目录
- 前言
- [一、设置Vuforia AR环境](#一、设置Vuforia AR环境)
-
- [1. 添加AR Camera](#1. 添加AR Camera)
- [2. 设置目标图像](#2. 设置目标图像)
- 二、创建塔防游戏基础
-
- [1. 导入素材](#1. 导入素材)
- [2. 搭建场景](#2. 搭建场景)
- [3. 创建敌人](#3. 创建敌人)
- [4. 创建脚本](#4. 创建脚本)
前言
在增强现实(AR)技术快速发展的今天,Vuforia作为一个强大的AR开发平台,为开发者提供了许多便捷的工具和功能。在本篇博客中,我们将介绍如何使用Vuforia在Unity中创建一个简单的塔防游戏。通过结合Vuforia的图像识别和增强现实技术,我们可以将传统的塔防游戏带入一个全新的维度。
一、设置Vuforia AR环境
1. 添加AR Camera
在Unity场景中添加一个Vuforia AR Camera。可以在GameObject -> Vuforia Engine -> AR Camera
中找到。AR Camera将用于检测和跟踪目标图像。
2. 设置目标图像
Vuforia需要一个目标图像来识别并生成增强现实内容。在Vuforia开发者门户中,上传你的目标图像并生成一个目标数据库。下载生成的数据库并将其导入到Unity项目中。在Unity中,创建一个目标图像对象,路径为GameObject -> Vuforia Engine -> Image Target
,并选择你导入的目标数据库。
二、创建塔防游戏基础
1. 导入素材

2. 搭建场景
创建一个图片大小的plane
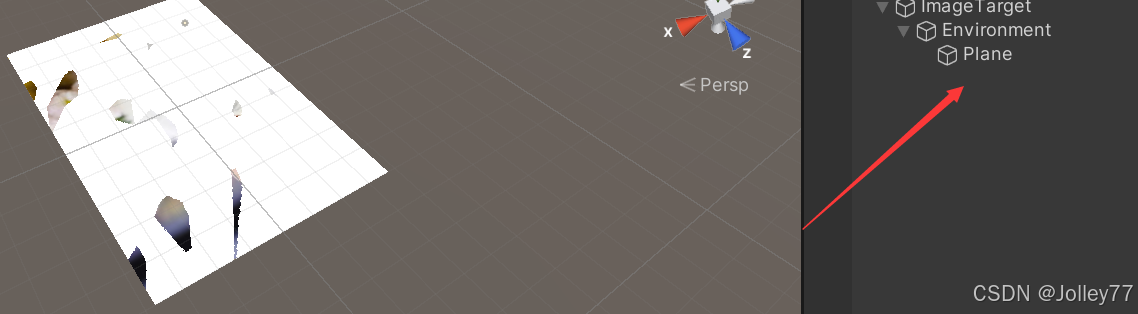
创建4个cube,然后围起来
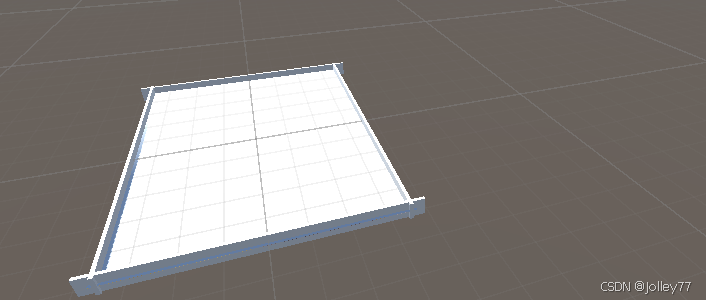
创建小方块作为炮台部署位置
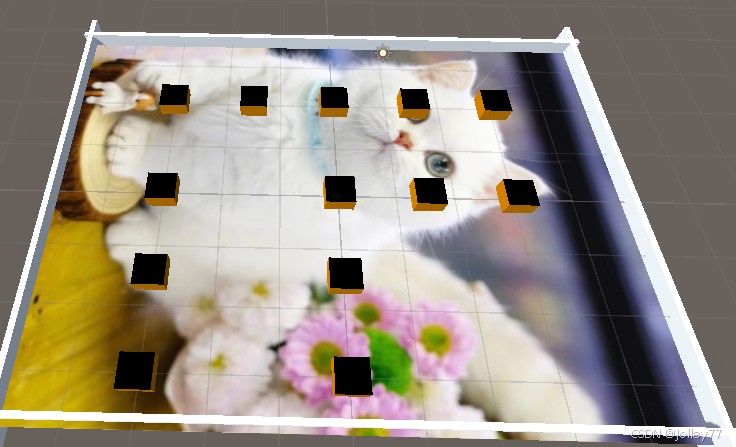
3. 创建敌人
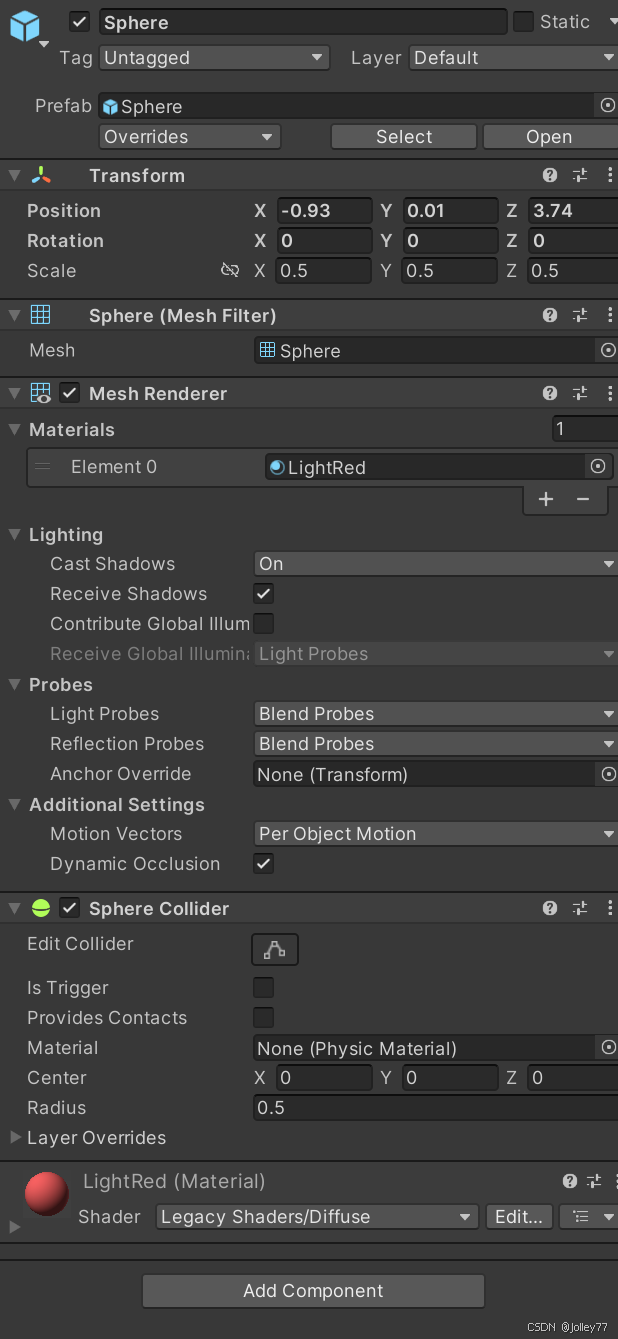
创建敌人移动的路径点
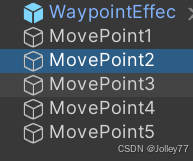
4. 创建脚本
小球生成脚本
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SpawnManager : MonoBehaviour
{
public GameObject enemyPrefab;
public GameObject parent;
void Awake()
{
}
void Start ()
{
CreateEnemy();
}
void Update ()
{
}
public void CreateEnemy()
{
GameObject enemy = Instantiate(enemyPrefab, parent.transform);
enemy.transform.SetParent(parent.transform);
enemy.transform.localPosition = new Vector3(-1f, 0.25f, 4f);
}
}
GameManager 脚本
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameManager : MonoBehaviour
{
public static GameManager Instance { get; private set; }
public List<Transform> pointList;
void Awake()
{
if (Instance==null)
{
Instance = this;
}
else
{
Destroy(gameObject);
}
}
void Start ()
{
}
void Update ()
{
}
}
敌人移动脚本
csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Enemy : MonoBehaviour
{
public int currentIndex;
public float moveSpeed;
void Awake()
{
moveSpeed = 0.2f;
currentIndex = 0;
}
void Start()
{
}
void Update()
{
Move();
}
public void Move()
{
int nextPoint = currentIndex + 1;
if (GameManager.Instance.pointList.Count <= nextPoint)
{
//TODO
return;
}
Vector3 v3 = transform.InverseTransformPoint(GameManager.Instance.pointList[nextPoint].position);
transform.Translate(v3 * (Time.deltaTime * moveSpeed));
if (IsArrive(GameManager.Instance.pointList[nextPoint]))
{
currentIndex++;
}
}
bool IsArrive(Transform t)
{
float distance = Vector3.Distance(transform.position, t.position);
if (distance < 0.05f)
{
return true;
}
return false;
}
}