字典
字典是C#中的一种集合,它存储键值对,并且每个键与一个值相关联。
创建字典
Dictionary<键的类型, 值的类型> 字典名字 = new Dictionary<键的类型, 值的类型>();
cs
Dictionary<int, string> dicStudent = new Dictionary<int, string>();
字典基本操作
添加元素
cs
dicStudent.Add(2, "张三");
dicStudent.Add(5, "李四");
dicStudent.Add(8, "王五");
访问元素
字典名[键名];
cs
Console.WriteLine(dicStudent[5]);

检查键是否存在
cs
if (dicStudent.ContainsKey(1))
{
Console.WriteLine("键存在");
}
else
{
Console.WriteLine("键不存在");
}
if (dicStudent.ContainsKey(2))
{
Console.WriteLine("键存在");
}
else
{
Console.WriteLine("键不存在");
}
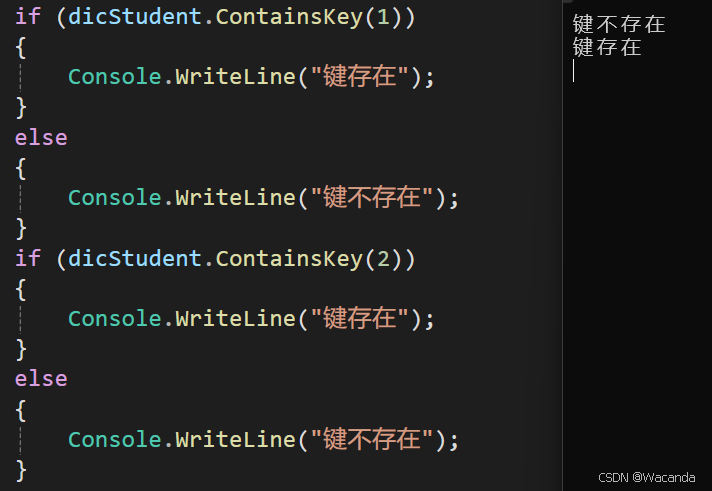
字典容量
cs
int count = dicStudent.Count;
Console.WriteLine(count);
遍历字典
cs
foreach (KeyValuePair<int, string> item in dicStudent)
{
Console.WriteLine($"{item.Key}:{item.Value}");
}
为了简化字典遍历的写法使用 var 代替 KeyValuePair<int, string> 类型。
cs
foreach (var item in dicStudent)
{
Console.WriteLine($"{item.Key}:{item.Value}");
}
删除元素
cs
dicStudent.Remove(5); //移除5键这个键值对
foreach (var item in dicStudent)
{
Console.WriteLine($"{item.Key}:{item.Value}");
}

获取键的列表
cs
var keys = dicStudent.Keys;
foreach (var key in keys)
{
Console.WriteLine(key);
}
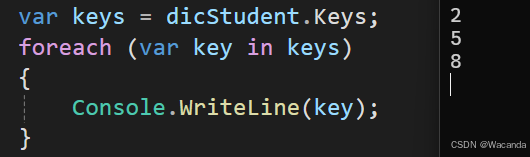
获取值的列表
cs
var values = dicStudent.Values;
foreach (var value in values)
{
Console.WriteLine(value);
}
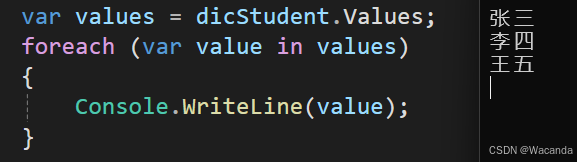
清空字典
cs
dicStudent.Clear();