1.h5,使用原生方式监听页面滚动下拉加载更多
<template>
<div></div>
</template>
<script>
export default {
data() {
return {
loadflag: true,
maxpages: 0, //最大页码
currentpage: 0, //当前页
listData: [],
config: {
page: 1,
pageSize: 15,
total: 0,
pages: 0,
},
}
},
created() {
this.getList()
window.addEventListener('scroll', this.handleScroll, true)
},
methods: {
handleScroll() {
const _this = this
let scrollTop =
document.documentElement.scrollTop || document.body.scrollTop
//变量windowHeight是可视区的高度
let windowHeight =
document.documentElement.clientHeight || document.body.clientHeight
//变量scrollHeight是滚动条的总高度
let scrollHeight =
document.documentElement.scrollHeight || document.body.scrollHeight
//滚动条到底部的条件
let data = scrollTop + windowHeight
if (
data == scrollHeight ||
(scrollHeight - data <= 60 && _this.loadflag)
) {
let currentpage = _this.currentpage
let maxpages = _this.maxpages
if (maxpages > currentpage && _this.loadflag) {
_this.loadflag = false
_this.config.page++
_this.getList()
}
}
},
async getList() {
let params = {
page: this.config.page,
pageSize: this.config.pageSize,
}
const res = await apiGet(posterGetBrochureList, params)
this.isShowList = true
if (res.code == 200) {
const { total, records, current, pages } = res.data
this.config.page = current //当前页
this.config.pages = pages //总共多少页
this.config.total = total //总条数
this.maxpages = pages
this.currentpage = current
if (current <= pages) {
this.loadflag = true
this.listData = [...this.listData, ...records]
} else {
this.listData = records
}
}
},
},
destroyed() {
//离开页面的时候移除监听滚动事件,提高性能
window.removeEventListener('scroll', this.handleScroll, true)
},
}
</script>
<style lang="scss" scoped></style>
2.使用组件vant(van-list)
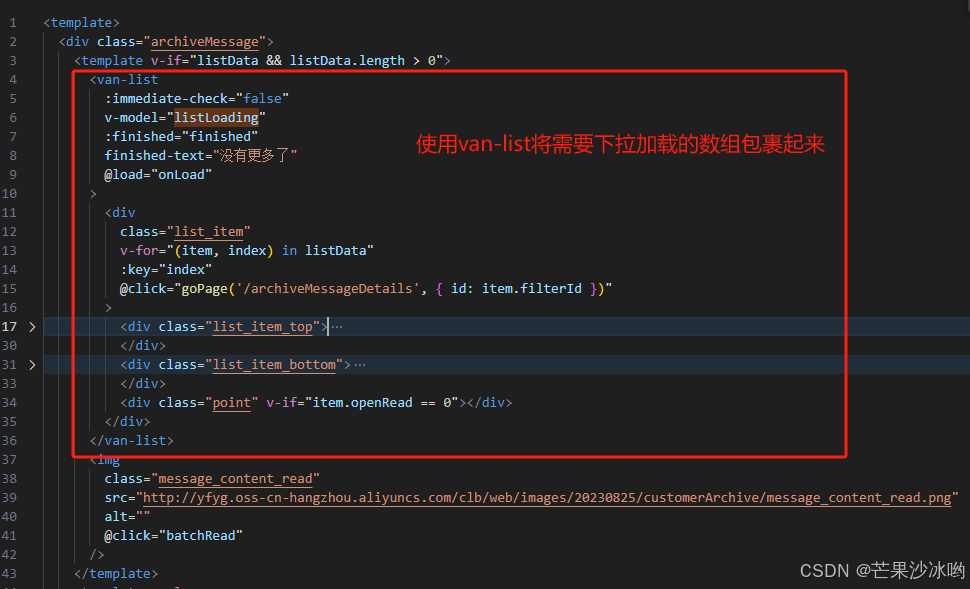
<template>
<div></div>
</template>
<script>
export default {
data() {
return {
listLoading: false, //分页加载
finished: false, //分页是否加载完成
listData: [],
config: {
page: 1,
pageSize: 10,
total: 0,
},
}
},
created() {
this.getList()
},
methods: {
//关键函数,监听下拉加载更多
onLoad() {
this.getList()
},
async getList() {
const formData = {
page: this.config.page,
pageSize: this.config.pageSize,
}
const res = await apiGet(apigetqueryArchiveMatchedPageList, formData)
if (res.code == 200) {
const data = res.data
this.listData = [...this.listData, ...data.records]
this.listLoading = false
//判断如果当前请求条数小于十条,就停止下拉加载
if (data.records.length < 10) {
this.finished = true
}
this.config.page++
}
},
},
}
</script>
<style lang="scss" scoped></style>
3.uniapp使用scroll-view下拉加载更多
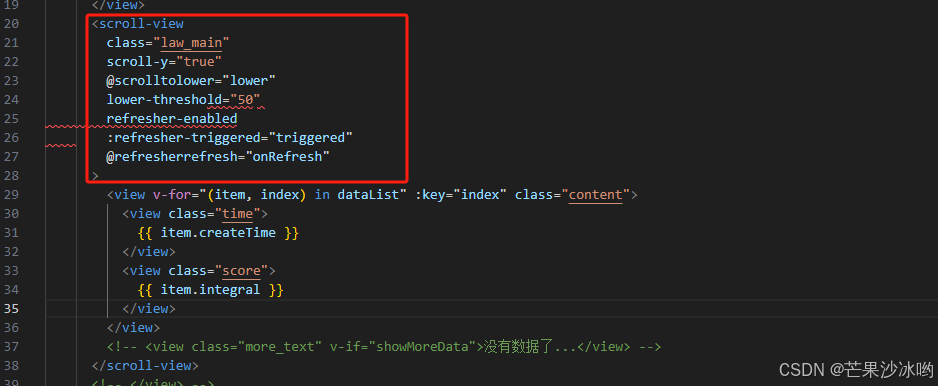
<scroll-view
class="law_main"
scroll-y="true"
@scrolltolower="lower"
lower-threshold="50"
refresher-enabled
:refresher-triggered="triggered"
@refresherrefresh="onRefresh"
>
<view v-for="(item, index) in dataList" :key="index" class="content">
<view class="time">
{{ item.createTime }}
</view>
<view class="score">
{{ item.integral }}
</view>
</view>
<!-- <view class="more_text" v-if="showMoreData">没有数据了...</view> -->
</scroll-view>
<script>
import { exam } from '@/api/index.js'
export default {
data() {
return {
dataList: [],
pageNum: 1,
pageSize: 20,
totalPage: 1,
triggered: false,
isfreshing: false,
showMoreData: false,
title: '暂无数据',
}
},
onShow() {
this.getData()
},
methods: {
lower(e) {
if (this.pageNum < this.totalPage) {
this.pageNum += 1
this.getData()
}
},
onRefresh() {
if (!this.triggered) {
if (this.isfreshing) return
this.isfreshing = true
if (!this.triggered) {
this.triggered = true
}
this.showMoreData = false
this.emptyData = false
this.dataList = []
this.pageNum = 1
this.getData()
}
},
getData() {
let params = {
current: this.pageNum,
size: this.pageSize,
}
uni.showLoading({
title: '正在加载',
})
try {
exam.answerPaperUserPaperList(params).then((res) => {
if (this.pageNum == 1) {
this.dataList = res.data.records
this.triggered = false
this.isfreshing = false
} else {
this.dataList = this.dataList.concat(res.data.records)
}
// if (this.dataList.length == res.data.total && this.dataList.length > 20) {
// this.showMoreData = true;
// }
res.data.total == this.pageSize
? (this.totalPage = 1)
: (this.totalPage = parseInt(res.data.total / this.pageSize + 1))
if (!this.dataList.length) {
this.emptyData = true
this.showMoreData = false
}
uni.hideLoading()
})
} catch (error) {
uni.hideLoading()
}
},
},
onUnload() {},
}
</script>
tips:使用scroll-view下拉加载更多时,需要给一个高度,否则下拉加载将不生效
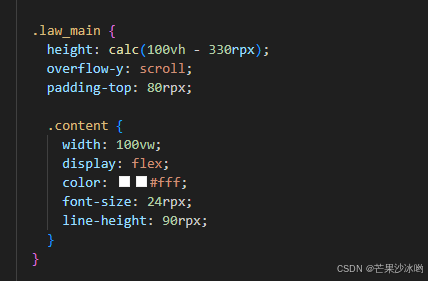
4.uniapp监听页面触底加载更多onReachBottom
<script>
import { articlePageListApi } from '@/service/newpage.js'
import qs from 'qs'
export default {
data() {
return {
params: {
page: 1,
pageSize: 10,
},
status: 'loadmore',// loadmore/loading / nomore
artlist: [],
hasmore: true,
}
},
onLoad() {
this.params.page = 1
this.artlist = []
this.hasmore = true
this.getMylist()
},
//关键代码下拉刷新加载更多
onReachBottom() {
this.status = 'loading'
if (this.hasmore) {
this.status = 'loading'
this.params.page++
this.getMylist()
} else {
let timmer = setTimeout(() => {
this.status = 'nomore'
}, 1000)
}
},
methods: {
getMylist() {
articlePageListApi(
qs.stringify({
...this.params,
}),
).then((res) => {
if (res.data) {
const { list } = res.data
if (this.artlist?.length < list.total) {
this.hasmore = true
this.status = 'loadmore'
} else {
this.hasmore = false
this.status = 'nomore'
}
this.artlist = [...this.artlist, ...list.records]
}
})
},
},
}
</script>