目录
1.HTML
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>计算器</title>
<link rel="stylesheet" href="../css/calculator.css">
<style>
.calculator{
width: 250px;
height: 430px;
margin: 100px auto;
border: 1px #ccc solid;
}
h3{
width: 100%;
height: 50px;
border-bottom: 1px #ccc solid;
}
h4{
width: 100%;
height: 30px;
border-bottom: 1px #ccc solid;
}
.list{
width: 100%;
height: 350px;
display: flex;
flex-wrap: wrap;
justify-content: space-around;
align-content: space-around;
}
.list li{
width: 50px;
height: 50px;
line-height: 50px;
text-align: center;
border: 1px #ccc solid;
}
</style>
</head>
<body>
<div class="calculator">
<h3></h3>
<h4></h4>
<ul class="list">
<!-- <li>AC</li>
<li>%</li>
<li>←</li>
<li>÷</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>×</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>-</li>
<li>1</li>
<li>2</li>
<li>3</li>
<li>+</li>
<li>00</li>
<li>0</li>
<li>.</li>
<li>=</li> -->
</ul>
</div>
</body>
</html>
<script src="../js/calculator.js"></script>
<script>
let list = document.querySelector('.list');
let h3 = document.querySelector('h3');
let h4 = document.querySelector('h4');
let listData = ['AC','%','←','÷',7,8,9,'×',4,5,6,'-',1,2,3,'+','00',0,'.',"="];
listData.forEach(item => {
let li = `<li>${item}</li>`;
list.innerHTML+=li;
});
let li = list.querySelectorAll('li');
list.addEventListener('click',(e)=>{
let target = e.target;
// 检查点击的目标是否是 li 元素
if (target.tagName.toLowerCase() === 'li') {
h3.innerHTML+=target.innerHTML;
console.log(h3.innerHTML.slice(-2),'qqq');
if(h3.innerHTML.slice(-2).match(/^[\+\-\×\÷\%]+$/)){
console.log(h3.innerHTML);
h3.innerHTML=h3.innerHTML.substring(0, h3.innerHTML.length - 2) + target.innerHTML;
};
if(target.innerHTML=='←'){
let txt = h3.innerHTML;
console.log(txt.length);
h3.innerHTML=txt.substring(0, txt.length - 2);
console.log(target,h3.innerHTML);
}
if(target.innerHTML=='AC'){
h3.innerHTML='';
h4.innerHTML='';
}
if(target.innerHTML=='='){
let txt = h3.innerHTML.replace(/\u00F7/g, "/").replace(/\u00D7/g, "*");
h3.innerHTML=h3.innerHTML.substring(0, txt.length - 1);
let data = {
txt:txt.substring(0, txt.length - 1)
};
fetch("http://localhost:8080/api/calculator", {
method: "POST",
headers: {
'Content-Type': 'application/json', // 设置请求头的 Content-Type
},
body: JSON.stringify(data),
}).then(response => response.json()).then(res => {
h4.innerHTML=res.answer;
console.log("res:", res);
}).catch(err => {
console.log("err:", err);
});
}
// 阻止事件冒泡
e.stopPropagation();
}
});
</script>
2.CSS
css
*{margin: 0;padding: 0;}
ul,li,ol{list-style-type: none;}
button{cursor: pointer;border: none;background: rgba(255, 255, 255, 1);}
2.JS
javascript
let list = document.querySelector('.list');
let h3 = document.querySelector('h3');
let h4 = document.querySelector('h4');
let listData = ['AC','%','←','÷',7,8,9,'×',4,5,6,'-',1,2,3,'+','00',0,'.',"="];
listData.forEach(item => {
let li = `<li>${item}</li>`;
list.innerHTML+=li;
});
let li = list.querySelectorAll('li');
list.addEventListener('click',(e)=>{
let target = e.target;
// 检查点击的目标是否是 li 元素
if (target.tagName.toLowerCase() === 'li') {
h3.innerHTML+=target.innerHTML;
console.log(h3.innerHTML.slice(-2),'qqq');
if(h3.innerHTML.slice(-2).match(/^[\+\-\×\÷\%]+$/)){
console.log(h3.innerHTML);
h3.innerHTML=h3.innerHTML.substring(0, h3.innerHTML.length - 2) + target.innerHTML;
};
if(target.innerHTML=='←'){
let txt = h3.innerHTML;
console.log(txt.length);
h3.innerHTML=txt.substring(0, txt.length - 2);
console.log(target,h3.innerHTML);
}
if(target.innerHTML=='AC'){
h3.innerHTML='';
h4.innerHTML='';
}
if(target.innerHTML=='='){
let txt = h3.innerHTML.replace(/\u00F7/g, "/").replace(/\u00D7/g, "*");
h3.innerHTML=h3.innerHTML.substring(0, txt.length - 1);
let data = {
txt:txt.substring(0, txt.length - 1)
};
fetch("http://localhost:8080/api/calculator", {
method: "POST",
headers: {
'Content-Type': 'application/json', // 设置请求头的 Content-Type
},
body: JSON.stringify(data),
}).then(response => response.json()).then(res => {
h4.innerHTML=res.answer;
console.log("res:", res);
}).catch(err => {
console.log("err:", err);
});
}
// 阻止事件冒泡
e.stopPropagation();
}
});
4.资源
无
5.运行截图
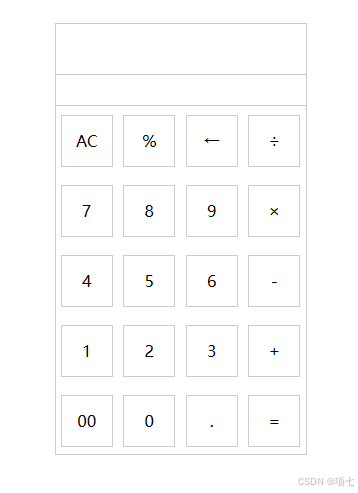
6.下载连接
在此处下载压缩包
【免费】原生js配合Node.js的计算器资源-CSDN文库
7.注意事项
此压缩包 包含前后端简单交互,后端需要用到Node.js,运行口令 nodemon app.js
或者在serve文件夹直接运行run.bat文件,运行成功后打开html即可。注意:run.bat运行成功后不要退出cmd。