文章目录

🎉欢迎来到SpringBoot框架学习专栏~
引言
在现代Web应用程序中,文件上传和下载是非常常见的功能。无论是用户上传头像、上传文档还是下载报告,都需要一个高效、安全的文件处理系统。Spring Boot 作为一个流行的Java框架,为开发者提供了简便的方式来实现这些功能。在这篇文章中,我们将探讨如何在Spring Boot应用中实现文件的上传和下载,并包含详细的代码解析和技术分析。
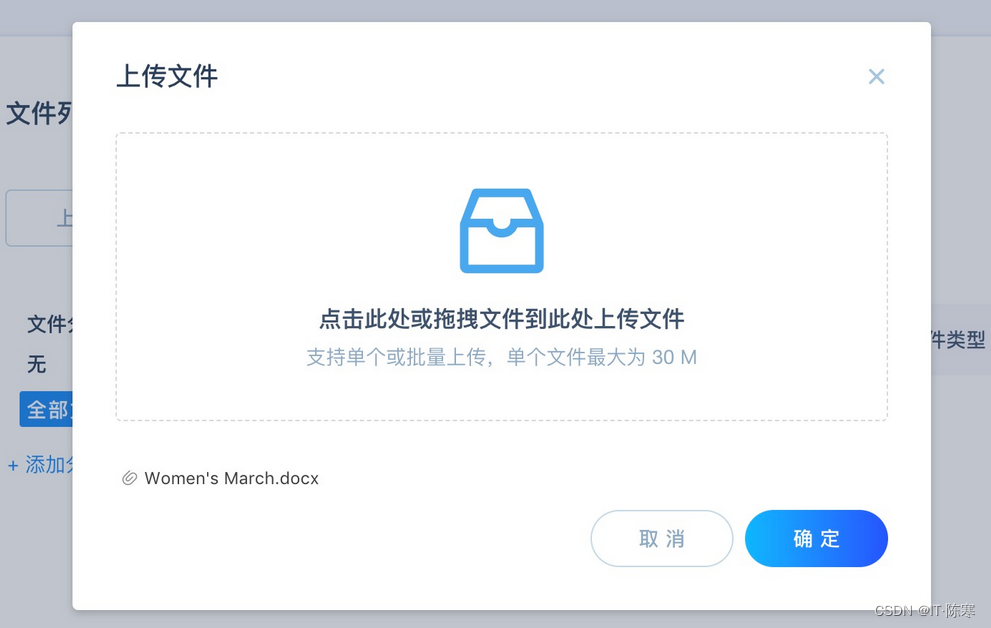
1. 环境配置
在开始编码之前,我们需要配置Spring Boot项目。假设你已经创建了一个Spring Boot项目,以下是需要添加的依赖项:
xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
</dependencies>
2. 文件上传
2.1 配置文件上传路径
首先,我们需要配置文件上传的存储路径。在application.properties
中添加以下配置:
properties
file.upload-dir=uploads
2.2 创建上传服务
创建一个服务类来处理文件的存储逻辑。我们将使用Spring的MultipartFile
接口来处理上传的文件。
java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.UUID;
@Service
public class FileStorageService {
@Value("${file.upload-dir}")
private String uploadDir;
public String storeFile(MultipartFile file) throws IOException {
// 生成唯一文件名
String fileName = UUID.randomUUID().toString() + "_" + file.getOriginalFilename();
Path targetLocation = Paths.get(uploadDir).resolve(fileName);
// 创建目录
Files.createDirectories(targetLocation.getParent());
// 保存文件
Files.copy(file.getInputStream(), targetLocation);
return fileName;
}
}
2.3 创建上传控制器
接下来,创建一个控制器来处理文件上传请求。
java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
@RestController
@RequestMapping("/api/files")
public class FileUploadController {
@Autowired
private FileStorageService fileStorageService;
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
try {
String fileName = fileStorageService.storeFile(file);
return ResponseEntity.ok("文件上传成功: " + fileName);
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("文件上传失败: " + e.getMessage());
}
}
}
3. 文件下载
3.1 创建下载服务
同样地,我们需要创建一个服务类来处理文件的下载逻辑。
java
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.stereotype.Service;
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
@Service
public class FileDownloadService {
@Value("${file.upload-dir}")
private String uploadDir;
public Resource loadFileAsResource(String fileName) throws IOException {
Path filePath = Paths.get(uploadDir).resolve(fileName).normalize();
Resource resource = new UrlResource(filePath.toUri());
if (resource.exists() || resource.isReadable()) {
return resource;
} else {
throw new IOException("文件未找到或不可读: " + fileName);
}
}
}
3.2 创建下载控制器
然后,创建一个控制器来处理文件下载请求。
java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.Resource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api/files")
public class FileDownloadController {
@Autowired
private FileDownloadService fileDownloadService;
@GetMapping("/download/{fileName:.+}")
public ResponseEntity<Resource> downloadFile(@PathVariable String fileName) {
try {
Resource resource = fileDownloadService.loadFileAsResource(fileName);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(null);
}
}
}
4. 前端页面
为了更好地展示文件上传和下载功能,我们可以使用Thymeleaf来创建一个简单的前端页面。
4.1 文件上传页面
html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>文件上传</title>
</head>
<body>
<h1>文件上传</h1>
<form method="POST" enctype="multipart/form-data" action="/api/files/upload">
<input type="file" name="file" />
<button type="submit">上传</button>
</form>
</body>
</html>
4.2 文件下载页面
html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>文件下载</title>
</head>
<body>
<h1>文件下载</h1>
<form method="GET" action="/api/files/download/{fileName}">
<input type="text" name="fileName" placeholder="文件名" />
<button type="submit">下载</button>
</form>
</body>
</html>
5. 技术分析
在这篇文章中,我们实现了Spring Boot中的文件上传和下载功能,涉及到的技术包括:
- Spring Boot Starter Web:提供了基础的Web开发功能。
- Spring Boot Starter Thymeleaf:用于前端页面的渲染。
- Spring Boot Starter Data JPA:如果需要将文件信息存储到数据库中,可以使用该依赖。
- MultipartFile:Spring提供的用于处理文件上传的接口。
- Resource:用于文件下载时的资源加载。
这些技术的结合使得我们能够快速、安全地实现文件处理功能。在实际应用中,你可能还需要考虑文件大小限制、文件类型验证、安全性等方面的需求。
结论
通过本文的讲解,相信你已经掌握了在Spring Boot中实现文件上传和下载的基本方法和技术细节。这些功能不仅增强了应用的实用性,也为用户提供了更好的体验。希望你能根据实际项目需求,进一步优化和扩展这些功能。
如果你对本文有任何疑问或建议,欢迎在评论区留言讨论。Happy coding!
🧸结尾 ❤️ 感谢您的支持和鼓励! 😊🙏
📜您可能感兴趣的内容:
- 【Java面试技巧】Java面试八股文 - 掌握面试必备知识(目录篇)
- 【Java学习路线】2023年完整版Java学习路线图
- 【AIGC人工智能】Chat GPT是什么,初学者怎么使用Chat GPT,需要注意些什么
- 【Java实战项目】SpringBoot+SSM实战:打造高效便捷的企业级Java外卖订购系统
- 【数据结构学习】从零起步:学习数据结构的完整路径