目录
-
- [1. 实现Interceptor接口](#1. 实现Interceptor接口)
- [2. 注册配置文件](#2. 注册配置文件)

假如我们想实现多租户,或者在某些 SQL 后面自动拼接查询条件。在开发过程中大部分场景可能都是一个查询写一个 SQL 去处理,我们如果想修改最终 SQL 可以通过修改各个 mapper.xml 中的 SQL 来处理。
但实际过程中我们可能穿插着 ORM 和 SQL 的混合使用,隐藏在代码中不容易被发现,还有假如项目中有很多很多的 SQL 我们不可能一个一个的去修改解决。
这个时候我们就需要通过 mybatis 拦截 SQL 并且最终修改 SQL。
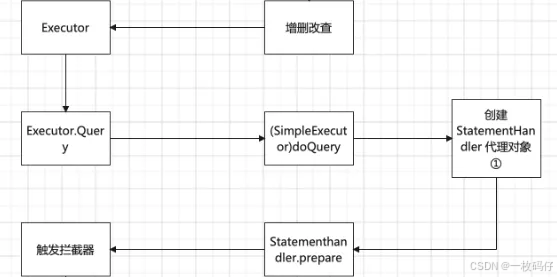
具体操作如下:
1. 实现Interceptor接口
实现Interceptor接口,并写相关逻辑
java
package cn.source.framework.interceptor.impl;
import cn.source.common.core.domain.BaseEntity;
import cn.source.common.core.domain.entity.SysUser;
import cn.source.common.utils.DateUtils;
import cn.source.common.utils.SecurityUtils;
import org.apache.ibatis.executor.Executor;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.mapping.SqlCommandType;
import org.apache.ibatis.plugin.*;
import java.util.Properties;
/**
* @Description: 自定义拦截器,注入创建人,创建日期,修改人,修改日期,企业id
*/
@Intercepts({
@Signature(
type = Executor.class,
method = "update",
args = {MappedStatement.class, Object.class}),
})
public class HandleBaseInfoInterceptor implements Interceptor {
@Override
public Object intercept(Invocation invocation) throws Throwable {
Object[] args = invocation.getArgs();
MappedStatement mappedStatement = (MappedStatement) args[0];
SqlCommandType sqlCommandType = mappedStatement.getSqlCommandType();
// 用户类不处理,会导致获取不到用户信息出错
if (args[1] instanceof SysUser) {
return invocation.proceed();
}
if (args[1] instanceof BaseEntity) {
BaseEntity baseEntity = (BaseEntity) args[1];
if (sqlCommandType == SqlCommandType.UPDATE) {
baseEntity.setUpdateTime(DateUtils.getNowDate());
baseEntity.setUpdateBy(SecurityUtils.getUsername());
} else if (sqlCommandType == SqlCommandType.INSERT) {
baseEntity.setCreateTime(DateUtils.getNowDate());
baseEntity.setCreateBy(SecurityUtils.getUsername());
// System.out.println("赋值企业ID:" + baseEntity.getCompanyId());
baseEntity.setCompanyId(SecurityUtils.getLoginUser().getUser().getCompanyId());
}
}
return invocation.proceed();
}
@Override
public Object plugin(Object target) {
return Plugin.wrap(target, this);
}
@Override
public void setProperties(Properties properties) {
}
}
2. 注册配置文件
将插件注册到 mybatis 的配置文件 mybatis-config.xml
xml
<plugins>
<!-- 自定义拦截器,注入企业id-->
<plugin interceptor="cn.source.framework.interceptor.impl.HandleSelectInterceptor"> </plugin>
<!-- 自定义拦截器,注入创建人,创建日期,修改人,修改日期,企业id-->
<plugin interceptor="cn.source.framework.interceptor.impl.HandleBaseInfoInterceptor"> </plugin>
</plugins>