1、加依赖
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.16</version>
</dependency>
2、写接口,这块不需要登录成功才能操作的,所以写controller就行了,不涉及服务
package com.hmblogs.backend.controller;
import cn.hutool.captcha.CaptchaUtil;
import cn.hutool.captcha.LineCaptcha;
import com.hmblogs.backend.config.CaptchaProperties;
import com.hmblogs.backend.util.JedisUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import redis.clients.jedis.Jedis;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
@RestController
@RequestMapping("/captcha")
public class CaptchaController {
@Autowired
private CaptchaProperties captchaProp;
@RequestMapping("/get")
public void getCaptcha(HttpServletRequest request, HttpServletResponse response, HttpSession session) {
// 定义图形验证码的长和宽(配置默认值)
LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(captchaProp.getWidth(), captchaProp.getHeight());
// 细节问题,不影响程序
// 设置返回类型
response.setContentType("image/jpeg");
// 静止缓存
response.setHeader("Progma", "No-cache");
try {
// 图形验证码写出,可以写出到文件,也可以写出到流
lineCaptcha.write(response.getOutputStream());
// 这里是缓存图形验证码逻辑,也可以放库里,或者session里,但要用用户名区别,因为还没登录所以不要使用用户ID区别
// 在相关功能(例如登录)的时候,要对应验证判断一下
Jedis jedis = JedisUtil.getJedisConn();
String username = request.getParameter("username");
jedis.setex("imageToken:"+username,2*60, lineCaptcha.getCode());
// 关流
response.getOutputStream().close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
3、对应的配置文件
package com.hmblogs.backend.config;
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
@Data
@Configuration
@ConfigurationProperties(prefix = "captcha")
public class CaptchaProperties {
private Integer width;
private Integer height;
}
4、对应的配置文件的配置
captcha:
width: 200
height: 100
5、开发页面,使用的是vue的ref来控制页面图形验证码区域显示,点击图片区域则会换一个图形验证码
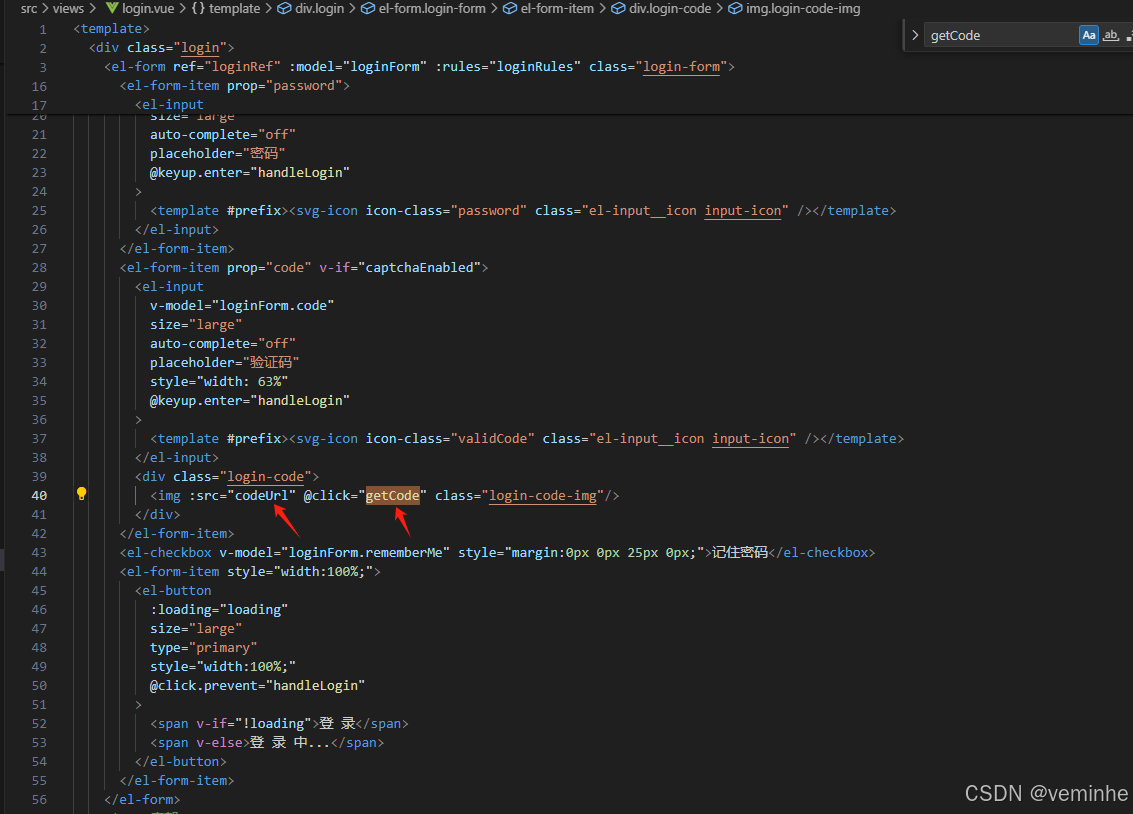
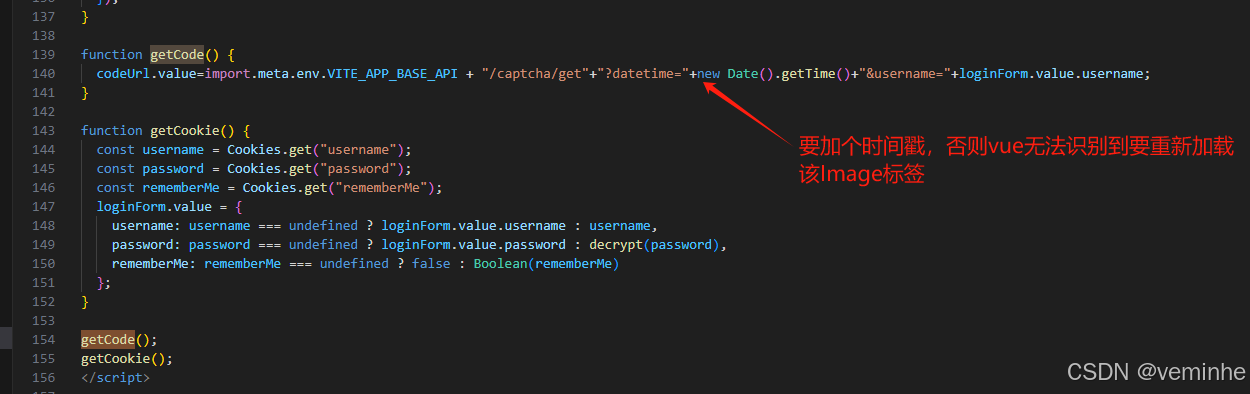
6、验证
页面加载时,有调用该接口
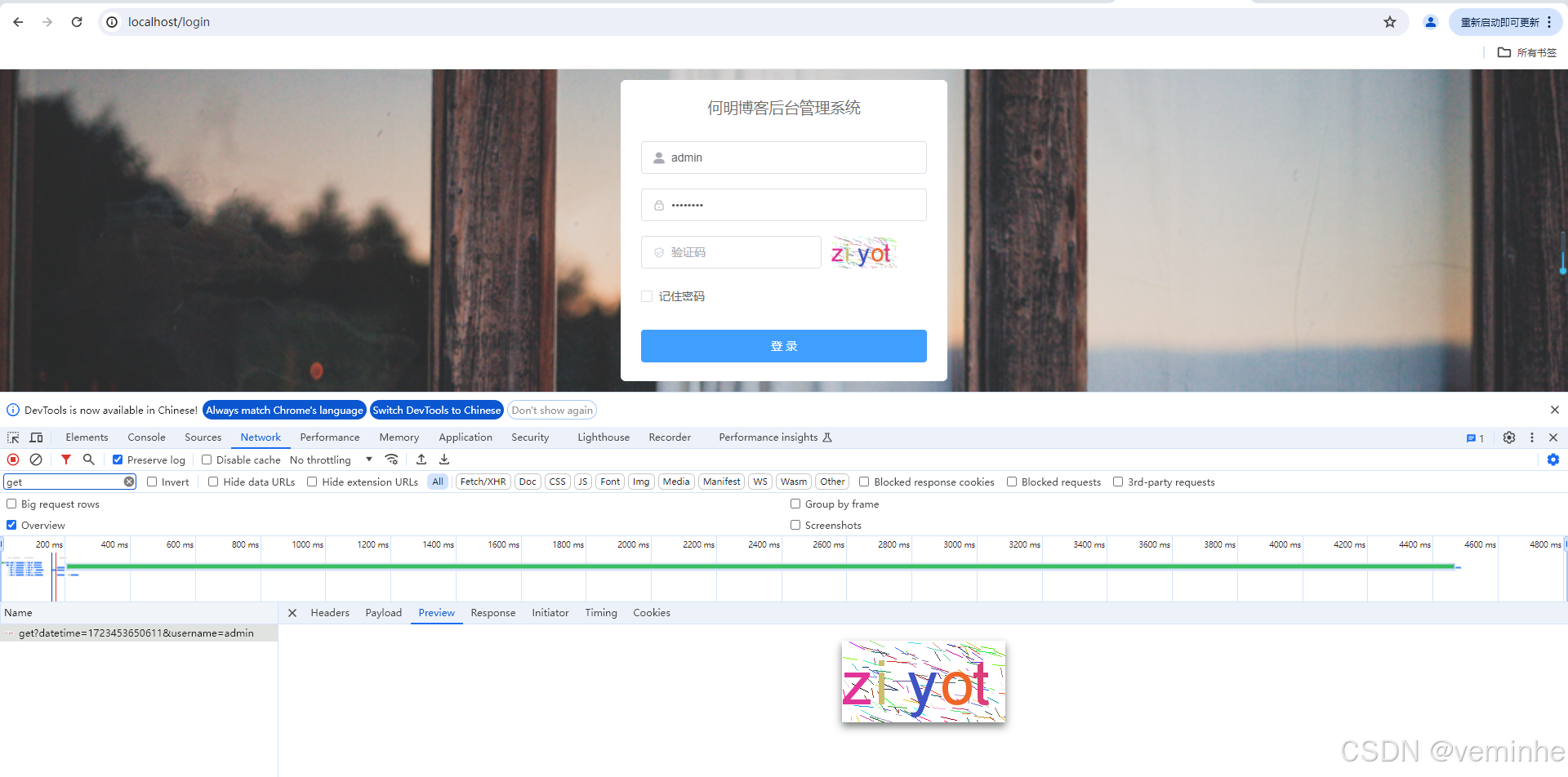
查看redis的缓存,和页面看到的一致
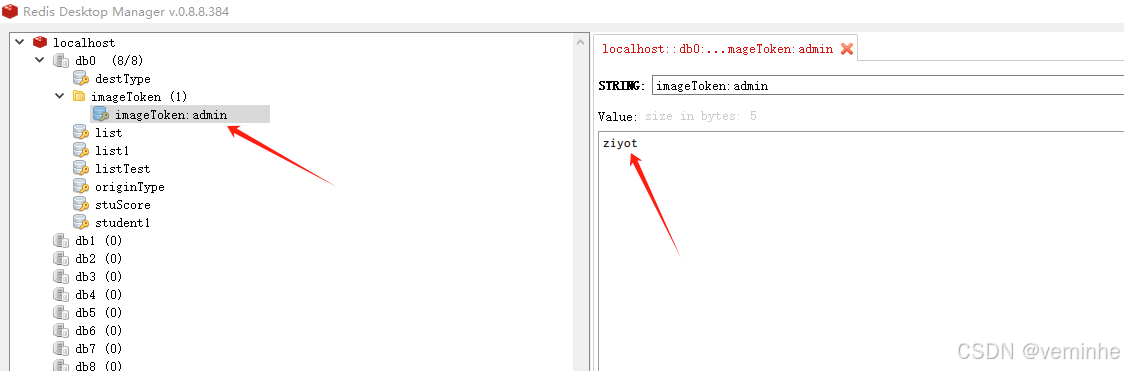
点击图形区域,发现又调用了该接口
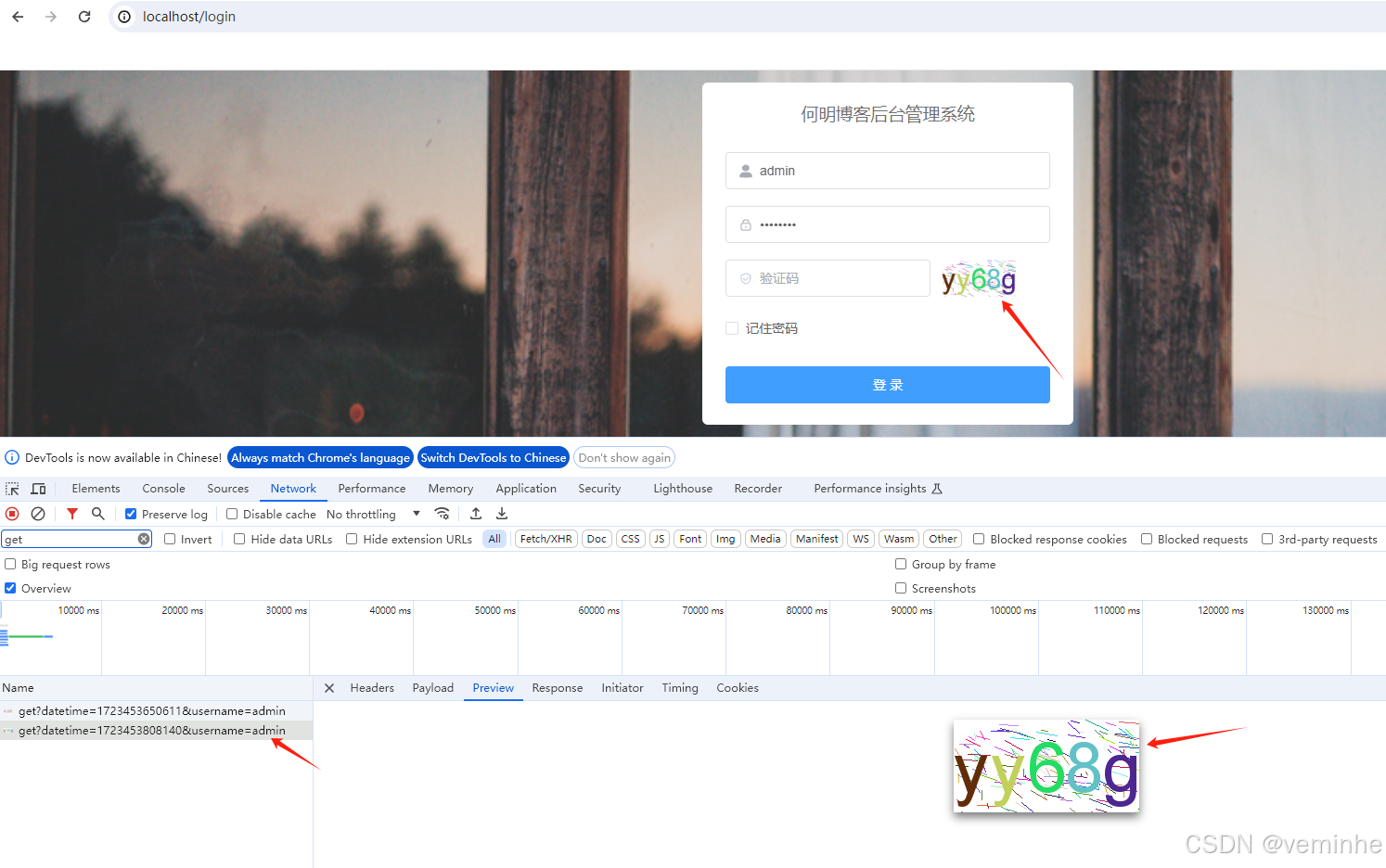
查看redis缓存
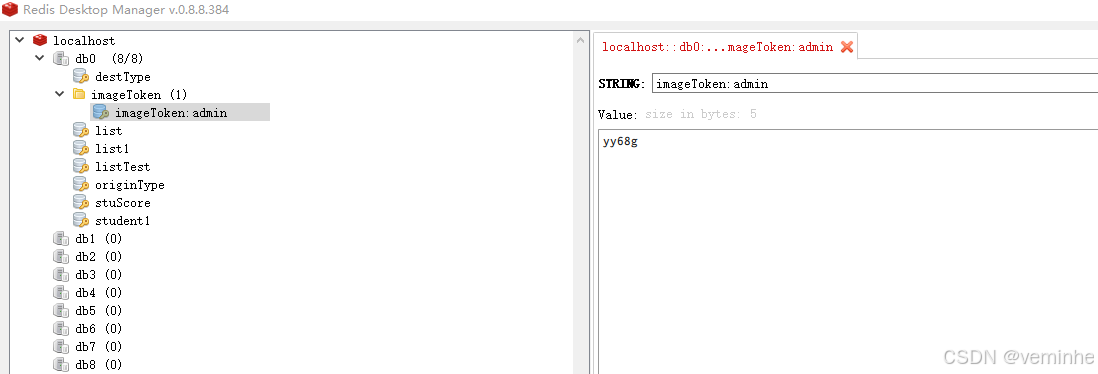
验证完毕。