在Vue 3中通过自定义指令实现数字的动态增加动画,可以利用Vue的自定义指令功能,这允许你扩展Vue的内置指令,使得DOM操作更加灵活和强大。以下是如何创建一个自定义指令来实现数字动态增加动画的步骤:
效果演示
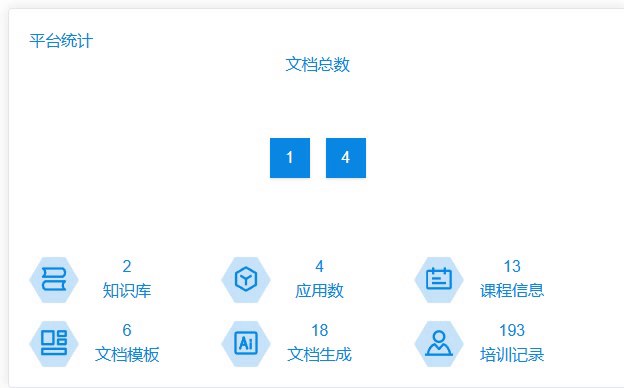
代码实现
1、定义指令代码
ts
import { Directive } from 'vue';
// 动画持续时间,ms
const DURATION = 1500;
/**
* @description 基于Vue3自定义指令,实现数字递增动画效果
*
* @example `<div v-increase="100"></div>`
*/
export const increase: Directive = {
// 在绑定元素的父组件
// 及他自己的所有子节点都挂载完成后调用
mounted(el, binding) {
const { value: maxCount } = binding;
el.$animation = animate((progress) => {
el.innerText = Math.floor(maxCount * progress);
}, DURATION);
},
// 绑定元素的父组件卸载后调用
unmounted(el) {
el.$animation.cancel();
},
};
/**
* @description 基于requestAnimationFrame,实现在持续时间内执行动画的方法
*
* @param fn 动画执行函数,参数progress表示完成的进度
* @param duration 动画持续时间
* @returns {object.cancel} 取消动画
*/
export const animate = function (fn: (progress: number) => void, duration: number) {
const animate = () => {
animation = requestAnimationFrame(animate);
const now = new Date().getTime();
const progress = Math.floor(((now - START) / duration) * 100) / 100;
fn(progress > 1 ? 1 : progress);
// 到达持续时间,结束动画
if (now - START > duration) {
cancel();
}
};
const cancel = () => {
cancelAnimationFrame(animation);
};
const START = new Date().getTime();
let animation = requestAnimationFrame(animate);
return {
cancel: () => {
cancelAnimationFrame(animation);
},
};
};
2、注册自定义指令
ts
import { type App } from 'vue';
import { increase } from './increase';
/** 挂载自定义指令 */
export function loadDirectives(app: App) {
app.directive('increase', increase);
}
3、加载自定义指令
main.ts
ts
import { loadDirectives } from '@/directives';
/** 加载自定义指令 */
loadDirectives(app);