文章目录
函数式接口
有且仅有一个抽象方法的接口。
格式:
txt
修饰符 interface 接口名称 {
public abstract 返回值类型 方法名称(可选参数信息);
// 其他非抽象方法内容
}
java
public interface MyFunctionalInterface {
void myMethod();
}
Java 8中专门为函数式接口引入了一个新的注解: @FunctionalInterface该注
解可用于一个接口的定义上:
java
@FunctionalInterface
public interface MyFunctionalInterface {
void myMethod();
}
自定义函数式接口进行使用
java
public class TestFunctionalInterface {
// 使用自定义的函数式接口作为方法参数
private static void doSomething(MyFunctionalInterface inter) {
inter.myMethod(); // 调用自定义的函数式接口方法
}
public static void main(String[] args) {
// 调用使用函数式接口的方法
doSomething(() ‐> System.out.println("Lambda执行啦!"));
}
}
函数式编程
Java语言通过Lambda表达式与方法引用等,为开发者打开了函数式编程的大门。
- Lambda的延迟执行
有些场景的代码执行后,结果不一定会被使用,从而造成性能浪费。而Lambda表达式是延迟执行的,这正好可以作为解决方案,提升性能。
性能浪费的日志案例:
java
public class Demo01Logger {
private static void log(int level, String msg) {
if (level == 1) {
System.out.println(msg);
}
}
public static void main(String[] args) {
String msgA = "Hello";
String msgB = "World";
String msgC = "Java";
log(1, msgA + msgB + msgC);
}
}
这段代码在执行的过程中,无论日志的等级是否为1都会先进行字符串的拼接操作,这就导致了性能的浪费。通过lambda表达式的延迟执行可以有效解决。
- 定义一个函数式接口
java
@FunctionalInterface
public interface MessageBuilder {
String buildMessage();
}
- 编写改造的log()方法
java
public class Demo02LoggerLambda {
private static void log(int level, MessageBuilder builder) {
if (level == 1) {
System.out.println(builder.buildMessage());
}
}
public static void main(String[] args) {
String msgA = "Hello";
String msgB = "World";
String msgC = "Java";
log(1, () ‐> msgA + msgB + msgC );
}
}
这样的话只有当日志级别达到要求的时候,才会执行字符串的拼接操作。
证明:
java
public class Demo03LoggerDelay {
private static void log(int level, MessageBuilder builder) {
if (level == 1) {
System.out.println(builder.buildMessage());
}
}
public static void main(String[] args) {
String msgA = "Hello";
String msgB = "World";
String msgC = "Java";
log(2, () -> {
System.out.println("Lambda执行!");
return msgA + msgB + msgC;
});
}
}
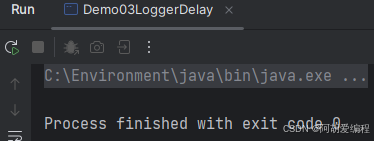
可以看到当我们执行该程序时,通过判断我们传递的数值2并不等于1则没有进行Lambda表达式中的拼接操作。
使用Lambda作为参数和返回值:
这里通过将 java.util.Comparator作为方法的返回值来实现排序规则。
java
import java.util.Arrays;
import java.util.Comparator;
public class Demo06Comparator {
private static Comparator<String> newComparator() {
return (a, b) ‐> b.length() ‐ a.length();
}
public static void main(String[] args) {
String[] array = { "abc", "ab", "abcd" };
System.out.println(Arrays.toString(array));
Arrays.sort(array, newComparator());
System.out.println(Arrays.toString(array));
}
}
常用函数式接口
- Supplier接口
java.util.function.Supplier<T> 接口仅包含一个无参的方法: T get() 。用来获取一个泛型参数指定类型的对象数据。
java
import java.util.function.Supplier;
public class Demo08Supplier {
private static String getString(Supplier<String> function) {
return function.get();
}
public static void main(String[] args) {
String msgA = "Hello";
String msgB = "World";
System.out.println(getString(() -> msgA + msgB));
}
}
这里Supplier接口指定了String类型,意思是获取一个String类型的数据。
- Consumer接口
java.util.function.Consumer<T> 接口则正好与Supplier接口相反,它不是生产一个数据,而是消费一个数据,其数据类型由泛型决定。
**抽象方法 void accept(T t) **
java
import java.util.function.Consumer;
public class Demo09Consumer {
private static void consumeString(Consumer<String> function) {
function.accept("Hello");
}
public static void main(String[] args) {
consumeString(s -> System.out.println(s));
}
}
默认方法:andThen
java
import java.util.function.Consumer;
public class Demo10ConsumerAndThen {
private static void consumeString(Consumer<String> one, Consumer<String> two) {
one.andThen(two).accept("Hello");
}
public static void main(String[] args) {
consumeString(
s -> System.out.println(s.toUpperCase()),
s -> System.out.println(s.toLowerCase()));
}
}
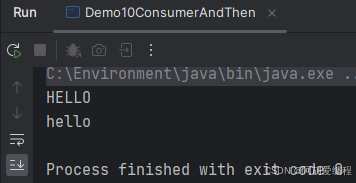
- Predicate接口
抽象方法: boolean test(T t)
要对某种类型的数据进行判断,从而得到一个boolean值结果
java
import java.util.function.Predicate;
public class Demo15PredicateTest {
private static void method(Predicate<String> predicate) {
boolean veryLong = predicate.test("HelloWorld");
System.out.println("字符串很长吗:" + veryLong);
}
public static void main(String[] args) {
method(s -> s.length() > 5);
}
}
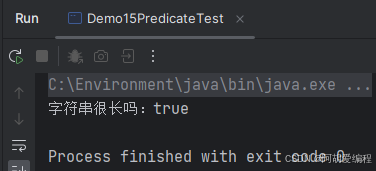
这里通过Lambda表达式作为返回值,通过test()方法判断字符串的长度是否大于5。
默认方法:and
将两个 Predicate 条件使用"与"逻辑连接起来实现"并且"的效果时,可以使用default方法 and
java
import java.util.function.Predicate;
public class Demo16PredicateAnd {
private static void method(Predicate<String> one, Predicate<String> two) {
boolean isValid = one.and(two).test("Helloworld");
System.out.println("字符串符合要求吗:" + isValid);
}
public static void main(String[] args) {
method(s -> s.contains("H"), s -> s.contains("W"));
}
}
这里结果为false,这是因为字符串中并不同时包含大写字母H和大写字母W。
默认的方法的参数列表和返回值全是Predicate接口。
默认方法:or
实现逻辑关系中的"或"
java
import java.util.function.Predicate;
public class Demo16PredicateAnd {
private static void method(Predicate<String> one, Predicate<String> two) {
boolean isValid = one.or(two).test("Helloworld");
System.out.println("字符串符合要求吗:" + isValid);
}
public static void main(String[] args) {
method(s ‐> s.contains("H"), s ‐> s.contains("W"));
}
}
这里结果为true,这是因为字符串中虽然不包含大写字母W,但是包含大写字母H。
默认方法:negate
执行了test方法之后,对结果boolean值进行"!"取反而已
java
import java.util.function.Predicate;
public class Demo17PredicateNegate {
private static void method(Predicate<String> predicate) {
boolean veryLong = predicate.negate().test("HelloWorld");
System.out.println("字符串很长吗:" + veryLong);
}
public static void main(String[] args) {
method(s ‐> s.length() < 5);
}
}
- Function接口
根据一个类型的数据得到另一个类型的数据,前者称为前置条件,后者称为后置条件。
抽象方法:apply
将 String 类型转换为 Integer 类型。
java
import java.util.function.Function;
public class Demo11FunctionApply {
private static void method(Function<String, Integer> function) {
int num = function.apply("10");
System.out.println(num + 20);
}
public static void main(String[] args) {
method(s ‐> Integer.parseInt(s));
}
}
默认方法:andThen
java
import java.util.function.Function;
public class Demo12FunctionAndThen {
private static void method(Function<String, Integer> one, Function<Integer, Integer> two) {
int num = one.andThen(two).apply("10");
System.out.println(num + 20);
}
public static void main(String[] args) {
method(str->Integer.parseInt(str)+10, i -> i *= 10);
}
}
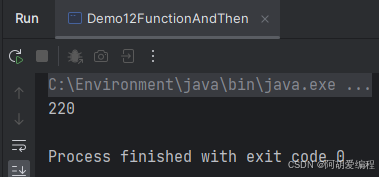
欢迎java热爱者了解文章,作者将会持续更新中,期待各位友友的关注和收藏。。。