axios
Axios 是一个基于 promise 网络请求库,作用于node.js 和浏览器中。 它是 isomorphic 的(即同一套代码可以运行在浏览器和node.js中)。在服务端它使用原生 node.js http
模块, 而在客户端 (浏览端) 则使用 XMLHttpRequests。
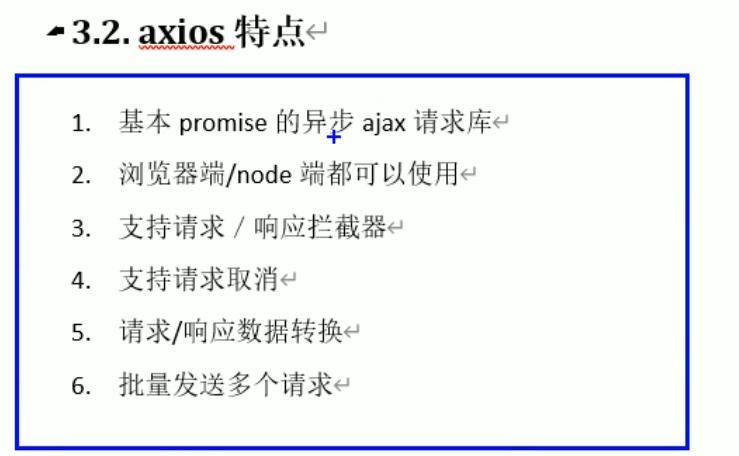
get请求:
javascript
<script>
function register(){
axios({
method:'GET',
url:'http://localhost:8080/java_web_final2_war_exploded/demo11'
}).then(
response => console.log(response),
error => console.log(error)
)
}
</script>
post请求:
javascript
axios.post('http://localhost:8080/java_web_final2_war_exploded/demo11', {
firstName: 'Fred',
lastName: 'Flintstone'
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
多并发:
javascript
function getUserAccount() {
return axios.get('/user/12345');
}
function getUserPermissions() {
return axios.get('/user/12345/permissions');
}
const [acct, perm] = await Promise.all([getUserAccount(), getUserPermissions()]);
// OR
Promise.all([getUserAccount(), getUserPermissions()])
.then(function ([acct, perm]) {
// ...
});
HTTP
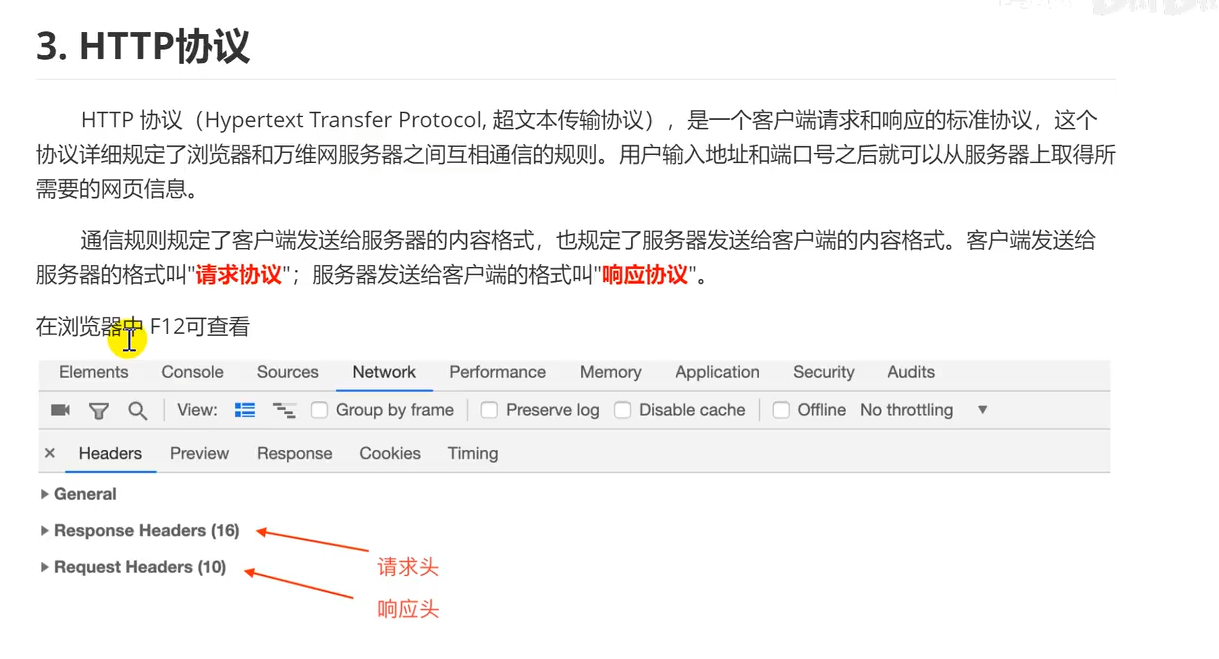
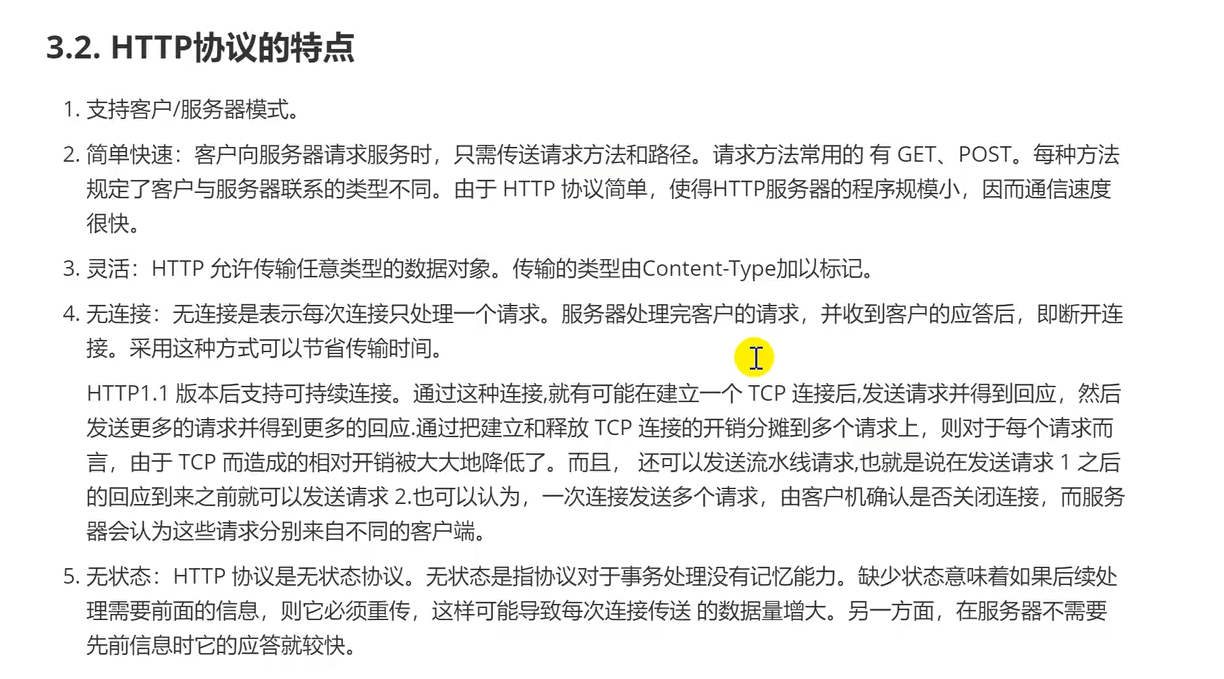
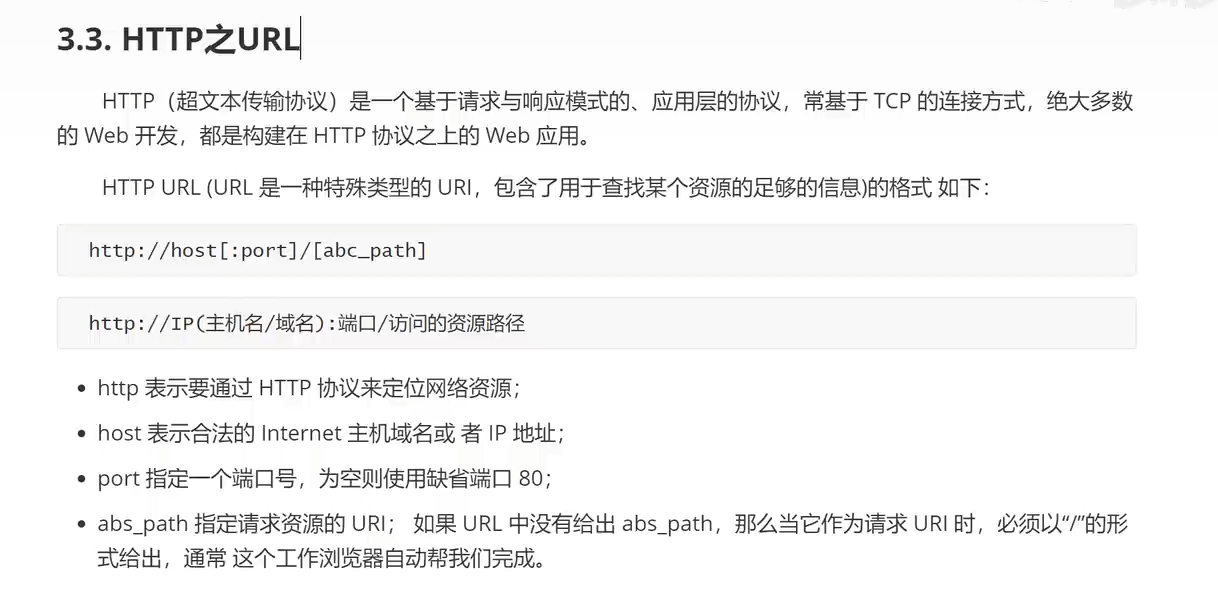
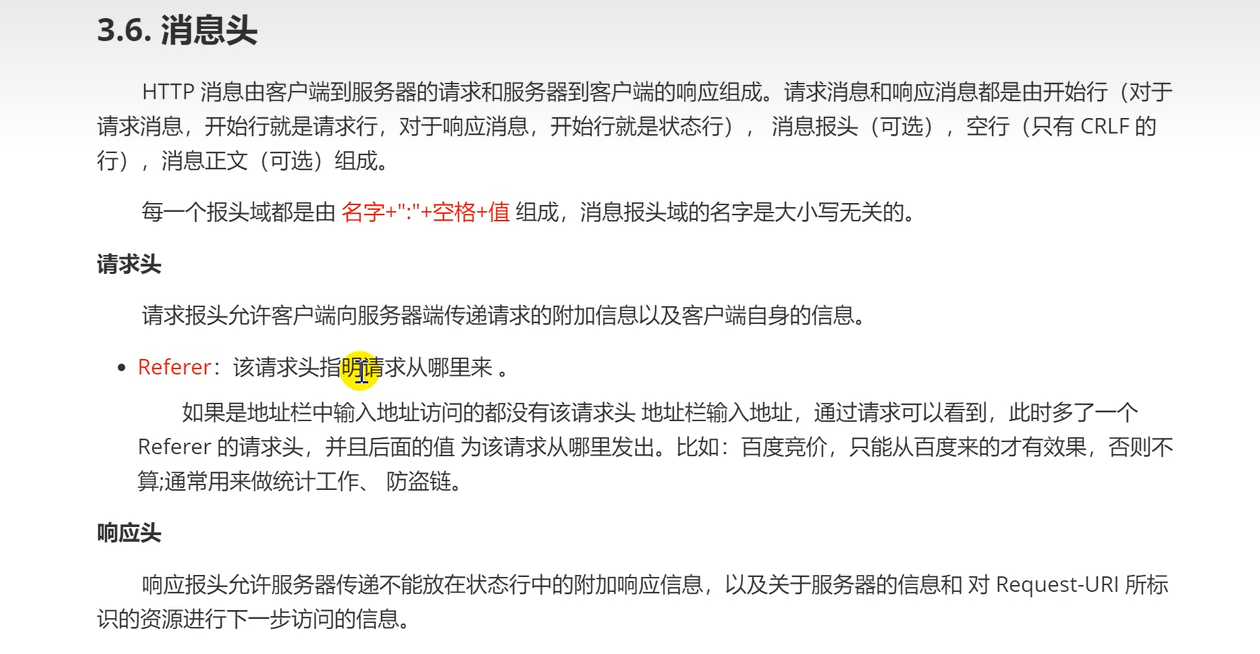
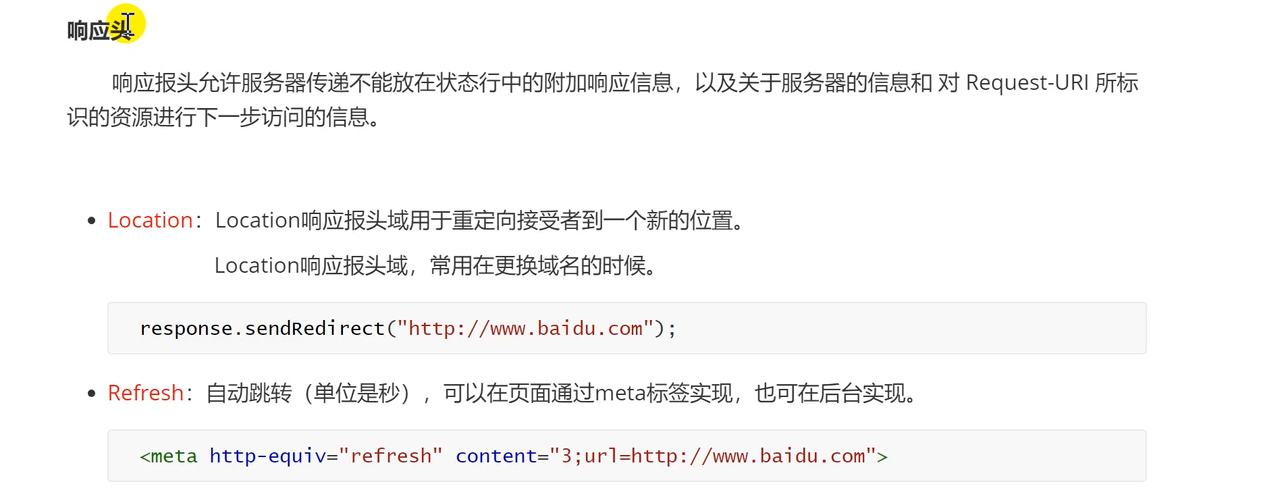