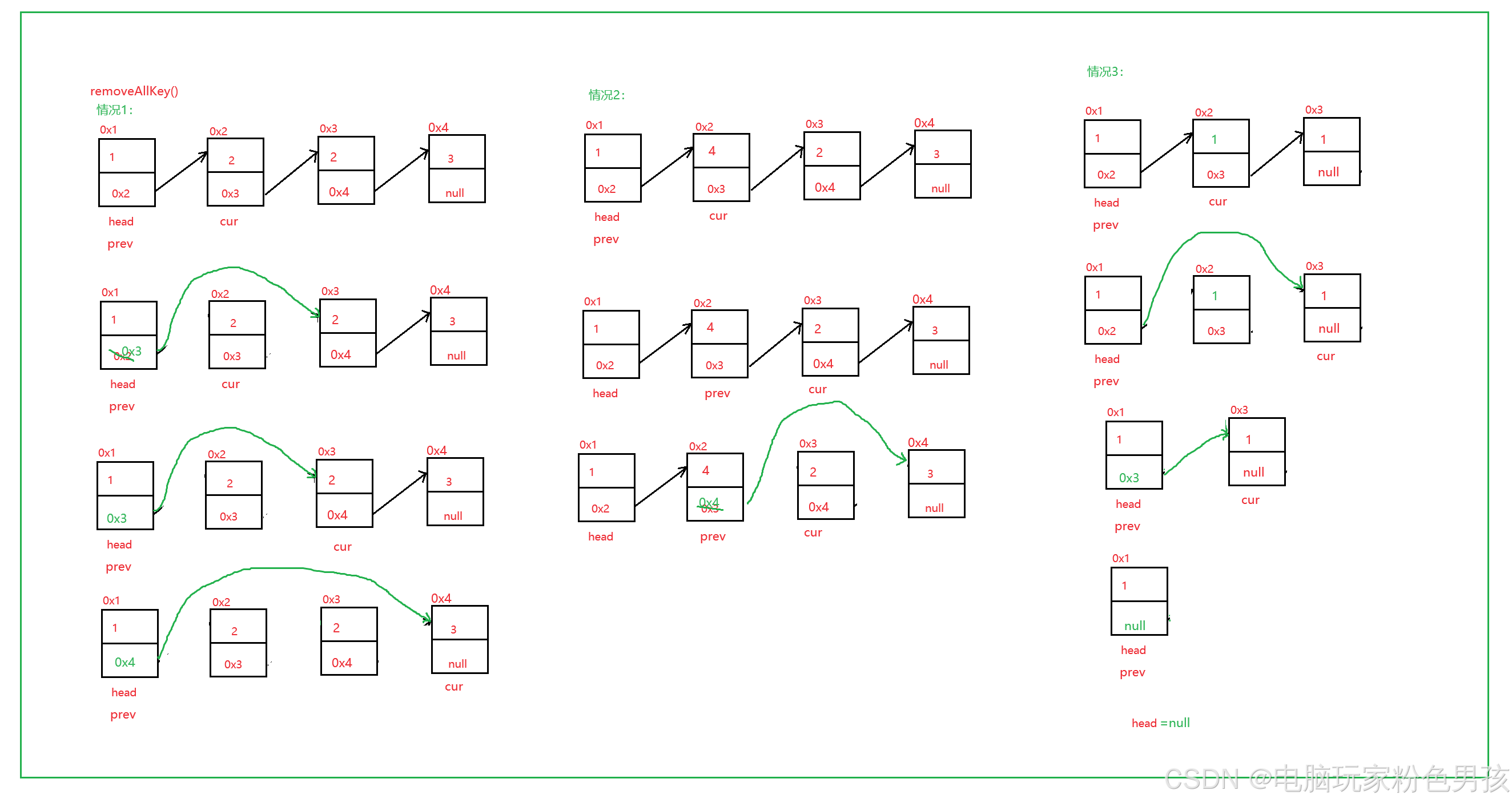
7.clear()
java
public void clear() {
ListNode cur = head;
while (cur != null) {
ListNode curNext = cur.next;
cur.next = null;
cur = curNext;
}
head = null;
}
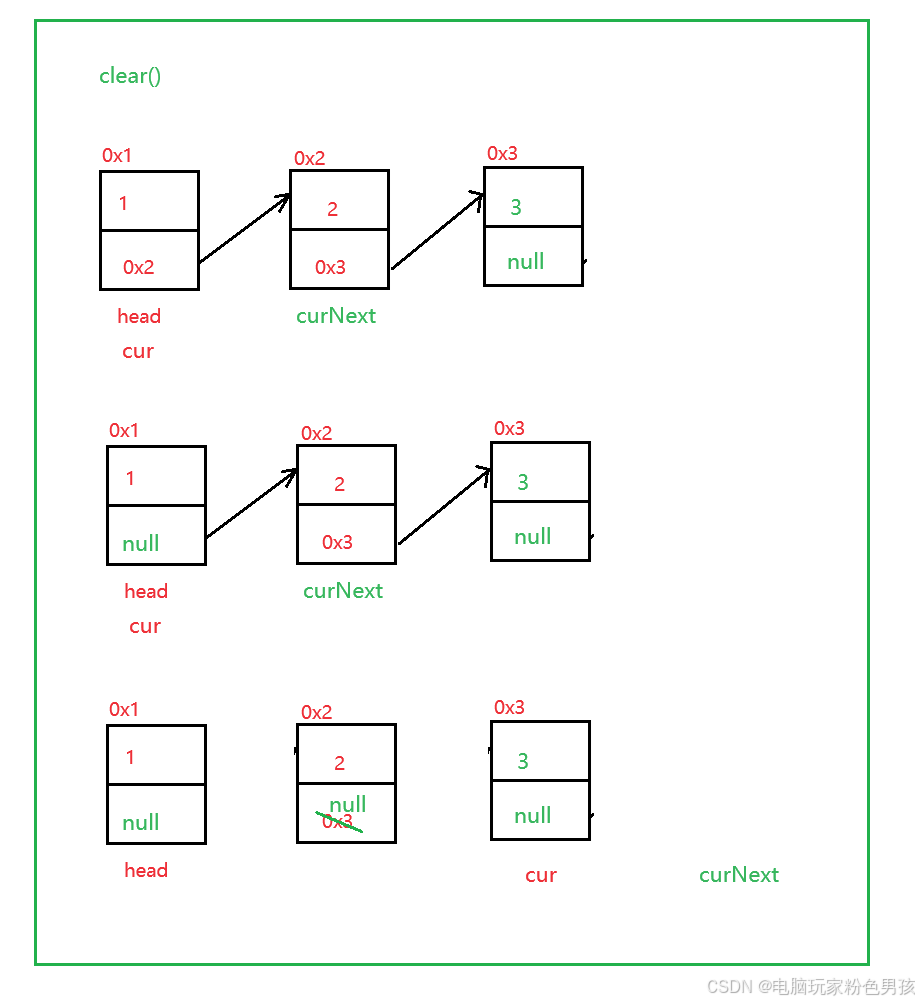
8.size()
java
public int size() {
int count = 0;
ListNode cur = head;
while (cur != null) {
count++;
cur = cur.next;
}
return count;
}
9.display()
java
public void display() {
ListNode cur = head;
while (cur != null) {
System.out.print(cur.val + " ");
cur = cur.next;
}
}