迭代器模式
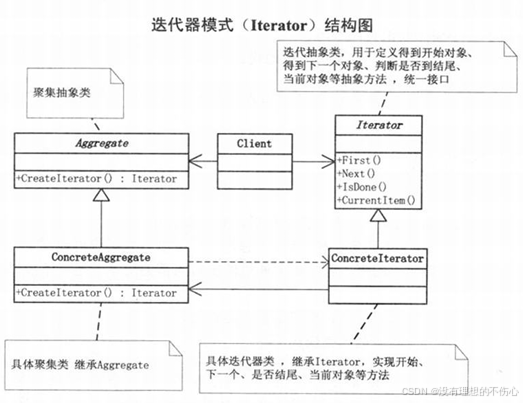
C++
cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 迭代抽象类,用于定义得到开始对象、得到下一个对象、判断是否到结尾、当前对象等抽象方法,统一接口
class Iterator
{
public:
Iterator(){};
virtual ~Iterator(){};
virtual string First() = 0;
virtual string Next() = 0;
virtual string CurrentItem() = 0;
virtual bool IsDone() = 0;
};
// 聚集抽象类
class Aggregate
{
public:
virtual int Count() = 0;
virtual void Push(const string &strValue) = 0;
virtual string Pop(const int nIndex) = 0;
virtual Iterator *CreateIterator() = 0;
};
// 具体迭代器类,继承Iterator 实现开始、下一个、是否结尾、当前对象等方法
class ConcreteIterator : public Iterator
{
public:
ConcreteIterator(Aggregate *pAggregate) : m_nCurrent(0), Iterator()
{
m_Aggregate = pAggregate;
}
string First()
{
return m_Aggregate->Pop(0);
}
string Next()
{
string strRet;
m_nCurrent++;
if (m_nCurrent < m_Aggregate->Count())
{
strRet = m_Aggregate->Pop(m_nCurrent);
}
return strRet;
}
string CurrentItem()
{
return m_Aggregate->Pop(m_nCurrent);
}
bool IsDone()
{
return ((m_nCurrent >= m_Aggregate->Count()) ? true : false);
}
private:
Aggregate *m_Aggregate;
int m_nCurrent;
};
// 具体聚集类 继承
class ConcreteAggregate : public Aggregate
{
public:
ConcreteAggregate() : m_pIterator(NULL)
{
m_vecItems.clear();
}
~ConcreteAggregate()
{
if (NULL != m_pIterator)
{
delete m_pIterator;
m_pIterator = NULL;
}
}
Iterator *CreateIterator()
{
if (NULL == m_pIterator)
{
m_pIterator = new ConcreteIterator(this);
}
return m_pIterator;
}
int Count()
{
return m_vecItems.size();
}
void Push(const string &strValue)
{
m_vecItems.push_back(strValue);
}
string Pop(const int nIndex)
{
string strRet;
if (nIndex < Count())
{
strRet = m_vecItems[nIndex];
}
return strRet;
}
private:
vector<string> m_vecItems;
Iterator *m_pIterator;
};
int main()
{
ConcreteAggregate *pName = NULL;
pName = new ConcreteAggregate();
if (NULL != pName)
{
pName->Push("hello");
pName->Push("word");
pName->Push("cxue");
}
Iterator *iter = NULL;
iter = pName->CreateIterator();
if (NULL != iter)
{
string strItem = iter->First();
while (!iter->IsDone())
{
cout << iter->CurrentItem() << " is ok" << endl;
iter->Next();
}
}
return 0;
}
C
c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct _Iterator
{
struct _Aggregate *aggregate;
int current;
} Iterator;
typedef struct _Aggregate
{
int count;
char **items;
} Aggregate;
// 创建迭代器
Iterator *Iterator_Create(Aggregate *agg)
{
Iterator *it = (Iterator *)malloc(sizeof(Iterator));
it->aggregate = agg;
it->current = 0;
return it;
}
// 释放迭代器
void Iterator_Destroy(Iterator *it)
{
free(it);
}
// 获取第一个元素
char *Iterator_First(Iterator *it)
{
if (it && it->aggregate && it->aggregate->count > 0)
{
return it->aggregate->items[0];
}
return NULL;
}
// 移动到下一个元素
char *Iterator_Next(Iterator *it)
{
if (it && it->aggregate && it->current >= 0 && it->current < it->aggregate->count)
{
it->current++;
return it->aggregate->items[it->current];
}
return NULL;
}
// 当前元素
char *Iterator_CurrentItem(Iterator *it)
{
if (it && it->aggregate && it->current < it->aggregate->count)
{
return it->aggregate->items[it->current];
}
return NULL;
}
// 判断是否结束
int Iterator_IsDone(Iterator *it)
{
return it->current >= it->aggregate->count;
}
// 创建聚合
Aggregate *Aggregate_Create()
{
Aggregate *agg = (Aggregate *)malloc(sizeof(Aggregate));
agg->count = 0;
agg->items = NULL;
return agg;
}
// 销毁聚合
void Aggregate_Destroy(Aggregate *agg)
{
if (agg->items)
{
int i;
for (i = 0; i < agg->count; i++)
{
free(agg->items[i]);
}
free(agg->items);
}
free(agg);
}
// 添加元素到聚合
void Aggregate_Add(Aggregate *agg, const char *item)
{
if (agg)
{
agg->items = realloc(agg->items, sizeof(char *) * (++agg->count));
agg->items[agg->count - 1] = strdup(item);
}
}
int main()
{
Aggregate *pName = Aggregate_Create();
Aggregate_Add(pName, "hello");
Aggregate_Add(pName, "world");
Aggregate_Add(pName, "cxue");
Iterator *iter = Iterator_Create(pName);
char *strItem = Iterator_First(iter);
while (!Iterator_IsDone(iter))
{
printf("%s is ok\n", Iterator_CurrentItem(iter));
strItem = Iterator_Next(iter);
}
Iterator_Destroy(iter);
Aggregate_Destroy(pName);
return 0;
}