目录
[Book 结构体](#Book 结构体)
[User 结构体](#User 结构体)
实现功能
用户管理
- 添加用户:输入用户ID、用户名和密码,将新用户添加到系统中。
- 删除用户:根据用户ID删除指定用户。
- 修改用户:根据用户ID修改用户的用户名和密码。
- 显示所有用户:列出系统中的所有用户信息。
- 查找用户:根据用户ID查找并显示用户信息。
图书管理
- 添加图书:输入图书ID、书名、作者和出版社,将新图书添加到系统中。
- 删除图书:根据图书ID删除指定图书。
- 显示所有图书:列出系统中的所有图书信息。
- 查找图书:根据图书ID查找并显示图书信息。
借阅与归还
- 借阅图书:根据用户ID和图书ID记录借阅操作,并设置图书的到期日期。
- 归还图书:根据用户ID和图书ID记录归还操作,更新图书的状态。
未归还图书
- 列出未归还图书:显示所有未归还的图书信息,包括ID、书名、作者、出版社和到期日期。
部分效果图
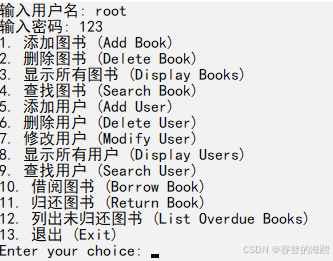
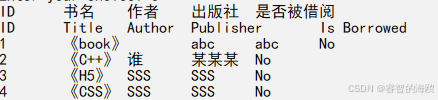
结构体
Book
结构体
id
: 图书ID
title
: 书名
author
: 作者
publisher
: 出版社
isBorrowed
: 是否被借阅
dueDate
: 到期日期(tm
结构体)
User
结构体
id
: 用户ID
username
: 用户名
password
: 密码
borrowedBooks
: 借阅图书记录(书ID和归还时间的配对)
源代码
编译时在连接器命令行加入
cpp
-std=c++11
完整代码
cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <algorithm>
#include <ctime>
#include <iomanip>
using namespace std;
// 图书信息
struct Book {
string id;
string title;
string author;
string publisher;
bool isBorrowed;
tm dueDate;
};
// 用户信息
struct User {
string id;
string username;
string password;
vector<pair<string, tm>> borrowedBooks; // 书ID和归还时间
};
// 全局变量
vector<Book> books;
vector<User> users;
// 打印日期
void printDate(const tm& date) {
cout << (date.tm_year + 1900) << '-' << (date.tm_mon + 1) << '-' << date.tm_mday << ' '
<< date.tm_hour << ':' << date.tm_min << ':' << date.tm_sec;
}
// 加载用户数据
void loadUsers() {
ifstream infile("users.txt");
if (!infile) return;
User user;
string line;
while (getline(infile, line)) {
istringstream ss(line);
ss >> user.id >> user.username >> user.password;
users.push_back(user);
}
}
// 保存用户数据
void saveUsers() {
ofstream outfile("users.txt");
for (const auto& user : users) {
outfile << user.id << ' ' << user.username << ' ' << user.password << '\n';
}
}
// 加载图书数据
void loadBooks() {
ifstream infile("books.txt");
if (!infile) return;
Book book;
string line;
while (getline(infile, line)) {
istringstream ss(line);
ss >> book.id >> book.title >> book.author >> book.publisher >> book.isBorrowed;
ss >> book.dueDate.tm_year >> book.dueDate.tm_mon >> book.dueDate.tm_mday
>> book.dueDate.tm_hour >> book.dueDate.tm_min >> book.dueDate.tm_sec;
book.dueDate.tm_year -= 1900;
book.dueDate.tm_mon -= 1;
books.push_back(book);
}
}
// 保存图书数据
void saveBooks() {
ofstream outfile("books.txt");
for (const auto& book : books) {
outfile << book.id << ' ' << book.title << ' ' << book.author << ' ' << book.publisher << ' '
<< book.isBorrowed << ' '
<< (book.dueDate.tm_year + 1900) << ' '
<< (book.dueDate.tm_mon + 1) << ' '
<< book.dueDate.tm_mday << ' '
<< book.dueDate.tm_hour << ' '
<< book.dueDate.tm_min << ' '
<< book.dueDate.tm_sec << '\n';
}
}
// 验证管理员账号密码
bool authenticate() {
string username, password;
cout << "输入用户名: ";
cin >> username;
cout << "输入密码: ";
cin >> password;
return username == "root" && password == "123";
}
// 修改管理员密码
void changePassword() {
string newPassword;
cout << "输入新密码: ";
cin >> newPassword;
// 在实际应用中,需要保存新密码
cout << "密码已更改! (Password changed!)\n";
}
// 添加图书
void addBook() {
Book book;
cout << "输入图书ID: ";
cin >> book.id;
cout << "输入书名: ";
cin.ignore(); // 清除输入缓冲区
getline(cin, book.title);
cout << "输入作者: ";
getline(cin, book.author);
cout << "输入出版社: ";
getline(cin, book.publisher);
book.isBorrowed = false;
books.push_back(book);
saveBooks();
cout << "图书已添加! (Book added!)\n";
}
// 删除图书
void deleteBook() {
string id;
cout << "输入要删除的图书ID: ";
cin >> id;
auto it = remove_if(books.begin(), books.end(), [&id](const Book& book) { return book.id == id; });
if (it != books.end()) {
books.erase(it, books.end());
saveBooks();
cout << "图书已删除! (Book deleted!)\n";
} else {
cout << "图书未找到! (Book not found!)\n";
}
}
// 显示所有图书
void displayBooks() {
cout << "ID\t书名\t作者\t出版社\t是否被借阅\n";
cout << "ID\tTitle\tAuthor\tPublisher\tIs Borrowed\n";
for (const auto& book : books) {
cout << book.id << '\t' << book.title << '\t' << book.author << '\t' << book.publisher << '\t'
<< (book.isBorrowed ? "Yes" : "No") << '\n';
}
}
// 查找图书
void searchBook() {
string id;
cout << "输入图书ID: ";
cin >> id;
auto it = find_if(books.begin(), books.end(), [&id](const Book& book) { return book.id == id; });
if (it != books.end()) {
const Book& book = *it;
cout << "ID: " << book.id << '\n' << "书名: " << book.title << '\n' << "作者: " << book.author << '\n'
<< "出版社: " << book.publisher << '\n' << "是否被借阅: " << (book.isBorrowed ? "Yes" : "No") << '\n';
cout << "到期日期: ";
printDate(book.dueDate);
cout << '\n';
} else {
cout << "图书未找到! (Book not found!)\n";
}
}
// 添加用户
void addUser() {
User user;
cout << "输入用户ID: ";
cin >> user.id;
cout << "输入用户名: ";
cin >> user.username;
cout << "输入密码: ";
cin >> user.password;
users.push_back(user);
saveUsers();
cout << "用户已添加! (User added!)\n";
}
// 删除用户
void deleteUser() {
string id;
cout << "输入要删除的用户ID: ";
cin >> id;
auto it = remove_if(users.begin(), users.end(), [&id](const User& user) { return user.id == id; });
if (it != users.end()) {
users.erase(it, users.end());
saveUsers();
cout << "用户已删除! (User deleted!)\n";
} else {
cout << "用户未找到! (User not found!)\n";
}
}
// 修改用户
void modifyUser() {
string id;
cout << "输入要修改的用户ID: ";
cin >> id;
auto it = find_if(users.begin(), users.end(), [&id](const User& user) { return user.id == id; });
if (it != users.end()) {
User& user = *it;
cout << "输入新的用户名: ";
cin >> user.username;
cout << "输入新的密码: ";
cin >> user.password;
saveUsers();
cout << "用户已修改! (User modified!)\n";
} else {
cout << "用户未找到! (User not found!)\n";
}
}
// 显示所有用户
void displayUsers() {
cout << "ID\t用户名\t密码\n";
cout << "ID\tUsername\tPassword\n";
for (const auto& user : users) {
cout << user.id << '\t' << user.username << '\t' << user.password << '\n';
}
}
// 查找用户
void searchUser() {
string id;
cout << "输入用户ID: ";
cin >> id;
auto it = find_if(users.begin(), users.end(), [&id](const User& user) { return user.id == id; });
if (it != users.end()) {
const User& user = *it;
cout << "ID: " << user.id << '\n' << "用户名: " << user.username << '\n' << "密码: " << user.password << '\n';
} else {
cout << "用户未找到! (User not found!)\n";
}
}
// 借阅图书
void borrowBook() {
string userId, bookId;
cout << "输入用户ID: ";
cin >> userId;
cout << "输入图书ID: ";
cin >> bookId;
auto userIt = find_if(users.begin(), users.end(), [&userId](const User& user) { return user.id == userId; });
auto bookIt = find_if(books.begin(), books.end(), [&bookId](const Book& book) { return book.id == bookId; });
if (userIt != users.end() && bookIt != books.end()) {
User& user = *userIt;
Book& book = *bookIt;
if (!book.isBorrowed) {
book.isBorrowed = true;
time_t now = time(0);
tm* now_tm = localtime(&now);
book.dueDate = *now_tm;
user.borrowedBooks.push_back(make_pair(bookId, book.dueDate));
saveBooks();
saveUsers();
cout << "图书已借出! (Book borrowed!)\n";
} else {
cout << "图书已被借出! (Book is already borrowed!)\n";
}
} else {
cout << "用户或图书未找到! (User or book not found!)\n";
}
}
// 归还图书
void returnBook() {
string userId, bookId;
cout << "输入用户ID: ";
cin >> userId;
cout << "输入图书ID: ";
cin >> bookId;
auto userIt = find_if(users.begin(), users.end(), [&userId](const User& user) { return user.id == userId; });
auto bookIt = find_if(books.begin(), books.end(), [&bookId](const Book& book) { return book.id == bookId; });
if (userIt != users.end() && bookIt != books.end()) {
User& user = *userIt;
Book& book = *bookIt;
if (book.isBorrowed) {
book.isBorrowed = false;
auto borrowedBookIt = find_if(user.borrowedBooks.begin(), user.borrowedBooks.end(), [&bookId](const pair<string, tm>& borrowedBook) { return borrowedBook.first == bookId; });
if (borrowedBookIt != user.borrowedBooks.end()) {
user.borrowedBooks.erase(borrowedBookIt);
}
saveBooks();
saveUsers();
cout << "图书已归还! (Book returned!)\n";
} else {
cout << "图书没有被借出! (Book was not borrowed!)\n";
}
} else {
cout << "用户或图书未找到! (User or book not found!)\n";
}
}
// 列出逾期图书
void listOverdueBooks() {
time_t now = time(0);
tm* now_tm = localtime(&now);
cout << "ID\t书名\t作者\t出版社\t到期日期\n";
cout << "ID\tTitle\tAuthor\tPublisher\tDue Date\n";
for (const auto& book : books) {
if (book.isBorrowed && difftime(mktime(now_tm), mktime(const_cast<tm*>(&book.dueDate))) > 0) {
cout << book.id << '\t' << book.title << '\t' << book.author << '\t' << book.publisher << '\t';
printDate(book.dueDate);
cout << '\n';
}
}
}
// 主菜单
int main() {
check:
if (!authenticate()) {
cout << "认证失败! (Authentication failed!)\n";
goto check;
}
loadUsers();
loadBooks();
int choice;
do {
cout << "1. 添加图书 (Add Book)\n2. 删除图书 (Delete Book)\n3. 显示所有图书 (Display Books)\n"
<< "4. 查找图书 (Search Book)\n5. 添加用户 (Add User)\n6. 删除用户 (Delete User)\n"
<< "7. 修改用户 (Modify User)\n8. 显示所有用户 (Display Users)\n9. 查找用户 (Search User)\n"
<< "10. 借阅图书 (Borrow Book)\n11. 归还图书 (Return Book)\n12. 列出未归还图书 (List Overdue Books)\n"
<< "13. 退出 (Exit)\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1: addBook(); break;
case 2: deleteBook(); break;
case 3: displayBooks(); break;
case 4: searchBook(); break;
case 5: addUser(); break;
case 6: deleteUser(); break;
case 7: modifyUser(); break;
case 8: displayUsers(); break;
case 9: searchUser(); break;
case 10: borrowBook(); break;
case 11: returnBook(); break;
case 12: listOverdueBooks(); break;
case 13: break;
default: cout << "无效选择! (Invalid choice!)\n"; break;
}
} while (choice != 13);
saveUsers();
saveBooks();
return 0;
}