介绍
SpringData是Spring中数据操作的模块,包含对各种数据库的集成,其中redis的集成模块就叫做SpringDataRedis。
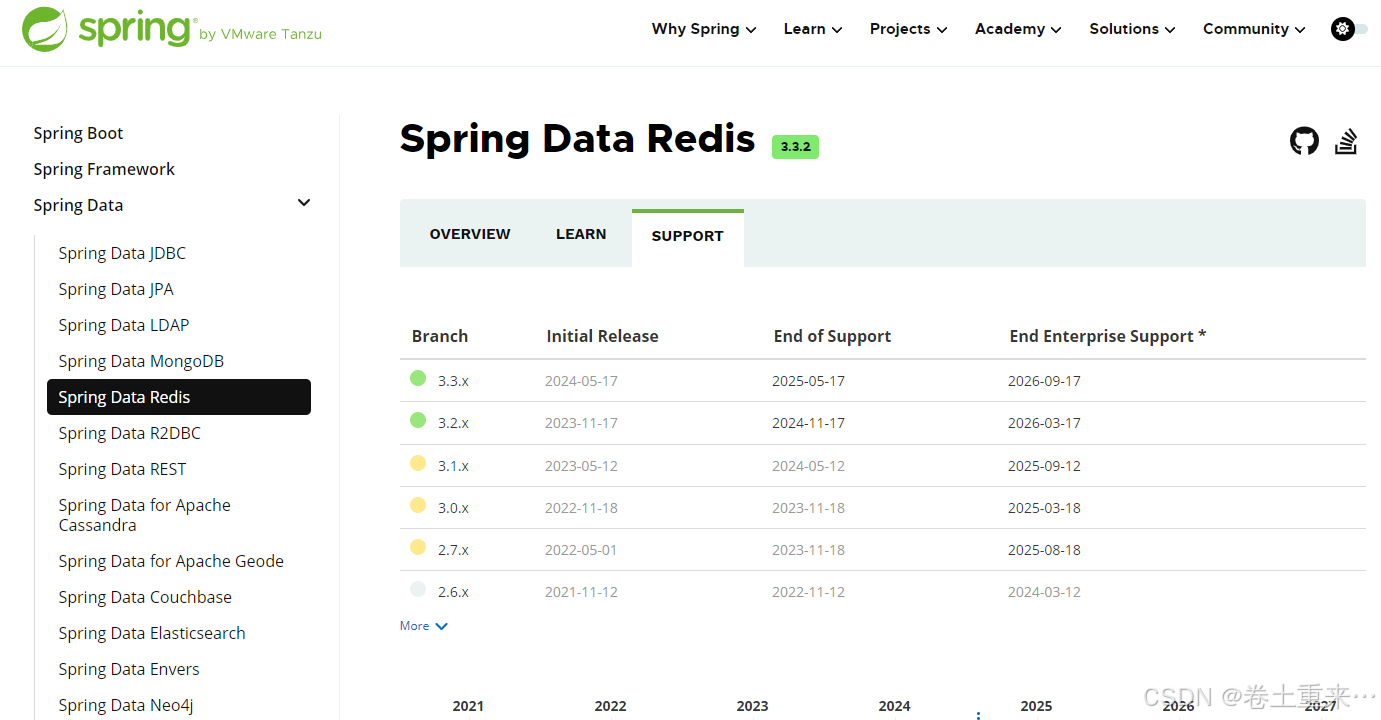
spring的思想从来都不是重新生产,而是整合其他技术。
SpringDataRedis的特点
1.提供了对不同redis客户端的整合(jedis,lettuce)
2.提供了RedisTemplate统一api来操作redis
3.支持redis的发布订阅模型
4.支持redis的哨兵和redis集群
5.支持基于lettuce的响应式编程
6.支持基于jdk,json,字符串,spring对象的数据序列化和反序列化
7.支持基于redis的JDKCollection实现
redisTemplate的操作方法
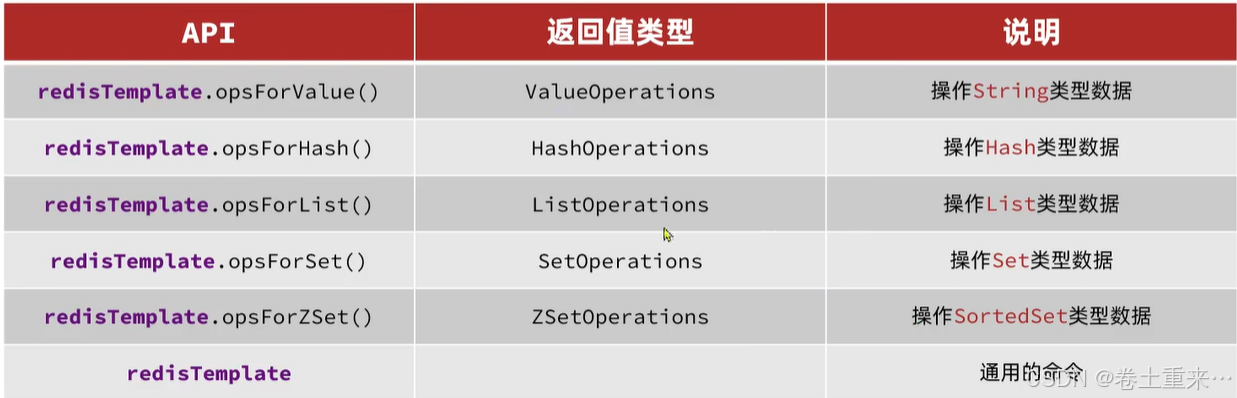
基于SpringBoot整合SpringDataRedis
1.引入依赖
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency>
<!--连接池依赖,redis使用连接池--> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-pool2</artifactId> </dependency>
2.配置文件
spring: redis: host: 192.168.171.130 port: 6379 password: 123456 lettuce: pool: max-idle: 8 # 最大空闲连接 max-active: 8 # 最大连接 min-idle: 0 # 最小空闲连接 max-wait: 100ms # 连接等待时间
这里引入的是lettuce的连接池,因为springBootDataRedis默认使用的是lettuce连接池。但是如果向使用jedis的连接池,就需要再引入jedis的依赖,同时配置文件也要使用jedis连接池的配置。
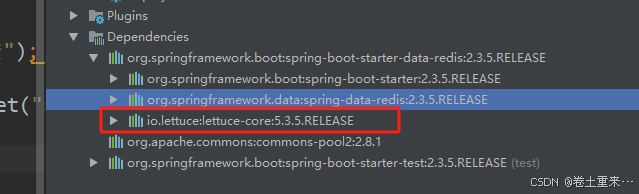
3.使用
java
package com.xkj.org;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
@SpringBootTest
public class SpringBootDataRedisTest {
@Autowired
private RedisTemplate redisTemplate;
@Test
void testString() {
//插入一条String类型的数据
redisTemplate.opsForValue().set("name", "张安");
//读取一条String类型的数据
Object value = redisTemplate.opsForValue().get("name");
System.out.println("value="+value);
}
}
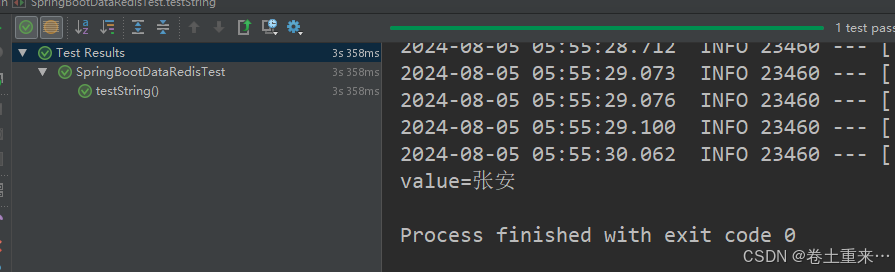
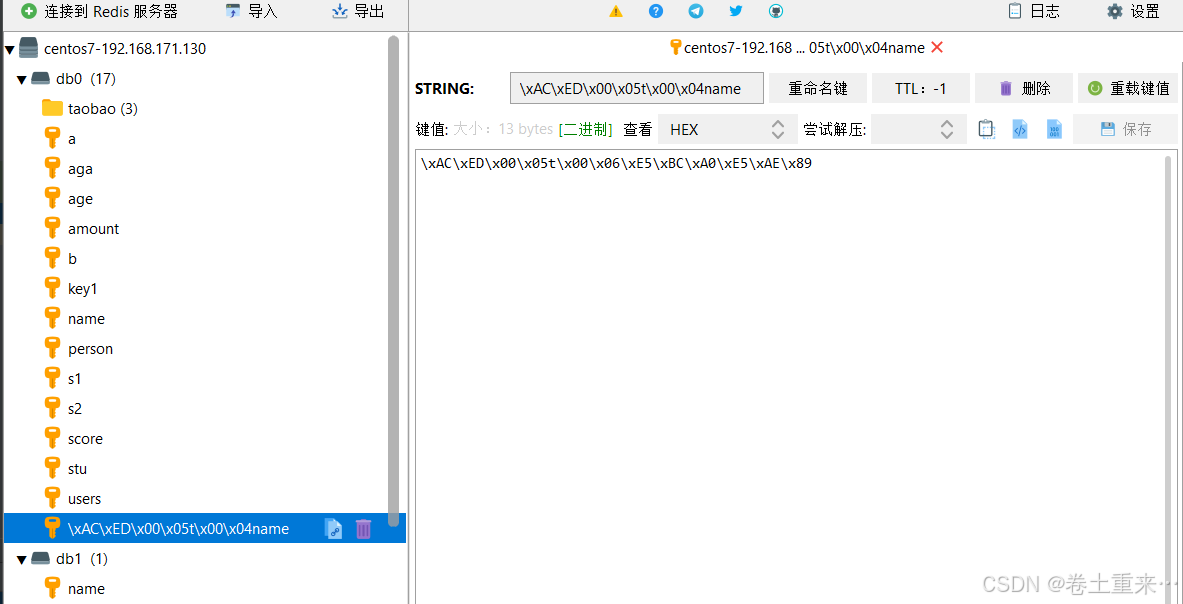
**注意:**可以看出name和value都被序列化了。默认采用了jdk的序列化。
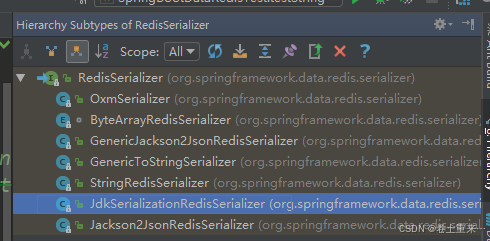
缺点:
1.可读性差
2.内存占用大
需要改变redis的序列化方式,
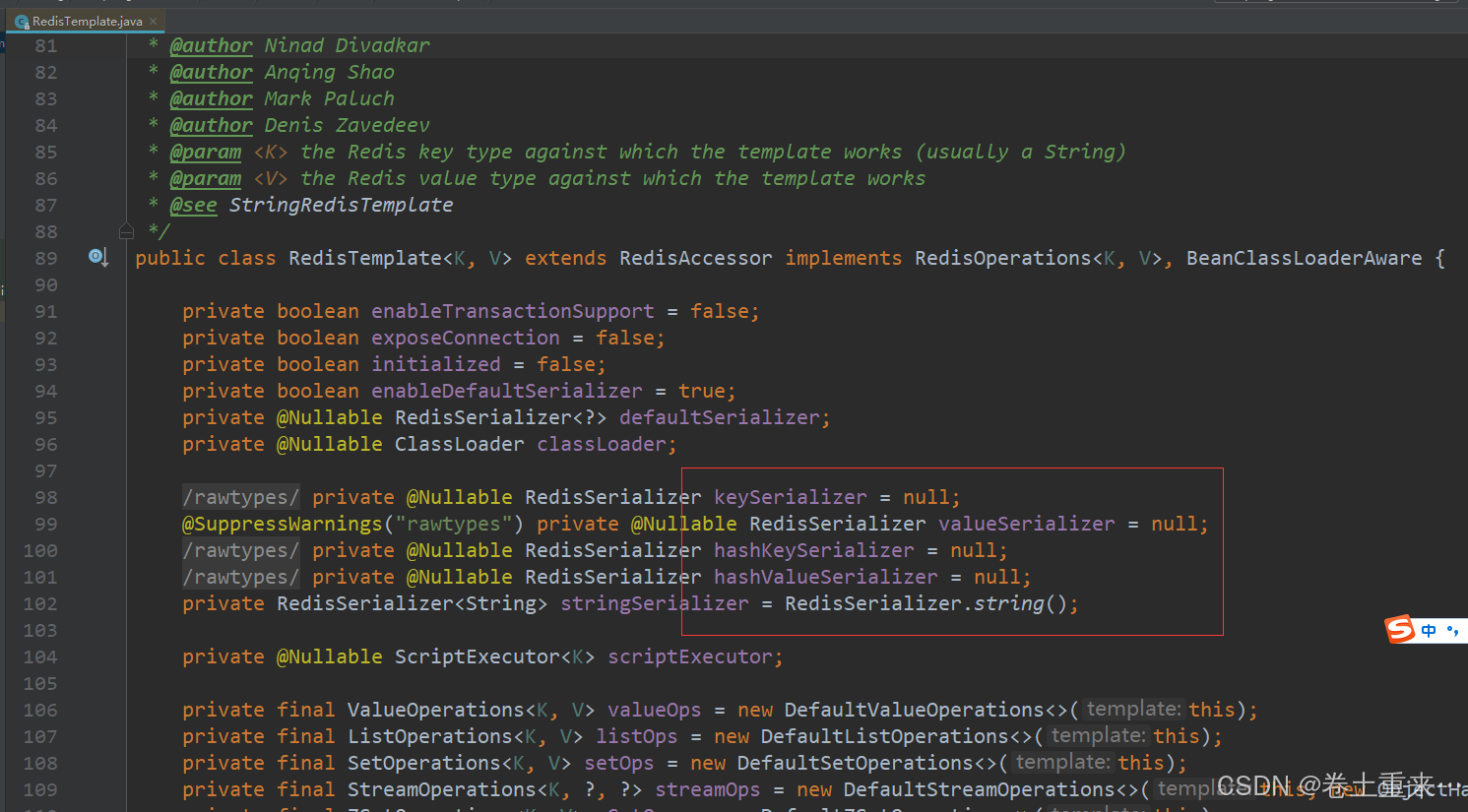
推荐使用
StringRedisSerializer来序列化字符串key。
GenericJackson2JsonRedisSerializer来序列化value对象,转成json字符串。
RedisTemplate配置类
java
package com.xkj.org.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.RedisSerializer;
@Configuration
public class RedisConfig {
/**
* redis连接工厂对象会由spring自动创建
* @param redisConnectionFactory
* @return
*/
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
//1.创建RedisTemplate对象
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
//2.设置连接工厂
redisTemplate.setConnectionFactory(redisConnectionFactory);
//3.创建json序列化工具
GenericJackson2JsonRedisSerializer genericJackson2JsonRedisSerializer = new GenericJackson2JsonRedisSerializer();
//4.设置key的序列化
redisTemplate.setKeySerializer(RedisSerializer.string());
redisTemplate.setHashKeySerializer(RedisSerializer.string());
//5.设置value的序列化
redisTemplate.setValueSerializer(genericJackson2JsonRedisSerializer);
redisTemplate.setHashValueSerializer(genericJackson2JsonRedisSerializer);
//6.返回
return redisTemplate;
}
}
测试类
java
package com.xkj.org;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
@SpringBootTest
public class SpringBootDataRedisTest {
@Autowired
private RedisTemplate<String, Object> redisTemplate;
@Test
void testString() {
//插入一条String类型的数据
redisTemplate.opsForValue().set("name", "张安");
//读取一条String类型的数据
Object value = redisTemplate.opsForValue().get("name");
System.out.println("value="+value);
}
}
如果测试过程中报错:

是因为使用了jackson,没有引入对应的依赖。
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> </dependency>
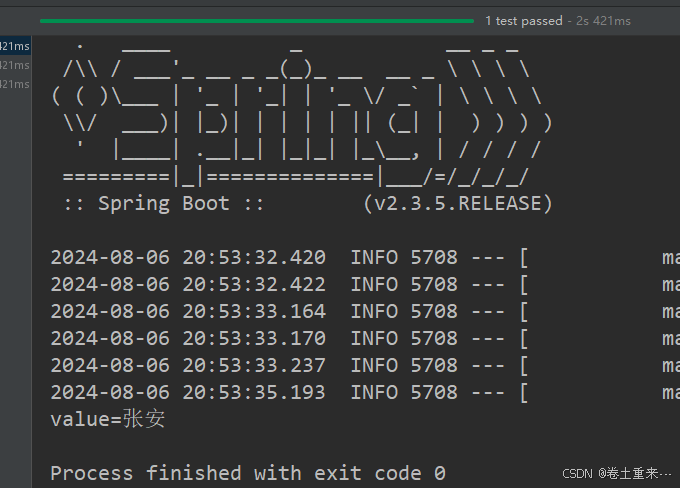
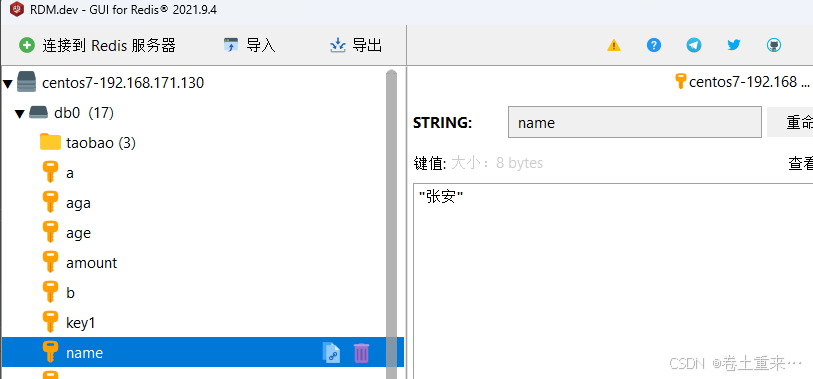
value存入java对象
java
@Test
void testStudent() {
redisTemplate.opsForValue().set("student:100", new Student("小明", 22));
Student student = (Student) redisTemplate.opsForValue().get("student:100");
System.out.println("student="+student);
}
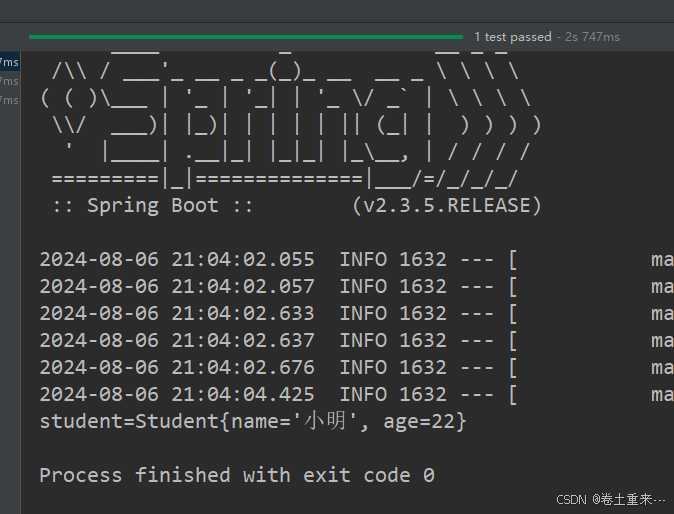
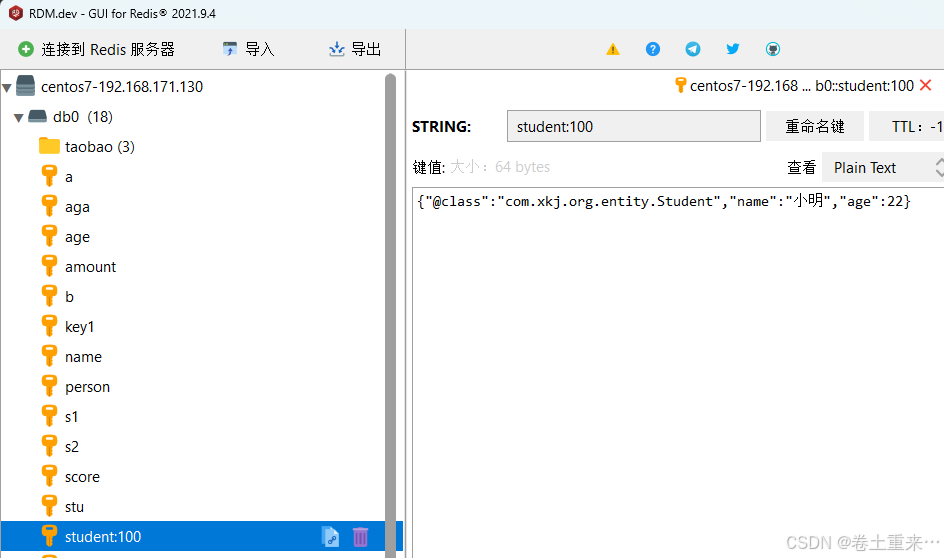